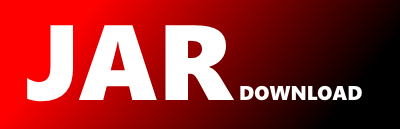
software.amazon.awscdk.services.cloudwatch.SingleValueWidgetProps Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cloudwatch Show documentation
Show all versions of cloudwatch Show documentation
The CDK Construct Library for AWS::CloudWatch
package software.amazon.awscdk.services.cloudwatch;
/**
* Properties for a SingleValueWidget.
*/
@javax.annotation.Generated(value = "jsii-pacmak/0.19.0 (build 7c562bc)", date = "2019-10-22T08:20:38.289Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface SingleValueWidgetProps extends software.amazon.jsii.JsiiSerializable, software.amazon.awscdk.services.cloudwatch.MetricWidgetProps {
/**
* Metrics to display.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
java.util.List getMetrics();
/**
* @return a {@link Builder} of {@link SingleValueWidgetProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link SingleValueWidgetProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder {
private java.util.List metrics;
private java.lang.Number height;
private java.lang.String region;
private java.lang.String title;
private java.lang.Number width;
/**
* Sets the value of Metrics
* @param metrics Metrics to display. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder metrics(java.util.List metrics) {
this.metrics = metrics;
return this;
}
/**
* Sets the value of Height
* @param height Height of the widget.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder height(java.lang.Number height) {
this.height = height;
return this;
}
/**
* Sets the value of Region
* @param region The region the metrics of this graph should be taken from.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder region(java.lang.String region) {
this.region = region;
return this;
}
/**
* Sets the value of Title
* @param title Title for the graph.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder title(java.lang.String title) {
this.title = title;
return this;
}
/**
* Sets the value of Width
* @param width Width of the widget, in a grid of 24 units wide.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder width(java.lang.Number width) {
this.width = width;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link SingleValueWidgetProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public SingleValueWidgetProps build() {
return new Jsii$Proxy(metrics, height, region, title, width);
}
}
/**
* An implementation for {@link SingleValueWidgetProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements SingleValueWidgetProps {
private final java.util.List metrics;
private final java.lang.Number height;
private final java.lang.String region;
private final java.lang.String title;
private final java.lang.Number width;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.setObjRef(objRef);
this.metrics = this.jsiiGet("metrics", java.util.List.class);
this.height = this.jsiiGet("height", java.lang.Number.class);
this.region = this.jsiiGet("region", java.lang.String.class);
this.title = this.jsiiGet("title", java.lang.String.class);
this.width = this.jsiiGet("width", java.lang.Number.class);
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
private Jsii$Proxy(java.util.List metrics, java.lang.Number height, java.lang.String region, java.lang.String title, java.lang.Number width) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.metrics = java.util.Objects.requireNonNull(metrics, "metrics is required");
this.height = height;
this.region = region;
this.title = title;
this.width = width;
}
@Override
public java.util.List getMetrics() {
return this.metrics;
}
@Override
public java.lang.Number getHeight() {
return this.height;
}
@Override
public java.lang.String getRegion() {
return this.region;
}
@Override
public java.lang.String getTitle() {
return this.title;
}
@Override
public java.lang.Number getWidth() {
return this.width;
}
@Override
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("metrics", om.valueToTree(this.getMetrics()));
if (this.getHeight() != null) {
obj.set("height", om.valueToTree(this.getHeight()));
}
if (this.getRegion() != null) {
obj.set("region", om.valueToTree(this.getRegion()));
}
if (this.getTitle() != null) {
obj.set("title", om.valueToTree(this.getTitle()));
}
if (this.getWidth() != null) {
obj.set("width", om.valueToTree(this.getWidth()));
}
return obj;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
SingleValueWidgetProps.Jsii$Proxy that = (SingleValueWidgetProps.Jsii$Proxy) o;
if (!metrics.equals(that.metrics)) return false;
if (this.height != null ? !this.height.equals(that.height) : that.height != null) return false;
if (this.region != null ? !this.region.equals(that.region) : that.region != null) return false;
if (this.title != null ? !this.title.equals(that.title) : that.title != null) return false;
return this.width != null ? this.width.equals(that.width) : that.width == null;
}
@Override
public int hashCode() {
int result = this.metrics.hashCode();
result = 31 * result + (this.height != null ? this.height.hashCode() : 0);
result = 31 * result + (this.region != null ? this.region.hashCode() : 0);
result = 31 * result + (this.title != null ? this.title.hashCode() : 0);
result = 31 * result + (this.width != null ? this.width.hashCode() : 0);
return result;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy