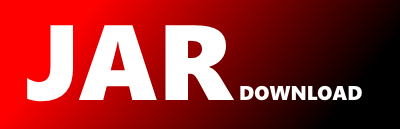
software.amazon.awscdk.Token Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cdk Show documentation
Show all versions of cdk Show documentation
AWS Cloud Development Kit Core Library
package software.amazon.awscdk;
/**
* Represents a special or lazily-evaluated value.
*
* Can be used to delay evaluation of a certain value in case, for example,
* that it requires some context or late-bound data. Can also be used to
* mark values that need special processing at document rendering time.
*
* Tokens can be embedded into strings while retaining their original
* semantics.
*/
@javax.annotation.Generated(value = "jsii-pacmak/0.10.5 (build 46bc9b0)", date = "2019-05-06T20:49:40.962Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.$Module.class, fqn = "@aws-cdk/cdk.Token")
public class Token extends software.amazon.jsii.JsiiObject {
protected Token(final software.amazon.jsii.JsiiObject.InitializationMode mode) {
super(mode);
}
/**
* Creates a token that resolves to `value`.
*
* If value is a function, the function is evaluated upon resolution and
* the value it returns will be used as the token's value.
*
* displayName is used to represent the Token when it's embedded into a string; it
* will look something like this:
*
* "embedded in a larger string is ${Token[DISPLAY_NAME.123]}"
*
* This value is used as a hint to humans what the meaning of the Token is,
* and does not have any effect on the evaluation.
*
* Must contain only alphanumeric and simple separator characters (_.:-).
*
* @param valueOrFunction What this token will evaluate to, literal or function.
* @param displayName A human-readable display hint for this Token.
*/
public Token(@javax.annotation.Nullable final java.lang.Object valueOrFunction, @javax.annotation.Nullable final java.lang.String displayName) {
super(software.amazon.jsii.JsiiObject.InitializationMode.Jsii);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { valueOrFunction, displayName });
}
/**
* Creates a token that resolves to `value`.
*
* If value is a function, the function is evaluated upon resolution and
* the value it returns will be used as the token's value.
*
* displayName is used to represent the Token when it's embedded into a string; it
* will look something like this:
*
* "embedded in a larger string is ${Token[DISPLAY_NAME.123]}"
*
* This value is used as a hint to humans what the meaning of the Token is,
* and does not have any effect on the evaluation.
*
* Must contain only alphanumeric and simple separator characters (_.:-).
*
* @param valueOrFunction What this token will evaluate to, literal or function.
*/
public Token(@javax.annotation.Nullable final java.lang.Object valueOrFunction) {
super(software.amazon.jsii.JsiiObject.InitializationMode.Jsii);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { valueOrFunction });
}
/**
* Creates a token that resolves to `value`.
*
* If value is a function, the function is evaluated upon resolution and
* the value it returns will be used as the token's value.
*
* displayName is used to represent the Token when it's embedded into a string; it
* will look something like this:
*
* "embedded in a larger string is ${Token[DISPLAY_NAME.123]}"
*
* This value is used as a hint to humans what the meaning of the Token is,
* and does not have any effect on the evaluation.
*
* Must contain only alphanumeric and simple separator characters (_.:-).
*/
public Token() {
super(software.amazon.jsii.JsiiObject.InitializationMode.Jsii);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this);
}
/**
* Returns true if obj is a token (i.e. has the resolve() method or is a string or array which includes token markers).
*
* @param obj The object to test.
*/
public static java.lang.Boolean isToken(@javax.annotation.Nullable final java.lang.Object obj) {
return software.amazon.jsii.JsiiObject.jsiiStaticCall(software.amazon.awscdk.Token.class, "isToken", java.lang.Boolean.class, new Object[] { obj });
}
/**
* @deprecated use `Token.isToken`
*/
public static java.lang.Boolean unresolved(@javax.annotation.Nullable final java.lang.Object obj) {
return software.amazon.jsii.JsiiObject.jsiiStaticCall(software.amazon.awscdk.Token.class, "unresolved", java.lang.Boolean.class, new Object[] { obj });
}
/**
* @return The resolved value for this token.
*/
@javax.annotation.Nullable
public java.lang.Object resolve(final software.amazon.awscdk.ResolveContext _context) {
return this.jsiiCall("resolve", java.lang.Object.class, new Object[] { java.util.Objects.requireNonNull(_context, "_context is required") });
}
/**
* Turn this Token into JSON.
*
* This gets called by JSON.stringify(). We want to prohibit this, because
* it's not possible to do this properly, so we just throw an error here.
*/
@javax.annotation.Nullable
public java.lang.Object toJSON() {
return this.jsiiCall("toJSON", java.lang.Object.class);
}
/**
* Return a string list representation of this token.
*
* Call this if the Token intrinsically evaluates to a list of strings.
* If so, you can represent the Token in a similar way in the type
* system.
*
* Note that even though the Token is represented as a list of strings, you
* still cannot do any operations on it such as concatenation, indexing,
* or taking its length. The only useful operations you can do to these lists
* is constructing a `FnJoin` or a `FnSelect` on it.
*/
public java.util.List toList() {
return this.jsiiCall("toList", java.util.List.class);
}
/**
* Return a reversible string representation of this token.
*
* If the Token is initialized with a literal, the stringified value of the
* literal is returned. Otherwise, a special quoted string representation
* of the Token is returned that can be embedded into other strings.
*
* Strings with quoted Tokens in them can be restored back into
* complex values with the Tokens restored by calling `resolve()`
* on the string.
*/
public java.lang.String toString() {
return this.jsiiCall("toString", java.lang.String.class);
}
/**
* A human-readable display hint for this Token.
*/
@javax.annotation.Nullable
public java.lang.String getDisplayName() {
return this.jsiiGet("displayName", java.lang.String.class);
}
/**
* What this token will evaluate to, literal or function.
*/
@javax.annotation.Nullable
public java.lang.Object getValueOrFunction() {
return this.jsiiGet("valueOrFunction", java.lang.Object.class);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy