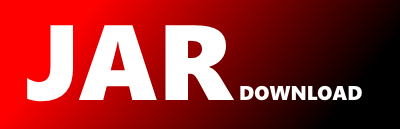
software.amazon.awscdk.CodeDeployLambdaAliasUpdate Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cdk Show documentation
Show all versions of cdk Show documentation
AWS Cloud Development Kit Core Library
package software.amazon.awscdk;
/**
* To perform an AWS CodeDeploy deployment when the version changes on an AWS::Lambda::Alias resource, use the CodeDeployLambdaAliasUpdate update policy.
*/
@javax.annotation.Generated(value = "jsii-pacmak/0.10.5 (build 46bc9b0)", date = "2019-05-06T20:49:40.942Z")
public interface CodeDeployLambdaAliasUpdate extends software.amazon.jsii.JsiiSerializable {
/**
* The name of the AWS CodeDeploy application.
*/
java.lang.String getApplicationName();
/**
* The name of the AWS CodeDeploy deployment group.
*
* This is where the traffic-shifting policy is set.
*/
java.lang.String getDeploymentGroupName();
/**
* The name of the Lambda function to run after traffic routing completes.
*/
java.lang.String getAfterAllowTrafficHook();
/**
* The name of the Lambda function to run before traffic routing starts.
*/
java.lang.String getBeforeAllowTrafficHook();
/**
* @return a {@link Builder} of {@link CodeDeployLambdaAliasUpdate}
*/
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link CodeDeployLambdaAliasUpdate}
*/
final class Builder {
private java.lang.String _applicationName;
private java.lang.String _deploymentGroupName;
@javax.annotation.Nullable
private java.lang.String _afterAllowTrafficHook;
@javax.annotation.Nullable
private java.lang.String _beforeAllowTrafficHook;
/**
* Sets the value of ApplicationName
* @param value The name of the AWS CodeDeploy application.
* @return {@code this}
*/
public Builder withApplicationName(final java.lang.String value) {
this._applicationName = java.util.Objects.requireNonNull(value, "applicationName is required");
return this;
}
/**
* Sets the value of DeploymentGroupName
* @param value The name of the AWS CodeDeploy deployment group.
* @return {@code this}
*/
public Builder withDeploymentGroupName(final java.lang.String value) {
this._deploymentGroupName = java.util.Objects.requireNonNull(value, "deploymentGroupName is required");
return this;
}
/**
* Sets the value of AfterAllowTrafficHook
* @param value The name of the Lambda function to run after traffic routing completes.
* @return {@code this}
*/
public Builder withAfterAllowTrafficHook(@javax.annotation.Nullable final java.lang.String value) {
this._afterAllowTrafficHook = value;
return this;
}
/**
* Sets the value of BeforeAllowTrafficHook
* @param value The name of the Lambda function to run before traffic routing starts.
* @return {@code this}
*/
public Builder withBeforeAllowTrafficHook(@javax.annotation.Nullable final java.lang.String value) {
this._beforeAllowTrafficHook = value;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link CodeDeployLambdaAliasUpdate}
* @throws NullPointerException if any required attribute was not provided
*/
public CodeDeployLambdaAliasUpdate build() {
return new CodeDeployLambdaAliasUpdate() {
private final java.lang.String $applicationName = java.util.Objects.requireNonNull(_applicationName, "applicationName is required");
private final java.lang.String $deploymentGroupName = java.util.Objects.requireNonNull(_deploymentGroupName, "deploymentGroupName is required");
@javax.annotation.Nullable
private final java.lang.String $afterAllowTrafficHook = _afterAllowTrafficHook;
@javax.annotation.Nullable
private final java.lang.String $beforeAllowTrafficHook = _beforeAllowTrafficHook;
@Override
public java.lang.String getApplicationName() {
return this.$applicationName;
}
@Override
public java.lang.String getDeploymentGroupName() {
return this.$deploymentGroupName;
}
@Override
public java.lang.String getAfterAllowTrafficHook() {
return this.$afterAllowTrafficHook;
}
@Override
public java.lang.String getBeforeAllowTrafficHook() {
return this.$beforeAllowTrafficHook;
}
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("applicationName", om.valueToTree(this.getApplicationName()));
obj.set("deploymentGroupName", om.valueToTree(this.getDeploymentGroupName()));
obj.set("afterAllowTrafficHook", om.valueToTree(this.getAfterAllowTrafficHook()));
obj.set("beforeAllowTrafficHook", om.valueToTree(this.getBeforeAllowTrafficHook()));
return obj;
}
};
}
}
/**
* A proxy class which represents a concrete javascript instance of this type.
*/
final static class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements software.amazon.awscdk.CodeDeployLambdaAliasUpdate {
protected Jsii$Proxy(final software.amazon.jsii.JsiiObject.InitializationMode mode) {
super(mode);
}
/**
* The name of the AWS CodeDeploy application.
*/
@Override
public java.lang.String getApplicationName() {
return this.jsiiGet("applicationName", java.lang.String.class);
}
/**
* The name of the AWS CodeDeploy deployment group.
*
* This is where the traffic-shifting policy is set.
*/
@Override
public java.lang.String getDeploymentGroupName() {
return this.jsiiGet("deploymentGroupName", java.lang.String.class);
}
/**
* The name of the Lambda function to run after traffic routing completes.
*/
@Override
@javax.annotation.Nullable
public java.lang.String getAfterAllowTrafficHook() {
return this.jsiiGet("afterAllowTrafficHook", java.lang.String.class);
}
/**
* The name of the Lambda function to run before traffic routing starts.
*/
@Override
@javax.annotation.Nullable
public java.lang.String getBeforeAllowTrafficHook() {
return this.jsiiGet("beforeAllowTrafficHook", java.lang.String.class);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy