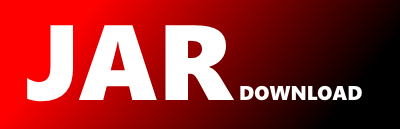
software.amazon.awscdk.services.cloudfront.CustomOriginConfig Maven / Gradle / Ivy
Show all versions of cloudfront Show documentation
package software.amazon.awscdk.services.cloudfront;
/**
* A custom origin configuration.
*
* Example:
*
*
* Bucket sourceBucket;
* OriginAccessIdentity oai;
* CloudFrontWebDistribution.Builder.create(this, "MyCfWebDistribution")
* .originConfigs(List.of(SourceConfiguration.builder()
* .s3OriginSource(S3OriginConfig.builder()
* .s3BucketSource(sourceBucket)
* .originAccessIdentity(oai)
* .build())
* .behaviors(List.of(Behavior.builder().isDefaultBehavior(true).build()))
* .build(), SourceConfiguration.builder()
* .customOriginSource(CustomOriginConfig.builder()
* .domainName("MYALIAS")
* .build())
* .behaviors(List.of(Behavior.builder().pathPattern("/somewhere").build()))
* .build()))
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.84.0 (build 5404dcf)", date = "2023-06-19T16:30:43.671Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.cloudfront.$Module.class, fqn = "@aws-cdk/aws-cloudfront.CustomOriginConfig")
@software.amazon.jsii.Jsii.Proxy(CustomOriginConfig.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface CustomOriginConfig extends software.amazon.jsii.JsiiSerializable {
/**
* The domain name of the custom origin.
*
* Should not include the path - that should be in the parent SourceConfiguration
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getDomainName();
/**
* The SSL versions to use when interacting with the origin.
*
* Default: OriginSslPolicy.TLS_V1_2
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getAllowedOriginSSLVersions() {
return null;
}
/**
* The origin HTTP port.
*
* Default: 80
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Number getHttpPort() {
return null;
}
/**
* The origin HTTPS port.
*
* Default: 443
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Number getHttpsPort() {
return null;
}
/**
* Any additional headers to pass to the origin.
*
* Default: - No additional headers are passed.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.Map getOriginHeaders() {
return null;
}
/**
* The keep alive timeout when making calls in seconds.
*
* Default: Duration.seconds(5)
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.core.Duration getOriginKeepaliveTimeout() {
return null;
}
/**
* The relative path to the origin root to use for sources.
*
* Default: /
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getOriginPath() {
return null;
}
/**
* The protocol (http or https) policy to use when interacting with the origin.
*
* Default: OriginProtocolPolicy.HttpsOnly
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.cloudfront.OriginProtocolPolicy getOriginProtocolPolicy() {
return null;
}
/**
* The read timeout when calling the origin in seconds.
*
* Default: Duration.seconds(30)
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.core.Duration getOriginReadTimeout() {
return null;
}
/**
* When you enable Origin Shield in the AWS Region that has the lowest latency to your origin, you can get better network performance.
*
* Default: - origin shield not enabled
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getOriginShieldRegion() {
return null;
}
/**
* @return a {@link Builder} of {@link CustomOriginConfig}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link CustomOriginConfig}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String domainName;
java.util.List allowedOriginSslVersions;
java.lang.Number httpPort;
java.lang.Number httpsPort;
java.util.Map originHeaders;
software.amazon.awscdk.core.Duration originKeepaliveTimeout;
java.lang.String originPath;
software.amazon.awscdk.services.cloudfront.OriginProtocolPolicy originProtocolPolicy;
software.amazon.awscdk.core.Duration originReadTimeout;
java.lang.String originShieldRegion;
/**
* Sets the value of {@link CustomOriginConfig#getDomainName}
* @param domainName The domain name of the custom origin. This parameter is required.
* Should not include the path - that should be in the parent SourceConfiguration
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder domainName(java.lang.String domainName) {
this.domainName = domainName;
return this;
}
/**
* Sets the value of {@link CustomOriginConfig#getAllowedOriginSslVersions}
* @param allowedOriginSslVersions The SSL versions to use when interacting with the origin.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@SuppressWarnings("unchecked")
public Builder allowedOriginSslVersions(java.util.List extends software.amazon.awscdk.services.cloudfront.OriginSslPolicy> allowedOriginSslVersions) {
this.allowedOriginSslVersions = (java.util.List)allowedOriginSslVersions;
return this;
}
/**
* Sets the value of {@link CustomOriginConfig#getHttpPort}
* @param httpPort The origin HTTP port.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder httpPort(java.lang.Number httpPort) {
this.httpPort = httpPort;
return this;
}
/**
* Sets the value of {@link CustomOriginConfig#getHttpsPort}
* @param httpsPort The origin HTTPS port.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder httpsPort(java.lang.Number httpsPort) {
this.httpsPort = httpsPort;
return this;
}
/**
* Sets the value of {@link CustomOriginConfig#getOriginHeaders}
* @param originHeaders Any additional headers to pass to the origin.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder originHeaders(java.util.Map originHeaders) {
this.originHeaders = originHeaders;
return this;
}
/**
* Sets the value of {@link CustomOriginConfig#getOriginKeepaliveTimeout}
* @param originKeepaliveTimeout The keep alive timeout when making calls in seconds.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder originKeepaliveTimeout(software.amazon.awscdk.core.Duration originKeepaliveTimeout) {
this.originKeepaliveTimeout = originKeepaliveTimeout;
return this;
}
/**
* Sets the value of {@link CustomOriginConfig#getOriginPath}
* @param originPath The relative path to the origin root to use for sources.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder originPath(java.lang.String originPath) {
this.originPath = originPath;
return this;
}
/**
* Sets the value of {@link CustomOriginConfig#getOriginProtocolPolicy}
* @param originProtocolPolicy The protocol (http or https) policy to use when interacting with the origin.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder originProtocolPolicy(software.amazon.awscdk.services.cloudfront.OriginProtocolPolicy originProtocolPolicy) {
this.originProtocolPolicy = originProtocolPolicy;
return this;
}
/**
* Sets the value of {@link CustomOriginConfig#getOriginReadTimeout}
* @param originReadTimeout The read timeout when calling the origin in seconds.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder originReadTimeout(software.amazon.awscdk.core.Duration originReadTimeout) {
this.originReadTimeout = originReadTimeout;
return this;
}
/**
* Sets the value of {@link CustomOriginConfig#getOriginShieldRegion}
* @param originShieldRegion When you enable Origin Shield in the AWS Region that has the lowest latency to your origin, you can get better network performance.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder originShieldRegion(java.lang.String originShieldRegion) {
this.originShieldRegion = originShieldRegion;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link CustomOriginConfig}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public CustomOriginConfig build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link CustomOriginConfig}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements CustomOriginConfig {
private final java.lang.String domainName;
private final java.util.List allowedOriginSslVersions;
private final java.lang.Number httpPort;
private final java.lang.Number httpsPort;
private final java.util.Map originHeaders;
private final software.amazon.awscdk.core.Duration originKeepaliveTimeout;
private final java.lang.String originPath;
private final software.amazon.awscdk.services.cloudfront.OriginProtocolPolicy originProtocolPolicy;
private final software.amazon.awscdk.core.Duration originReadTimeout;
private final java.lang.String originShieldRegion;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.domainName = software.amazon.jsii.Kernel.get(this, "domainName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.allowedOriginSslVersions = software.amazon.jsii.Kernel.get(this, "allowedOriginSSLVersions", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.cloudfront.OriginSslPolicy.class)));
this.httpPort = software.amazon.jsii.Kernel.get(this, "httpPort", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.httpsPort = software.amazon.jsii.Kernel.get(this, "httpsPort", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.originHeaders = software.amazon.jsii.Kernel.get(this, "originHeaders", software.amazon.jsii.NativeType.mapOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class)));
this.originKeepaliveTimeout = software.amazon.jsii.Kernel.get(this, "originKeepaliveTimeout", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.Duration.class));
this.originPath = software.amazon.jsii.Kernel.get(this, "originPath", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.originProtocolPolicy = software.amazon.jsii.Kernel.get(this, "originProtocolPolicy", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.cloudfront.OriginProtocolPolicy.class));
this.originReadTimeout = software.amazon.jsii.Kernel.get(this, "originReadTimeout", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.Duration.class));
this.originShieldRegion = software.amazon.jsii.Kernel.get(this, "originShieldRegion", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
@SuppressWarnings("unchecked")
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.domainName = java.util.Objects.requireNonNull(builder.domainName, "domainName is required");
this.allowedOriginSslVersions = (java.util.List)builder.allowedOriginSslVersions;
this.httpPort = builder.httpPort;
this.httpsPort = builder.httpsPort;
this.originHeaders = builder.originHeaders;
this.originKeepaliveTimeout = builder.originKeepaliveTimeout;
this.originPath = builder.originPath;
this.originProtocolPolicy = builder.originProtocolPolicy;
this.originReadTimeout = builder.originReadTimeout;
this.originShieldRegion = builder.originShieldRegion;
}
@Override
public final java.lang.String getDomainName() {
return this.domainName;
}
@Override
public final java.util.List getAllowedOriginSSLVersions() {
return this.allowedOriginSslVersions;
}
@Override
public final java.lang.Number getHttpPort() {
return this.httpPort;
}
@Override
public final java.lang.Number getHttpsPort() {
return this.httpsPort;
}
@Override
public final java.util.Map getOriginHeaders() {
return this.originHeaders;
}
@Override
public final software.amazon.awscdk.core.Duration getOriginKeepaliveTimeout() {
return this.originKeepaliveTimeout;
}
@Override
public final java.lang.String getOriginPath() {
return this.originPath;
}
@Override
public final software.amazon.awscdk.services.cloudfront.OriginProtocolPolicy getOriginProtocolPolicy() {
return this.originProtocolPolicy;
}
@Override
public final software.amazon.awscdk.core.Duration getOriginReadTimeout() {
return this.originReadTimeout;
}
@Override
public final java.lang.String getOriginShieldRegion() {
return this.originShieldRegion;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("domainName", om.valueToTree(this.getDomainName()));
if (this.getAllowedOriginSSLVersions() != null) {
data.set("allowedOriginSSLVersions", om.valueToTree(this.getAllowedOriginSSLVersions()));
}
if (this.getHttpPort() != null) {
data.set("httpPort", om.valueToTree(this.getHttpPort()));
}
if (this.getHttpsPort() != null) {
data.set("httpsPort", om.valueToTree(this.getHttpsPort()));
}
if (this.getOriginHeaders() != null) {
data.set("originHeaders", om.valueToTree(this.getOriginHeaders()));
}
if (this.getOriginKeepaliveTimeout() != null) {
data.set("originKeepaliveTimeout", om.valueToTree(this.getOriginKeepaliveTimeout()));
}
if (this.getOriginPath() != null) {
data.set("originPath", om.valueToTree(this.getOriginPath()));
}
if (this.getOriginProtocolPolicy() != null) {
data.set("originProtocolPolicy", om.valueToTree(this.getOriginProtocolPolicy()));
}
if (this.getOriginReadTimeout() != null) {
data.set("originReadTimeout", om.valueToTree(this.getOriginReadTimeout()));
}
if (this.getOriginShieldRegion() != null) {
data.set("originShieldRegion", om.valueToTree(this.getOriginShieldRegion()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-cloudfront.CustomOriginConfig"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
CustomOriginConfig.Jsii$Proxy that = (CustomOriginConfig.Jsii$Proxy) o;
if (!domainName.equals(that.domainName)) return false;
if (this.allowedOriginSslVersions != null ? !this.allowedOriginSslVersions.equals(that.allowedOriginSslVersions) : that.allowedOriginSslVersions != null) return false;
if (this.httpPort != null ? !this.httpPort.equals(that.httpPort) : that.httpPort != null) return false;
if (this.httpsPort != null ? !this.httpsPort.equals(that.httpsPort) : that.httpsPort != null) return false;
if (this.originHeaders != null ? !this.originHeaders.equals(that.originHeaders) : that.originHeaders != null) return false;
if (this.originKeepaliveTimeout != null ? !this.originKeepaliveTimeout.equals(that.originKeepaliveTimeout) : that.originKeepaliveTimeout != null) return false;
if (this.originPath != null ? !this.originPath.equals(that.originPath) : that.originPath != null) return false;
if (this.originProtocolPolicy != null ? !this.originProtocolPolicy.equals(that.originProtocolPolicy) : that.originProtocolPolicy != null) return false;
if (this.originReadTimeout != null ? !this.originReadTimeout.equals(that.originReadTimeout) : that.originReadTimeout != null) return false;
return this.originShieldRegion != null ? this.originShieldRegion.equals(that.originShieldRegion) : that.originShieldRegion == null;
}
@Override
public final int hashCode() {
int result = this.domainName.hashCode();
result = 31 * result + (this.allowedOriginSslVersions != null ? this.allowedOriginSslVersions.hashCode() : 0);
result = 31 * result + (this.httpPort != null ? this.httpPort.hashCode() : 0);
result = 31 * result + (this.httpsPort != null ? this.httpsPort.hashCode() : 0);
result = 31 * result + (this.originHeaders != null ? this.originHeaders.hashCode() : 0);
result = 31 * result + (this.originKeepaliveTimeout != null ? this.originKeepaliveTimeout.hashCode() : 0);
result = 31 * result + (this.originPath != null ? this.originPath.hashCode() : 0);
result = 31 * result + (this.originProtocolPolicy != null ? this.originProtocolPolicy.hashCode() : 0);
result = 31 * result + (this.originReadTimeout != null ? this.originReadTimeout.hashCode() : 0);
result = 31 * result + (this.originShieldRegion != null ? this.originShieldRegion.hashCode() : 0);
return result;
}
}
}