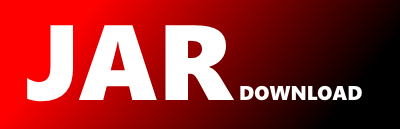
software.amazon.awscdk.IResourceOptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cdk Show documentation
Show all versions of cdk Show documentation
AWS Cloud Development Kit Core Library
package software.amazon.awscdk;
@javax.annotation.Generated(value = "jsii-pacmak/0.10.5 (build 46bc9b0)", date = "2019-05-06T20:49:40.952Z")
public interface IResourceOptions extends software.amazon.jsii.JsiiSerializable {
/**
* A condition to associate with this resource.
*
* This means that only if the condition evaluates to 'true' when the stack
* is deployed, the resource will be included. This is provided to allow CDK projects to produce legacy templates, but noramlly
* there is no need to use it in CDK projects.
*/
software.amazon.awscdk.CfnCondition getCondition();
/**
* A condition to associate with this resource.
*
* This means that only if the condition evaluates to 'true' when the stack
* is deployed, the resource will be included. This is provided to allow CDK projects to produce legacy templates, but noramlly
* there is no need to use it in CDK projects.
*/
void setCondition(final software.amazon.awscdk.CfnCondition value);
/**
* Associate the CreationPolicy attribute with a resource to prevent its status from reaching create complete until AWS CloudFormation receives a specified number of success signals or the timeout period is exceeded.
*
* To signal a
* resource, you can use the cfn-signal helper script or SignalResource API. AWS CloudFormation publishes valid signals
* to the stack events so that you track the number of signals sent.
*/
software.amazon.awscdk.CreationPolicy getCreationPolicy();
/**
* Associate the CreationPolicy attribute with a resource to prevent its status from reaching create complete until AWS CloudFormation receives a specified number of success signals or the timeout period is exceeded.
*
* To signal a
* resource, you can use the cfn-signal helper script or SignalResource API. AWS CloudFormation publishes valid signals
* to the stack events so that you track the number of signals sent.
*/
void setCreationPolicy(final software.amazon.awscdk.CreationPolicy value);
/**
* With the DeletionPolicy attribute you can preserve or (in some cases) backup a resource when its stack is deleted. You specify a DeletionPolicy attribute for each resource that you want to control. If a resource has no DeletionPolicy attribute, AWS CloudFormation deletes the resource by default. Note that this capability also applies to update operations that lead to resources being removed.
*/
software.amazon.awscdk.DeletionPolicy getDeletionPolicy();
/**
* With the DeletionPolicy attribute you can preserve or (in some cases) backup a resource when its stack is deleted. You specify a DeletionPolicy attribute for each resource that you want to control. If a resource has no DeletionPolicy attribute, AWS CloudFormation deletes the resource by default. Note that this capability also applies to update operations that lead to resources being removed.
*/
void setDeletionPolicy(final software.amazon.awscdk.DeletionPolicy value);
/**
* Metadata associated with the CloudFormation resource.
*
* This is not the same as the construct metadata which can be added
* using construct.addMetadata(), but would not appear in the CloudFormation template automatically.
*/
java.util.Map getMetadata();
/**
* Metadata associated with the CloudFormation resource.
*
* This is not the same as the construct metadata which can be added
* using construct.addMetadata(), but would not appear in the CloudFormation template automatically.
*/
void setMetadata(final java.util.Map value);
/**
* Use the UpdatePolicy attribute to specify how AWS CloudFormation handles updates to the AWS::AutoScaling::AutoScalingGroup resource.
*
* AWS CloudFormation invokes one of three update policies depending on the type of change you make or whether a
* scheduled action is associated with the Auto Scaling group.
*/
software.amazon.awscdk.UpdatePolicy getUpdatePolicy();
/**
* Use the UpdatePolicy attribute to specify how AWS CloudFormation handles updates to the AWS::AutoScaling::AutoScalingGroup resource.
*
* AWS CloudFormation invokes one of three update policies depending on the type of change you make or whether a
* scheduled action is associated with the Auto Scaling group.
*/
void setUpdatePolicy(final software.amazon.awscdk.UpdatePolicy value);
/**
* Use the UpdateReplacePolicy attribute to retain or (in some cases) backup the existing physical instance of a resource when it is replaced during a stack update operation.
*/
software.amazon.awscdk.DeletionPolicy getUpdateReplacePolicy();
/**
* Use the UpdateReplacePolicy attribute to retain or (in some cases) backup the existing physical instance of a resource when it is replaced during a stack update operation.
*/
void setUpdateReplacePolicy(final software.amazon.awscdk.DeletionPolicy value);
/**
* A proxy class which represents a concrete javascript instance of this type.
*/
final static class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements software.amazon.awscdk.IResourceOptions {
protected Jsii$Proxy(final software.amazon.jsii.JsiiObject.InitializationMode mode) {
super(mode);
}
/**
* A condition to associate with this resource.
*
* This means that only if the condition evaluates to 'true' when the stack
* is deployed, the resource will be included. This is provided to allow CDK projects to produce legacy templates, but noramlly
* there is no need to use it in CDK projects.
*/
@Override
@javax.annotation.Nullable
public software.amazon.awscdk.CfnCondition getCondition() {
return this.jsiiGet("condition", software.amazon.awscdk.CfnCondition.class);
}
/**
* A condition to associate with this resource.
*
* This means that only if the condition evaluates to 'true' when the stack
* is deployed, the resource will be included. This is provided to allow CDK projects to produce legacy templates, but noramlly
* there is no need to use it in CDK projects.
*/
@Override
public void setCondition(@javax.annotation.Nullable final software.amazon.awscdk.CfnCondition value) {
this.jsiiSet("condition", value);
}
/**
* Associate the CreationPolicy attribute with a resource to prevent its status from reaching create complete until AWS CloudFormation receives a specified number of success signals or the timeout period is exceeded.
*
* To signal a
* resource, you can use the cfn-signal helper script or SignalResource API. AWS CloudFormation publishes valid signals
* to the stack events so that you track the number of signals sent.
*/
@Override
@javax.annotation.Nullable
public software.amazon.awscdk.CreationPolicy getCreationPolicy() {
return this.jsiiGet("creationPolicy", software.amazon.awscdk.CreationPolicy.class);
}
/**
* Associate the CreationPolicy attribute with a resource to prevent its status from reaching create complete until AWS CloudFormation receives a specified number of success signals or the timeout period is exceeded.
*
* To signal a
* resource, you can use the cfn-signal helper script or SignalResource API. AWS CloudFormation publishes valid signals
* to the stack events so that you track the number of signals sent.
*/
@Override
public void setCreationPolicy(@javax.annotation.Nullable final software.amazon.awscdk.CreationPolicy value) {
this.jsiiSet("creationPolicy", value);
}
/**
* With the DeletionPolicy attribute you can preserve or (in some cases) backup a resource when its stack is deleted. You specify a DeletionPolicy attribute for each resource that you want to control. If a resource has no DeletionPolicy attribute, AWS CloudFormation deletes the resource by default. Note that this capability also applies to update operations that lead to resources being removed.
*/
@Override
@javax.annotation.Nullable
public software.amazon.awscdk.DeletionPolicy getDeletionPolicy() {
return this.jsiiGet("deletionPolicy", software.amazon.awscdk.DeletionPolicy.class);
}
/**
* With the DeletionPolicy attribute you can preserve or (in some cases) backup a resource when its stack is deleted. You specify a DeletionPolicy attribute for each resource that you want to control. If a resource has no DeletionPolicy attribute, AWS CloudFormation deletes the resource by default. Note that this capability also applies to update operations that lead to resources being removed.
*/
@Override
public void setDeletionPolicy(@javax.annotation.Nullable final software.amazon.awscdk.DeletionPolicy value) {
this.jsiiSet("deletionPolicy", value);
}
/**
* Metadata associated with the CloudFormation resource.
*
* This is not the same as the construct metadata which can be added
* using construct.addMetadata(), but would not appear in the CloudFormation template automatically.
*/
@Override
@javax.annotation.Nullable
public java.util.Map getMetadata() {
return this.jsiiGet("metadata", java.util.Map.class);
}
/**
* Metadata associated with the CloudFormation resource.
*
* This is not the same as the construct metadata which can be added
* using construct.addMetadata(), but would not appear in the CloudFormation template automatically.
*/
@Override
public void setMetadata(@javax.annotation.Nullable final java.util.Map value) {
this.jsiiSet("metadata", value);
}
/**
* Use the UpdatePolicy attribute to specify how AWS CloudFormation handles updates to the AWS::AutoScaling::AutoScalingGroup resource.
*
* AWS CloudFormation invokes one of three update policies depending on the type of change you make or whether a
* scheduled action is associated with the Auto Scaling group.
*/
@Override
@javax.annotation.Nullable
public software.amazon.awscdk.UpdatePolicy getUpdatePolicy() {
return this.jsiiGet("updatePolicy", software.amazon.awscdk.UpdatePolicy.class);
}
/**
* Use the UpdatePolicy attribute to specify how AWS CloudFormation handles updates to the AWS::AutoScaling::AutoScalingGroup resource.
*
* AWS CloudFormation invokes one of three update policies depending on the type of change you make or whether a
* scheduled action is associated with the Auto Scaling group.
*/
@Override
public void setUpdatePolicy(@javax.annotation.Nullable final software.amazon.awscdk.UpdatePolicy value) {
this.jsiiSet("updatePolicy", value);
}
/**
* Use the UpdateReplacePolicy attribute to retain or (in some cases) backup the existing physical instance of a resource when it is replaced during a stack update operation.
*/
@Override
@javax.annotation.Nullable
public software.amazon.awscdk.DeletionPolicy getUpdateReplacePolicy() {
return this.jsiiGet("updateReplacePolicy", software.amazon.awscdk.DeletionPolicy.class);
}
/**
* Use the UpdateReplacePolicy attribute to retain or (in some cases) backup the existing physical instance of a resource when it is replaced during a stack update operation.
*/
@Override
public void setUpdateReplacePolicy(@javax.annotation.Nullable final software.amazon.awscdk.DeletionPolicy value) {
this.jsiiSet("updateReplacePolicy", value);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy