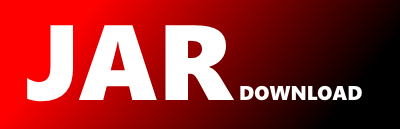
software.amazon.awscdk.CfnRuleProps Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cdk Show documentation
Show all versions of cdk Show documentation
AWS Cloud Development Kit Core Library
package software.amazon.awscdk;
/**
* A rule can include a RuleCondition property and must include an Assertions property. For each rule, you can define only one rule condition; you can define one or more asserts within the Assertions property. You define a rule condition and assertions by using rule-specific intrinsic functions.
*
* You can use the following rule-specific intrinsic functions to define rule conditions and assertions:
*
* Fn::And
* Fn::Contains
* Fn::EachMemberEquals
* Fn::EachMemberIn
* Fn::Equals
* Fn::If
* Fn::Not
* Fn::Or
* Fn::RefAll
* Fn::ValueOf
* Fn::ValueOfAll
*
* https://docs.aws.amazon.com/servicecatalog/latest/adminguide/reference-template_constraint_rules.html
*/
@javax.annotation.Generated(value = "jsii-pacmak/0.10.5 (build 46bc9b0)", date = "2019-05-06T20:49:40.941Z")
public interface CfnRuleProps extends software.amazon.jsii.JsiiSerializable {
/**
* Assertions which define the rule.
*/
java.util.List getAssertions();
/**
* If the rule condition evaluates to false, the rule doesn't take effect. If the function in the rule condition evaluates to true, expressions in each assert are evaluated and applied.
*/
software.amazon.awscdk.ICfnConditionExpression getRuleCondition();
/**
* @return a {@link Builder} of {@link CfnRuleProps}
*/
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link CfnRuleProps}
*/
final class Builder {
@javax.annotation.Nullable
private java.util.List _assertions;
@javax.annotation.Nullable
private software.amazon.awscdk.ICfnConditionExpression _ruleCondition;
/**
* Sets the value of Assertions
* @param value Assertions which define the rule.
* @return {@code this}
*/
public Builder withAssertions(@javax.annotation.Nullable final java.util.List value) {
this._assertions = value;
return this;
}
/**
* Sets the value of RuleCondition
* @param value If the rule condition evaluates to false, the rule doesn't take effect. If the function in the rule condition evaluates to true, expressions in each assert are evaluated and applied.
* @return {@code this}
*/
public Builder withRuleCondition(@javax.annotation.Nullable final software.amazon.awscdk.ICfnConditionExpression value) {
this._ruleCondition = value;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link CfnRuleProps}
* @throws NullPointerException if any required attribute was not provided
*/
public CfnRuleProps build() {
return new CfnRuleProps() {
@javax.annotation.Nullable
private final java.util.List $assertions = _assertions;
@javax.annotation.Nullable
private final software.amazon.awscdk.ICfnConditionExpression $ruleCondition = _ruleCondition;
@Override
public java.util.List getAssertions() {
return this.$assertions;
}
@Override
public software.amazon.awscdk.ICfnConditionExpression getRuleCondition() {
return this.$ruleCondition;
}
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("assertions", om.valueToTree(this.getAssertions()));
obj.set("ruleCondition", om.valueToTree(this.getRuleCondition()));
return obj;
}
};
}
}
/**
* A proxy class which represents a concrete javascript instance of this type.
*/
final static class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements software.amazon.awscdk.CfnRuleProps {
protected Jsii$Proxy(final software.amazon.jsii.JsiiObject.InitializationMode mode) {
super(mode);
}
/**
* Assertions which define the rule.
*/
@Override
@javax.annotation.Nullable
public java.util.List getAssertions() {
return this.jsiiGet("assertions", java.util.List.class);
}
/**
* If the rule condition evaluates to false, the rule doesn't take effect. If the function in the rule condition evaluates to true, expressions in each assert are evaluated and applied.
*/
@Override
@javax.annotation.Nullable
public software.amazon.awscdk.ICfnConditionExpression getRuleCondition() {
return this.jsiiGet("ruleCondition", software.amazon.awscdk.ICfnConditionExpression.class);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy