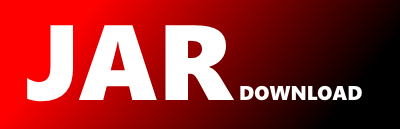
software.amazon.awscdk.CreationPolicy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cdk Show documentation
Show all versions of cdk Show documentation
AWS Cloud Development Kit Core Library
package software.amazon.awscdk;
/**
* Associate the CreationPolicy attribute with a resource to prevent its status from reaching create complete until AWS CloudFormation receives a specified number of success signals or the timeout period is exceeded.
*
* To signal a
* resource, you can use the cfn-signal helper script or SignalResource API. AWS CloudFormation publishes valid signals
* to the stack events so that you track the number of signals sent.
*
* The creation policy is invoked only when AWS CloudFormation creates the associated resource. Currently, the only
* AWS CloudFormation resources that support creation policies are AWS::AutoScaling::AutoScalingGroup, AWS::EC2::Instance,
* and AWS::CloudFormation::WaitCondition.
*
* Use the CreationPolicy attribute when you want to wait on resource configuration actions before stack creation proceeds.
* For example, if you install and configure software applications on an EC2 instance, you might want those applications to
* be running before proceeding. In such cases, you can add a CreationPolicy attribute to the instance, and then send a success
* signal to the instance after the applications are installed and configured. For a detailed example, see Deploying Applications
* on Amazon EC2 with AWS CloudFormation.
*/
@javax.annotation.Generated(value = "jsii-pacmak/0.10.5 (build 46bc9b0)", date = "2019-05-06T20:49:40.944Z")
public interface CreationPolicy extends software.amazon.jsii.JsiiSerializable {
/**
* For an Auto Scaling group replacement update, specifies how many instances must signal success for the update to succeed.
*/
software.amazon.awscdk.AutoScalingCreationPolicy getAutoScalingCreationPolicy();
/**
* When AWS CloudFormation creates the associated resource, configures the number of required success signals and the length of time that AWS CloudFormation waits for those signals.
*/
software.amazon.awscdk.ResourceSignal getResourceSignal();
/**
* @return a {@link Builder} of {@link CreationPolicy}
*/
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link CreationPolicy}
*/
final class Builder {
@javax.annotation.Nullable
private software.amazon.awscdk.AutoScalingCreationPolicy _autoScalingCreationPolicy;
@javax.annotation.Nullable
private software.amazon.awscdk.ResourceSignal _resourceSignal;
/**
* Sets the value of AutoScalingCreationPolicy
* @param value For an Auto Scaling group replacement update, specifies how many instances must signal success for the update to succeed.
* @return {@code this}
*/
public Builder withAutoScalingCreationPolicy(@javax.annotation.Nullable final software.amazon.awscdk.AutoScalingCreationPolicy value) {
this._autoScalingCreationPolicy = value;
return this;
}
/**
* Sets the value of ResourceSignal
* @param value When AWS CloudFormation creates the associated resource, configures the number of required success signals and the length of time that AWS CloudFormation waits for those signals.
* @return {@code this}
*/
public Builder withResourceSignal(@javax.annotation.Nullable final software.amazon.awscdk.ResourceSignal value) {
this._resourceSignal = value;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link CreationPolicy}
* @throws NullPointerException if any required attribute was not provided
*/
public CreationPolicy build() {
return new CreationPolicy() {
@javax.annotation.Nullable
private final software.amazon.awscdk.AutoScalingCreationPolicy $autoScalingCreationPolicy = _autoScalingCreationPolicy;
@javax.annotation.Nullable
private final software.amazon.awscdk.ResourceSignal $resourceSignal = _resourceSignal;
@Override
public software.amazon.awscdk.AutoScalingCreationPolicy getAutoScalingCreationPolicy() {
return this.$autoScalingCreationPolicy;
}
@Override
public software.amazon.awscdk.ResourceSignal getResourceSignal() {
return this.$resourceSignal;
}
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("autoScalingCreationPolicy", om.valueToTree(this.getAutoScalingCreationPolicy()));
obj.set("resourceSignal", om.valueToTree(this.getResourceSignal()));
return obj;
}
};
}
}
/**
* A proxy class which represents a concrete javascript instance of this type.
*/
final static class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements software.amazon.awscdk.CreationPolicy {
protected Jsii$Proxy(final software.amazon.jsii.JsiiObject.InitializationMode mode) {
super(mode);
}
/**
* For an Auto Scaling group replacement update, specifies how many instances must signal success for the update to succeed.
*/
@Override
@javax.annotation.Nullable
public software.amazon.awscdk.AutoScalingCreationPolicy getAutoScalingCreationPolicy() {
return this.jsiiGet("autoScalingCreationPolicy", software.amazon.awscdk.AutoScalingCreationPolicy.class);
}
/**
* When AWS CloudFormation creates the associated resource, configures the number of required success signals and the length of time that AWS CloudFormation waits for those signals.
*/
@Override
@javax.annotation.Nullable
public software.amazon.awscdk.ResourceSignal getResourceSignal() {
return this.jsiiGet("resourceSignal", software.amazon.awscdk.ResourceSignal.class);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy