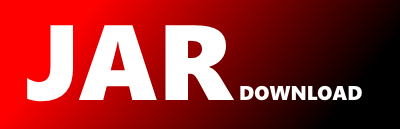
software.amazon.awscdk.services.cloudfront.CloudFrontWebDistributionProps Maven / Gradle / Ivy
Show all versions of cloudfront Show documentation
package software.amazon.awscdk.services.cloudfront;
/**
* Example:
*
*
* Bucket sourceBucket;
* ViewerCertificate viewerCertificate = ViewerCertificate.fromIamCertificate("MYIAMROLEIDENTIFIER", ViewerCertificateOptions.builder()
* .aliases(List.of("MYALIAS"))
* .build());
* CloudFrontWebDistribution.Builder.create(this, "MyCfWebDistribution")
* .originConfigs(List.of(SourceConfiguration.builder()
* .s3OriginSource(S3OriginConfig.builder()
* .s3BucketSource(sourceBucket)
* .build())
* .behaviors(List.of(Behavior.builder().isDefaultBehavior(true).build()))
* .build()))
* .viewerCertificate(viewerCertificate)
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.84.0 (build 5404dcf)", date = "2023-06-19T16:30:43.669Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.cloudfront.$Module.class, fqn = "@aws-cdk/aws-cloudfront.CloudFrontWebDistributionProps")
@software.amazon.jsii.Jsii.Proxy(CloudFrontWebDistributionProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface CloudFrontWebDistributionProps extends software.amazon.jsii.JsiiSerializable {
/**
* The origin configurations for this distribution.
*
* Behaviors are a part of the origin.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.util.List getOriginConfigs();
/**
* (deprecated) AliasConfiguration is used to configured CloudFront to respond to requests on custom domain names.
*
* Default: - None.
*
* @deprecated see {@link CloudFrontWebDistributionProps#viewerCertificate} with {@link ViewerCertificate#acmCertificate}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Deprecated)
@Deprecated
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.cloudfront.AliasConfiguration getAliasConfiguration() {
return null;
}
/**
* A comment for this distribution in the CloudFront console.
*
* Default: - No comment is added to distribution.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getComment() {
return null;
}
/**
* The default object to serve.
*
* Default: - "index.html" is served.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getDefaultRootObject() {
return null;
}
/**
* Enable or disable the distribution.
*
* Default: true
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Boolean getEnabled() {
return null;
}
/**
* If your distribution should have IPv6 enabled.
*
* Default: true
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Boolean getEnableIpV6() {
return null;
}
/**
* How CloudFront should handle requests that are not successful (eg PageNotFound).
*
* By default, CloudFront does not replace HTTP status codes in the 4xx and 5xx range
* with custom error messages. CloudFront does not cache HTTP status codes.
*
* Default: - No custom error configuration.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getErrorConfigurations() {
return null;
}
/**
* Controls the countries in which your content is distributed.
*
* Default: No geo restriction
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.cloudfront.GeoRestriction getGeoRestriction() {
return null;
}
/**
* The max supported HTTP Versions.
*
* Default: HttpVersion.HTTP2
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.cloudfront.HttpVersion getHttpVersion() {
return null;
}
/**
* Optional - if we should enable logging.
*
* You can pass an empty object ({}) to have us auto create a bucket for logging.
* Omission of this property indicates no logging is to be enabled.
*
* Default: - no logging is enabled by default.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.cloudfront.LoggingConfiguration getLoggingConfig() {
return null;
}
/**
* The price class for the distribution (this impacts how many locations CloudFront uses for your distribution, and billing).
*
* Default: PriceClass.PRICE_CLASS_100 the cheapest option for CloudFront is picked by default.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.cloudfront.PriceClass getPriceClass() {
return null;
}
/**
* Specifies whether you want viewers to use HTTP or HTTPS to request your objects, whether you're using an alternate domain name with HTTPS, and if so, if you're using AWS Certificate Manager (ACM) or a third-party certificate authority.
*
* Default: ViewerCertificate.fromCloudFrontDefaultCertificate()
*
* @see https://aws.amazon.com/premiumsupport/knowledge-center/custom-ssl-certificate-cloudfront/
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.cloudfront.ViewerCertificate getViewerCertificate() {
return null;
}
/**
* The default viewer policy for incoming clients.
*
* Default: RedirectToHTTPs
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.cloudfront.ViewerProtocolPolicy getViewerProtocolPolicy() {
return null;
}
/**
* Unique identifier that specifies the AWS WAF web ACL to associate with this CloudFront distribution.
*
* To specify a web ACL created using the latest version of AWS WAF, use the ACL ARN, for example
* arn:aws:wafv2:us-east-1:123456789012:global/webacl/ExampleWebACL/473e64fd-f30b-4765-81a0-62ad96dd167a
.
*
* To specify a web ACL created using AWS WAF Classic, use the ACL ID, for example 473e64fd-f30b-4765-81a0-62ad96dd167a
.
*
* Default: - No AWS Web Application Firewall web access control list (web ACL).
*
* @see https://docs.aws.amazon.com/cloudfront/latest/APIReference/API_CreateDistribution.html#API_CreateDistribution_RequestParameters.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getWebACLId() {
return null;
}
/**
* @return a {@link Builder} of {@link CloudFrontWebDistributionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link CloudFrontWebDistributionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.util.List originConfigs;
software.amazon.awscdk.services.cloudfront.AliasConfiguration aliasConfiguration;
java.lang.String comment;
java.lang.String defaultRootObject;
java.lang.Boolean enabled;
java.lang.Boolean enableIpV6;
java.util.List errorConfigurations;
software.amazon.awscdk.services.cloudfront.GeoRestriction geoRestriction;
software.amazon.awscdk.services.cloudfront.HttpVersion httpVersion;
software.amazon.awscdk.services.cloudfront.LoggingConfiguration loggingConfig;
software.amazon.awscdk.services.cloudfront.PriceClass priceClass;
software.amazon.awscdk.services.cloudfront.ViewerCertificate viewerCertificate;
software.amazon.awscdk.services.cloudfront.ViewerProtocolPolicy viewerProtocolPolicy;
java.lang.String webAclId;
/**
* Sets the value of {@link CloudFrontWebDistributionProps#getOriginConfigs}
* @param originConfigs The origin configurations for this distribution. This parameter is required.
* Behaviors are a part of the origin.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@SuppressWarnings("unchecked")
public Builder originConfigs(java.util.List extends software.amazon.awscdk.services.cloudfront.SourceConfiguration> originConfigs) {
this.originConfigs = (java.util.List)originConfigs;
return this;
}
/**
* Sets the value of {@link CloudFrontWebDistributionProps#getAliasConfiguration}
* @param aliasConfiguration AliasConfiguration is used to configured CloudFront to respond to requests on custom domain names.
* @return {@code this}
* @deprecated see {@link CloudFrontWebDistributionProps#viewerCertificate} with {@link ViewerCertificate#acmCertificate}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Deprecated)
@Deprecated
public Builder aliasConfiguration(software.amazon.awscdk.services.cloudfront.AliasConfiguration aliasConfiguration) {
this.aliasConfiguration = aliasConfiguration;
return this;
}
/**
* Sets the value of {@link CloudFrontWebDistributionProps#getComment}
* @param comment A comment for this distribution in the CloudFront console.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder comment(java.lang.String comment) {
this.comment = comment;
return this;
}
/**
* Sets the value of {@link CloudFrontWebDistributionProps#getDefaultRootObject}
* @param defaultRootObject The default object to serve.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder defaultRootObject(java.lang.String defaultRootObject) {
this.defaultRootObject = defaultRootObject;
return this;
}
/**
* Sets the value of {@link CloudFrontWebDistributionProps#getEnabled}
* @param enabled Enable or disable the distribution.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder enabled(java.lang.Boolean enabled) {
this.enabled = enabled;
return this;
}
/**
* Sets the value of {@link CloudFrontWebDistributionProps#getEnableIpV6}
* @param enableIpV6 If your distribution should have IPv6 enabled.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder enableIpV6(java.lang.Boolean enableIpV6) {
this.enableIpV6 = enableIpV6;
return this;
}
/**
* Sets the value of {@link CloudFrontWebDistributionProps#getErrorConfigurations}
* @param errorConfigurations How CloudFront should handle requests that are not successful (eg PageNotFound).
* By default, CloudFront does not replace HTTP status codes in the 4xx and 5xx range
* with custom error messages. CloudFront does not cache HTTP status codes.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@SuppressWarnings("unchecked")
public Builder errorConfigurations(java.util.List extends software.amazon.awscdk.services.cloudfront.CfnDistribution.CustomErrorResponseProperty> errorConfigurations) {
this.errorConfigurations = (java.util.List)errorConfigurations;
return this;
}
/**
* Sets the value of {@link CloudFrontWebDistributionProps#getGeoRestriction}
* @param geoRestriction Controls the countries in which your content is distributed.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder geoRestriction(software.amazon.awscdk.services.cloudfront.GeoRestriction geoRestriction) {
this.geoRestriction = geoRestriction;
return this;
}
/**
* Sets the value of {@link CloudFrontWebDistributionProps#getHttpVersion}
* @param httpVersion The max supported HTTP Versions.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder httpVersion(software.amazon.awscdk.services.cloudfront.HttpVersion httpVersion) {
this.httpVersion = httpVersion;
return this;
}
/**
* Sets the value of {@link CloudFrontWebDistributionProps#getLoggingConfig}
* @param loggingConfig Optional - if we should enable logging.
* You can pass an empty object ({}) to have us auto create a bucket for logging.
* Omission of this property indicates no logging is to be enabled.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder loggingConfig(software.amazon.awscdk.services.cloudfront.LoggingConfiguration loggingConfig) {
this.loggingConfig = loggingConfig;
return this;
}
/**
* Sets the value of {@link CloudFrontWebDistributionProps#getPriceClass}
* @param priceClass The price class for the distribution (this impacts how many locations CloudFront uses for your distribution, and billing).
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder priceClass(software.amazon.awscdk.services.cloudfront.PriceClass priceClass) {
this.priceClass = priceClass;
return this;
}
/**
* Sets the value of {@link CloudFrontWebDistributionProps#getViewerCertificate}
* @param viewerCertificate Specifies whether you want viewers to use HTTP or HTTPS to request your objects, whether you're using an alternate domain name with HTTPS, and if so, if you're using AWS Certificate Manager (ACM) or a third-party certificate authority.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder viewerCertificate(software.amazon.awscdk.services.cloudfront.ViewerCertificate viewerCertificate) {
this.viewerCertificate = viewerCertificate;
return this;
}
/**
* Sets the value of {@link CloudFrontWebDistributionProps#getViewerProtocolPolicy}
* @param viewerProtocolPolicy The default viewer policy for incoming clients.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder viewerProtocolPolicy(software.amazon.awscdk.services.cloudfront.ViewerProtocolPolicy viewerProtocolPolicy) {
this.viewerProtocolPolicy = viewerProtocolPolicy;
return this;
}
/**
* Sets the value of {@link CloudFrontWebDistributionProps#getWebAclId}
* @param webAclId Unique identifier that specifies the AWS WAF web ACL to associate with this CloudFront distribution.
* To specify a web ACL created using the latest version of AWS WAF, use the ACL ARN, for example
* arn:aws:wafv2:us-east-1:123456789012:global/webacl/ExampleWebACL/473e64fd-f30b-4765-81a0-62ad96dd167a
.
*
* To specify a web ACL created using AWS WAF Classic, use the ACL ID, for example 473e64fd-f30b-4765-81a0-62ad96dd167a
.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder webAclId(java.lang.String webAclId) {
this.webAclId = webAclId;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link CloudFrontWebDistributionProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public CloudFrontWebDistributionProps build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link CloudFrontWebDistributionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements CloudFrontWebDistributionProps {
private final java.util.List originConfigs;
private final software.amazon.awscdk.services.cloudfront.AliasConfiguration aliasConfiguration;
private final java.lang.String comment;
private final java.lang.String defaultRootObject;
private final java.lang.Boolean enabled;
private final java.lang.Boolean enableIpV6;
private final java.util.List errorConfigurations;
private final software.amazon.awscdk.services.cloudfront.GeoRestriction geoRestriction;
private final software.amazon.awscdk.services.cloudfront.HttpVersion httpVersion;
private final software.amazon.awscdk.services.cloudfront.LoggingConfiguration loggingConfig;
private final software.amazon.awscdk.services.cloudfront.PriceClass priceClass;
private final software.amazon.awscdk.services.cloudfront.ViewerCertificate viewerCertificate;
private final software.amazon.awscdk.services.cloudfront.ViewerProtocolPolicy viewerProtocolPolicy;
private final java.lang.String webAclId;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.originConfigs = software.amazon.jsii.Kernel.get(this, "originConfigs", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.cloudfront.SourceConfiguration.class)));
this.aliasConfiguration = software.amazon.jsii.Kernel.get(this, "aliasConfiguration", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.cloudfront.AliasConfiguration.class));
this.comment = software.amazon.jsii.Kernel.get(this, "comment", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.defaultRootObject = software.amazon.jsii.Kernel.get(this, "defaultRootObject", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.enabled = software.amazon.jsii.Kernel.get(this, "enabled", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.enableIpV6 = software.amazon.jsii.Kernel.get(this, "enableIpV6", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.errorConfigurations = software.amazon.jsii.Kernel.get(this, "errorConfigurations", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.cloudfront.CfnDistribution.CustomErrorResponseProperty.class)));
this.geoRestriction = software.amazon.jsii.Kernel.get(this, "geoRestriction", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.cloudfront.GeoRestriction.class));
this.httpVersion = software.amazon.jsii.Kernel.get(this, "httpVersion", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.cloudfront.HttpVersion.class));
this.loggingConfig = software.amazon.jsii.Kernel.get(this, "loggingConfig", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.cloudfront.LoggingConfiguration.class));
this.priceClass = software.amazon.jsii.Kernel.get(this, "priceClass", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.cloudfront.PriceClass.class));
this.viewerCertificate = software.amazon.jsii.Kernel.get(this, "viewerCertificate", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.cloudfront.ViewerCertificate.class));
this.viewerProtocolPolicy = software.amazon.jsii.Kernel.get(this, "viewerProtocolPolicy", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.cloudfront.ViewerProtocolPolicy.class));
this.webAclId = software.amazon.jsii.Kernel.get(this, "webACLId", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
@SuppressWarnings("unchecked")
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.originConfigs = (java.util.List)java.util.Objects.requireNonNull(builder.originConfigs, "originConfigs is required");
this.aliasConfiguration = builder.aliasConfiguration;
this.comment = builder.comment;
this.defaultRootObject = builder.defaultRootObject;
this.enabled = builder.enabled;
this.enableIpV6 = builder.enableIpV6;
this.errorConfigurations = (java.util.List)builder.errorConfigurations;
this.geoRestriction = builder.geoRestriction;
this.httpVersion = builder.httpVersion;
this.loggingConfig = builder.loggingConfig;
this.priceClass = builder.priceClass;
this.viewerCertificate = builder.viewerCertificate;
this.viewerProtocolPolicy = builder.viewerProtocolPolicy;
this.webAclId = builder.webAclId;
}
@Override
public final java.util.List getOriginConfigs() {
return this.originConfigs;
}
@Override
public final software.amazon.awscdk.services.cloudfront.AliasConfiguration getAliasConfiguration() {
return this.aliasConfiguration;
}
@Override
public final java.lang.String getComment() {
return this.comment;
}
@Override
public final java.lang.String getDefaultRootObject() {
return this.defaultRootObject;
}
@Override
public final java.lang.Boolean getEnabled() {
return this.enabled;
}
@Override
public final java.lang.Boolean getEnableIpV6() {
return this.enableIpV6;
}
@Override
public final java.util.List getErrorConfigurations() {
return this.errorConfigurations;
}
@Override
public final software.amazon.awscdk.services.cloudfront.GeoRestriction getGeoRestriction() {
return this.geoRestriction;
}
@Override
public final software.amazon.awscdk.services.cloudfront.HttpVersion getHttpVersion() {
return this.httpVersion;
}
@Override
public final software.amazon.awscdk.services.cloudfront.LoggingConfiguration getLoggingConfig() {
return this.loggingConfig;
}
@Override
public final software.amazon.awscdk.services.cloudfront.PriceClass getPriceClass() {
return this.priceClass;
}
@Override
public final software.amazon.awscdk.services.cloudfront.ViewerCertificate getViewerCertificate() {
return this.viewerCertificate;
}
@Override
public final software.amazon.awscdk.services.cloudfront.ViewerProtocolPolicy getViewerProtocolPolicy() {
return this.viewerProtocolPolicy;
}
@Override
public final java.lang.String getWebACLId() {
return this.webAclId;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("originConfigs", om.valueToTree(this.getOriginConfigs()));
if (this.getAliasConfiguration() != null) {
data.set("aliasConfiguration", om.valueToTree(this.getAliasConfiguration()));
}
if (this.getComment() != null) {
data.set("comment", om.valueToTree(this.getComment()));
}
if (this.getDefaultRootObject() != null) {
data.set("defaultRootObject", om.valueToTree(this.getDefaultRootObject()));
}
if (this.getEnabled() != null) {
data.set("enabled", om.valueToTree(this.getEnabled()));
}
if (this.getEnableIpV6() != null) {
data.set("enableIpV6", om.valueToTree(this.getEnableIpV6()));
}
if (this.getErrorConfigurations() != null) {
data.set("errorConfigurations", om.valueToTree(this.getErrorConfigurations()));
}
if (this.getGeoRestriction() != null) {
data.set("geoRestriction", om.valueToTree(this.getGeoRestriction()));
}
if (this.getHttpVersion() != null) {
data.set("httpVersion", om.valueToTree(this.getHttpVersion()));
}
if (this.getLoggingConfig() != null) {
data.set("loggingConfig", om.valueToTree(this.getLoggingConfig()));
}
if (this.getPriceClass() != null) {
data.set("priceClass", om.valueToTree(this.getPriceClass()));
}
if (this.getViewerCertificate() != null) {
data.set("viewerCertificate", om.valueToTree(this.getViewerCertificate()));
}
if (this.getViewerProtocolPolicy() != null) {
data.set("viewerProtocolPolicy", om.valueToTree(this.getViewerProtocolPolicy()));
}
if (this.getWebACLId() != null) {
data.set("webACLId", om.valueToTree(this.getWebACLId()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-cloudfront.CloudFrontWebDistributionProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
CloudFrontWebDistributionProps.Jsii$Proxy that = (CloudFrontWebDistributionProps.Jsii$Proxy) o;
if (!originConfigs.equals(that.originConfigs)) return false;
if (this.aliasConfiguration != null ? !this.aliasConfiguration.equals(that.aliasConfiguration) : that.aliasConfiguration != null) return false;
if (this.comment != null ? !this.comment.equals(that.comment) : that.comment != null) return false;
if (this.defaultRootObject != null ? !this.defaultRootObject.equals(that.defaultRootObject) : that.defaultRootObject != null) return false;
if (this.enabled != null ? !this.enabled.equals(that.enabled) : that.enabled != null) return false;
if (this.enableIpV6 != null ? !this.enableIpV6.equals(that.enableIpV6) : that.enableIpV6 != null) return false;
if (this.errorConfigurations != null ? !this.errorConfigurations.equals(that.errorConfigurations) : that.errorConfigurations != null) return false;
if (this.geoRestriction != null ? !this.geoRestriction.equals(that.geoRestriction) : that.geoRestriction != null) return false;
if (this.httpVersion != null ? !this.httpVersion.equals(that.httpVersion) : that.httpVersion != null) return false;
if (this.loggingConfig != null ? !this.loggingConfig.equals(that.loggingConfig) : that.loggingConfig != null) return false;
if (this.priceClass != null ? !this.priceClass.equals(that.priceClass) : that.priceClass != null) return false;
if (this.viewerCertificate != null ? !this.viewerCertificate.equals(that.viewerCertificate) : that.viewerCertificate != null) return false;
if (this.viewerProtocolPolicy != null ? !this.viewerProtocolPolicy.equals(that.viewerProtocolPolicy) : that.viewerProtocolPolicy != null) return false;
return this.webAclId != null ? this.webAclId.equals(that.webAclId) : that.webAclId == null;
}
@Override
public final int hashCode() {
int result = this.originConfigs.hashCode();
result = 31 * result + (this.aliasConfiguration != null ? this.aliasConfiguration.hashCode() : 0);
result = 31 * result + (this.comment != null ? this.comment.hashCode() : 0);
result = 31 * result + (this.defaultRootObject != null ? this.defaultRootObject.hashCode() : 0);
result = 31 * result + (this.enabled != null ? this.enabled.hashCode() : 0);
result = 31 * result + (this.enableIpV6 != null ? this.enableIpV6.hashCode() : 0);
result = 31 * result + (this.errorConfigurations != null ? this.errorConfigurations.hashCode() : 0);
result = 31 * result + (this.geoRestriction != null ? this.geoRestriction.hashCode() : 0);
result = 31 * result + (this.httpVersion != null ? this.httpVersion.hashCode() : 0);
result = 31 * result + (this.loggingConfig != null ? this.loggingConfig.hashCode() : 0);
result = 31 * result + (this.priceClass != null ? this.priceClass.hashCode() : 0);
result = 31 * result + (this.viewerCertificate != null ? this.viewerCertificate.hashCode() : 0);
result = 31 * result + (this.viewerProtocolPolicy != null ? this.viewerProtocolPolicy.hashCode() : 0);
result = 31 * result + (this.webAclId != null ? this.webAclId.hashCode() : 0);
return result;
}
}
}