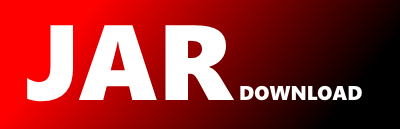
software.amazon.awscdk.CfnOutputProps Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cdk Show documentation
Show all versions of cdk Show documentation
AWS Cloud Development Kit Core Library
package software.amazon.awscdk;
@javax.annotation.Generated(value = "jsii-pacmak/0.10.5 (build 46bc9b0)", date = "2019-05-06T20:49:40.938Z")
public interface CfnOutputProps extends software.amazon.jsii.JsiiSerializable {
/**
* The value of the property returned by the aws cloudformation describe-stacks command. The value of an output can include literals, parameter references, pseudo-parameters, a mapping value, or intrinsic functions.
*/
java.lang.Object getValue();
/**
* A condition from the "Conditions" section to associate with this output value.
*
* If the condition evaluates to `false`, this output value will not
* be included in the stack.
*/
software.amazon.awscdk.CfnCondition getCondition();
/**
* A String type that describes the output value. The description can be a maximum of 4 K in length.
*/
java.lang.String getDescription();
/**
* Disables the automatic allocation of an export name for this output.
*
* This prohibits exporting this value, either by specifying `export` or
* by calling `makeImportValue()`.
*
* Default: false
*/
java.lang.Boolean getDisableExport();
/**
* The name used to export the value of this output across stacks.
*
* To import the value from another stack, use `FnImportValue(export)`. You
* can create an import value token by calling `output.makeImportValue()`.
*
* Default: Automatically allocate a name when `makeImportValue()` is
* called.
*/
java.lang.String getExport();
/**
* @return a {@link Builder} of {@link CfnOutputProps}
*/
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link CfnOutputProps}
*/
final class Builder {
private java.lang.Object _value;
@javax.annotation.Nullable
private software.amazon.awscdk.CfnCondition _condition;
@javax.annotation.Nullable
private java.lang.String _description;
@javax.annotation.Nullable
private java.lang.Boolean _disableExport;
@javax.annotation.Nullable
private java.lang.String _export;
/**
* Sets the value of Value
* @param value The value of the property returned by the aws cloudformation describe-stacks command. The value of an output can include literals, parameter references, pseudo-parameters, a mapping value, or intrinsic functions.
* @return {@code this}
*/
public Builder withValue(final java.lang.Object value) {
this._value = java.util.Objects.requireNonNull(value, "value is required");
return this;
}
/**
* Sets the value of Condition
* @param value A condition from the "Conditions" section to associate with this output value.
* @return {@code this}
*/
public Builder withCondition(@javax.annotation.Nullable final software.amazon.awscdk.CfnCondition value) {
this._condition = value;
return this;
}
/**
* Sets the value of Description
* @param value A String type that describes the output value. The description can be a maximum of 4 K in length.
* @return {@code this}
*/
public Builder withDescription(@javax.annotation.Nullable final java.lang.String value) {
this._description = value;
return this;
}
/**
* Sets the value of DisableExport
* @param value Disables the automatic allocation of an export name for this output.
* @return {@code this}
*/
public Builder withDisableExport(@javax.annotation.Nullable final java.lang.Boolean value) {
this._disableExport = value;
return this;
}
/**
* Sets the value of Export
* @param value The name used to export the value of this output across stacks.
* @return {@code this}
*/
public Builder withExport(@javax.annotation.Nullable final java.lang.String value) {
this._export = value;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link CfnOutputProps}
* @throws NullPointerException if any required attribute was not provided
*/
public CfnOutputProps build() {
return new CfnOutputProps() {
private final java.lang.Object $value = java.util.Objects.requireNonNull(_value, "value is required");
@javax.annotation.Nullable
private final software.amazon.awscdk.CfnCondition $condition = _condition;
@javax.annotation.Nullable
private final java.lang.String $description = _description;
@javax.annotation.Nullable
private final java.lang.Boolean $disableExport = _disableExport;
@javax.annotation.Nullable
private final java.lang.String $export = _export;
@Override
public java.lang.Object getValue() {
return this.$value;
}
@Override
public software.amazon.awscdk.CfnCondition getCondition() {
return this.$condition;
}
@Override
public java.lang.String getDescription() {
return this.$description;
}
@Override
public java.lang.Boolean getDisableExport() {
return this.$disableExport;
}
@Override
public java.lang.String getExport() {
return this.$export;
}
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("value", om.valueToTree(this.getValue()));
obj.set("condition", om.valueToTree(this.getCondition()));
obj.set("description", om.valueToTree(this.getDescription()));
obj.set("disableExport", om.valueToTree(this.getDisableExport()));
obj.set("export", om.valueToTree(this.getExport()));
return obj;
}
};
}
}
/**
* A proxy class which represents a concrete javascript instance of this type.
*/
final static class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements software.amazon.awscdk.CfnOutputProps {
protected Jsii$Proxy(final software.amazon.jsii.JsiiObject.InitializationMode mode) {
super(mode);
}
/**
* The value of the property returned by the aws cloudformation describe-stacks command. The value of an output can include literals, parameter references, pseudo-parameters, a mapping value, or intrinsic functions.
*/
@Override
@javax.annotation.Nullable
public java.lang.Object getValue() {
return this.jsiiGet("value", java.lang.Object.class);
}
/**
* A condition from the "Conditions" section to associate with this output value.
*
* If the condition evaluates to `false`, this output value will not
* be included in the stack.
*/
@Override
@javax.annotation.Nullable
public software.amazon.awscdk.CfnCondition getCondition() {
return this.jsiiGet("condition", software.amazon.awscdk.CfnCondition.class);
}
/**
* A String type that describes the output value. The description can be a maximum of 4 K in length.
*/
@Override
@javax.annotation.Nullable
public java.lang.String getDescription() {
return this.jsiiGet("description", java.lang.String.class);
}
/**
* Disables the automatic allocation of an export name for this output.
*
* This prohibits exporting this value, either by specifying `export` or
* by calling `makeImportValue()`.
*
* Default: false
*/
@Override
@javax.annotation.Nullable
public java.lang.Boolean getDisableExport() {
return this.jsiiGet("disableExport", java.lang.Boolean.class);
}
/**
* The name used to export the value of this output across stacks.
*
* To import the value from another stack, use `FnImportValue(export)`. You
* can create an import value token by calling `output.makeImportValue()`.
*
* Default: Automatically allocate a name when `makeImportValue()` is
* called.
*/
@Override
@javax.annotation.Nullable
public java.lang.String getExport() {
return this.jsiiGet("export", java.lang.String.class);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy