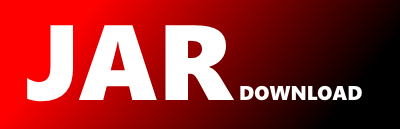
software.amazon.awscdk.MetadataEntry Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cdk Show documentation
Show all versions of cdk Show documentation
AWS Cloud Development Kit Core Library
package software.amazon.awscdk;
/**
* An metadata entry in the construct.
*/
@javax.annotation.Generated(value = "jsii-pacmak/0.10.5 (build 46bc9b0)", date = "2019-05-06T20:49:40.955Z")
public interface MetadataEntry extends software.amazon.jsii.JsiiSerializable {
/**
* A stack trace for when the entry was created.
*/
java.util.List getTrace();
/**
* The type of the metadata entry.
*/
java.lang.String getType();
/**
* The data.
*/
java.lang.Object getData();
/**
* @return a {@link Builder} of {@link MetadataEntry}
*/
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link MetadataEntry}
*/
final class Builder {
private java.util.List _trace;
private java.lang.String _type;
@javax.annotation.Nullable
private java.lang.Object _data;
/**
* Sets the value of Trace
* @param value A stack trace for when the entry was created.
* @return {@code this}
*/
public Builder withTrace(final java.util.List value) {
this._trace = java.util.Objects.requireNonNull(value, "trace is required");
return this;
}
/**
* Sets the value of Type
* @param value The type of the metadata entry.
* @return {@code this}
*/
public Builder withType(final java.lang.String value) {
this._type = java.util.Objects.requireNonNull(value, "type is required");
return this;
}
/**
* Sets the value of Data
* @param value The data.
* @return {@code this}
*/
public Builder withData(@javax.annotation.Nullable final java.lang.Object value) {
this._data = value;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link MetadataEntry}
* @throws NullPointerException if any required attribute was not provided
*/
public MetadataEntry build() {
return new MetadataEntry() {
private final java.util.List $trace = java.util.Objects.requireNonNull(_trace, "trace is required");
private final java.lang.String $type = java.util.Objects.requireNonNull(_type, "type is required");
@javax.annotation.Nullable
private final java.lang.Object $data = _data;
@Override
public java.util.List getTrace() {
return this.$trace;
}
@Override
public java.lang.String getType() {
return this.$type;
}
@Override
public java.lang.Object getData() {
return this.$data;
}
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("trace", om.valueToTree(this.getTrace()));
obj.set("type", om.valueToTree(this.getType()));
obj.set("data", om.valueToTree(this.getData()));
return obj;
}
};
}
}
/**
* A proxy class which represents a concrete javascript instance of this type.
*/
final static class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements software.amazon.awscdk.MetadataEntry {
protected Jsii$Proxy(final software.amazon.jsii.JsiiObject.InitializationMode mode) {
super(mode);
}
/**
* A stack trace for when the entry was created.
*/
@Override
public java.util.List getTrace() {
return this.jsiiGet("trace", java.util.List.class);
}
/**
* The type of the metadata entry.
*/
@Override
public java.lang.String getType() {
return this.jsiiGet("type", java.lang.String.class);
}
/**
* The data.
*/
@Override
@javax.annotation.Nullable
public java.lang.Object getData() {
return this.jsiiGet("data", java.lang.Object.class);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy