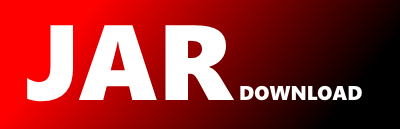
software.amazon.awscdk.services.cloudwatch.DashboardProps Maven / Gradle / Ivy
Show all versions of cloudwatch Show documentation
package software.amazon.awscdk.services.cloudwatch;
/**
* Properties for defining a CloudWatch Dashboard.
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.30.0 (build adae23f)", date = "2021-06-17T00:23:00.252Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.cloudwatch.$Module.class, fqn = "@aws-cdk/aws-cloudwatch.DashboardProps")
@software.amazon.jsii.Jsii.Proxy(DashboardProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface DashboardProps extends software.amazon.jsii.JsiiSerializable {
/**
* Name of the dashboard.
*
* If set, must only contain alphanumerics, dash (-) and underscore (_)
*
* Default: - automatically generated name
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getDashboardName() {
return null;
}
/**
* The end of the time range to use for each widget on the dashboard when the dashboard loads.
*
* If you specify a value for end, you must also specify a value for start.
* Specify an absolute time in the ISO 8601 format. For example, 2018-12-17T06:00:00.000Z.
*
* Default: When the dashboard loads, the end date will be the current time.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getEnd() {
return null;
}
/**
* Use this field to specify the period for the graphs when the dashboard loads.
*
* Specifying Auto
causes the period of all graphs on the dashboard to automatically adapt to the time range of the dashboard.
* Specifying Inherit
ensures that the period set for each graph is always obeyed.
*
* Default: Auto
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.cloudwatch.PeriodOverride getPeriodOverride() {
return null;
}
/**
* The start of the time range to use for each widget on the dashboard.
*
* You can specify start without specifying end to specify a relative time range that ends with the current time.
* In this case, the value of start must begin with -P, and you can use M, H, D, W and M as abbreviations for
* minutes, hours, days, weeks and months. For example, -PT8H shows the last 8 hours and -P3M shows the last three months.
* You can also use start along with an end field, to specify an absolute time range.
* When specifying an absolute time range, use the ISO 8601 format. For example, 2018-12-17T06:00:00.000Z.
*
* Default: When the dashboard loads, the start time will be the default time range.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getStart() {
return null;
}
/**
* Initial set of widgets on the dashboard.
*
* One array represents a row of widgets.
*
* Default: - No widgets
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List> getWidgets() {
return null;
}
/**
* @return a {@link Builder} of {@link DashboardProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link DashboardProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
private java.lang.String dashboardName;
private java.lang.String end;
private software.amazon.awscdk.services.cloudwatch.PeriodOverride periodOverride;
private java.lang.String start;
private java.util.List> widgets;
/**
* Sets the value of {@link DashboardProps#getDashboardName}
* @param dashboardName Name of the dashboard.
* If set, must only contain alphanumerics, dash (-) and underscore (_)
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder dashboardName(java.lang.String dashboardName) {
this.dashboardName = dashboardName;
return this;
}
/**
* Sets the value of {@link DashboardProps#getEnd}
* @param end The end of the time range to use for each widget on the dashboard when the dashboard loads.
* If you specify a value for end, you must also specify a value for start.
* Specify an absolute time in the ISO 8601 format. For example, 2018-12-17T06:00:00.000Z.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder end(java.lang.String end) {
this.end = end;
return this;
}
/**
* Sets the value of {@link DashboardProps#getPeriodOverride}
* @param periodOverride Use this field to specify the period for the graphs when the dashboard loads.
* Specifying Auto
causes the period of all graphs on the dashboard to automatically adapt to the time range of the dashboard.
* Specifying Inherit
ensures that the period set for each graph is always obeyed.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder periodOverride(software.amazon.awscdk.services.cloudwatch.PeriodOverride periodOverride) {
this.periodOverride = periodOverride;
return this;
}
/**
* Sets the value of {@link DashboardProps#getStart}
* @param start The start of the time range to use for each widget on the dashboard.
* You can specify start without specifying end to specify a relative time range that ends with the current time.
* In this case, the value of start must begin with -P, and you can use M, H, D, W and M as abbreviations for
* minutes, hours, days, weeks and months. For example, -PT8H shows the last 8 hours and -P3M shows the last three months.
* You can also use start along with an end field, to specify an absolute time range.
* When specifying an absolute time range, use the ISO 8601 format. For example, 2018-12-17T06:00:00.000Z.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder start(java.lang.String start) {
this.start = start;
return this;
}
/**
* Sets the value of {@link DashboardProps#getWidgets}
* @param widgets Initial set of widgets on the dashboard.
* One array represents a row of widgets.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@SuppressWarnings("unchecked")
public Builder widgets(java.util.List extends java.util.List extends software.amazon.awscdk.services.cloudwatch.IWidget>> widgets) {
this.widgets = (java.util.List>)widgets;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link DashboardProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public DashboardProps build() {
return new Jsii$Proxy(dashboardName, end, periodOverride, start, widgets);
}
}
/**
* An implementation for {@link DashboardProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements DashboardProps {
private final java.lang.String dashboardName;
private final java.lang.String end;
private final software.amazon.awscdk.services.cloudwatch.PeriodOverride periodOverride;
private final java.lang.String start;
private final java.util.List> widgets;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.dashboardName = software.amazon.jsii.Kernel.get(this, "dashboardName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.end = software.amazon.jsii.Kernel.get(this, "end", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.periodOverride = software.amazon.jsii.Kernel.get(this, "periodOverride", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.cloudwatch.PeriodOverride.class));
this.start = software.amazon.jsii.Kernel.get(this, "start", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.widgets = software.amazon.jsii.Kernel.get(this, "widgets", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.cloudwatch.IWidget.class))));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
@SuppressWarnings("unchecked")
protected Jsii$Proxy(final java.lang.String dashboardName, final java.lang.String end, final software.amazon.awscdk.services.cloudwatch.PeriodOverride periodOverride, final java.lang.String start, final java.util.List extends java.util.List extends software.amazon.awscdk.services.cloudwatch.IWidget>> widgets) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.dashboardName = dashboardName;
this.end = end;
this.periodOverride = periodOverride;
this.start = start;
this.widgets = (java.util.List>)widgets;
}
@Override
public final java.lang.String getDashboardName() {
return this.dashboardName;
}
@Override
public final java.lang.String getEnd() {
return this.end;
}
@Override
public final software.amazon.awscdk.services.cloudwatch.PeriodOverride getPeriodOverride() {
return this.periodOverride;
}
@Override
public final java.lang.String getStart() {
return this.start;
}
@Override
public final java.util.List> getWidgets() {
return this.widgets;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getDashboardName() != null) {
data.set("dashboardName", om.valueToTree(this.getDashboardName()));
}
if (this.getEnd() != null) {
data.set("end", om.valueToTree(this.getEnd()));
}
if (this.getPeriodOverride() != null) {
data.set("periodOverride", om.valueToTree(this.getPeriodOverride()));
}
if (this.getStart() != null) {
data.set("start", om.valueToTree(this.getStart()));
}
if (this.getWidgets() != null) {
data.set("widgets", om.valueToTree(this.getWidgets()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-cloudwatch.DashboardProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
DashboardProps.Jsii$Proxy that = (DashboardProps.Jsii$Proxy) o;
if (this.dashboardName != null ? !this.dashboardName.equals(that.dashboardName) : that.dashboardName != null) return false;
if (this.end != null ? !this.end.equals(that.end) : that.end != null) return false;
if (this.periodOverride != null ? !this.periodOverride.equals(that.periodOverride) : that.periodOverride != null) return false;
if (this.start != null ? !this.start.equals(that.start) : that.start != null) return false;
return this.widgets != null ? this.widgets.equals(that.widgets) : that.widgets == null;
}
@Override
public final int hashCode() {
int result = this.dashboardName != null ? this.dashboardName.hashCode() : 0;
result = 31 * result + (this.end != null ? this.end.hashCode() : 0);
result = 31 * result + (this.periodOverride != null ? this.periodOverride.hashCode() : 0);
result = 31 * result + (this.start != null ? this.start.hashCode() : 0);
result = 31 * result + (this.widgets != null ? this.widgets.hashCode() : 0);
return result;
}
}
}