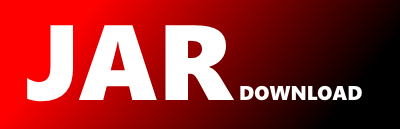
software.amazon.awscdk.ArnComponents Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cdk Show documentation
Show all versions of cdk Show documentation
AWS Cloud Development Kit Core Library
package software.amazon.awscdk;
@javax.annotation.Generated(value = "jsii-pacmak/0.10.5 (build 46bc9b0)", date = "2019-05-06T20:49:40.932Z")
public interface ArnComponents extends software.amazon.jsii.JsiiSerializable {
/**
* Resource type (e.g. "table", "autoScalingGroup", "certificate"). For some resource types, e.g. S3 buckets, this field defines the bucket name.
*/
java.lang.String getResource();
/**
* The service namespace that identifies the AWS product (for example, 's3', 'iam', 'codepipline').
*/
java.lang.String getService();
/**
* The ID of the AWS account that owns the resource, without the hyphens. For example, 123456789012. Note that the ARNs for some resources don't require an account number, so this component might be omitted.
*
* Default: The account the stack is deployed to.
*/
java.lang.String getAccount();
/**
* The partition that the resource is in.
*
* For standard AWS regions, the
* partition is aws. If you have resources in other partitions, the
* partition is aws-partitionname. For example, the partition for resources
* in the China (Beijing) region is aws-cn.
*
* Default: The AWS partition the stack is deployed to.
*/
java.lang.String getPartition();
/**
* The region the resource resides in.
*
* Note that the ARNs for some resources
* do not require a region, so this component might be omitted.
*
* Default: The region the stack is deployed to.
*/
java.lang.String getRegion();
/**
* Resource name or path within the resource (i.e. S3 bucket object key) or a wildcard such as ``"*"``. This is service-dependent.
*/
java.lang.String getResourceName();
/**
* Separator between resource type and the resource.
*
* Can be either '/', ':' or an empty string. Will only be used if resourceName is defined.
*
* Default: '/'
*/
java.lang.String getSep();
/**
* @return a {@link Builder} of {@link ArnComponents}
*/
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link ArnComponents}
*/
final class Builder {
private java.lang.String _resource;
private java.lang.String _service;
@javax.annotation.Nullable
private java.lang.String _account;
@javax.annotation.Nullable
private java.lang.String _partition;
@javax.annotation.Nullable
private java.lang.String _region;
@javax.annotation.Nullable
private java.lang.String _resourceName;
@javax.annotation.Nullable
private java.lang.String _sep;
/**
* Sets the value of Resource
* @param value Resource type (e.g. "table", "autoScalingGroup", "certificate"). For some resource types, e.g. S3 buckets, this field defines the bucket name.
* @return {@code this}
*/
public Builder withResource(final java.lang.String value) {
this._resource = java.util.Objects.requireNonNull(value, "resource is required");
return this;
}
/**
* Sets the value of Service
* @param value The service namespace that identifies the AWS product (for example, 's3', 'iam', 'codepipline').
* @return {@code this}
*/
public Builder withService(final java.lang.String value) {
this._service = java.util.Objects.requireNonNull(value, "service is required");
return this;
}
/**
* Sets the value of Account
* @param value The ID of the AWS account that owns the resource, without the hyphens. For example, 123456789012. Note that the ARNs for some resources don't require an account number, so this component might be omitted.
* @return {@code this}
*/
public Builder withAccount(@javax.annotation.Nullable final java.lang.String value) {
this._account = value;
return this;
}
/**
* Sets the value of Partition
* @param value The partition that the resource is in.
* @return {@code this}
*/
public Builder withPartition(@javax.annotation.Nullable final java.lang.String value) {
this._partition = value;
return this;
}
/**
* Sets the value of Region
* @param value The region the resource resides in.
* @return {@code this}
*/
public Builder withRegion(@javax.annotation.Nullable final java.lang.String value) {
this._region = value;
return this;
}
/**
* Sets the value of ResourceName
* @param value Resource name or path within the resource (i.e. S3 bucket object key) or a wildcard such as ``"*"``. This is service-dependent.
* @return {@code this}
*/
public Builder withResourceName(@javax.annotation.Nullable final java.lang.String value) {
this._resourceName = value;
return this;
}
/**
* Sets the value of Sep
* @param value Separator between resource type and the resource.
* @return {@code this}
*/
public Builder withSep(@javax.annotation.Nullable final java.lang.String value) {
this._sep = value;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link ArnComponents}
* @throws NullPointerException if any required attribute was not provided
*/
public ArnComponents build() {
return new ArnComponents() {
private final java.lang.String $resource = java.util.Objects.requireNonNull(_resource, "resource is required");
private final java.lang.String $service = java.util.Objects.requireNonNull(_service, "service is required");
@javax.annotation.Nullable
private final java.lang.String $account = _account;
@javax.annotation.Nullable
private final java.lang.String $partition = _partition;
@javax.annotation.Nullable
private final java.lang.String $region = _region;
@javax.annotation.Nullable
private final java.lang.String $resourceName = _resourceName;
@javax.annotation.Nullable
private final java.lang.String $sep = _sep;
@Override
public java.lang.String getResource() {
return this.$resource;
}
@Override
public java.lang.String getService() {
return this.$service;
}
@Override
public java.lang.String getAccount() {
return this.$account;
}
@Override
public java.lang.String getPartition() {
return this.$partition;
}
@Override
public java.lang.String getRegion() {
return this.$region;
}
@Override
public java.lang.String getResourceName() {
return this.$resourceName;
}
@Override
public java.lang.String getSep() {
return this.$sep;
}
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("resource", om.valueToTree(this.getResource()));
obj.set("service", om.valueToTree(this.getService()));
obj.set("account", om.valueToTree(this.getAccount()));
obj.set("partition", om.valueToTree(this.getPartition()));
obj.set("region", om.valueToTree(this.getRegion()));
obj.set("resourceName", om.valueToTree(this.getResourceName()));
obj.set("sep", om.valueToTree(this.getSep()));
return obj;
}
};
}
}
/**
* A proxy class which represents a concrete javascript instance of this type.
*/
final static class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements software.amazon.awscdk.ArnComponents {
protected Jsii$Proxy(final software.amazon.jsii.JsiiObject.InitializationMode mode) {
super(mode);
}
/**
* Resource type (e.g. "table", "autoScalingGroup", "certificate"). For some resource types, e.g. S3 buckets, this field defines the bucket name.
*/
@Override
public java.lang.String getResource() {
return this.jsiiGet("resource", java.lang.String.class);
}
/**
* The service namespace that identifies the AWS product (for example, 's3', 'iam', 'codepipline').
*/
@Override
public java.lang.String getService() {
return this.jsiiGet("service", java.lang.String.class);
}
/**
* The ID of the AWS account that owns the resource, without the hyphens. For example, 123456789012. Note that the ARNs for some resources don't require an account number, so this component might be omitted.
*
* Default: The account the stack is deployed to.
*/
@Override
@javax.annotation.Nullable
public java.lang.String getAccount() {
return this.jsiiGet("account", java.lang.String.class);
}
/**
* The partition that the resource is in.
*
* For standard AWS regions, the
* partition is aws. If you have resources in other partitions, the
* partition is aws-partitionname. For example, the partition for resources
* in the China (Beijing) region is aws-cn.
*
* Default: The AWS partition the stack is deployed to.
*/
@Override
@javax.annotation.Nullable
public java.lang.String getPartition() {
return this.jsiiGet("partition", java.lang.String.class);
}
/**
* The region the resource resides in.
*
* Note that the ARNs for some resources
* do not require a region, so this component might be omitted.
*
* Default: The region the stack is deployed to.
*/
@Override
@javax.annotation.Nullable
public java.lang.String getRegion() {
return this.jsiiGet("region", java.lang.String.class);
}
/**
* Resource name or path within the resource (i.e. S3 bucket object key) or a wildcard such as ``"*"``. This is service-dependent.
*/
@Override
@javax.annotation.Nullable
public java.lang.String getResourceName() {
return this.jsiiGet("resourceName", java.lang.String.class);
}
/**
* Separator between resource type and the resource.
*
* Can be either '/', ':' or an empty string. Will only be used if resourceName is defined.
*
* Default: '/'
*/
@Override
@javax.annotation.Nullable
public java.lang.String getSep() {
return this.jsiiGet("sep", java.lang.String.class);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy