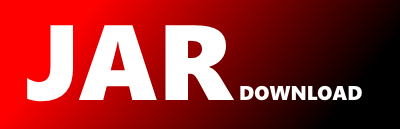
software.amazon.awscdk.SecretsManagerSecretOptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cdk Show documentation
Show all versions of cdk Show documentation
AWS Cloud Development Kit Core Library
package software.amazon.awscdk;
/**
* Options for referencing a secret value from Secrets Manager.
*/
@javax.annotation.Generated(value = "jsii-pacmak/0.10.5 (build 46bc9b0)", date = "2019-05-06T20:49:40.957Z")
public interface SecretsManagerSecretOptions extends software.amazon.jsii.JsiiSerializable {
/**
* The key of a JSON field to retrieve.
*
* This can only be used if the secret
* stores a JSON object.
*
* Default: - returns all the content stored in the Secrets Manager secret.
*/
java.lang.String getJsonField();
/**
* Specifies the unique identifier of the version of the secret you want to use.
*
* Can specify at most one of `versionId` and `versionStage`.
*
* Default: AWSCURRENT
*/
java.lang.String getVersionId();
/**
* Specified the secret version that you want to retrieve by the staging label attached to the version.
*
* Can specify at most one of `versionId` and `versionStage`.
*
* Default: AWSCURRENT
*/
java.lang.String getVersionStage();
/**
* @return a {@link Builder} of {@link SecretsManagerSecretOptions}
*/
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link SecretsManagerSecretOptions}
*/
final class Builder {
@javax.annotation.Nullable
private java.lang.String _jsonField;
@javax.annotation.Nullable
private java.lang.String _versionId;
@javax.annotation.Nullable
private java.lang.String _versionStage;
/**
* Sets the value of JsonField
* @param value The key of a JSON field to retrieve.
* @return {@code this}
*/
public Builder withJsonField(@javax.annotation.Nullable final java.lang.String value) {
this._jsonField = value;
return this;
}
/**
* Sets the value of VersionId
* @param value Specifies the unique identifier of the version of the secret you want to use.
* @return {@code this}
*/
public Builder withVersionId(@javax.annotation.Nullable final java.lang.String value) {
this._versionId = value;
return this;
}
/**
* Sets the value of VersionStage
* @param value Specified the secret version that you want to retrieve by the staging label attached to the version.
* @return {@code this}
*/
public Builder withVersionStage(@javax.annotation.Nullable final java.lang.String value) {
this._versionStage = value;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link SecretsManagerSecretOptions}
* @throws NullPointerException if any required attribute was not provided
*/
public SecretsManagerSecretOptions build() {
return new SecretsManagerSecretOptions() {
@javax.annotation.Nullable
private final java.lang.String $jsonField = _jsonField;
@javax.annotation.Nullable
private final java.lang.String $versionId = _versionId;
@javax.annotation.Nullable
private final java.lang.String $versionStage = _versionStage;
@Override
public java.lang.String getJsonField() {
return this.$jsonField;
}
@Override
public java.lang.String getVersionId() {
return this.$versionId;
}
@Override
public java.lang.String getVersionStage() {
return this.$versionStage;
}
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("jsonField", om.valueToTree(this.getJsonField()));
obj.set("versionId", om.valueToTree(this.getVersionId()));
obj.set("versionStage", om.valueToTree(this.getVersionStage()));
return obj;
}
};
}
}
/**
* A proxy class which represents a concrete javascript instance of this type.
*/
final static class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements software.amazon.awscdk.SecretsManagerSecretOptions {
protected Jsii$Proxy(final software.amazon.jsii.JsiiObject.InitializationMode mode) {
super(mode);
}
/**
* The key of a JSON field to retrieve.
*
* This can only be used if the secret
* stores a JSON object.
*
* Default: - returns all the content stored in the Secrets Manager secret.
*/
@Override
@javax.annotation.Nullable
public java.lang.String getJsonField() {
return this.jsiiGet("jsonField", java.lang.String.class);
}
/**
* Specifies the unique identifier of the version of the secret you want to use.
*
* Can specify at most one of `versionId` and `versionStage`.
*
* Default: AWSCURRENT
*/
@Override
@javax.annotation.Nullable
public java.lang.String getVersionId() {
return this.jsiiGet("versionId", java.lang.String.class);
}
/**
* Specified the secret version that you want to retrieve by the staging label attached to the version.
*
* Can specify at most one of `versionId` and `versionStage`.
*
* Default: AWSCURRENT
*/
@Override
@javax.annotation.Nullable
public java.lang.String getVersionStage() {
return this.jsiiGet("versionStage", java.lang.String.class);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy