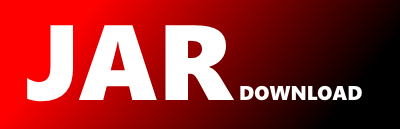
software.amazon.awscdk.services.cloudwatch.YAxisProps Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cloudwatch Show documentation
Show all versions of cloudwatch Show documentation
The CDK Construct Library for AWS::CloudWatch
package software.amazon.awscdk.services.cloudwatch;
/**
* Properties for a Y-Axis.
*/
@javax.annotation.Generated(value = "jsii-pacmak/0.19.0 (build 7c562bc)", date = "2019-10-22T08:20:38.290Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface YAxisProps extends software.amazon.jsii.JsiiSerializable {
/**
* The label.
*
* Default: No label
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
java.lang.String getLabel();
/**
* The max value.
*
* Default: No maximum value
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
java.lang.Number getMax();
/**
* The min value.
*
* Default: 0
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
java.lang.Number getMin();
/**
* Whether to show units.
*
* Default: true
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
java.lang.Boolean getShowUnits();
/**
* @return a {@link Builder} of {@link YAxisProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link YAxisProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder {
private java.lang.String label;
private java.lang.Number max;
private java.lang.Number min;
private java.lang.Boolean showUnits;
/**
* Sets the value of Label
* @param label The label.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder label(java.lang.String label) {
this.label = label;
return this;
}
/**
* Sets the value of Max
* @param max The max value.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder max(java.lang.Number max) {
this.max = max;
return this;
}
/**
* Sets the value of Min
* @param min The min value.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder min(java.lang.Number min) {
this.min = min;
return this;
}
/**
* Sets the value of ShowUnits
* @param showUnits Whether to show units.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder showUnits(java.lang.Boolean showUnits) {
this.showUnits = showUnits;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link YAxisProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public YAxisProps build() {
return new Jsii$Proxy(label, max, min, showUnits);
}
}
/**
* An implementation for {@link YAxisProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements YAxisProps {
private final java.lang.String label;
private final java.lang.Number max;
private final java.lang.Number min;
private final java.lang.Boolean showUnits;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.setObjRef(objRef);
this.label = this.jsiiGet("label", java.lang.String.class);
this.max = this.jsiiGet("max", java.lang.Number.class);
this.min = this.jsiiGet("min", java.lang.Number.class);
this.showUnits = this.jsiiGet("showUnits", java.lang.Boolean.class);
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
private Jsii$Proxy(java.lang.String label, java.lang.Number max, java.lang.Number min, java.lang.Boolean showUnits) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.label = label;
this.max = max;
this.min = min;
this.showUnits = showUnits;
}
@Override
public java.lang.String getLabel() {
return this.label;
}
@Override
public java.lang.Number getMax() {
return this.max;
}
@Override
public java.lang.Number getMin() {
return this.min;
}
@Override
public java.lang.Boolean getShowUnits() {
return this.showUnits;
}
@Override
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getLabel() != null) {
obj.set("label", om.valueToTree(this.getLabel()));
}
if (this.getMax() != null) {
obj.set("max", om.valueToTree(this.getMax()));
}
if (this.getMin() != null) {
obj.set("min", om.valueToTree(this.getMin()));
}
if (this.getShowUnits() != null) {
obj.set("showUnits", om.valueToTree(this.getShowUnits()));
}
return obj;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
YAxisProps.Jsii$Proxy that = (YAxisProps.Jsii$Proxy) o;
if (this.label != null ? !this.label.equals(that.label) : that.label != null) return false;
if (this.max != null ? !this.max.equals(that.max) : that.max != null) return false;
if (this.min != null ? !this.min.equals(that.min) : that.min != null) return false;
return this.showUnits != null ? this.showUnits.equals(that.showUnits) : that.showUnits == null;
}
@Override
public int hashCode() {
int result = this.label != null ? this.label.hashCode() : 0;
result = 31 * result + (this.max != null ? this.max.hashCode() : 0);
result = 31 * result + (this.min != null ? this.min.hashCode() : 0);
result = 31 * result + (this.showUnits != null ? this.showUnits.hashCode() : 0);
return result;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy