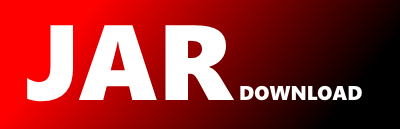
software.amazon.awscdk.Stack Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cdk Show documentation
Show all versions of cdk Show documentation
AWS Cloud Development Kit Core Library
package software.amazon.awscdk;
/**
* A root construct which represents a single CloudFormation stack.
*/
@javax.annotation.Generated(value = "jsii-pacmak/0.10.5 (build 46bc9b0)", date = "2019-05-06T20:49:40.958Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.$Module.class, fqn = "@aws-cdk/cdk.Stack")
public class Stack extends software.amazon.awscdk.Construct {
protected Stack(final software.amazon.jsii.JsiiObject.InitializationMode mode) {
super(mode);
}
/**
* Creates a new stack.
*
* @param scope Parent of this stack, usually a Program instance.
* @param name The name of the CloudFormation stack.
* @param props Stack properties.
*/
public Stack(@javax.annotation.Nullable final software.amazon.awscdk.Construct scope, @javax.annotation.Nullable final java.lang.String name, @javax.annotation.Nullable final software.amazon.awscdk.StackProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.Jsii);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { scope, name, props });
}
/**
* Creates a new stack.
*
* @param scope Parent of this stack, usually a Program instance.
* @param name The name of the CloudFormation stack.
*/
public Stack(@javax.annotation.Nullable final software.amazon.awscdk.Construct scope, @javax.annotation.Nullable final java.lang.String name) {
super(software.amazon.jsii.JsiiObject.InitializationMode.Jsii);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { scope, name });
}
/**
* Creates a new stack.
*
* @param scope Parent of this stack, usually a Program instance.
*/
public Stack(@javax.annotation.Nullable final software.amazon.awscdk.Construct scope) {
super(software.amazon.jsii.JsiiObject.InitializationMode.Jsii);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { scope });
}
/**
* Creates a new stack.
*/
public Stack() {
super(software.amazon.jsii.JsiiObject.InitializationMode.Jsii);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this);
}
/**
* Adds a metadata annotation "aws:cdk:physical-name" to the construct if physicalName is non-null.
*
* This can be used later by tools and aspects to determine if resources
* have been created with physical names.
*/
public static void annotatePhysicalName(final software.amazon.awscdk.Construct construct, @javax.annotation.Nullable final java.lang.String physicalName) {
software.amazon.jsii.JsiiObject.jsiiStaticCall(software.amazon.awscdk.Stack.class, "annotatePhysicalName", Void.class, new Object[] { java.util.Objects.requireNonNull(construct, "construct is required"), physicalName });
}
/**
* Adds a metadata annotation "aws:cdk:physical-name" to the construct if physicalName is non-null.
*
* This can be used later by tools and aspects to determine if resources
* have been created with physical names.
*/
public static void annotatePhysicalName(final software.amazon.awscdk.Construct construct) {
software.amazon.jsii.JsiiObject.jsiiStaticCall(software.amazon.awscdk.Stack.class, "annotatePhysicalName", Void.class, new Object[] { java.util.Objects.requireNonNull(construct, "construct is required") });
}
/**
* Return whether the given object is a Stack.
*
* We do attribute detection since we can't reliably use 'instanceof'.
*/
public static java.lang.Boolean isStack(@javax.annotation.Nullable final java.lang.Object obj) {
return software.amazon.jsii.JsiiObject.jsiiStaticCall(software.amazon.awscdk.Stack.class, "isStack", java.lang.Boolean.class, new Object[] { obj });
}
/**
* Add a dependency between this stack and another stack.
*/
public void addDependency(final software.amazon.awscdk.Stack stack, @javax.annotation.Nullable final java.lang.String reason) {
this.jsiiCall("addDependency", Void.class, new Object[] { java.util.Objects.requireNonNull(stack, "stack is required"), reason });
}
/**
* Add a dependency between this stack and another stack.
*/
public void addDependency(final software.amazon.awscdk.Stack stack) {
this.jsiiCall("addDependency", Void.class, new Object[] { java.util.Objects.requireNonNull(stack, "stack is required") });
}
/**
* Return the stacks this stack depends on.
*/
public java.util.List dependencies() {
return this.jsiiCall("dependencies", java.util.List.class);
}
/**
* Looks up a resource by path.
*
* @return The Resource or undefined if not found
*/
@javax.annotation.Nullable
public software.amazon.awscdk.CfnResource findResource(final java.lang.String path) {
return this.jsiiCall("findResource", software.amazon.awscdk.CfnResource.class, new Object[] { java.util.Objects.requireNonNull(path, "path is required") });
}
/**
* Creates an ARN from components.
*
* If `partition`, `region` or `account` are not specified, the stack's
* partition, region and account will be used.
*
* If any component is the empty string, an empty string will be inserted
* into the generated ARN at the location that component corresponds to.
*
* The ARN will be formatted as follows:
*
* arn:{partition}:{service}:{region}:{account}:{resource}{sep}}{resource-name}
*
* The required ARN pieces that are omitted will be taken from the stack that
* the 'scope' is attached to. If all ARN pieces are supplied, the supplied scope
* can be 'undefined'.
*/
public java.lang.String formatArn(final software.amazon.awscdk.ArnComponents components) {
return this.jsiiCall("formatArn", java.lang.String.class, new Object[] { java.util.Objects.requireNonNull(components, "components is required") });
}
@javax.annotation.Nullable
public software.amazon.awscdk.App parentApp() {
return this.jsiiCall("parentApp", software.amazon.awscdk.App.class);
}
/**
* Given an ARN, parses it and returns components.
*
* If the ARN is a concrete string, it will be parsed and validated. The
* separator (`sep`) will be set to '/' if the 6th component includes a '/',
* in which case, `resource` will be set to the value before the '/' and
* `resourceName` will be the rest. In case there is no '/', `resource` will
* be set to the 6th components and `resourceName` will be set to the rest
* of the string.
*
* If the ARN includes tokens (or is a token), the ARN cannot be validated,
* since we don't have the actual value yet at the time of this function
* call. You will have to know the separator and the type of ARN. The
* resulting `ArnComponents` object will contain tokens for the
* subexpressions of the ARN, not string literals. In this case this
* function cannot properly parse the complete final resourceName (path) out
* of ARNs that use '/' to both separate the 'resource' from the
* 'resourceName' AND to subdivide the resourceName further. For example, in
* S3 ARNs:
*
* arn:aws:s3:::my_corporate_bucket/path/to/exampleobject.png
*
* After parsing the resourceName will not contain
* 'path/to/exampleobject.png' but simply 'path'. This is a limitation
* because there is no slicing functionality in CloudFormation templates.
*
* @return an ArnComponents object which allows access to the various
* components of the ARN.
* @param arn The ARN string to parse.
* @param sepIfToken The separator used to separate resource from resourceName.
* @param hasName Whether there is a name component in the ARN at all.
*/
public software.amazon.awscdk.ArnComponents parseArn(final java.lang.String arn, @javax.annotation.Nullable final java.lang.String sepIfToken, @javax.annotation.Nullable final java.lang.Boolean hasName) {
return this.jsiiCall("parseArn", software.amazon.awscdk.ArnComponents.class, new Object[] { java.util.Objects.requireNonNull(arn, "arn is required"), sepIfToken, hasName });
}
/**
* Given an ARN, parses it and returns components.
*
* If the ARN is a concrete string, it will be parsed and validated. The
* separator (`sep`) will be set to '/' if the 6th component includes a '/',
* in which case, `resource` will be set to the value before the '/' and
* `resourceName` will be the rest. In case there is no '/', `resource` will
* be set to the 6th components and `resourceName` will be set to the rest
* of the string.
*
* If the ARN includes tokens (or is a token), the ARN cannot be validated,
* since we don't have the actual value yet at the time of this function
* call. You will have to know the separator and the type of ARN. The
* resulting `ArnComponents` object will contain tokens for the
* subexpressions of the ARN, not string literals. In this case this
* function cannot properly parse the complete final resourceName (path) out
* of ARNs that use '/' to both separate the 'resource' from the
* 'resourceName' AND to subdivide the resourceName further. For example, in
* S3 ARNs:
*
* arn:aws:s3:::my_corporate_bucket/path/to/exampleobject.png
*
* After parsing the resourceName will not contain
* 'path/to/exampleobject.png' but simply 'path'. This is a limitation
* because there is no slicing functionality in CloudFormation templates.
*
* @return an ArnComponents object which allows access to the various
* components of the ARN.
* @param arn The ARN string to parse.
* @param sepIfToken The separator used to separate resource from resourceName.
*/
public software.amazon.awscdk.ArnComponents parseArn(final java.lang.String arn, @javax.annotation.Nullable final java.lang.String sepIfToken) {
return this.jsiiCall("parseArn", software.amazon.awscdk.ArnComponents.class, new Object[] { java.util.Objects.requireNonNull(arn, "arn is required"), sepIfToken });
}
/**
* Given an ARN, parses it and returns components.
*
* If the ARN is a concrete string, it will be parsed and validated. The
* separator (`sep`) will be set to '/' if the 6th component includes a '/',
* in which case, `resource` will be set to the value before the '/' and
* `resourceName` will be the rest. In case there is no '/', `resource` will
* be set to the 6th components and `resourceName` will be set to the rest
* of the string.
*
* If the ARN includes tokens (or is a token), the ARN cannot be validated,
* since we don't have the actual value yet at the time of this function
* call. You will have to know the separator and the type of ARN. The
* resulting `ArnComponents` object will contain tokens for the
* subexpressions of the ARN, not string literals. In this case this
* function cannot properly parse the complete final resourceName (path) out
* of ARNs that use '/' to both separate the 'resource' from the
* 'resourceName' AND to subdivide the resourceName further. For example, in
* S3 ARNs:
*
* arn:aws:s3:::my_corporate_bucket/path/to/exampleobject.png
*
* After parsing the resourceName will not contain
* 'path/to/exampleobject.png' but simply 'path'. This is a limitation
* because there is no slicing functionality in CloudFormation templates.
*
* @return an ArnComponents object which allows access to the various
* components of the ARN.
* @param arn The ARN string to parse.
*/
public software.amazon.awscdk.ArnComponents parseArn(final java.lang.String arn) {
return this.jsiiCall("parseArn", software.amazon.awscdk.ArnComponents.class, new Object[] { java.util.Objects.requireNonNull(arn, "arn is required") });
}
/**
* Prepare stack.
*
* Find all CloudFormation references and tell them we're consuming them.
*
* Find all dependencies as well and add the appropriate DependsOn fields.
*/
@Override
protected void prepare() {
this.jsiiCall("prepare", Void.class);
}
/**
* Rename a generated logical identities.
*/
public void renameLogical(final java.lang.String oldId, final java.lang.String newId) {
this.jsiiCall("renameLogical", Void.class, new Object[] { java.util.Objects.requireNonNull(oldId, "oldId is required"), java.util.Objects.requireNonNull(newId, "newId is required") });
}
/**
* Indicate that a context key was expected.
*
* Contains instructions on how the key should be supplied.
*
* @param key Key that uniquely identifies this missing context.
* @param details The set of parameters needed to obtain the context (specific to context provider).
*/
public void reportMissingContext(final java.lang.String key, final software.amazon.awscdk.cxapi.MissingContext details) {
this.jsiiCall("reportMissingContext", Void.class, new Object[] { java.util.Objects.requireNonNull(key, "key is required"), java.util.Objects.requireNonNull(details, "details is required") });
}
/**
* Returns the AWS account ID of this Stack, or throws an exception if the account ID is not set in the environment.
*
* @return the AWS account ID of this Stack
* @param why more information about why is the account ID required.
*/
public java.lang.String requireAccountId(@javax.annotation.Nullable final java.lang.String why) {
return this.jsiiCall("requireAccountId", java.lang.String.class, new Object[] { why });
}
/**
* Returns the AWS account ID of this Stack, or throws an exception if the account ID is not set in the environment.
*
* @return the AWS account ID of this Stack
*/
public java.lang.String requireAccountId() {
return this.jsiiCall("requireAccountId", java.lang.String.class);
}
/**
* @return The region in which this stack is deployed. Throws if region is not defined.
* @param why more information about why region is required.
*/
public java.lang.String requireRegion(@javax.annotation.Nullable final java.lang.String why) {
return this.jsiiCall("requireRegion", java.lang.String.class, new Object[] { why });
}
/**
* @return The region in which this stack is deployed. Throws if region is not defined.
*/
public java.lang.String requireRegion() {
return this.jsiiCall("requireRegion", java.lang.String.class);
}
/**
* Sets the value of a CloudFormation parameter.
*
* @param parameter The parameter to set the value for.
* @param value The value, can use `${}` notation to reference other assembly block attributes.
*/
public void setParameterValue(final software.amazon.awscdk.CfnParameter parameter, final java.lang.String value) {
this.jsiiCall("setParameterValue", Void.class, new Object[] { java.util.Objects.requireNonNull(parameter, "parameter is required"), java.util.Objects.requireNonNull(value, "value is required") });
}
protected void synthesize(final software.amazon.awscdk.ISynthesisSession session) {
this.jsiiCall("synthesize", Void.class, new Object[] { java.util.Objects.requireNonNull(session, "session is required") });
}
/**
* The account in which this stack is defined.
*
* Either returns the literal account for this stack if it was specified
* literally upon Stack construction, or a symbolic value that will evaluate
* to the correct account at deployment time.
*/
public java.lang.String getAccountId() {
return this.jsiiGet("accountId", java.lang.String.class);
}
/**
* Should the Stack be deployed when running `cdk deploy` without arguments (and listed when running `cdk synth` without arguments). Setting this to `false` is useful when you have a Stack in your CDK app that you don't want to deploy using the CDK toolkit - for example, because you're planning on deploying it through CodePipeline.
*
* By default, this is `true`.
*/
public java.lang.Boolean getAutoDeploy() {
return this.jsiiGet("autoDeploy", java.lang.Boolean.class);
}
/**
* The environment in which this stack is deployed.
*/
public software.amazon.awscdk.Environment getEnv() {
return this.jsiiGet("env", software.amazon.awscdk.Environment.class);
}
/**
* Returns the environment specification for this stack (aws://account/region).
*/
public java.lang.String getEnvironment() {
return this.jsiiGet("environment", java.lang.String.class);
}
/**
* Logical ID generation strategy.
*/
public software.amazon.awscdk.LogicalIDs getLogicalIds() {
return this.jsiiGet("logicalIds", software.amazon.awscdk.LogicalIDs.class);
}
/**
* Lists all missing contextual information. This is returned when the stack is synthesized under the 'missing' attribute and allows tooling to obtain the context and re-synthesize.
*/
public java.util.Map getMissingContext() {
return this.jsiiGet("missingContext", java.util.Map.class);
}
/**
* The CloudFormation stack name.
*
* This is the stack name either configuration via the `stackName` property
* or automatically derived from the construct path.
*/
public java.lang.String getName() {
return this.jsiiGet("name", java.lang.String.class);
}
/**
* Returns the list of notification Amazon Resource Names (ARNs) for the current stack.
*/
public java.util.List getNotificationArns() {
return this.jsiiGet("notificationArns", java.util.List.class);
}
/**
* The partition in which this stack is defined.
*/
public java.lang.String getPartition() {
return this.jsiiGet("partition", java.lang.String.class);
}
/**
* The region in which this stack is defined.
*
* Either returns the literal region for this stack if it was specified
* literally upon Stack construction, or a symbolic value that will evaluate
* to the correct region at deployment time.
*/
public java.lang.String getRegion() {
return this.jsiiGet("region", java.lang.String.class);
}
/**
* The ID of the stack.
*
* Example:
*
* After resolving, looks like arn:aws:cloudformation:us-west-2:123456789012:stack/teststack/51af3dc0-da77-11e4-872e-1234567db123
*/
public java.lang.String getStackId() {
return this.jsiiGet("stackId", java.lang.String.class);
}
/**
* The name of the stack currently being deployed.
*
* Only available at deployment time; this will always return an unresolved value.
*/
public java.lang.String getStackName() {
return this.jsiiGet("stackName", java.lang.String.class);
}
/**
* Options for CloudFormation template (like version, transform, description).
*/
public software.amazon.awscdk.ITemplateOptions getTemplateOptions() {
return this.jsiiGet("templateOptions", software.amazon.awscdk.ITemplateOptions.class);
}
/**
* The Amazon domain suffix for the region in which this stack is defined.
*/
public java.lang.String getUrlSuffix() {
return this.jsiiGet("urlSuffix", java.lang.String.class);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy