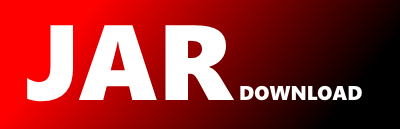
software.amazon.awscdk.services.appstream.CfnImageBuilderProps Maven / Gradle / Ivy
Show all versions of appstream Show documentation
package software.amazon.awscdk.services.appstream;
/**
* Properties for defining a `CfnImageBuilder`.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.appstream.*;
* CfnImageBuilderProps cfnImageBuilderProps = CfnImageBuilderProps.builder()
* .instanceType("instanceType")
* .name("name")
* // the properties below are optional
* .accessEndpoints(List.of(AccessEndpointProperty.builder()
* .endpointType("endpointType")
* .vpceId("vpceId")
* .build()))
* .appstreamAgentVersion("appstreamAgentVersion")
* .description("description")
* .displayName("displayName")
* .domainJoinInfo(DomainJoinInfoProperty.builder()
* .directoryName("directoryName")
* .organizationalUnitDistinguishedName("organizationalUnitDistinguishedName")
* .build())
* .enableDefaultInternetAccess(false)
* .iamRoleArn("iamRoleArn")
* .imageArn("imageArn")
* .imageName("imageName")
* .tags(List.of(CfnTag.builder()
* .key("key")
* .value("value")
* .build()))
* .vpcConfig(VpcConfigProperty.builder()
* .securityGroupIds(List.of("securityGroupIds"))
* .subnetIds(List.of("subnetIds"))
* .build())
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.54.0 (build b1b977a)", date = "2022-03-17T12:21:36.662Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.appstream.$Module.class, fqn = "@aws-cdk/aws-appstream.CfnImageBuilderProps")
@software.amazon.jsii.Jsii.Proxy(CfnImageBuilderProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface CfnImageBuilderProps extends software.amazon.jsii.JsiiSerializable {
/**
* The instance type to use when launching the image builder. The following instance types are available:.
*
*
* - stream.standard.small
* - stream.standard.medium
* - stream.standard.large
* - stream.compute.large
* - stream.compute.xlarge
* - stream.compute.2xlarge
* - stream.compute.4xlarge
* - stream.compute.8xlarge
* - stream.memory.large
* - stream.memory.xlarge
* - stream.memory.2xlarge
* - stream.memory.4xlarge
* - stream.memory.8xlarge
* - stream.memory.z1d.large
* - stream.memory.z1d.xlarge
* - stream.memory.z1d.2xlarge
* - stream.memory.z1d.3xlarge
* - stream.memory.z1d.6xlarge
* - stream.memory.z1d.12xlarge
* - stream.graphics-design.large
* - stream.graphics-design.xlarge
* - stream.graphics-design.2xlarge
* - stream.graphics-design.4xlarge
* - stream.graphics-desktop.2xlarge
* - stream.graphics.g4dn.xlarge
* - stream.graphics.g4dn.2xlarge
* - stream.graphics.g4dn.4xlarge
* - stream.graphics.g4dn.8xlarge
* - stream.graphics.g4dn.12xlarge
* - stream.graphics.g4dn.16xlarge
* - stream.graphics-pro.4xlarge
* - stream.graphics-pro.8xlarge
* - stream.graphics-pro.16xlarge
*
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getInstanceType();
/**
* A unique name for the image builder.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getName();
/**
* The list of virtual private cloud (VPC) interface endpoint objects.
*
* Administrators can connect to the image builder only through the specified endpoints.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getAccessEndpoints() {
return null;
}
/**
* The version of the AppStream 2.0 agent to use for this image builder. To use the latest version of the AppStream 2.0 agent, specify [LATEST].
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getAppstreamAgentVersion() {
return null;
}
/**
* The description to display.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getDescription() {
return null;
}
/**
* The image builder name to display.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getDisplayName() {
return null;
}
/**
* The name of the directory and organizational unit (OU) to use to join the image builder to a Microsoft Active Directory domain.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getDomainJoinInfo() {
return null;
}
/**
* Enables or disables default internet access for the image builder.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getEnableDefaultInternetAccess() {
return null;
}
/**
* The ARN of the IAM role that is applied to the image builder.
*
* To assume a role, the image builder calls the AWS Security Token Service AssumeRole
API operation and passes the ARN of the role to use. The operation creates a new session with temporary credentials. AppStream 2.0 retrieves the temporary credentials and creates the appstream_machine_role credential profile on the instance.
*
* For more information, see Using an IAM Role to Grant Permissions to Applications and Scripts Running on AppStream 2.0 Streaming Instances in the Amazon AppStream 2.0 Administration Guide .
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getIamRoleArn() {
return null;
}
/**
* The ARN of the public, private, or shared image to use.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getImageArn() {
return null;
}
/**
* The name of the image used to create the image builder.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getImageName() {
return null;
}
/**
* An array of key-value pairs.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getTags() {
return null;
}
/**
* The VPC configuration for the image builder.
*
* You can specify only one subnet.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getVpcConfig() {
return null;
}
/**
* @return a {@link Builder} of {@link CfnImageBuilderProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link CfnImageBuilderProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String instanceType;
java.lang.String name;
java.lang.Object accessEndpoints;
java.lang.String appstreamAgentVersion;
java.lang.String description;
java.lang.String displayName;
java.lang.Object domainJoinInfo;
java.lang.Object enableDefaultInternetAccess;
java.lang.String iamRoleArn;
java.lang.String imageArn;
java.lang.String imageName;
java.util.List tags;
java.lang.Object vpcConfig;
/**
* Sets the value of {@link CfnImageBuilderProps#getInstanceType}
* @param instanceType The instance type to use when launching the image builder. The following instance types are available:. This parameter is required.
*
* - stream.standard.small
* - stream.standard.medium
* - stream.standard.large
* - stream.compute.large
* - stream.compute.xlarge
* - stream.compute.2xlarge
* - stream.compute.4xlarge
* - stream.compute.8xlarge
* - stream.memory.large
* - stream.memory.xlarge
* - stream.memory.2xlarge
* - stream.memory.4xlarge
* - stream.memory.8xlarge
* - stream.memory.z1d.large
* - stream.memory.z1d.xlarge
* - stream.memory.z1d.2xlarge
* - stream.memory.z1d.3xlarge
* - stream.memory.z1d.6xlarge
* - stream.memory.z1d.12xlarge
* - stream.graphics-design.large
* - stream.graphics-design.xlarge
* - stream.graphics-design.2xlarge
* - stream.graphics-design.4xlarge
* - stream.graphics-desktop.2xlarge
* - stream.graphics.g4dn.xlarge
* - stream.graphics.g4dn.2xlarge
* - stream.graphics.g4dn.4xlarge
* - stream.graphics.g4dn.8xlarge
* - stream.graphics.g4dn.12xlarge
* - stream.graphics.g4dn.16xlarge
* - stream.graphics-pro.4xlarge
* - stream.graphics-pro.8xlarge
* - stream.graphics-pro.16xlarge
*
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder instanceType(java.lang.String instanceType) {
this.instanceType = instanceType;
return this;
}
/**
* Sets the value of {@link CfnImageBuilderProps#getName}
* @param name A unique name for the image builder. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder name(java.lang.String name) {
this.name = name;
return this;
}
/**
* Sets the value of {@link CfnImageBuilderProps#getAccessEndpoints}
* @param accessEndpoints The list of virtual private cloud (VPC) interface endpoint objects.
* Administrators can connect to the image builder only through the specified endpoints.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder accessEndpoints(software.amazon.awscdk.core.IResolvable accessEndpoints) {
this.accessEndpoints = accessEndpoints;
return this;
}
/**
* Sets the value of {@link CfnImageBuilderProps#getAccessEndpoints}
* @param accessEndpoints The list of virtual private cloud (VPC) interface endpoint objects.
* Administrators can connect to the image builder only through the specified endpoints.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder accessEndpoints(java.util.List extends java.lang.Object> accessEndpoints) {
this.accessEndpoints = accessEndpoints;
return this;
}
/**
* Sets the value of {@link CfnImageBuilderProps#getAppstreamAgentVersion}
* @param appstreamAgentVersion The version of the AppStream 2.0 agent to use for this image builder. To use the latest version of the AppStream 2.0 agent, specify [LATEST].
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder appstreamAgentVersion(java.lang.String appstreamAgentVersion) {
this.appstreamAgentVersion = appstreamAgentVersion;
return this;
}
/**
* Sets the value of {@link CfnImageBuilderProps#getDescription}
* @param description The description to display.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder description(java.lang.String description) {
this.description = description;
return this;
}
/**
* Sets the value of {@link CfnImageBuilderProps#getDisplayName}
* @param displayName The image builder name to display.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder displayName(java.lang.String displayName) {
this.displayName = displayName;
return this;
}
/**
* Sets the value of {@link CfnImageBuilderProps#getDomainJoinInfo}
* @param domainJoinInfo The name of the directory and organizational unit (OU) to use to join the image builder to a Microsoft Active Directory domain.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder domainJoinInfo(software.amazon.awscdk.core.IResolvable domainJoinInfo) {
this.domainJoinInfo = domainJoinInfo;
return this;
}
/**
* Sets the value of {@link CfnImageBuilderProps#getDomainJoinInfo}
* @param domainJoinInfo The name of the directory and organizational unit (OU) to use to join the image builder to a Microsoft Active Directory domain.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder domainJoinInfo(software.amazon.awscdk.services.appstream.CfnImageBuilder.DomainJoinInfoProperty domainJoinInfo) {
this.domainJoinInfo = domainJoinInfo;
return this;
}
/**
* Sets the value of {@link CfnImageBuilderProps#getEnableDefaultInternetAccess}
* @param enableDefaultInternetAccess Enables or disables default internet access for the image builder.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder enableDefaultInternetAccess(java.lang.Boolean enableDefaultInternetAccess) {
this.enableDefaultInternetAccess = enableDefaultInternetAccess;
return this;
}
/**
* Sets the value of {@link CfnImageBuilderProps#getEnableDefaultInternetAccess}
* @param enableDefaultInternetAccess Enables or disables default internet access for the image builder.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder enableDefaultInternetAccess(software.amazon.awscdk.core.IResolvable enableDefaultInternetAccess) {
this.enableDefaultInternetAccess = enableDefaultInternetAccess;
return this;
}
/**
* Sets the value of {@link CfnImageBuilderProps#getIamRoleArn}
* @param iamRoleArn The ARN of the IAM role that is applied to the image builder.
* To assume a role, the image builder calls the AWS Security Token Service AssumeRole
API operation and passes the ARN of the role to use. The operation creates a new session with temporary credentials. AppStream 2.0 retrieves the temporary credentials and creates the appstream_machine_role credential profile on the instance.
*
* For more information, see Using an IAM Role to Grant Permissions to Applications and Scripts Running on AppStream 2.0 Streaming Instances in the Amazon AppStream 2.0 Administration Guide .
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder iamRoleArn(java.lang.String iamRoleArn) {
this.iamRoleArn = iamRoleArn;
return this;
}
/**
* Sets the value of {@link CfnImageBuilderProps#getImageArn}
* @param imageArn The ARN of the public, private, or shared image to use.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder imageArn(java.lang.String imageArn) {
this.imageArn = imageArn;
return this;
}
/**
* Sets the value of {@link CfnImageBuilderProps#getImageName}
* @param imageName The name of the image used to create the image builder.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder imageName(java.lang.String imageName) {
this.imageName = imageName;
return this;
}
/**
* Sets the value of {@link CfnImageBuilderProps#getTags}
* @param tags An array of key-value pairs.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@SuppressWarnings("unchecked")
public Builder tags(java.util.List extends software.amazon.awscdk.core.CfnTag> tags) {
this.tags = (java.util.List)tags;
return this;
}
/**
* Sets the value of {@link CfnImageBuilderProps#getVpcConfig}
* @param vpcConfig The VPC configuration for the image builder.
* You can specify only one subnet.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder vpcConfig(software.amazon.awscdk.core.IResolvable vpcConfig) {
this.vpcConfig = vpcConfig;
return this;
}
/**
* Sets the value of {@link CfnImageBuilderProps#getVpcConfig}
* @param vpcConfig The VPC configuration for the image builder.
* You can specify only one subnet.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder vpcConfig(software.amazon.awscdk.services.appstream.CfnImageBuilder.VpcConfigProperty vpcConfig) {
this.vpcConfig = vpcConfig;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link CfnImageBuilderProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public CfnImageBuilderProps build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link CfnImageBuilderProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements CfnImageBuilderProps {
private final java.lang.String instanceType;
private final java.lang.String name;
private final java.lang.Object accessEndpoints;
private final java.lang.String appstreamAgentVersion;
private final java.lang.String description;
private final java.lang.String displayName;
private final java.lang.Object domainJoinInfo;
private final java.lang.Object enableDefaultInternetAccess;
private final java.lang.String iamRoleArn;
private final java.lang.String imageArn;
private final java.lang.String imageName;
private final java.util.List tags;
private final java.lang.Object vpcConfig;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.instanceType = software.amazon.jsii.Kernel.get(this, "instanceType", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.name = software.amazon.jsii.Kernel.get(this, "name", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.accessEndpoints = software.amazon.jsii.Kernel.get(this, "accessEndpoints", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.appstreamAgentVersion = software.amazon.jsii.Kernel.get(this, "appstreamAgentVersion", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.description = software.amazon.jsii.Kernel.get(this, "description", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.displayName = software.amazon.jsii.Kernel.get(this, "displayName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.domainJoinInfo = software.amazon.jsii.Kernel.get(this, "domainJoinInfo", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.enableDefaultInternetAccess = software.amazon.jsii.Kernel.get(this, "enableDefaultInternetAccess", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.iamRoleArn = software.amazon.jsii.Kernel.get(this, "iamRoleArn", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.imageArn = software.amazon.jsii.Kernel.get(this, "imageArn", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.imageName = software.amazon.jsii.Kernel.get(this, "imageName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.tags = software.amazon.jsii.Kernel.get(this, "tags", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.CfnTag.class)));
this.vpcConfig = software.amazon.jsii.Kernel.get(this, "vpcConfig", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
@SuppressWarnings("unchecked")
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.instanceType = java.util.Objects.requireNonNull(builder.instanceType, "instanceType is required");
this.name = java.util.Objects.requireNonNull(builder.name, "name is required");
this.accessEndpoints = builder.accessEndpoints;
this.appstreamAgentVersion = builder.appstreamAgentVersion;
this.description = builder.description;
this.displayName = builder.displayName;
this.domainJoinInfo = builder.domainJoinInfo;
this.enableDefaultInternetAccess = builder.enableDefaultInternetAccess;
this.iamRoleArn = builder.iamRoleArn;
this.imageArn = builder.imageArn;
this.imageName = builder.imageName;
this.tags = (java.util.List)builder.tags;
this.vpcConfig = builder.vpcConfig;
}
@Override
public final java.lang.String getInstanceType() {
return this.instanceType;
}
@Override
public final java.lang.String getName() {
return this.name;
}
@Override
public final java.lang.Object getAccessEndpoints() {
return this.accessEndpoints;
}
@Override
public final java.lang.String getAppstreamAgentVersion() {
return this.appstreamAgentVersion;
}
@Override
public final java.lang.String getDescription() {
return this.description;
}
@Override
public final java.lang.String getDisplayName() {
return this.displayName;
}
@Override
public final java.lang.Object getDomainJoinInfo() {
return this.domainJoinInfo;
}
@Override
public final java.lang.Object getEnableDefaultInternetAccess() {
return this.enableDefaultInternetAccess;
}
@Override
public final java.lang.String getIamRoleArn() {
return this.iamRoleArn;
}
@Override
public final java.lang.String getImageArn() {
return this.imageArn;
}
@Override
public final java.lang.String getImageName() {
return this.imageName;
}
@Override
public final java.util.List getTags() {
return this.tags;
}
@Override
public final java.lang.Object getVpcConfig() {
return this.vpcConfig;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("instanceType", om.valueToTree(this.getInstanceType()));
data.set("name", om.valueToTree(this.getName()));
if (this.getAccessEndpoints() != null) {
data.set("accessEndpoints", om.valueToTree(this.getAccessEndpoints()));
}
if (this.getAppstreamAgentVersion() != null) {
data.set("appstreamAgentVersion", om.valueToTree(this.getAppstreamAgentVersion()));
}
if (this.getDescription() != null) {
data.set("description", om.valueToTree(this.getDescription()));
}
if (this.getDisplayName() != null) {
data.set("displayName", om.valueToTree(this.getDisplayName()));
}
if (this.getDomainJoinInfo() != null) {
data.set("domainJoinInfo", om.valueToTree(this.getDomainJoinInfo()));
}
if (this.getEnableDefaultInternetAccess() != null) {
data.set("enableDefaultInternetAccess", om.valueToTree(this.getEnableDefaultInternetAccess()));
}
if (this.getIamRoleArn() != null) {
data.set("iamRoleArn", om.valueToTree(this.getIamRoleArn()));
}
if (this.getImageArn() != null) {
data.set("imageArn", om.valueToTree(this.getImageArn()));
}
if (this.getImageName() != null) {
data.set("imageName", om.valueToTree(this.getImageName()));
}
if (this.getTags() != null) {
data.set("tags", om.valueToTree(this.getTags()));
}
if (this.getVpcConfig() != null) {
data.set("vpcConfig", om.valueToTree(this.getVpcConfig()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-appstream.CfnImageBuilderProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
CfnImageBuilderProps.Jsii$Proxy that = (CfnImageBuilderProps.Jsii$Proxy) o;
if (!instanceType.equals(that.instanceType)) return false;
if (!name.equals(that.name)) return false;
if (this.accessEndpoints != null ? !this.accessEndpoints.equals(that.accessEndpoints) : that.accessEndpoints != null) return false;
if (this.appstreamAgentVersion != null ? !this.appstreamAgentVersion.equals(that.appstreamAgentVersion) : that.appstreamAgentVersion != null) return false;
if (this.description != null ? !this.description.equals(that.description) : that.description != null) return false;
if (this.displayName != null ? !this.displayName.equals(that.displayName) : that.displayName != null) return false;
if (this.domainJoinInfo != null ? !this.domainJoinInfo.equals(that.domainJoinInfo) : that.domainJoinInfo != null) return false;
if (this.enableDefaultInternetAccess != null ? !this.enableDefaultInternetAccess.equals(that.enableDefaultInternetAccess) : that.enableDefaultInternetAccess != null) return false;
if (this.iamRoleArn != null ? !this.iamRoleArn.equals(that.iamRoleArn) : that.iamRoleArn != null) return false;
if (this.imageArn != null ? !this.imageArn.equals(that.imageArn) : that.imageArn != null) return false;
if (this.imageName != null ? !this.imageName.equals(that.imageName) : that.imageName != null) return false;
if (this.tags != null ? !this.tags.equals(that.tags) : that.tags != null) return false;
return this.vpcConfig != null ? this.vpcConfig.equals(that.vpcConfig) : that.vpcConfig == null;
}
@Override
public final int hashCode() {
int result = this.instanceType.hashCode();
result = 31 * result + (this.name.hashCode());
result = 31 * result + (this.accessEndpoints != null ? this.accessEndpoints.hashCode() : 0);
result = 31 * result + (this.appstreamAgentVersion != null ? this.appstreamAgentVersion.hashCode() : 0);
result = 31 * result + (this.description != null ? this.description.hashCode() : 0);
result = 31 * result + (this.displayName != null ? this.displayName.hashCode() : 0);
result = 31 * result + (this.domainJoinInfo != null ? this.domainJoinInfo.hashCode() : 0);
result = 31 * result + (this.enableDefaultInternetAccess != null ? this.enableDefaultInternetAccess.hashCode() : 0);
result = 31 * result + (this.iamRoleArn != null ? this.iamRoleArn.hashCode() : 0);
result = 31 * result + (this.imageArn != null ? this.imageArn.hashCode() : 0);
result = 31 * result + (this.imageName != null ? this.imageName.hashCode() : 0);
result = 31 * result + (this.tags != null ? this.tags.hashCode() : 0);
result = 31 * result + (this.vpcConfig != null ? this.vpcConfig.hashCode() : 0);
return result;
}
}
}