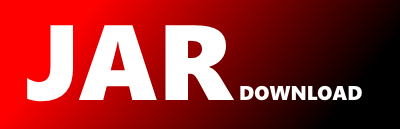
software.amazon.awscdk.services.cloudwatch.HorizontalAnnotation Maven / Gradle / Ivy
Show all versions of cloudwatch Show documentation
package software.amazon.awscdk.services.cloudwatch;
/**
* Horizontal annotation to be added to a graph.
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.30.0 (build adae23f)", date = "2021-06-17T00:23:00.253Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.cloudwatch.$Module.class, fqn = "@aws-cdk/aws-cloudwatch.HorizontalAnnotation")
@software.amazon.jsii.Jsii.Proxy(HorizontalAnnotation.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface HorizontalAnnotation extends software.amazon.jsii.JsiiSerializable {
/**
* The value of the annotation.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.Number getValue();
/**
* The hex color code, prefixed with '#' (e.g. '#00ff00'), to be used for the annotation. The `Color` class has a set of standard colors that can be used here.
*
* Default: - Automatic color
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getColor() {
return null;
}
/**
* Add shading above or below the annotation.
*
* Default: No shading
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.cloudwatch.Shading getFill() {
return null;
}
/**
* Label for the annotation.
*
* Default: - No label
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getLabel() {
return null;
}
/**
* Whether the annotation is visible.
*
* Default: true
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Boolean getVisible() {
return null;
}
/**
* @return a {@link Builder} of {@link HorizontalAnnotation}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link HorizontalAnnotation}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
private java.lang.Number value;
private java.lang.String color;
private software.amazon.awscdk.services.cloudwatch.Shading fill;
private java.lang.String label;
private java.lang.Boolean visible;
/**
* Sets the value of {@link HorizontalAnnotation#getValue}
* @param value The value of the annotation. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder value(java.lang.Number value) {
this.value = value;
return this;
}
/**
* Sets the value of {@link HorizontalAnnotation#getColor}
* @param color The hex color code, prefixed with '#' (e.g. '#00ff00'), to be used for the annotation. The `Color` class has a set of standard colors that can be used here.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder color(java.lang.String color) {
this.color = color;
return this;
}
/**
* Sets the value of {@link HorizontalAnnotation#getFill}
* @param fill Add shading above or below the annotation.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder fill(software.amazon.awscdk.services.cloudwatch.Shading fill) {
this.fill = fill;
return this;
}
/**
* Sets the value of {@link HorizontalAnnotation#getLabel}
* @param label Label for the annotation.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder label(java.lang.String label) {
this.label = label;
return this;
}
/**
* Sets the value of {@link HorizontalAnnotation#getVisible}
* @param visible Whether the annotation is visible.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder visible(java.lang.Boolean visible) {
this.visible = visible;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link HorizontalAnnotation}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public HorizontalAnnotation build() {
return new Jsii$Proxy(value, color, fill, label, visible);
}
}
/**
* An implementation for {@link HorizontalAnnotation}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements HorizontalAnnotation {
private final java.lang.Number value;
private final java.lang.String color;
private final software.amazon.awscdk.services.cloudwatch.Shading fill;
private final java.lang.String label;
private final java.lang.Boolean visible;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.value = software.amazon.jsii.Kernel.get(this, "value", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.color = software.amazon.jsii.Kernel.get(this, "color", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.fill = software.amazon.jsii.Kernel.get(this, "fill", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.cloudwatch.Shading.class));
this.label = software.amazon.jsii.Kernel.get(this, "label", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.visible = software.amazon.jsii.Kernel.get(this, "visible", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final java.lang.Number value, final java.lang.String color, final software.amazon.awscdk.services.cloudwatch.Shading fill, final java.lang.String label, final java.lang.Boolean visible) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.value = java.util.Objects.requireNonNull(value, "value is required");
this.color = color;
this.fill = fill;
this.label = label;
this.visible = visible;
}
@Override
public final java.lang.Number getValue() {
return this.value;
}
@Override
public final java.lang.String getColor() {
return this.color;
}
@Override
public final software.amazon.awscdk.services.cloudwatch.Shading getFill() {
return this.fill;
}
@Override
public final java.lang.String getLabel() {
return this.label;
}
@Override
public final java.lang.Boolean getVisible() {
return this.visible;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("value", om.valueToTree(this.getValue()));
if (this.getColor() != null) {
data.set("color", om.valueToTree(this.getColor()));
}
if (this.getFill() != null) {
data.set("fill", om.valueToTree(this.getFill()));
}
if (this.getLabel() != null) {
data.set("label", om.valueToTree(this.getLabel()));
}
if (this.getVisible() != null) {
data.set("visible", om.valueToTree(this.getVisible()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-cloudwatch.HorizontalAnnotation"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
HorizontalAnnotation.Jsii$Proxy that = (HorizontalAnnotation.Jsii$Proxy) o;
if (!value.equals(that.value)) return false;
if (this.color != null ? !this.color.equals(that.color) : that.color != null) return false;
if (this.fill != null ? !this.fill.equals(that.fill) : that.fill != null) return false;
if (this.label != null ? !this.label.equals(that.label) : that.label != null) return false;
return this.visible != null ? this.visible.equals(that.visible) : that.visible == null;
}
@Override
public final int hashCode() {
int result = this.value.hashCode();
result = 31 * result + (this.color != null ? this.color.hashCode() : 0);
result = 31 * result + (this.fill != null ? this.fill.hashCode() : 0);
result = 31 * result + (this.label != null ? this.label.hashCode() : 0);
result = 31 * result + (this.visible != null ? this.visible.hashCode() : 0);
return result;
}
}
}