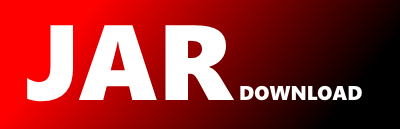
software.amazon.awscdk.CfnParameterProps Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cdk Show documentation
Show all versions of cdk Show documentation
AWS Cloud Development Kit Core Library
package software.amazon.awscdk;
@javax.annotation.Generated(value = "jsii-pacmak/0.10.5 (build 46bc9b0)", date = "2019-05-06T20:49:40.939Z")
public interface CfnParameterProps extends software.amazon.jsii.JsiiSerializable {
/**
* The data type for the parameter (DataType).
*/
java.lang.String getType();
/**
* A regular expression that represents the patterns to allow for String types.
*/
java.lang.String getAllowedPattern();
/**
* An array containing the list of values allowed for the parameter.
*/
java.util.List getAllowedValues();
/**
* A string that explains a constraint when the constraint is violated. For example, without a constraint description, a parameter that has an allowed pattern of [A-Za-z0-9]+ displays the following error message when the user specifies an invalid value:.
*/
java.lang.String getConstraintDescription();
/**
* A value of the appropriate type for the template to use if no value is specified when a stack is created.
*
* If you define constraints for the parameter, you must specify
* a value that adheres to those constraints.
*/
java.lang.Object getDefault();
/**
* A string of up to 4000 characters that describes the parameter.
*/
java.lang.String getDescription();
/**
* An integer value that determines the largest number of characters you want to allow for String types.
*/
java.lang.Number getMaxLength();
/**
* A numeric value that determines the largest numeric value you want to allow for Number types.
*/
java.lang.Number getMaxValue();
/**
* An integer value that determines the smallest number of characters you want to allow for String types.
*/
java.lang.Number getMinLength();
/**
* A numeric value that determines the smallest numeric value you want to allow for Number types.
*/
java.lang.Number getMinValue();
/**
* Whether to mask the parameter value when anyone makes a call that describes the stack. If you set the value to ``true``, the parameter value is masked with asterisks (``*****``).
*/
java.lang.Boolean getNoEcho();
/**
* @return a {@link Builder} of {@link CfnParameterProps}
*/
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link CfnParameterProps}
*/
final class Builder {
private java.lang.String _type;
@javax.annotation.Nullable
private java.lang.String _allowedPattern;
@javax.annotation.Nullable
private java.util.List _allowedValues;
@javax.annotation.Nullable
private java.lang.String _constraintDescription;
@javax.annotation.Nullable
private java.lang.Object _default;
@javax.annotation.Nullable
private java.lang.String _description;
@javax.annotation.Nullable
private java.lang.Number _maxLength;
@javax.annotation.Nullable
private java.lang.Number _maxValue;
@javax.annotation.Nullable
private java.lang.Number _minLength;
@javax.annotation.Nullable
private java.lang.Number _minValue;
@javax.annotation.Nullable
private java.lang.Boolean _noEcho;
/**
* Sets the value of Type
* @param value The data type for the parameter (DataType).
* @return {@code this}
*/
public Builder withType(final java.lang.String value) {
this._type = java.util.Objects.requireNonNull(value, "type is required");
return this;
}
/**
* Sets the value of AllowedPattern
* @param value A regular expression that represents the patterns to allow for String types.
* @return {@code this}
*/
public Builder withAllowedPattern(@javax.annotation.Nullable final java.lang.String value) {
this._allowedPattern = value;
return this;
}
/**
* Sets the value of AllowedValues
* @param value An array containing the list of values allowed for the parameter.
* @return {@code this}
*/
public Builder withAllowedValues(@javax.annotation.Nullable final java.util.List value) {
this._allowedValues = value;
return this;
}
/**
* Sets the value of ConstraintDescription
* @param value A string that explains a constraint when the constraint is violated. For example, without a constraint description, a parameter that has an allowed pattern of [A-Za-z0-9]+ displays the following error message when the user specifies an invalid value:.
* @return {@code this}
*/
public Builder withConstraintDescription(@javax.annotation.Nullable final java.lang.String value) {
this._constraintDescription = value;
return this;
}
/**
* Sets the value of Default
* @param value A value of the appropriate type for the template to use if no value is specified when a stack is created.
* @return {@code this}
*/
public Builder withDefault(@javax.annotation.Nullable final java.lang.Object value) {
this._default = value;
return this;
}
/**
* Sets the value of Description
* @param value A string of up to 4000 characters that describes the parameter.
* @return {@code this}
*/
public Builder withDescription(@javax.annotation.Nullable final java.lang.String value) {
this._description = value;
return this;
}
/**
* Sets the value of MaxLength
* @param value An integer value that determines the largest number of characters you want to allow for String types.
* @return {@code this}
*/
public Builder withMaxLength(@javax.annotation.Nullable final java.lang.Number value) {
this._maxLength = value;
return this;
}
/**
* Sets the value of MaxValue
* @param value A numeric value that determines the largest numeric value you want to allow for Number types.
* @return {@code this}
*/
public Builder withMaxValue(@javax.annotation.Nullable final java.lang.Number value) {
this._maxValue = value;
return this;
}
/**
* Sets the value of MinLength
* @param value An integer value that determines the smallest number of characters you want to allow for String types.
* @return {@code this}
*/
public Builder withMinLength(@javax.annotation.Nullable final java.lang.Number value) {
this._minLength = value;
return this;
}
/**
* Sets the value of MinValue
* @param value A numeric value that determines the smallest numeric value you want to allow for Number types.
* @return {@code this}
*/
public Builder withMinValue(@javax.annotation.Nullable final java.lang.Number value) {
this._minValue = value;
return this;
}
/**
* Sets the value of NoEcho
* @param value Whether to mask the parameter value when anyone makes a call that describes the stack. If you set the value to ``true``, the parameter value is masked with asterisks (``*****``).
* @return {@code this}
*/
public Builder withNoEcho(@javax.annotation.Nullable final java.lang.Boolean value) {
this._noEcho = value;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link CfnParameterProps}
* @throws NullPointerException if any required attribute was not provided
*/
public CfnParameterProps build() {
return new CfnParameterProps() {
private final java.lang.String $type = java.util.Objects.requireNonNull(_type, "type is required");
@javax.annotation.Nullable
private final java.lang.String $allowedPattern = _allowedPattern;
@javax.annotation.Nullable
private final java.util.List $allowedValues = _allowedValues;
@javax.annotation.Nullable
private final java.lang.String $constraintDescription = _constraintDescription;
@javax.annotation.Nullable
private final java.lang.Object $default = _default;
@javax.annotation.Nullable
private final java.lang.String $description = _description;
@javax.annotation.Nullable
private final java.lang.Number $maxLength = _maxLength;
@javax.annotation.Nullable
private final java.lang.Number $maxValue = _maxValue;
@javax.annotation.Nullable
private final java.lang.Number $minLength = _minLength;
@javax.annotation.Nullable
private final java.lang.Number $minValue = _minValue;
@javax.annotation.Nullable
private final java.lang.Boolean $noEcho = _noEcho;
@Override
public java.lang.String getType() {
return this.$type;
}
@Override
public java.lang.String getAllowedPattern() {
return this.$allowedPattern;
}
@Override
public java.util.List getAllowedValues() {
return this.$allowedValues;
}
@Override
public java.lang.String getConstraintDescription() {
return this.$constraintDescription;
}
@Override
public java.lang.Object getDefault() {
return this.$default;
}
@Override
public java.lang.String getDescription() {
return this.$description;
}
@Override
public java.lang.Number getMaxLength() {
return this.$maxLength;
}
@Override
public java.lang.Number getMaxValue() {
return this.$maxValue;
}
@Override
public java.lang.Number getMinLength() {
return this.$minLength;
}
@Override
public java.lang.Number getMinValue() {
return this.$minValue;
}
@Override
public java.lang.Boolean getNoEcho() {
return this.$noEcho;
}
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("type", om.valueToTree(this.getType()));
obj.set("allowedPattern", om.valueToTree(this.getAllowedPattern()));
obj.set("allowedValues", om.valueToTree(this.getAllowedValues()));
obj.set("constraintDescription", om.valueToTree(this.getConstraintDescription()));
obj.set("default", om.valueToTree(this.getDefault()));
obj.set("description", om.valueToTree(this.getDescription()));
obj.set("maxLength", om.valueToTree(this.getMaxLength()));
obj.set("maxValue", om.valueToTree(this.getMaxValue()));
obj.set("minLength", om.valueToTree(this.getMinLength()));
obj.set("minValue", om.valueToTree(this.getMinValue()));
obj.set("noEcho", om.valueToTree(this.getNoEcho()));
return obj;
}
};
}
}
/**
* A proxy class which represents a concrete javascript instance of this type.
*/
final static class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements software.amazon.awscdk.CfnParameterProps {
protected Jsii$Proxy(final software.amazon.jsii.JsiiObject.InitializationMode mode) {
super(mode);
}
/**
* The data type for the parameter (DataType).
*/
@Override
public java.lang.String getType() {
return this.jsiiGet("type", java.lang.String.class);
}
/**
* A regular expression that represents the patterns to allow for String types.
*/
@Override
@javax.annotation.Nullable
public java.lang.String getAllowedPattern() {
return this.jsiiGet("allowedPattern", java.lang.String.class);
}
/**
* An array containing the list of values allowed for the parameter.
*/
@Override
@javax.annotation.Nullable
public java.util.List getAllowedValues() {
return this.jsiiGet("allowedValues", java.util.List.class);
}
/**
* A string that explains a constraint when the constraint is violated. For example, without a constraint description, a parameter that has an allowed pattern of [A-Za-z0-9]+ displays the following error message when the user specifies an invalid value:.
*/
@Override
@javax.annotation.Nullable
public java.lang.String getConstraintDescription() {
return this.jsiiGet("constraintDescription", java.lang.String.class);
}
/**
* A value of the appropriate type for the template to use if no value is specified when a stack is created.
*
* If you define constraints for the parameter, you must specify
* a value that adheres to those constraints.
*/
@Override
@javax.annotation.Nullable
public java.lang.Object getDefault() {
return this.jsiiGet("default", java.lang.Object.class);
}
/**
* A string of up to 4000 characters that describes the parameter.
*/
@Override
@javax.annotation.Nullable
public java.lang.String getDescription() {
return this.jsiiGet("description", java.lang.String.class);
}
/**
* An integer value that determines the largest number of characters you want to allow for String types.
*/
@Override
@javax.annotation.Nullable
public java.lang.Number getMaxLength() {
return this.jsiiGet("maxLength", java.lang.Number.class);
}
/**
* A numeric value that determines the largest numeric value you want to allow for Number types.
*/
@Override
@javax.annotation.Nullable
public java.lang.Number getMaxValue() {
return this.jsiiGet("maxValue", java.lang.Number.class);
}
/**
* An integer value that determines the smallest number of characters you want to allow for String types.
*/
@Override
@javax.annotation.Nullable
public java.lang.Number getMinLength() {
return this.jsiiGet("minLength", java.lang.Number.class);
}
/**
* A numeric value that determines the smallest numeric value you want to allow for Number types.
*/
@Override
@javax.annotation.Nullable
public java.lang.Number getMinValue() {
return this.jsiiGet("minValue", java.lang.Number.class);
}
/**
* Whether to mask the parameter value when anyone makes a call that describes the stack. If you set the value to ``true``, the parameter value is masked with asterisks (``*****``).
*/
@Override
@javax.annotation.Nullable
public java.lang.Boolean getNoEcho() {
return this.jsiiGet("noEcho", java.lang.Boolean.class);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy