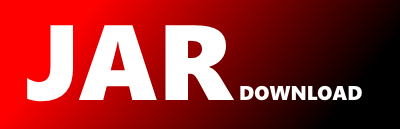
software.amazon.awscdk.CfnElement Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cdk Show documentation
Show all versions of cdk Show documentation
AWS Cloud Development Kit Core Library
package software.amazon.awscdk;
/**
* An element of a CloudFormation stack.
*/
@javax.annotation.Generated(value = "jsii-pacmak/0.10.5 (build 46bc9b0)", date = "2019-05-06T20:49:40.936Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.$Module.class, fqn = "@aws-cdk/cdk.CfnElement")
public abstract class CfnElement extends software.amazon.awscdk.Construct {
protected CfnElement(final software.amazon.jsii.JsiiObject.InitializationMode mode) {
super(mode);
}
/**
* Creates an entity and binds it to a tree. Note that the root of the tree must be a Stack object (not just any Root).
*
* @param scope The parent construct.
*/
public CfnElement(final software.amazon.awscdk.Construct scope, final java.lang.String id) {
super(software.amazon.jsii.JsiiObject.InitializationMode.Jsii);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required") });
}
/**
* Returns `true` if a construct is a stack element (i.e. part of the synthesized cloudformation template).
*
* Uses duck-typing instead of `instanceof` to allow stack elements from different
* versions of this library to be included in the same stack.
*
* @return The construct as a stack element or undefined if it is not a stack element.
*/
public static java.lang.Boolean isCfnElement(final software.amazon.awscdk.IConstruct construct) {
return software.amazon.jsii.JsiiObject.jsiiStaticCall(software.amazon.awscdk.CfnElement.class, "isCfnElement", java.lang.Boolean.class, new Object[] { java.util.Objects.requireNonNull(construct, "construct is required") });
}
/**
* Overrides the auto-generated logical ID with a specific ID.
*
* @param newLogicalId The new logical ID to use for this stack element.
*/
public void overrideLogicalId(final java.lang.String newLogicalId) {
this.jsiiCall("overrideLogicalId", Void.class, new Object[] { java.util.Objects.requireNonNull(newLogicalId, "newLogicalId is required") });
}
/**
* Automatically detect references in this CfnElement.
*/
@Override
protected void prepare() {
this.jsiiCall("prepare", Void.class);
}
/**
* @return the stack trace of the point where this Resource was created from, sourced
* from the +metadata+ entry typed +aws:cdk:logicalId+, and with the bottom-most
* node +internal+ entries filtered.
*/
public java.util.List getCreationStackTrace() {
return this.jsiiGet("creationStackTrace", java.util.List.class);
}
/**
* The logical ID for this CloudFormation stack element.
*
* The logical ID of the element
* is calculated from the path of the resource node in the construct tree.
*
* To override this value, use `overrideLogicalId(newLogicalId)`.
*
* @return the logical ID as a stringified token. This value will only get
* resolved during synthesis.
*/
public java.lang.String getLogicalId() {
return this.jsiiGet("logicalId", java.lang.String.class);
}
/**
* Return the path with respect to the stack.
*/
public java.lang.String getStackPath() {
return this.jsiiGet("stackPath", java.lang.String.class);
}
/**
* A proxy class which represents a concrete javascript instance of this type.
*/
final static class Jsii$Proxy extends software.amazon.awscdk.CfnElement {
protected Jsii$Proxy(final software.amazon.jsii.JsiiObject.InitializationMode mode) {
super(mode);
}
/**
* The construct node in the scope tree.
*/
@Override
public software.amazon.awscdk.ConstructNode getNode() {
return this.jsiiGet("node", software.amazon.awscdk.ConstructNode.class);
}
/**
* The set of constructs that form the root of this dependable.
*
* All resources under all returned constructs are included in the ordering
* dependency.
*/
@Override
public java.util.List getDependencyRoots() {
return this.jsiiGet("dependencyRoots", java.util.List.class);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy