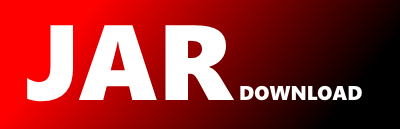
software.amazon.awscdk.CfnResource Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cdk Show documentation
Show all versions of cdk Show documentation
AWS Cloud Development Kit Core Library
package software.amazon.awscdk;
/**
* Represents a CloudFormation resource.
*/
@javax.annotation.Generated(value = "jsii-pacmak/0.10.5 (build 46bc9b0)", date = "2019-05-06T20:49:40.940Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.$Module.class, fqn = "@aws-cdk/cdk.CfnResource")
public class CfnResource extends software.amazon.awscdk.CfnRefElement {
protected CfnResource(final software.amazon.jsii.JsiiObject.InitializationMode mode) {
super(mode);
}
/**
* Creates a resource construct.
*/
public CfnResource(final software.amazon.awscdk.Construct scope, final java.lang.String id, final software.amazon.awscdk.CfnResourceProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.Jsii);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* A decoration used to create a CloudFormation attribute property.
*
* @param customName Custom name for the attribute (default is the name of the property) NOTE: we return "any" here to satistfy jsii, which doesn't support lambdas.
*/
@javax.annotation.Nullable
public static java.lang.Object attribute(@javax.annotation.Nullable final java.lang.String customName) {
return software.amazon.jsii.JsiiObject.jsiiStaticCall(software.amazon.awscdk.CfnResource.class, "attribute", java.lang.Object.class, new Object[] { customName });
}
/**
* A decoration used to create a CloudFormation attribute property.
*/
@javax.annotation.Nullable
public static java.lang.Object attribute() {
return software.amazon.jsii.JsiiObject.jsiiStaticCall(software.amazon.awscdk.CfnResource.class, "attribute", java.lang.Object.class);
}
/**
* Check whether the given construct is a CfnResource.
*/
public static java.lang.Boolean isCfnResource(final software.amazon.awscdk.IConstruct construct) {
return software.amazon.jsii.JsiiObject.jsiiStaticCall(software.amazon.awscdk.CfnResource.class, "isCfnResource", java.lang.Boolean.class, new Object[] { java.util.Objects.requireNonNull(construct, "construct is required") });
}
/**
* Check whether the given construct is Taggable.
*/
public static java.lang.Boolean isTaggable(@javax.annotation.Nullable final java.lang.Object construct) {
return software.amazon.jsii.JsiiObject.jsiiStaticCall(software.amazon.awscdk.CfnResource.class, "isTaggable", java.lang.Boolean.class, new Object[] { construct });
}
/**
* Syntactic sugar for `addOverride(path, undefined)`.
*
* @param path The path of the value to delete.
*/
public void addDeletionOverride(final java.lang.String path) {
this.jsiiCall("addDeletionOverride", Void.class, new Object[] { java.util.Objects.requireNonNull(path, "path is required") });
}
/**
* Indicates that this resource depends on another resource and cannot be provisioned unless the other resource has been successfully provisioned.
*/
public void addDependsOn(final software.amazon.awscdk.CfnResource resource) {
this.jsiiCall("addDependsOn", Void.class, new Object[] { java.util.Objects.requireNonNull(resource, "resource is required") });
}
/**
* Adds an override to the synthesized CloudFormation resource.
*
* To add a
* property override, either use `addPropertyOverride` or prefix `path` with
* "Properties." (i.e. `Properties.TopicName`).
*
* @param path The path of the property, you can use dot notation to override values in complex types.
* @param value The value.
*/
public void addOverride(final java.lang.String path, @javax.annotation.Nullable final java.lang.Object value) {
this.jsiiCall("addOverride", Void.class, new Object[] { java.util.Objects.requireNonNull(path, "path is required"), value });
}
/**
* Adds an override that deletes the value of a property from the resource definition.
*
* @param propertyPath The path to the property.
*/
public void addPropertyDeletionOverride(final java.lang.String propertyPath) {
this.jsiiCall("addPropertyDeletionOverride", Void.class, new Object[] { java.util.Objects.requireNonNull(propertyPath, "propertyPath is required") });
}
/**
* Adds an override to a resource property.
*
* Syntactic sugar for `addOverride("Properties.<...>", value)`.
*
* @param propertyPath The path of the property.
* @param value The value.
*/
public void addPropertyOverride(final java.lang.String propertyPath, @javax.annotation.Nullable final java.lang.Object value) {
this.jsiiCall("addPropertyOverride", Void.class, new Object[] { java.util.Objects.requireNonNull(propertyPath, "propertyPath is required"), value });
}
/**
* Returns a token for an runtime attribute of this resource. Ideally, use generated attribute accessors (e.g. `resource.arn`), but this can be used for future compatibility in case there is no generated attribute.
*
* @param attributeName The name of the attribute.
*/
public software.amazon.awscdk.CfnReference getAtt(final java.lang.String attributeName) {
return this.jsiiCall("getAtt", software.amazon.awscdk.CfnReference.class, new Object[] { java.util.Objects.requireNonNull(attributeName, "attributeName is required") });
}
protected java.util.Map renderProperties(@javax.annotation.Nullable final java.lang.Object properties) {
return this.jsiiCall("renderProperties", java.util.Map.class, new Object[] { properties });
}
/**
* Options for this resource, such as condition, update policy etc.
*/
public software.amazon.awscdk.IResourceOptions getOptions() {
return this.jsiiGet("options", software.amazon.awscdk.IResourceOptions.class);
}
/**
* AWS resource properties.
*
* This object is rendered via a call to "renderProperties(this.properties)".
*/
@javax.annotation.Nullable
protected java.lang.Object getProperties() {
return this.jsiiGet("properties", java.lang.Object.class);
}
/**
* AWS resource type.
*/
public java.lang.String getResourceType() {
return this.jsiiGet("resourceType", java.lang.String.class);
}
/**
* AWS resource property overrides.
*
* During synthesis, the method "renderProperties(this.overrides)" is called
* with this object, and merged on top of the output of
* "renderProperties(this.properties)".
*
* Derived classes should expose a strongly-typed version of this object as
* a public property called `propertyOverrides`.
*/
@javax.annotation.Nullable
protected java.lang.Object getUntypedPropertyOverrides() {
return this.jsiiGet("untypedPropertyOverrides", java.lang.Object.class);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy