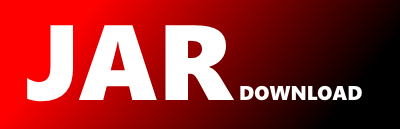
software.amazon.awscdk.StackProps Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cdk Show documentation
Show all versions of cdk Show documentation
AWS Cloud Development Kit Core Library
package software.amazon.awscdk;
@javax.annotation.Generated(value = "jsii-pacmak/0.10.5 (build 46bc9b0)", date = "2019-05-06T20:49:40.959Z")
public interface StackProps extends software.amazon.jsii.JsiiSerializable {
/**
* Should the Stack be deployed when running `cdk deploy` without arguments (and listed when running `cdk synth` without arguments). Setting this to `false` is useful when you have a Stack in your CDK app that you don't want to deploy using the CDK toolkit - for example, because you're planning on deploying it through CodePipeline.
*
* Default: true
*/
java.lang.Boolean getAutoDeploy();
/**
* The AWS environment (account/region) where this stack will be deployed.
*
* If not supplied, the `default-account` and `default-region` context parameters will be
* used. If they are undefined, it will not be possible to deploy the stack.
*/
software.amazon.awscdk.Environment getEnv();
/**
* Strategy for logical ID generation.
*
* Optional. If not supplied, the HashedNamingScheme will be used.
*/
software.amazon.awscdk.IAddressingScheme getNamingScheme();
/**
* Name to deploy the stack with.
*
* Default: Derived from construct path
*/
java.lang.String getStackName();
/**
* @return a {@link Builder} of {@link StackProps}
*/
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link StackProps}
*/
final class Builder {
@javax.annotation.Nullable
private java.lang.Boolean _autoDeploy;
@javax.annotation.Nullable
private software.amazon.awscdk.Environment _env;
@javax.annotation.Nullable
private software.amazon.awscdk.IAddressingScheme _namingScheme;
@javax.annotation.Nullable
private java.lang.String _stackName;
/**
* Sets the value of AutoDeploy
* @param value Should the Stack be deployed when running `cdk deploy` without arguments (and listed when running `cdk synth` without arguments). Setting this to `false` is useful when you have a Stack in your CDK app that you don't want to deploy using the CDK toolkit - for example, because you're planning on deploying it through CodePipeline.
* @return {@code this}
*/
public Builder withAutoDeploy(@javax.annotation.Nullable final java.lang.Boolean value) {
this._autoDeploy = value;
return this;
}
/**
* Sets the value of Env
* @param value The AWS environment (account/region) where this stack will be deployed.
* @return {@code this}
*/
public Builder withEnv(@javax.annotation.Nullable final software.amazon.awscdk.Environment value) {
this._env = value;
return this;
}
/**
* Sets the value of NamingScheme
* @param value Strategy for logical ID generation.
* @return {@code this}
*/
public Builder withNamingScheme(@javax.annotation.Nullable final software.amazon.awscdk.IAddressingScheme value) {
this._namingScheme = value;
return this;
}
/**
* Sets the value of StackName
* @param value Name to deploy the stack with.
* @return {@code this}
*/
public Builder withStackName(@javax.annotation.Nullable final java.lang.String value) {
this._stackName = value;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link StackProps}
* @throws NullPointerException if any required attribute was not provided
*/
public StackProps build() {
return new StackProps() {
@javax.annotation.Nullable
private final java.lang.Boolean $autoDeploy = _autoDeploy;
@javax.annotation.Nullable
private final software.amazon.awscdk.Environment $env = _env;
@javax.annotation.Nullable
private final software.amazon.awscdk.IAddressingScheme $namingScheme = _namingScheme;
@javax.annotation.Nullable
private final java.lang.String $stackName = _stackName;
@Override
public java.lang.Boolean getAutoDeploy() {
return this.$autoDeploy;
}
@Override
public software.amazon.awscdk.Environment getEnv() {
return this.$env;
}
@Override
public software.amazon.awscdk.IAddressingScheme getNamingScheme() {
return this.$namingScheme;
}
@Override
public java.lang.String getStackName() {
return this.$stackName;
}
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("autoDeploy", om.valueToTree(this.getAutoDeploy()));
obj.set("env", om.valueToTree(this.getEnv()));
obj.set("namingScheme", om.valueToTree(this.getNamingScheme()));
obj.set("stackName", om.valueToTree(this.getStackName()));
return obj;
}
};
}
}
/**
* A proxy class which represents a concrete javascript instance of this type.
*/
final static class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements software.amazon.awscdk.StackProps {
protected Jsii$Proxy(final software.amazon.jsii.JsiiObject.InitializationMode mode) {
super(mode);
}
/**
* Should the Stack be deployed when running `cdk deploy` without arguments (and listed when running `cdk synth` without arguments). Setting this to `false` is useful when you have a Stack in your CDK app that you don't want to deploy using the CDK toolkit - for example, because you're planning on deploying it through CodePipeline.
*
* Default: true
*/
@Override
@javax.annotation.Nullable
public java.lang.Boolean getAutoDeploy() {
return this.jsiiGet("autoDeploy", java.lang.Boolean.class);
}
/**
* The AWS environment (account/region) where this stack will be deployed.
*
* If not supplied, the `default-account` and `default-region` context parameters will be
* used. If they are undefined, it will not be possible to deploy the stack.
*/
@Override
@javax.annotation.Nullable
public software.amazon.awscdk.Environment getEnv() {
return this.jsiiGet("env", software.amazon.awscdk.Environment.class);
}
/**
* Strategy for logical ID generation.
*
* Optional. If not supplied, the HashedNamingScheme will be used.
*/
@Override
@javax.annotation.Nullable
public software.amazon.awscdk.IAddressingScheme getNamingScheme() {
return this.jsiiGet("namingScheme", software.amazon.awscdk.IAddressingScheme.class);
}
/**
* Name to deploy the stack with.
*
* Default: Derived from construct path
*/
@Override
@javax.annotation.Nullable
public java.lang.String getStackName() {
return this.jsiiGet("stackName", java.lang.String.class);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy