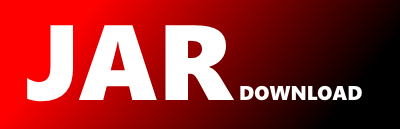
software.amazon.awscdk.services.cloudfront.CachePolicy Maven / Gradle / Ivy
package software.amazon.awscdk.services.cloudfront;
/**
* A Cache Policy configuration.
*
* Example:
*
*
* // Using an existing cache policy for a Distribution
* S3Origin bucketOrigin;
* Distribution.Builder.create(this, "myDistManagedPolicy")
* .defaultBehavior(BehaviorOptions.builder()
* .origin(bucketOrigin)
* .cachePolicy(CachePolicy.CACHING_OPTIMIZED)
* .build())
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.84.0 (build 5404dcf)", date = "2023-06-19T16:30:43.445Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.cloudfront.$Module.class, fqn = "@aws-cdk/aws-cloudfront.CachePolicy")
public class CachePolicy extends software.amazon.awscdk.core.Resource implements software.amazon.awscdk.services.cloudfront.ICachePolicy {
protected CachePolicy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected CachePolicy(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
static {
AMPLIFY = software.amazon.jsii.JsiiObject.jsiiStaticGet(software.amazon.awscdk.services.cloudfront.CachePolicy.class, "AMPLIFY", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.cloudfront.ICachePolicy.class));
CACHING_DISABLED = software.amazon.jsii.JsiiObject.jsiiStaticGet(software.amazon.awscdk.services.cloudfront.CachePolicy.class, "CACHING_DISABLED", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.cloudfront.ICachePolicy.class));
CACHING_OPTIMIZED = software.amazon.jsii.JsiiObject.jsiiStaticGet(software.amazon.awscdk.services.cloudfront.CachePolicy.class, "CACHING_OPTIMIZED", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.cloudfront.ICachePolicy.class));
CACHING_OPTIMIZED_FOR_UNCOMPRESSED_OBJECTS = software.amazon.jsii.JsiiObject.jsiiStaticGet(software.amazon.awscdk.services.cloudfront.CachePolicy.class, "CACHING_OPTIMIZED_FOR_UNCOMPRESSED_OBJECTS", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.cloudfront.ICachePolicy.class));
ELEMENTAL_MEDIA_PACKAGE = software.amazon.jsii.JsiiObject.jsiiStaticGet(software.amazon.awscdk.services.cloudfront.CachePolicy.class, "ELEMENTAL_MEDIA_PACKAGE", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.cloudfront.ICachePolicy.class));
}
/**
* @param scope This parameter is required.
* @param id This parameter is required.
* @param props
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public CachePolicy(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.cloudfront.CachePolicyProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), props });
}
/**
* @param scope This parameter is required.
* @param id This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public CachePolicy(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required") });
}
/**
* Imports a Cache Policy from its id.
*
* @param scope This parameter is required.
* @param id This parameter is required.
* @param cachePolicyId This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.cloudfront.ICachePolicy fromCachePolicyId(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull java.lang.String cachePolicyId) {
return software.amazon.jsii.JsiiObject.jsiiStaticCall(software.amazon.awscdk.services.cloudfront.CachePolicy.class, "fromCachePolicyId", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.cloudfront.ICachePolicy.class), new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(cachePolicyId, "cachePolicyId is required") });
}
/**
* This policy is designed for use with an origin that is an AWS Amplify web app.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public final static software.amazon.awscdk.services.cloudfront.ICachePolicy AMPLIFY;
/**
* Disables caching.
*
* This policy is useful for dynamic content and for requests that are not cacheable.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public final static software.amazon.awscdk.services.cloudfront.ICachePolicy CACHING_DISABLED;
/**
* Optimize cache efficiency by minimizing the values that CloudFront includes in the cache key.
*
* Query strings and cookies are not included in the cache key, and only the normalized 'Accept-Encoding' header is included.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public final static software.amazon.awscdk.services.cloudfront.ICachePolicy CACHING_OPTIMIZED;
/**
* Optimize cache efficiency by minimizing the values that CloudFront includes in the cache key.
*
* Query strings and cookies are not included in the cache key, and only the normalized 'Accept-Encoding' header is included.
* Disables cache compression.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public final static software.amazon.awscdk.services.cloudfront.ICachePolicy CACHING_OPTIMIZED_FOR_UNCOMPRESSED_OBJECTS;
/**
* Designed for use with an origin that is an AWS Elemental MediaPackage endpoint.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public final static software.amazon.awscdk.services.cloudfront.ICachePolicy ELEMENTAL_MEDIA_PACKAGE;
/**
* The ID of the cache policy.
*/
@Override
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String getCachePolicyId() {
return software.amazon.jsii.Kernel.get(this, "cachePolicyId", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* A fluent builder for {@link software.amazon.awscdk.services.cloudfront.CachePolicy}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
* @param scope This parameter is required.
* @param id This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static Builder create(final software.constructs.Construct scope, final java.lang.String id) {
return new Builder(scope, id);
}
private final software.constructs.Construct scope;
private final java.lang.String id;
private software.amazon.awscdk.services.cloudfront.CachePolicyProps.Builder props;
private Builder(final software.constructs.Construct scope, final java.lang.String id) {
this.scope = scope;
this.id = id;
}
/**
* A unique name to identify the cache policy.
*
* The name must only include '-', '_', or alphanumeric characters.
*
* Default: - generated from the `id`
*
* @return {@code this}
* @param cachePolicyName A unique name to identify the cache policy. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder cachePolicyName(final java.lang.String cachePolicyName) {
this.props().cachePolicyName(cachePolicyName);
return this;
}
/**
* A comment to describe the cache policy.
*
* Default: - no comment
*
* @return {@code this}
* @param comment A comment to describe the cache policy. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder comment(final java.lang.String comment) {
this.props().comment(comment);
return this;
}
/**
* Determines whether any cookies in viewer requests are included in the cache key and automatically included in requests that CloudFront sends to the origin.
*
* Default: CacheCookieBehavior.none()
*
* @return {@code this}
* @param cookieBehavior Determines whether any cookies in viewer requests are included in the cache key and automatically included in requests that CloudFront sends to the origin. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder cookieBehavior(final software.amazon.awscdk.services.cloudfront.CacheCookieBehavior cookieBehavior) {
this.props().cookieBehavior(cookieBehavior);
return this;
}
/**
* The default amount of time for objects to stay in the CloudFront cache.
*
* Only used when the origin does not send Cache-Control or Expires headers with the object.
*
* Default: - The greater of 1 day and ``minTtl``
*
* @return {@code this}
* @param defaultTtl The default amount of time for objects to stay in the CloudFront cache. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder defaultTtl(final software.amazon.awscdk.core.Duration defaultTtl) {
this.props().defaultTtl(defaultTtl);
return this;
}
/**
* Whether to normalize and include the Accept-Encoding
header in the cache key when the Accept-Encoding
header is 'br'.
*
* Default: false
*
* @return {@code this}
* @param enableAcceptEncodingBrotli Whether to normalize and include the Accept-Encoding
header in the cache key when the Accept-Encoding
header is 'br'. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder enableAcceptEncodingBrotli(final java.lang.Boolean enableAcceptEncodingBrotli) {
this.props().enableAcceptEncodingBrotli(enableAcceptEncodingBrotli);
return this;
}
/**
* Whether to normalize and include the Accept-Encoding
header in the cache key when the Accept-Encoding
header is 'gzip'.
*
* Default: false
*
* @return {@code this}
* @param enableAcceptEncodingGzip Whether to normalize and include the Accept-Encoding
header in the cache key when the Accept-Encoding
header is 'gzip'. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder enableAcceptEncodingGzip(final java.lang.Boolean enableAcceptEncodingGzip) {
this.props().enableAcceptEncodingGzip(enableAcceptEncodingGzip);
return this;
}
/**
* Determines whether any HTTP headers are included in the cache key and automatically included in requests that CloudFront sends to the origin.
*
* Default: CacheHeaderBehavior.none()
*
* @return {@code this}
* @param headerBehavior Determines whether any HTTP headers are included in the cache key and automatically included in requests that CloudFront sends to the origin. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder headerBehavior(final software.amazon.awscdk.services.cloudfront.CacheHeaderBehavior headerBehavior) {
this.props().headerBehavior(headerBehavior);
return this;
}
/**
* The maximum amount of time for objects to stay in the CloudFront cache.
*
* CloudFront uses this value only when the origin sends Cache-Control or Expires headers with the object.
*
* Default: - The greater of 1 year and ``defaultTtl``
*
* @return {@code this}
* @param maxTtl The maximum amount of time for objects to stay in the CloudFront cache. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder maxTtl(final software.amazon.awscdk.core.Duration maxTtl) {
this.props().maxTtl(maxTtl);
return this;
}
/**
* The minimum amount of time for objects to stay in the CloudFront cache.
*
* Default: Duration.seconds(0)
*
* @return {@code this}
* @param minTtl The minimum amount of time for objects to stay in the CloudFront cache. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder minTtl(final software.amazon.awscdk.core.Duration minTtl) {
this.props().minTtl(minTtl);
return this;
}
/**
* Determines whether any query strings are included in the cache key and automatically included in requests that CloudFront sends to the origin.
*
* Default: CacheQueryStringBehavior.none()
*
* @return {@code this}
* @param queryStringBehavior Determines whether any query strings are included in the cache key and automatically included in requests that CloudFront sends to the origin. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder queryStringBehavior(final software.amazon.awscdk.services.cloudfront.CacheQueryStringBehavior queryStringBehavior) {
this.props().queryStringBehavior(queryStringBehavior);
return this;
}
/**
* @return a newly built instance of {@link software.amazon.awscdk.services.cloudfront.CachePolicy}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public software.amazon.awscdk.services.cloudfront.CachePolicy build() {
return new software.amazon.awscdk.services.cloudfront.CachePolicy(
this.scope,
this.id,
this.props != null ? this.props.build() : null
);
}
private software.amazon.awscdk.services.cloudfront.CachePolicyProps.Builder props() {
if (this.props == null) {
this.props = new software.amazon.awscdk.services.cloudfront.CachePolicyProps.Builder();
}
return this.props;
}
}
}