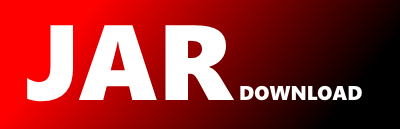
software.amazon.awscdk.ISessionStore Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cdk Show documentation
Show all versions of cdk Show documentation
AWS Cloud Development Kit Core Library
package software.amazon.awscdk;
@javax.annotation.Generated(value = "jsii-pacmak/0.10.5 (build 46bc9b0)", date = "2019-05-06T20:49:40.953Z")
public interface ISessionStore extends software.amazon.jsii.JsiiSerializable {
/**
* @return true if the file `fileName` exists in the store.
* @param name The name of the file or directory to look up.
*/
java.lang.Boolean exists(final java.lang.String name);
/**
* List all top-level files that were emitted to the store.
*/
java.util.List list();
/**
* Do not allow further writes into the store.
*/
void lock();
/**
* Creates a directory and returns it's full path.
*
* @param directoryName The name of the directory to create.
*/
java.lang.String mkdir(final java.lang.String directoryName);
/**
* Returns the list of files in a directory.
*
* @param directoryName The name of the artifact.
*/
java.util.List readdir(final java.lang.String directoryName);
/**
* Reads a file from the store.
*
* @param fileName The name of the file.
*/
java.lang.Object readFile(final java.lang.String fileName);
/**
* Reads a JSON object from the store.
*/
java.lang.Object readJson(final java.lang.String fileName);
/**
* Writes a file into the store.
*
* @param artifactName The name of the file.
* @param data The contents of the file.
*/
void writeFile(final java.lang.String artifactName, @javax.annotation.Nullable final java.lang.Object data);
/**
* Writes a formatted JSON output file to the store.
*
* @param artifactName the name of the artifact.
* @param json the JSON object.
*/
void writeJson(final java.lang.String artifactName, @javax.annotation.Nullable final java.lang.Object json);
/**
* A proxy class which represents a concrete javascript instance of this type.
*/
final static class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements software.amazon.awscdk.ISessionStore {
protected Jsii$Proxy(final software.amazon.jsii.JsiiObject.InitializationMode mode) {
super(mode);
}
/**
* @return true if the file `fileName` exists in the store.
* @param name The name of the file or directory to look up.
*/
@Override
public java.lang.Boolean exists(final java.lang.String name) {
return this.jsiiCall("exists", java.lang.Boolean.class, new Object[] { java.util.Objects.requireNonNull(name, "name is required") });
}
/**
* List all top-level files that were emitted to the store.
*/
@Override
public java.util.List list() {
return this.jsiiCall("list", java.util.List.class);
}
/**
* Do not allow further writes into the store.
*/
@Override
public void lock() {
this.jsiiCall("lock", Void.class);
}
/**
* Creates a directory and returns it's full path.
*
* @param directoryName The name of the directory to create.
*/
@Override
public java.lang.String mkdir(final java.lang.String directoryName) {
return this.jsiiCall("mkdir", java.lang.String.class, new Object[] { java.util.Objects.requireNonNull(directoryName, "directoryName is required") });
}
/**
* Returns the list of files in a directory.
*
* @param directoryName The name of the artifact.
*/
@Override
public java.util.List readdir(final java.lang.String directoryName) {
return this.jsiiCall("readdir", java.util.List.class, new Object[] { java.util.Objects.requireNonNull(directoryName, "directoryName is required") });
}
/**
* Reads a file from the store.
*
* @param fileName The name of the file.
*/
@Override
@javax.annotation.Nullable
public java.lang.Object readFile(final java.lang.String fileName) {
return this.jsiiCall("readFile", java.lang.Object.class, new Object[] { java.util.Objects.requireNonNull(fileName, "fileName is required") });
}
/**
* Reads a JSON object from the store.
*/
@Override
@javax.annotation.Nullable
public java.lang.Object readJson(final java.lang.String fileName) {
return this.jsiiCall("readJson", java.lang.Object.class, new Object[] { java.util.Objects.requireNonNull(fileName, "fileName is required") });
}
/**
* Writes a file into the store.
*
* @param artifactName The name of the file.
* @param data The contents of the file.
*/
@Override
public void writeFile(final java.lang.String artifactName, @javax.annotation.Nullable final java.lang.Object data) {
this.jsiiCall("writeFile", Void.class, new Object[] { java.util.Objects.requireNonNull(artifactName, "artifactName is required"), data });
}
/**
* Writes a formatted JSON output file to the store.
*
* @param artifactName the name of the artifact.
* @param json the JSON object.
*/
@Override
public void writeJson(final java.lang.String artifactName, @javax.annotation.Nullable final java.lang.Object json) {
this.jsiiCall("writeJson", Void.class, new Object[] { java.util.Objects.requireNonNull(artifactName, "artifactName is required"), json });
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy