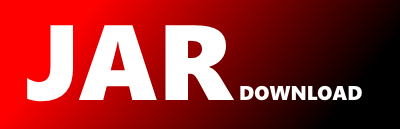
software.amazon.awscdk.services.cloudwatch.CommonMetricOptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cloudwatch Show documentation
Show all versions of cloudwatch Show documentation
The CDK Construct Library for AWS::CloudWatch
package software.amazon.awscdk.services.cloudwatch;
/**
* Options shared by most methods accepting metric options.
*/
@javax.annotation.Generated(value = "jsii-pacmak/0.19.0 (build 7c562bc)", date = "2019-10-22T08:20:38.280Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface CommonMetricOptions extends software.amazon.jsii.JsiiSerializable {
/**
* Color for this metric when added to a Graph in a Dashboard.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
java.lang.String getColor();
/**
* Dimensions of the metric.
*
* Default: - No dimensions.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
java.util.Map getDimensions();
/**
* Label for this metric when added to a Graph in a Dashboard.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
java.lang.String getLabel();
/**
* The period over which the specified statistic is applied.
*
* Default: Duration.minutes(5)
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
software.amazon.awscdk.core.Duration getPeriod();
/**
* What function to use for aggregating.
*
* Can be one of the following:
*
* - "Minimum" | "min"
* - "Maximum" | "max"
* - "Average" | "avg"
* - "Sum" | "sum"
* - "SampleCount | "n"
* - "pNN.NN"
*
* Default: Average
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
java.lang.String getStatistic();
/**
* Unit for the metric that is associated with the alarm.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
software.amazon.awscdk.services.cloudwatch.Unit getUnit();
/**
* @return a {@link Builder} of {@link CommonMetricOptions}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link CommonMetricOptions}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder {
private java.lang.String color;
private java.util.Map dimensions;
private java.lang.String label;
private software.amazon.awscdk.core.Duration period;
private java.lang.String statistic;
private software.amazon.awscdk.services.cloudwatch.Unit unit;
/**
* Sets the value of Color
* @param color Color for this metric when added to a Graph in a Dashboard.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder color(java.lang.String color) {
this.color = color;
return this;
}
/**
* Sets the value of Dimensions
* @param dimensions Dimensions of the metric.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder dimensions(java.util.Map dimensions) {
this.dimensions = dimensions;
return this;
}
/**
* Sets the value of Label
* @param label Label for this metric when added to a Graph in a Dashboard.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder label(java.lang.String label) {
this.label = label;
return this;
}
/**
* Sets the value of Period
* @param period The period over which the specified statistic is applied.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder period(software.amazon.awscdk.core.Duration period) {
this.period = period;
return this;
}
/**
* Sets the value of Statistic
* @param statistic What function to use for aggregating.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder statistic(java.lang.String statistic) {
this.statistic = statistic;
return this;
}
/**
* Sets the value of Unit
* @param unit Unit for the metric that is associated with the alarm.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder unit(software.amazon.awscdk.services.cloudwatch.Unit unit) {
this.unit = unit;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link CommonMetricOptions}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public CommonMetricOptions build() {
return new Jsii$Proxy(color, dimensions, label, period, statistic, unit);
}
}
/**
* An implementation for {@link CommonMetricOptions}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements CommonMetricOptions {
private final java.lang.String color;
private final java.util.Map dimensions;
private final java.lang.String label;
private final software.amazon.awscdk.core.Duration period;
private final java.lang.String statistic;
private final software.amazon.awscdk.services.cloudwatch.Unit unit;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.setObjRef(objRef);
this.color = this.jsiiGet("color", java.lang.String.class);
this.dimensions = this.jsiiGet("dimensions", java.util.Map.class);
this.label = this.jsiiGet("label", java.lang.String.class);
this.period = this.jsiiGet("period", software.amazon.awscdk.core.Duration.class);
this.statistic = this.jsiiGet("statistic", java.lang.String.class);
this.unit = this.jsiiGet("unit", software.amazon.awscdk.services.cloudwatch.Unit.class);
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
private Jsii$Proxy(java.lang.String color, java.util.Map dimensions, java.lang.String label, software.amazon.awscdk.core.Duration period, java.lang.String statistic, software.amazon.awscdk.services.cloudwatch.Unit unit) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.color = color;
this.dimensions = dimensions;
this.label = label;
this.period = period;
this.statistic = statistic;
this.unit = unit;
}
@Override
public java.lang.String getColor() {
return this.color;
}
@Override
public java.util.Map getDimensions() {
return this.dimensions;
}
@Override
public java.lang.String getLabel() {
return this.label;
}
@Override
public software.amazon.awscdk.core.Duration getPeriod() {
return this.period;
}
@Override
public java.lang.String getStatistic() {
return this.statistic;
}
@Override
public software.amazon.awscdk.services.cloudwatch.Unit getUnit() {
return this.unit;
}
@Override
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getColor() != null) {
obj.set("color", om.valueToTree(this.getColor()));
}
if (this.getDimensions() != null) {
obj.set("dimensions", om.valueToTree(this.getDimensions()));
}
if (this.getLabel() != null) {
obj.set("label", om.valueToTree(this.getLabel()));
}
if (this.getPeriod() != null) {
obj.set("period", om.valueToTree(this.getPeriod()));
}
if (this.getStatistic() != null) {
obj.set("statistic", om.valueToTree(this.getStatistic()));
}
if (this.getUnit() != null) {
obj.set("unit", om.valueToTree(this.getUnit()));
}
return obj;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
CommonMetricOptions.Jsii$Proxy that = (CommonMetricOptions.Jsii$Proxy) o;
if (this.color != null ? !this.color.equals(that.color) : that.color != null) return false;
if (this.dimensions != null ? !this.dimensions.equals(that.dimensions) : that.dimensions != null) return false;
if (this.label != null ? !this.label.equals(that.label) : that.label != null) return false;
if (this.period != null ? !this.period.equals(that.period) : that.period != null) return false;
if (this.statistic != null ? !this.statistic.equals(that.statistic) : that.statistic != null) return false;
return this.unit != null ? this.unit.equals(that.unit) : that.unit == null;
}
@Override
public int hashCode() {
int result = this.color != null ? this.color.hashCode() : 0;
result = 31 * result + (this.dimensions != null ? this.dimensions.hashCode() : 0);
result = 31 * result + (this.label != null ? this.label.hashCode() : 0);
result = 31 * result + (this.period != null ? this.period.hashCode() : 0);
result = 31 * result + (this.statistic != null ? this.statistic.hashCode() : 0);
result = 31 * result + (this.unit != null ? this.unit.hashCode() : 0);
return result;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy