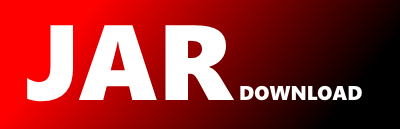
software.amazon.awscdk.assets.docker.DockerImageAssetProps Maven / Gradle / Ivy
package software.amazon.awscdk.assets.docker;
/**
* EXPERIMENTAL
*/
@javax.annotation.Generated(value = "jsii-pacmak/0.11.2 (build 27d16c2)", date = "2019-06-10T11:10:22.639Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public interface DockerImageAssetProps extends software.amazon.jsii.JsiiSerializable, software.amazon.awscdk.assets.CopyOptions {
/**
* The directory where the Dockerfile is stored.
*
* EXPERIMENTAL
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
java.lang.String getDirectory();
/**
* Build args to pass to the `docker build` command.
*
* Default: no build args are passed
*
* EXPERIMENTAL
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
java.util.Map getBuildArgs();
/**
* ECR repository name.
*
* Specify this property if you need to statically address the image, e.g.
* from a Kubernetes Pod. Note, this is only the repository name, without the
* registry and the tag parts.
*
* Default: automatically derived from the asset's ID.
*
* EXPERIMENTAL
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
java.lang.String getRepositoryName();
/**
* @return a {@link Builder} of {@link DockerImageAssetProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link DockerImageAssetProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
final class Builder {
private java.lang.String _directory;
@javax.annotation.Nullable
private java.util.Map _buildArgs;
@javax.annotation.Nullable
private java.lang.String _repositoryName;
@javax.annotation.Nullable
private java.util.List _exclude;
@javax.annotation.Nullable
private software.amazon.awscdk.assets.FollowMode _follow;
/**
* Sets the value of Directory
* @param value The directory where the Dockerfile is stored.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder withDirectory(final java.lang.String value) {
this._directory = java.util.Objects.requireNonNull(value, "directory is required");
return this;
}
/**
* Sets the value of BuildArgs
* @param value Build args to pass to the `docker build` command.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder withBuildArgs(@javax.annotation.Nullable final java.util.Map value) {
this._buildArgs = value;
return this;
}
/**
* Sets the value of RepositoryName
* @param value ECR repository name.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder withRepositoryName(@javax.annotation.Nullable final java.lang.String value) {
this._repositoryName = value;
return this;
}
/**
* Sets the value of Exclude
* @param value Glob patterns to exclude from the copy.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder withExclude(@javax.annotation.Nullable final java.util.List value) {
this._exclude = value;
return this;
}
/**
* Sets the value of Follow
* @param value A strategy for how to handle symlinks.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder withFollow(@javax.annotation.Nullable final software.amazon.awscdk.assets.FollowMode value) {
this._follow = value;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link DockerImageAssetProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public DockerImageAssetProps build() {
return new DockerImageAssetProps() {
private final java.lang.String $directory = java.util.Objects.requireNonNull(_directory, "directory is required");
@javax.annotation.Nullable
private final java.util.Map $buildArgs = _buildArgs;
@javax.annotation.Nullable
private final java.lang.String $repositoryName = _repositoryName;
@javax.annotation.Nullable
private final java.util.List $exclude = _exclude;
@javax.annotation.Nullable
private final software.amazon.awscdk.assets.FollowMode $follow = _follow;
@Override
public java.lang.String getDirectory() {
return this.$directory;
}
@Override
public java.util.Map getBuildArgs() {
return this.$buildArgs;
}
@Override
public java.lang.String getRepositoryName() {
return this.$repositoryName;
}
@Override
public java.util.List getExclude() {
return this.$exclude;
}
@Override
public software.amazon.awscdk.assets.FollowMode getFollow() {
return this.$follow;
}
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("directory", om.valueToTree(this.getDirectory()));
if (this.getBuildArgs() != null) {
obj.set("buildArgs", om.valueToTree(this.getBuildArgs()));
}
if (this.getRepositoryName() != null) {
obj.set("repositoryName", om.valueToTree(this.getRepositoryName()));
}
if (this.getExclude() != null) {
obj.set("exclude", om.valueToTree(this.getExclude()));
}
if (this.getFollow() != null) {
obj.set("follow", om.valueToTree(this.getFollow()));
}
return obj;
}
};
}
}
/**
* A proxy class which represents a concrete javascript instance of this type.
*/
final static class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements software.amazon.awscdk.assets.docker.DockerImageAssetProps {
protected Jsii$Proxy(final software.amazon.jsii.JsiiObject.InitializationMode mode) {
super(mode);
}
/**
* The directory where the Dockerfile is stored.
*
* EXPERIMENTAL
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public java.lang.String getDirectory() {
return this.jsiiGet("directory", java.lang.String.class);
}
/**
* Build args to pass to the `docker build` command.
*
* Default: no build args are passed
*
* EXPERIMENTAL
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@javax.annotation.Nullable
public java.util.Map getBuildArgs() {
return this.jsiiGet("buildArgs", java.util.Map.class);
}
/**
* ECR repository name.
*
* Specify this property if you need to statically address the image, e.g.
* from a Kubernetes Pod. Note, this is only the repository name, without the
* registry and the tag parts.
*
* Default: automatically derived from the asset's ID.
*
* EXPERIMENTAL
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@javax.annotation.Nullable
public java.lang.String getRepositoryName() {
return this.jsiiGet("repositoryName", java.lang.String.class);
}
/**
* Glob patterns to exclude from the copy.
*
* Default: nothing is excluded
*
* EXPERIMENTAL
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@javax.annotation.Nullable
public java.util.List getExclude() {
return this.jsiiGet("exclude", java.util.List.class);
}
/**
* A strategy for how to handle symlinks.
*
* Default: Never
*
* EXPERIMENTAL
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@javax.annotation.Nullable
public software.amazon.awscdk.assets.FollowMode getFollow() {
return this.jsiiGet("follow", software.amazon.awscdk.assets.FollowMode.class);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy