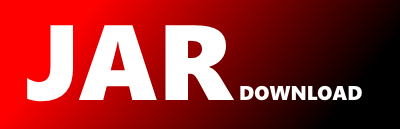
software.amazon.awscdk.services.appstream.CfnUser Maven / Gradle / Ivy
Show all versions of appstream Show documentation
package software.amazon.awscdk.services.appstream;
/**
* A CloudFormation `AWS::AppStream::User`.
*
* The AWS::AppStream::User
resource creates a new user in the AppStream 2.0 user pool.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.appstream.*;
* CfnUser cfnUser = CfnUser.Builder.create(this, "MyCfnUser")
* .authenticationType("authenticationType")
* .userName("userName")
* // the properties below are optional
* .firstName("firstName")
* .lastName("lastName")
* .messageAction("messageAction")
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.50.0 (build d1830a4)", date = "2022-01-09T19:25:18.719Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.appstream.$Module.class, fqn = "@aws-cdk/aws-appstream.CfnUser")
public class CfnUser extends software.amazon.awscdk.core.CfnResource implements software.amazon.awscdk.core.IInspectable {
protected CfnUser(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected CfnUser(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
static {
CFN_RESOURCE_TYPE_NAME = software.amazon.jsii.JsiiObject.jsiiStaticGet(software.amazon.awscdk.services.appstream.CfnUser.class, "CFN_RESOURCE_TYPE_NAME", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Create a new `AWS::AppStream::User`.
*
* @param scope - scope in which this resource is defined. This parameter is required.
* @param id - scoped id of the resource. This parameter is required.
* @param props - resource properties. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public CfnUser(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.appstream.CfnUserProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* Examines the CloudFormation resource and discloses attributes.
*
* @param inspector - tree inspector to collect and process attributes. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public void inspect(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.TreeInspector inspector) {
software.amazon.jsii.Kernel.call(this, "inspect", software.amazon.jsii.NativeType.VOID, new Object[] { java.util.Objects.requireNonNull(inspector, "inspector is required") });
}
/**
* @param props This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
protected @org.jetbrains.annotations.NotNull java.util.Map renderProperties(final @org.jetbrains.annotations.NotNull java.util.Map props) {
return java.util.Collections.unmodifiableMap(software.amazon.jsii.Kernel.call(this, "renderProperties", software.amazon.jsii.NativeType.mapOf(software.amazon.jsii.NativeType.forClass(java.lang.Object.class)), new Object[] { java.util.Objects.requireNonNull(props, "props is required") }));
}
/**
* The CloudFormation resource type name for this resource class.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public final static java.lang.String CFN_RESOURCE_TYPE_NAME;
/**
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
protected @org.jetbrains.annotations.NotNull java.util.Map getCfnProperties() {
return java.util.Collections.unmodifiableMap(software.amazon.jsii.Kernel.get(this, "cfnProperties", software.amazon.jsii.NativeType.mapOf(software.amazon.jsii.NativeType.forClass(java.lang.Object.class))));
}
/**
* The authentication type for the user.
*
* You must specify USERPOOL.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getAuthenticationType() {
return software.amazon.jsii.Kernel.get(this, "authenticationType", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The authentication type for the user.
*
* You must specify USERPOOL.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setAuthenticationType(final @org.jetbrains.annotations.NotNull java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "authenticationType", java.util.Objects.requireNonNull(value, "authenticationType is required"));
}
/**
* The email address of the user.
*
* Users' email addresses are case-sensitive. During login, if they specify an email address that doesn't use the same capitalization as the email address specified when their user pool account was created, a "user does not exist" error message displays.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getUserName() {
return software.amazon.jsii.Kernel.get(this, "userName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The email address of the user.
*
* Users' email addresses are case-sensitive. During login, if they specify an email address that doesn't use the same capitalization as the email address specified when their user pool account was created, a "user does not exist" error message displays.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setUserName(final @org.jetbrains.annotations.NotNull java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "userName", java.util.Objects.requireNonNull(value, "userName is required"));
}
/**
* The first name, or given name, of the user.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.String getFirstName() {
return software.amazon.jsii.Kernel.get(this, "firstName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The first name, or given name, of the user.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setFirstName(final @org.jetbrains.annotations.Nullable java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "firstName", value);
}
/**
* The last name, or surname, of the user.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.String getLastName() {
return software.amazon.jsii.Kernel.get(this, "lastName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The last name, or surname, of the user.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setLastName(final @org.jetbrains.annotations.Nullable java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "lastName", value);
}
/**
* The action to take for the welcome email that is sent to a user after the user is created in the user pool.
*
* If you specify SUPPRESS, no email is sent. If you specify RESEND, do not specify the first name or last name of the user. If the value is null, the email is sent.
*
*
*
* The temporary password in the welcome email is valid for only 7 days. If users don’t set their passwords within 7 days, you must send them a new welcome email.
*
*
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.String getMessageAction() {
return software.amazon.jsii.Kernel.get(this, "messageAction", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The action to take for the welcome email that is sent to a user after the user is created in the user pool.
*
* If you specify SUPPRESS, no email is sent. If you specify RESEND, do not specify the first name or last name of the user. If the value is null, the email is sent.
*
*
*
* The temporary password in the welcome email is valid for only 7 days. If users don’t set their passwords within 7 days, you must send them a new welcome email.
*
*
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setMessageAction(final @org.jetbrains.annotations.Nullable java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "messageAction", value);
}
/**
* A fluent builder for {@link software.amazon.awscdk.services.appstream.CfnUser}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
* @param scope - scope in which this resource is defined. This parameter is required.
* @param id - scoped id of the resource. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static Builder create(final software.amazon.awscdk.core.Construct scope, final java.lang.String id) {
return new Builder(scope, id);
}
private final software.amazon.awscdk.core.Construct scope;
private final java.lang.String id;
private final software.amazon.awscdk.services.appstream.CfnUserProps.Builder props;
private Builder(final software.amazon.awscdk.core.Construct scope, final java.lang.String id) {
this.scope = scope;
this.id = id;
this.props = new software.amazon.awscdk.services.appstream.CfnUserProps.Builder();
}
/**
* The authentication type for the user.
*
* You must specify USERPOOL.
*
* @return {@code this}
* @param authenticationType The authentication type for the user. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder authenticationType(final java.lang.String authenticationType) {
this.props.authenticationType(authenticationType);
return this;
}
/**
* The email address of the user.
*
* Users' email addresses are case-sensitive. During login, if they specify an email address that doesn't use the same capitalization as the email address specified when their user pool account was created, a "user does not exist" error message displays.
*
* @return {@code this}
* @param userName The email address of the user. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder userName(final java.lang.String userName) {
this.props.userName(userName);
return this;
}
/**
* The first name, or given name, of the user.
*
* @return {@code this}
* @param firstName The first name, or given name, of the user. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder firstName(final java.lang.String firstName) {
this.props.firstName(firstName);
return this;
}
/**
* The last name, or surname, of the user.
*
* @return {@code this}
* @param lastName The last name, or surname, of the user. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder lastName(final java.lang.String lastName) {
this.props.lastName(lastName);
return this;
}
/**
* The action to take for the welcome email that is sent to a user after the user is created in the user pool.
*
* If you specify SUPPRESS, no email is sent. If you specify RESEND, do not specify the first name or last name of the user. If the value is null, the email is sent.
*
*
*
* The temporary password in the welcome email is valid for only 7 days. If users don’t set their passwords within 7 days, you must send them a new welcome email.
*
*
*
* @return {@code this}
* @param messageAction The action to take for the welcome email that is sent to a user after the user is created in the user pool. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder messageAction(final java.lang.String messageAction) {
this.props.messageAction(messageAction);
return this;
}
/**
* @returns a newly built instance of {@link software.amazon.awscdk.services.appstream.CfnUser}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public software.amazon.awscdk.services.appstream.CfnUser build() {
return new software.amazon.awscdk.services.appstream.CfnUser(
this.scope,
this.id,
this.props.build()
);
}
}
}