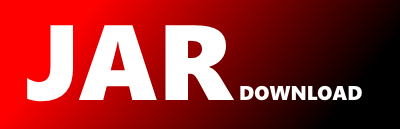
software.amazon.awscdk.AutoScalingRollingUpdate Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cdk Show documentation
Show all versions of cdk Show documentation
AWS Cloud Development Kit Core Library
package software.amazon.awscdk;
/**
* To specify how AWS CloudFormation handles rolling updates for an Auto Scaling group, use the AutoScalingRollingUpdate policy.
*
* Rolling updates enable you to specify whether AWS CloudFormation updates instances that are in an Auto Scaling
* group in batches or all at once.
*/
@javax.annotation.Generated(value = "jsii-pacmak/0.10.5 (build 46bc9b0)", date = "2019-05-06T20:49:40.933Z")
public interface AutoScalingRollingUpdate extends software.amazon.jsii.JsiiSerializable {
/**
* Specifies the maximum number of instances that AWS CloudFormation updates.
*/
java.lang.Number getMaxBatchSize();
/**
* Specifies the minimum number of instances that must be in service within the Auto Scaling group while AWS CloudFormation updates old instances.
*/
java.lang.Number getMinInstancesInService();
/**
* Specifies the percentage of instances in an Auto Scaling rolling update that must signal success for an update to succeed. You can specify a value from 0 to 100. AWS CloudFormation rounds to the nearest tenth of a percent. For example, if you update five instances with a minimum successful percentage of 50, three instances must signal success.
*
* If an instance doesn't send a signal within the time specified in the PauseTime property, AWS CloudFormation assumes
* that the instance wasn't updated.
*
* If you specify this property, you must also enable the WaitOnResourceSignals and PauseTime properties.
*/
java.lang.Number getMinSuccessfulInstancesPercent();
/**
* The amount of time that AWS CloudFormation pauses after making a change to a batch of instances to give those instances time to start software applications.
*
* For example, you might need to specify PauseTime when scaling up the number of
* instances in an Auto Scaling group.
*
* If you enable the WaitOnResourceSignals property, PauseTime is the amount of time that AWS CloudFormation should wait
* for the Auto Scaling group to receive the required number of valid signals from added or replaced instances. If the
* PauseTime is exceeded before the Auto Scaling group receives the required number of signals, the update fails. For best
* results, specify a time period that gives your applications sufficient time to get started. If the update needs to be
* rolled back, a short PauseTime can cause the rollback to fail.
*
* Specify PauseTime in the ISO8601 duration format (in the format PT#H#M#S, where each # is the number of hours, minutes,
* and seconds, respectively). The maximum PauseTime is one hour (PT1H).
*/
java.lang.String getPauseTime();
/**
* Specifies the Auto Scaling processes to suspend during a stack update.
*
* Suspending processes prevents Auto Scaling from
* interfering with a stack update. For example, you can suspend alarming so that Auto Scaling doesn't execute scaling
* policies associated with an alarm. For valid values, see the ScalingProcesses.member.N parameter for the SuspendProcesses
* action in the Auto Scaling API Reference.
*/
java.util.List getSuspendProcesses();
/**
* Specifies whether the Auto Scaling group waits on signals from new instances during an update.
*
* Use this property to
* ensure that instances have completed installing and configuring applications before the Auto Scaling group update proceeds.
* AWS CloudFormation suspends the update of an Auto Scaling group after new EC2 instances are launched into the group.
* AWS CloudFormation must receive a signal from each new instance within the specified PauseTime before continuing the update.
* To signal the Auto Scaling group, use the cfn-signal helper script or SignalResource API.
*
* To have instances wait for an Elastic Load Balancing health check before they signal success, add a health-check
* verification by using the cfn-init helper script. For an example, see the verify_instance_health command in the Auto Scaling
* rolling updates sample template.
*/
java.lang.Boolean getWaitOnResourceSignals();
/**
* @return a {@link Builder} of {@link AutoScalingRollingUpdate}
*/
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link AutoScalingRollingUpdate}
*/
final class Builder {
@javax.annotation.Nullable
private java.lang.Number _maxBatchSize;
@javax.annotation.Nullable
private java.lang.Number _minInstancesInService;
@javax.annotation.Nullable
private java.lang.Number _minSuccessfulInstancesPercent;
@javax.annotation.Nullable
private java.lang.String _pauseTime;
@javax.annotation.Nullable
private java.util.List _suspendProcesses;
@javax.annotation.Nullable
private java.lang.Boolean _waitOnResourceSignals;
/**
* Sets the value of MaxBatchSize
* @param value Specifies the maximum number of instances that AWS CloudFormation updates.
* @return {@code this}
*/
public Builder withMaxBatchSize(@javax.annotation.Nullable final java.lang.Number value) {
this._maxBatchSize = value;
return this;
}
/**
* Sets the value of MinInstancesInService
* @param value Specifies the minimum number of instances that must be in service within the Auto Scaling group while AWS CloudFormation updates old instances.
* @return {@code this}
*/
public Builder withMinInstancesInService(@javax.annotation.Nullable final java.lang.Number value) {
this._minInstancesInService = value;
return this;
}
/**
* Sets the value of MinSuccessfulInstancesPercent
* @param value Specifies the percentage of instances in an Auto Scaling rolling update that must signal success for an update to succeed. You can specify a value from 0 to 100. AWS CloudFormation rounds to the nearest tenth of a percent. For example, if you update five instances with a minimum successful percentage of 50, three instances must signal success.
* @return {@code this}
*/
public Builder withMinSuccessfulInstancesPercent(@javax.annotation.Nullable final java.lang.Number value) {
this._minSuccessfulInstancesPercent = value;
return this;
}
/**
* Sets the value of PauseTime
* @param value The amount of time that AWS CloudFormation pauses after making a change to a batch of instances to give those instances time to start software applications.
* @return {@code this}
*/
public Builder withPauseTime(@javax.annotation.Nullable final java.lang.String value) {
this._pauseTime = value;
return this;
}
/**
* Sets the value of SuspendProcesses
* @param value Specifies the Auto Scaling processes to suspend during a stack update.
* @return {@code this}
*/
public Builder withSuspendProcesses(@javax.annotation.Nullable final java.util.List value) {
this._suspendProcesses = value;
return this;
}
/**
* Sets the value of WaitOnResourceSignals
* @param value Specifies whether the Auto Scaling group waits on signals from new instances during an update.
* @return {@code this}
*/
public Builder withWaitOnResourceSignals(@javax.annotation.Nullable final java.lang.Boolean value) {
this._waitOnResourceSignals = value;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link AutoScalingRollingUpdate}
* @throws NullPointerException if any required attribute was not provided
*/
public AutoScalingRollingUpdate build() {
return new AutoScalingRollingUpdate() {
@javax.annotation.Nullable
private final java.lang.Number $maxBatchSize = _maxBatchSize;
@javax.annotation.Nullable
private final java.lang.Number $minInstancesInService = _minInstancesInService;
@javax.annotation.Nullable
private final java.lang.Number $minSuccessfulInstancesPercent = _minSuccessfulInstancesPercent;
@javax.annotation.Nullable
private final java.lang.String $pauseTime = _pauseTime;
@javax.annotation.Nullable
private final java.util.List $suspendProcesses = _suspendProcesses;
@javax.annotation.Nullable
private final java.lang.Boolean $waitOnResourceSignals = _waitOnResourceSignals;
@Override
public java.lang.Number getMaxBatchSize() {
return this.$maxBatchSize;
}
@Override
public java.lang.Number getMinInstancesInService() {
return this.$minInstancesInService;
}
@Override
public java.lang.Number getMinSuccessfulInstancesPercent() {
return this.$minSuccessfulInstancesPercent;
}
@Override
public java.lang.String getPauseTime() {
return this.$pauseTime;
}
@Override
public java.util.List getSuspendProcesses() {
return this.$suspendProcesses;
}
@Override
public java.lang.Boolean getWaitOnResourceSignals() {
return this.$waitOnResourceSignals;
}
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("maxBatchSize", om.valueToTree(this.getMaxBatchSize()));
obj.set("minInstancesInService", om.valueToTree(this.getMinInstancesInService()));
obj.set("minSuccessfulInstancesPercent", om.valueToTree(this.getMinSuccessfulInstancesPercent()));
obj.set("pauseTime", om.valueToTree(this.getPauseTime()));
obj.set("suspendProcesses", om.valueToTree(this.getSuspendProcesses()));
obj.set("waitOnResourceSignals", om.valueToTree(this.getWaitOnResourceSignals()));
return obj;
}
};
}
}
/**
* A proxy class which represents a concrete javascript instance of this type.
*/
final static class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements software.amazon.awscdk.AutoScalingRollingUpdate {
protected Jsii$Proxy(final software.amazon.jsii.JsiiObject.InitializationMode mode) {
super(mode);
}
/**
* Specifies the maximum number of instances that AWS CloudFormation updates.
*/
@Override
@javax.annotation.Nullable
public java.lang.Number getMaxBatchSize() {
return this.jsiiGet("maxBatchSize", java.lang.Number.class);
}
/**
* Specifies the minimum number of instances that must be in service within the Auto Scaling group while AWS CloudFormation updates old instances.
*/
@Override
@javax.annotation.Nullable
public java.lang.Number getMinInstancesInService() {
return this.jsiiGet("minInstancesInService", java.lang.Number.class);
}
/**
* Specifies the percentage of instances in an Auto Scaling rolling update that must signal success for an update to succeed. You can specify a value from 0 to 100. AWS CloudFormation rounds to the nearest tenth of a percent. For example, if you update five instances with a minimum successful percentage of 50, three instances must signal success.
*
* If an instance doesn't send a signal within the time specified in the PauseTime property, AWS CloudFormation assumes
* that the instance wasn't updated.
*
* If you specify this property, you must also enable the WaitOnResourceSignals and PauseTime properties.
*/
@Override
@javax.annotation.Nullable
public java.lang.Number getMinSuccessfulInstancesPercent() {
return this.jsiiGet("minSuccessfulInstancesPercent", java.lang.Number.class);
}
/**
* The amount of time that AWS CloudFormation pauses after making a change to a batch of instances to give those instances time to start software applications.
*
* For example, you might need to specify PauseTime when scaling up the number of
* instances in an Auto Scaling group.
*
* If you enable the WaitOnResourceSignals property, PauseTime is the amount of time that AWS CloudFormation should wait
* for the Auto Scaling group to receive the required number of valid signals from added or replaced instances. If the
* PauseTime is exceeded before the Auto Scaling group receives the required number of signals, the update fails. For best
* results, specify a time period that gives your applications sufficient time to get started. If the update needs to be
* rolled back, a short PauseTime can cause the rollback to fail.
*
* Specify PauseTime in the ISO8601 duration format (in the format PT#H#M#S, where each # is the number of hours, minutes,
* and seconds, respectively). The maximum PauseTime is one hour (PT1H).
*/
@Override
@javax.annotation.Nullable
public java.lang.String getPauseTime() {
return this.jsiiGet("pauseTime", java.lang.String.class);
}
/**
* Specifies the Auto Scaling processes to suspend during a stack update.
*
* Suspending processes prevents Auto Scaling from
* interfering with a stack update. For example, you can suspend alarming so that Auto Scaling doesn't execute scaling
* policies associated with an alarm. For valid values, see the ScalingProcesses.member.N parameter for the SuspendProcesses
* action in the Auto Scaling API Reference.
*/
@Override
@javax.annotation.Nullable
public java.util.List getSuspendProcesses() {
return this.jsiiGet("suspendProcesses", java.util.List.class);
}
/**
* Specifies whether the Auto Scaling group waits on signals from new instances during an update.
*
* Use this property to
* ensure that instances have completed installing and configuring applications before the Auto Scaling group update proceeds.
* AWS CloudFormation suspends the update of an Auto Scaling group after new EC2 instances are launched into the group.
* AWS CloudFormation must receive a signal from each new instance within the specified PauseTime before continuing the update.
* To signal the Auto Scaling group, use the cfn-signal helper script or SignalResource API.
*
* To have instances wait for an Elastic Load Balancing health check before they signal success, add a health-check
* verification by using the cfn-init helper script. For an example, see the verify_instance_health command in the Auto Scaling
* rolling updates sample template.
*/
@Override
@javax.annotation.Nullable
public java.lang.Boolean getWaitOnResourceSignals() {
return this.jsiiGet("waitOnResourceSignals", java.lang.Boolean.class);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy