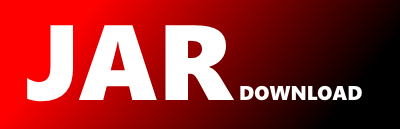
software.amazon.awscdk.services.appstream.CfnStackUserAssociation Maven / Gradle / Ivy
Show all versions of appstream Show documentation
package software.amazon.awscdk.services.appstream;
/**
* A CloudFormation `AWS::AppStream::StackUserAssociation`.
*
* The AWS::AppStream::StackUserAssociation
resource associates the specified users with the specified stacks for Amazon AppStream 2.0. Users in an AppStream 2.0 user pool cannot be assigned to stacks with fleets that are joined to an Active Directory domain.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.appstream.*;
* CfnStackUserAssociation cfnStackUserAssociation = CfnStackUserAssociation.Builder.create(this, "MyCfnStackUserAssociation")
* .authenticationType("authenticationType")
* .stackName("stackName")
* .userName("userName")
* // the properties below are optional
* .sendEmailNotification(false)
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.71.0 (build f1f58ae)", date = "2022-11-29T04:32:14.166Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.appstream.$Module.class, fqn = "@aws-cdk/aws-appstream.CfnStackUserAssociation")
public class CfnStackUserAssociation extends software.amazon.awscdk.core.CfnResource implements software.amazon.awscdk.core.IInspectable {
protected CfnStackUserAssociation(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected CfnStackUserAssociation(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
static {
CFN_RESOURCE_TYPE_NAME = software.amazon.jsii.JsiiObject.jsiiStaticGet(software.amazon.awscdk.services.appstream.CfnStackUserAssociation.class, "CFN_RESOURCE_TYPE_NAME", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Create a new `AWS::AppStream::StackUserAssociation`.
*
* @param scope - scope in which this resource is defined. This parameter is required.
* @param id - scoped id of the resource. This parameter is required.
* @param props - resource properties. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public CfnStackUserAssociation(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.appstream.CfnStackUserAssociationProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* Examines the CloudFormation resource and discloses attributes.
*
* @param inspector - tree inspector to collect and process attributes. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public void inspect(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.TreeInspector inspector) {
software.amazon.jsii.Kernel.call(this, "inspect", software.amazon.jsii.NativeType.VOID, new Object[] { java.util.Objects.requireNonNull(inspector, "inspector is required") });
}
/**
* @param props This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
protected @org.jetbrains.annotations.NotNull java.util.Map renderProperties(final @org.jetbrains.annotations.NotNull java.util.Map props) {
return java.util.Collections.unmodifiableMap(software.amazon.jsii.Kernel.call(this, "renderProperties", software.amazon.jsii.NativeType.mapOf(software.amazon.jsii.NativeType.forClass(java.lang.Object.class)), new Object[] { java.util.Objects.requireNonNull(props, "props is required") }));
}
/**
* The CloudFormation resource type name for this resource class.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public final static java.lang.String CFN_RESOURCE_TYPE_NAME;
/**
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
protected @org.jetbrains.annotations.NotNull java.util.Map getCfnProperties() {
return java.util.Collections.unmodifiableMap(software.amazon.jsii.Kernel.get(this, "cfnProperties", software.amazon.jsii.NativeType.mapOf(software.amazon.jsii.NativeType.forClass(java.lang.Object.class))));
}
/**
* The authentication type for the user who is associated with the stack.
*
* You must specify USERPOOL.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getAuthenticationType() {
return software.amazon.jsii.Kernel.get(this, "authenticationType", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The authentication type for the user who is associated with the stack.
*
* You must specify USERPOOL.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setAuthenticationType(final @org.jetbrains.annotations.NotNull java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "authenticationType", java.util.Objects.requireNonNull(value, "authenticationType is required"));
}
/**
* The name of the stack that is associated with the user.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getStackName() {
return software.amazon.jsii.Kernel.get(this, "stackName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The name of the stack that is associated with the user.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setStackName(final @org.jetbrains.annotations.NotNull java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "stackName", java.util.Objects.requireNonNull(value, "stackName is required"));
}
/**
* The email address of the user who is associated with the stack.
*
*
*
* Users' email addresses are case-sensitive.
*
*
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getUserName() {
return software.amazon.jsii.Kernel.get(this, "userName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* The email address of the user who is associated with the stack.
*
*
*
* Users' email addresses are case-sensitive.
*
*
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setUserName(final @org.jetbrains.annotations.NotNull java.lang.String value) {
software.amazon.jsii.Kernel.set(this, "userName", java.util.Objects.requireNonNull(value, "userName is required"));
}
/**
* Specifies whether a welcome email is sent to a user after the user is created in the user pool.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.Nullable java.lang.Object getSendEmailNotification() {
return software.amazon.jsii.Kernel.get(this, "sendEmailNotification", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* Specifies whether a welcome email is sent to a user after the user is created in the user pool.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setSendEmailNotification(final @org.jetbrains.annotations.Nullable java.lang.Boolean value) {
software.amazon.jsii.Kernel.set(this, "sendEmailNotification", value);
}
/**
* Specifies whether a welcome email is sent to a user after the user is created in the user pool.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public void setSendEmailNotification(final @org.jetbrains.annotations.Nullable software.amazon.awscdk.core.IResolvable value) {
software.amazon.jsii.Kernel.set(this, "sendEmailNotification", value);
}
/**
* A fluent builder for {@link software.amazon.awscdk.services.appstream.CfnStackUserAssociation}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
* @param scope - scope in which this resource is defined. This parameter is required.
* @param id - scoped id of the resource. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static Builder create(final software.amazon.awscdk.core.Construct scope, final java.lang.String id) {
return new Builder(scope, id);
}
private final software.amazon.awscdk.core.Construct scope;
private final java.lang.String id;
private final software.amazon.awscdk.services.appstream.CfnStackUserAssociationProps.Builder props;
private Builder(final software.amazon.awscdk.core.Construct scope, final java.lang.String id) {
this.scope = scope;
this.id = id;
this.props = new software.amazon.awscdk.services.appstream.CfnStackUserAssociationProps.Builder();
}
/**
* The authentication type for the user who is associated with the stack.
*
* You must specify USERPOOL.
*
* @return {@code this}
* @param authenticationType The authentication type for the user who is associated with the stack. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder authenticationType(final java.lang.String authenticationType) {
this.props.authenticationType(authenticationType);
return this;
}
/**
* The name of the stack that is associated with the user.
*
* @return {@code this}
* @param stackName The name of the stack that is associated with the user. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder stackName(final java.lang.String stackName) {
this.props.stackName(stackName);
return this;
}
/**
* The email address of the user who is associated with the stack.
*
*
*
* Users' email addresses are case-sensitive.
*
*
*
* @return {@code this}
* @param userName The email address of the user who is associated with the stack. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder userName(final java.lang.String userName) {
this.props.userName(userName);
return this;
}
/**
* Specifies whether a welcome email is sent to a user after the user is created in the user pool.
*
* @return {@code this}
* @param sendEmailNotification Specifies whether a welcome email is sent to a user after the user is created in the user pool. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder sendEmailNotification(final java.lang.Boolean sendEmailNotification) {
this.props.sendEmailNotification(sendEmailNotification);
return this;
}
/**
* Specifies whether a welcome email is sent to a user after the user is created in the user pool.
*
* @return {@code this}
* @param sendEmailNotification Specifies whether a welcome email is sent to a user after the user is created in the user pool. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder sendEmailNotification(final software.amazon.awscdk.core.IResolvable sendEmailNotification) {
this.props.sendEmailNotification(sendEmailNotification);
return this;
}
/**
* @returns a newly built instance of {@link software.amazon.awscdk.services.appstream.CfnStackUserAssociation}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public software.amazon.awscdk.services.appstream.CfnStackUserAssociation build() {
return new software.amazon.awscdk.services.appstream.CfnStackUserAssociation(
this.scope,
this.id,
this.props.build()
);
}
}
}