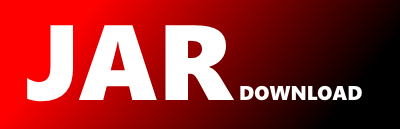
software.amazon.awscdk.services.codebuild.ProjectProps Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of codebuild Show documentation
Show all versions of codebuild Show documentation
CDK Constructs for AWS CodeBuild
package software.amazon.awscdk.services.codebuild;
@javax.annotation.Generated(value = "jsii-pacmak/0.7.11 (build 44c3b9b)", date = "2018-12-04T12:25:05.449Z")
public interface ProjectProps extends software.amazon.jsii.JsiiSerializable, software.amazon.awscdk.services.codebuild.CommonProjectProps {
/**
* Defines where build artifacts will be stored.
* Could be: PipelineBuildArtifacts, NoBuildArtifacts and S3BucketBuildArtifacts.
* @default NoBuildArtifacts
*/
software.amazon.awscdk.services.codebuild.BuildArtifacts getArtifacts();
/**
* Defines where build artifacts will be stored.
* Could be: PipelineBuildArtifacts, NoBuildArtifacts and S3BucketBuildArtifacts.
* @default NoBuildArtifacts
*/
void setArtifacts(final software.amazon.awscdk.services.codebuild.BuildArtifacts value);
/**
* The secondary artifacts for the Project.
* Can also be added after the Project has been created by using the {@link Project#addSecondaryArtifact} method.
* @default []
* @see https://docs.aws.amazon.com/codebuild/latest/userguide/sample-multi-in-out.html
*/
java.util.List getSecondaryArtifacts();
/**
* The secondary artifacts for the Project.
* Can also be added after the Project has been created by using the {@link Project#addSecondaryArtifact} method.
* @default []
* @see https://docs.aws.amazon.com/codebuild/latest/userguide/sample-multi-in-out.html
*/
void setSecondaryArtifacts(final java.util.List value);
/**
* The secondary sources for the Project.
* Can be also added after the Project has been created by using the {@link Project#addSecondarySource} method.
* @default []
* @see https://docs.aws.amazon.com/codebuild/latest/userguide/sample-multi-in-out.html
*/
java.util.List getSecondarySources();
/**
* The secondary sources for the Project.
* Can be also added after the Project has been created by using the {@link Project#addSecondarySource} method.
* @default []
* @see https://docs.aws.amazon.com/codebuild/latest/userguide/sample-multi-in-out.html
*/
void setSecondarySources(final java.util.List value);
/**
* The source of the build.
* *Note*: if {@link NoSource} is given as the source,
* then you need to provide an explicit `buildSpec`.
* @default NoSource
*/
software.amazon.awscdk.services.codebuild.BuildSource getSource();
/**
* The source of the build.
* *Note*: if {@link NoSource} is given as the source,
* then you need to provide an explicit `buildSpec`.
* @default NoSource
*/
void setSource(final software.amazon.awscdk.services.codebuild.BuildSource value);
/**
* @return a {@link Builder} of {@link ProjectProps}
*/
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link ProjectProps}
*/
final class Builder {
@javax.annotation.Nullable
private software.amazon.awscdk.services.codebuild.BuildArtifacts _artifacts;
@javax.annotation.Nullable
private java.util.List _secondaryArtifacts;
@javax.annotation.Nullable
private java.util.List _secondarySources;
@javax.annotation.Nullable
private software.amazon.awscdk.services.codebuild.BuildSource _source;
@javax.annotation.Nullable
private java.lang.Boolean _badge;
@javax.annotation.Nullable
private software.amazon.awscdk.assets.Asset _buildScriptAsset;
@javax.annotation.Nullable
private java.lang.String _buildScriptAssetEntrypoint;
@javax.annotation.Nullable
private java.lang.Object _buildSpec;
@javax.annotation.Nullable
private software.amazon.awscdk.services.s3.BucketRef _cacheBucket;
@javax.annotation.Nullable
private java.lang.String _cacheDir;
@javax.annotation.Nullable
private java.lang.String _description;
@javax.annotation.Nullable
private software.amazon.awscdk.services.kms.EncryptionKeyRef _encryptionKey;
@javax.annotation.Nullable
private software.amazon.awscdk.services.codebuild.BuildEnvironment _environment;
@javax.annotation.Nullable
private java.util.Map _environmentVariables;
@javax.annotation.Nullable
private java.lang.String _projectName;
@javax.annotation.Nullable
private software.amazon.awscdk.services.iam.Role _role;
@javax.annotation.Nullable
private java.lang.Number _timeout;
/**
* Sets the value of Artifacts
* @param value Defines where build artifacts will be stored.
Could be: PipelineBuildArtifacts, NoBuildArtifacts and S3BucketBuildArtifacts.
* @return {@code this}
*/
public Builder withArtifacts(@javax.annotation.Nullable final software.amazon.awscdk.services.codebuild.BuildArtifacts value) {
this._artifacts = value;
return this;
}
/**
* Sets the value of SecondaryArtifacts
* @param value The secondary artifacts for the Project.
Can also be added after the Project has been created by using the {@link Project#addSecondaryArtifact} method.
* @return {@code this}
*/
public Builder withSecondaryArtifacts(@javax.annotation.Nullable final java.util.List value) {
this._secondaryArtifacts = value;
return this;
}
/**
* Sets the value of SecondarySources
* @param value The secondary sources for the Project.
Can be also added after the Project has been created by using the {@link Project#addSecondarySource} method.
* @return {@code this}
*/
public Builder withSecondarySources(@javax.annotation.Nullable final java.util.List value) {
this._secondarySources = value;
return this;
}
/**
* Sets the value of Source
* @param value The source of the build.
*Note*: if {@link NoSource} is given as the source,
then you need to provide an explicit `buildSpec`.
* @return {@code this}
*/
public Builder withSource(@javax.annotation.Nullable final software.amazon.awscdk.services.codebuild.BuildSource value) {
this._source = value;
return this;
}
/**
* Sets the value of Badge
* @param value Indicates whether AWS CodeBuild generates a publicly accessible URL for
your project's build badge. For more information, see Build Badges Sample
in the AWS CodeBuild User Guide.
* @return {@code this}
*/
public Builder withBadge(@javax.annotation.Nullable final java.lang.Boolean value) {
this._badge = value;
return this;
}
/**
* Sets the value of BuildScriptAsset
* @param value Run a script from an asset as build script
If supplied together with buildSpec, the asset script will be run
_after_ the existing commands in buildspec.
This feature can also be used without a source, to simply run an
arbitrary script in a serverless way.
* @return {@code this}
*/
public Builder withBuildScriptAsset(@javax.annotation.Nullable final software.amazon.awscdk.assets.Asset value) {
this._buildScriptAsset = value;
return this;
}
/**
* Sets the value of BuildScriptAssetEntrypoint
* @param value The script in the asset to run.
* @return {@code this}
*/
public Builder withBuildScriptAssetEntrypoint(@javax.annotation.Nullable final java.lang.String value) {
this._buildScriptAssetEntrypoint = value;
return this;
}
/**
* Sets the value of BuildSpec
* @param value Filename or contents of buildspec in JSON format.
* @return {@code this}
*/
public Builder withBuildSpec(@javax.annotation.Nullable final java.lang.Object value) {
this._buildSpec = value;
return this;
}
/**
* Sets the value of CacheBucket
* @param value Bucket to store cached source artifacts
If not specified, source artifacts will not be cached.
* @return {@code this}
*/
public Builder withCacheBucket(@javax.annotation.Nullable final software.amazon.awscdk.services.s3.BucketRef value) {
this._cacheBucket = value;
return this;
}
/**
* Sets the value of CacheDir
* @param value Subdirectory to store cached artifacts
* @return {@code this}
*/
public Builder withCacheDir(@javax.annotation.Nullable final java.lang.String value) {
this._cacheDir = value;
return this;
}
/**
* Sets the value of Description
* @param value A description of the project. Use the description to identify the purpose
of the project.
* @return {@code this}
*/
public Builder withDescription(@javax.annotation.Nullable final java.lang.String value) {
this._description = value;
return this;
}
/**
* Sets the value of EncryptionKey
* @param value Encryption key to use to read and write artifacts
If not specified, a role will be created.
* @return {@code this}
*/
public Builder withEncryptionKey(@javax.annotation.Nullable final software.amazon.awscdk.services.kms.EncryptionKeyRef value) {
this._encryptionKey = value;
return this;
}
/**
* Sets the value of Environment
* @param value Build environment to use for the build.
* @return {@code this}
*/
public Builder withEnvironment(@javax.annotation.Nullable final software.amazon.awscdk.services.codebuild.BuildEnvironment value) {
this._environment = value;
return this;
}
/**
* Sets the value of EnvironmentVariables
* @param value Additional environment variables to add to the build environment.
* @return {@code this}
*/
public Builder withEnvironmentVariables(@javax.annotation.Nullable final java.util.Map value) {
this._environmentVariables = value;
return this;
}
/**
* Sets the value of ProjectName
* @param value The physical, human-readable name of the CodeBuild Project.
* @return {@code this}
*/
public Builder withProjectName(@javax.annotation.Nullable final java.lang.String value) {
this._projectName = value;
return this;
}
/**
* Sets the value of Role
* @param value Service Role to assume while running the build.
If not specified, a role will be created.
* @return {@code this}
*/
public Builder withRole(@javax.annotation.Nullable final software.amazon.awscdk.services.iam.Role value) {
this._role = value;
return this;
}
/**
* Sets the value of Timeout
* @param value The number of minutes after which AWS CodeBuild stops the build if it's
not complete. For valid values, see the timeoutInMinutes field in the AWS
CodeBuild User Guide.
* @return {@code this}
*/
public Builder withTimeout(@javax.annotation.Nullable final java.lang.Number value) {
this._timeout = value;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link ProjectProps}
* @throws NullPointerException if any required attribute was not provided
*/
public ProjectProps build() {
return new ProjectProps() {
@javax.annotation.Nullable
private software.amazon.awscdk.services.codebuild.BuildArtifacts $artifacts = _artifacts;
@javax.annotation.Nullable
private java.util.List $secondaryArtifacts = _secondaryArtifacts;
@javax.annotation.Nullable
private java.util.List $secondarySources = _secondarySources;
@javax.annotation.Nullable
private software.amazon.awscdk.services.codebuild.BuildSource $source = _source;
@javax.annotation.Nullable
private java.lang.Boolean $badge = _badge;
@javax.annotation.Nullable
private software.amazon.awscdk.assets.Asset $buildScriptAsset = _buildScriptAsset;
@javax.annotation.Nullable
private java.lang.String $buildScriptAssetEntrypoint = _buildScriptAssetEntrypoint;
@javax.annotation.Nullable
private java.lang.Object $buildSpec = _buildSpec;
@javax.annotation.Nullable
private software.amazon.awscdk.services.s3.BucketRef $cacheBucket = _cacheBucket;
@javax.annotation.Nullable
private java.lang.String $cacheDir = _cacheDir;
@javax.annotation.Nullable
private java.lang.String $description = _description;
@javax.annotation.Nullable
private software.amazon.awscdk.services.kms.EncryptionKeyRef $encryptionKey = _encryptionKey;
@javax.annotation.Nullable
private software.amazon.awscdk.services.codebuild.BuildEnvironment $environment = _environment;
@javax.annotation.Nullable
private java.util.Map $environmentVariables = _environmentVariables;
@javax.annotation.Nullable
private java.lang.String $projectName = _projectName;
@javax.annotation.Nullable
private software.amazon.awscdk.services.iam.Role $role = _role;
@javax.annotation.Nullable
private java.lang.Number $timeout = _timeout;
@Override
public software.amazon.awscdk.services.codebuild.BuildArtifacts getArtifacts() {
return this.$artifacts;
}
@Override
public void setArtifacts(@javax.annotation.Nullable final software.amazon.awscdk.services.codebuild.BuildArtifacts value) {
this.$artifacts = value;
}
@Override
public java.util.List getSecondaryArtifacts() {
return this.$secondaryArtifacts;
}
@Override
public void setSecondaryArtifacts(@javax.annotation.Nullable final java.util.List value) {
this.$secondaryArtifacts = value;
}
@Override
public java.util.List getSecondarySources() {
return this.$secondarySources;
}
@Override
public void setSecondarySources(@javax.annotation.Nullable final java.util.List value) {
this.$secondarySources = value;
}
@Override
public software.amazon.awscdk.services.codebuild.BuildSource getSource() {
return this.$source;
}
@Override
public void setSource(@javax.annotation.Nullable final software.amazon.awscdk.services.codebuild.BuildSource value) {
this.$source = value;
}
@Override
public java.lang.Boolean getBadge() {
return this.$badge;
}
@Override
public void setBadge(@javax.annotation.Nullable final java.lang.Boolean value) {
this.$badge = value;
}
@Override
public software.amazon.awscdk.assets.Asset getBuildScriptAsset() {
return this.$buildScriptAsset;
}
@Override
public void setBuildScriptAsset(@javax.annotation.Nullable final software.amazon.awscdk.assets.Asset value) {
this.$buildScriptAsset = value;
}
@Override
public java.lang.String getBuildScriptAssetEntrypoint() {
return this.$buildScriptAssetEntrypoint;
}
@Override
public void setBuildScriptAssetEntrypoint(@javax.annotation.Nullable final java.lang.String value) {
this.$buildScriptAssetEntrypoint = value;
}
@Override
public java.lang.Object getBuildSpec() {
return this.$buildSpec;
}
@Override
public void setBuildSpec(@javax.annotation.Nullable final java.lang.Object value) {
this.$buildSpec = value;
}
@Override
public software.amazon.awscdk.services.s3.BucketRef getCacheBucket() {
return this.$cacheBucket;
}
@Override
public void setCacheBucket(@javax.annotation.Nullable final software.amazon.awscdk.services.s3.BucketRef value) {
this.$cacheBucket = value;
}
@Override
public java.lang.String getCacheDir() {
return this.$cacheDir;
}
@Override
public void setCacheDir(@javax.annotation.Nullable final java.lang.String value) {
this.$cacheDir = value;
}
@Override
public java.lang.String getDescription() {
return this.$description;
}
@Override
public void setDescription(@javax.annotation.Nullable final java.lang.String value) {
this.$description = value;
}
@Override
public software.amazon.awscdk.services.kms.EncryptionKeyRef getEncryptionKey() {
return this.$encryptionKey;
}
@Override
public void setEncryptionKey(@javax.annotation.Nullable final software.amazon.awscdk.services.kms.EncryptionKeyRef value) {
this.$encryptionKey = value;
}
@Override
public software.amazon.awscdk.services.codebuild.BuildEnvironment getEnvironment() {
return this.$environment;
}
@Override
public void setEnvironment(@javax.annotation.Nullable final software.amazon.awscdk.services.codebuild.BuildEnvironment value) {
this.$environment = value;
}
@Override
public java.util.Map getEnvironmentVariables() {
return this.$environmentVariables;
}
@Override
public void setEnvironmentVariables(@javax.annotation.Nullable final java.util.Map value) {
this.$environmentVariables = value;
}
@Override
public java.lang.String getProjectName() {
return this.$projectName;
}
@Override
public void setProjectName(@javax.annotation.Nullable final java.lang.String value) {
this.$projectName = value;
}
@Override
public software.amazon.awscdk.services.iam.Role getRole() {
return this.$role;
}
@Override
public void setRole(@javax.annotation.Nullable final software.amazon.awscdk.services.iam.Role value) {
this.$role = value;
}
@Override
public java.lang.Number getTimeout() {
return this.$timeout;
}
@Override
public void setTimeout(@javax.annotation.Nullable final java.lang.Number value) {
this.$timeout = value;
}
};
}
}
/**
* A proxy class which represents a concrete javascript instance of this type.
*/
final static class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements software.amazon.awscdk.services.codebuild.ProjectProps {
protected Jsii$Proxy(final software.amazon.jsii.JsiiObject.InitializationMode mode) {
super(mode);
}
/**
* Defines where build artifacts will be stored.
* Could be: PipelineBuildArtifacts, NoBuildArtifacts and S3BucketBuildArtifacts.
* @default NoBuildArtifacts
*/
@Override
@javax.annotation.Nullable
public software.amazon.awscdk.services.codebuild.BuildArtifacts getArtifacts() {
return this.jsiiGet("artifacts", software.amazon.awscdk.services.codebuild.BuildArtifacts.class);
}
/**
* Defines where build artifacts will be stored.
* Could be: PipelineBuildArtifacts, NoBuildArtifacts and S3BucketBuildArtifacts.
* @default NoBuildArtifacts
*/
@Override
public void setArtifacts(@javax.annotation.Nullable final software.amazon.awscdk.services.codebuild.BuildArtifacts value) {
this.jsiiSet("artifacts", value);
}
/**
* The secondary artifacts for the Project.
* Can also be added after the Project has been created by using the {@link Project#addSecondaryArtifact} method.
* @default []
* @see https://docs.aws.amazon.com/codebuild/latest/userguide/sample-multi-in-out.html
*/
@Override
@javax.annotation.Nullable
public java.util.List getSecondaryArtifacts() {
return this.jsiiGet("secondaryArtifacts", java.util.List.class);
}
/**
* The secondary artifacts for the Project.
* Can also be added after the Project has been created by using the {@link Project#addSecondaryArtifact} method.
* @default []
* @see https://docs.aws.amazon.com/codebuild/latest/userguide/sample-multi-in-out.html
*/
@Override
public void setSecondaryArtifacts(@javax.annotation.Nullable final java.util.List value) {
this.jsiiSet("secondaryArtifacts", value);
}
/**
* The secondary sources for the Project.
* Can be also added after the Project has been created by using the {@link Project#addSecondarySource} method.
* @default []
* @see https://docs.aws.amazon.com/codebuild/latest/userguide/sample-multi-in-out.html
*/
@Override
@javax.annotation.Nullable
public java.util.List getSecondarySources() {
return this.jsiiGet("secondarySources", java.util.List.class);
}
/**
* The secondary sources for the Project.
* Can be also added after the Project has been created by using the {@link Project#addSecondarySource} method.
* @default []
* @see https://docs.aws.amazon.com/codebuild/latest/userguide/sample-multi-in-out.html
*/
@Override
public void setSecondarySources(@javax.annotation.Nullable final java.util.List value) {
this.jsiiSet("secondarySources", value);
}
/**
* The source of the build.
* *Note*: if {@link NoSource} is given as the source,
* then you need to provide an explicit `buildSpec`.
* @default NoSource
*/
@Override
@javax.annotation.Nullable
public software.amazon.awscdk.services.codebuild.BuildSource getSource() {
return this.jsiiGet("source", software.amazon.awscdk.services.codebuild.BuildSource.class);
}
/**
* The source of the build.
* *Note*: if {@link NoSource} is given as the source,
* then you need to provide an explicit `buildSpec`.
* @default NoSource
*/
@Override
public void setSource(@javax.annotation.Nullable final software.amazon.awscdk.services.codebuild.BuildSource value) {
this.jsiiSet("source", value);
}
/**
* Indicates whether AWS CodeBuild generates a publicly accessible URL for
* your project's build badge. For more information, see Build Badges Sample
* in the AWS CodeBuild User Guide.
*/
@Override
@javax.annotation.Nullable
public java.lang.Boolean getBadge() {
return this.jsiiGet("badge", java.lang.Boolean.class);
}
/**
* Indicates whether AWS CodeBuild generates a publicly accessible URL for
* your project's build badge. For more information, see Build Badges Sample
* in the AWS CodeBuild User Guide.
*/
@Override
public void setBadge(@javax.annotation.Nullable final java.lang.Boolean value) {
this.jsiiSet("badge", value);
}
/**
* Run a script from an asset as build script
*
* If supplied together with buildSpec, the asset script will be run
* _after_ the existing commands in buildspec.
*
* This feature can also be used without a source, to simply run an
* arbitrary script in a serverless way.
* @default No asset build script
*/
@Override
@javax.annotation.Nullable
public software.amazon.awscdk.assets.Asset getBuildScriptAsset() {
return this.jsiiGet("buildScriptAsset", software.amazon.awscdk.assets.Asset.class);
}
/**
* Run a script from an asset as build script
*
* If supplied together with buildSpec, the asset script will be run
* _after_ the existing commands in buildspec.
*
* This feature can also be used without a source, to simply run an
* arbitrary script in a serverless way.
* @default No asset build script
*/
@Override
public void setBuildScriptAsset(@javax.annotation.Nullable final software.amazon.awscdk.assets.Asset value) {
this.jsiiSet("buildScriptAsset", value);
}
/**
* The script in the asset to run.
* @default build.sh
*/
@Override
@javax.annotation.Nullable
public java.lang.String getBuildScriptAssetEntrypoint() {
return this.jsiiGet("buildScriptAssetEntrypoint", java.lang.String.class);
}
/**
* The script in the asset to run.
* @default build.sh
*/
@Override
public void setBuildScriptAssetEntrypoint(@javax.annotation.Nullable final java.lang.String value) {
this.jsiiSet("buildScriptAssetEntrypoint", value);
}
/**
* Filename or contents of buildspec in JSON format.
* @see https://docs.aws.amazon.com/codebuild/latest/userguide/build-spec-ref.html#build-spec-ref-example
*/
@Override
@javax.annotation.Nullable
public java.lang.Object getBuildSpec() {
return this.jsiiGet("buildSpec", java.lang.Object.class);
}
/**
* Filename or contents of buildspec in JSON format.
* @see https://docs.aws.amazon.com/codebuild/latest/userguide/build-spec-ref.html#build-spec-ref-example
*/
@Override
public void setBuildSpec(@javax.annotation.Nullable final java.lang.Object value) {
this.jsiiSet("buildSpec", value);
}
/**
* Bucket to store cached source artifacts
* If not specified, source artifacts will not be cached.
*/
@Override
@javax.annotation.Nullable
public software.amazon.awscdk.services.s3.BucketRef getCacheBucket() {
return this.jsiiGet("cacheBucket", software.amazon.awscdk.services.s3.BucketRef.class);
}
/**
* Bucket to store cached source artifacts
* If not specified, source artifacts will not be cached.
*/
@Override
public void setCacheBucket(@javax.annotation.Nullable final software.amazon.awscdk.services.s3.BucketRef value) {
this.jsiiSet("cacheBucket", value);
}
/**
* Subdirectory to store cached artifacts
*/
@Override
@javax.annotation.Nullable
public java.lang.String getCacheDir() {
return this.jsiiGet("cacheDir", java.lang.String.class);
}
/**
* Subdirectory to store cached artifacts
*/
@Override
public void setCacheDir(@javax.annotation.Nullable final java.lang.String value) {
this.jsiiSet("cacheDir", value);
}
/**
* A description of the project. Use the description to identify the purpose
* of the project.
*/
@Override
@javax.annotation.Nullable
public java.lang.String getDescription() {
return this.jsiiGet("description", java.lang.String.class);
}
/**
* A description of the project. Use the description to identify the purpose
* of the project.
*/
@Override
public void setDescription(@javax.annotation.Nullable final java.lang.String value) {
this.jsiiSet("description", value);
}
/**
* Encryption key to use to read and write artifacts
* If not specified, a role will be created.
*/
@Override
@javax.annotation.Nullable
public software.amazon.awscdk.services.kms.EncryptionKeyRef getEncryptionKey() {
return this.jsiiGet("encryptionKey", software.amazon.awscdk.services.kms.EncryptionKeyRef.class);
}
/**
* Encryption key to use to read and write artifacts
* If not specified, a role will be created.
*/
@Override
public void setEncryptionKey(@javax.annotation.Nullable final software.amazon.awscdk.services.kms.EncryptionKeyRef value) {
this.jsiiSet("encryptionKey", value);
}
/**
* Build environment to use for the build.
*/
@Override
@javax.annotation.Nullable
public software.amazon.awscdk.services.codebuild.BuildEnvironment getEnvironment() {
return this.jsiiGet("environment", software.amazon.awscdk.services.codebuild.BuildEnvironment.class);
}
/**
* Build environment to use for the build.
*/
@Override
public void setEnvironment(@javax.annotation.Nullable final software.amazon.awscdk.services.codebuild.BuildEnvironment value) {
this.jsiiSet("environment", value);
}
/**
* Additional environment variables to add to the build environment.
*/
@Override
@javax.annotation.Nullable
public java.util.Map getEnvironmentVariables() {
return this.jsiiGet("environmentVariables", java.util.Map.class);
}
/**
* Additional environment variables to add to the build environment.
*/
@Override
public void setEnvironmentVariables(@javax.annotation.Nullable final java.util.Map value) {
this.jsiiSet("environmentVariables", value);
}
/**
* The physical, human-readable name of the CodeBuild Project.
*/
@Override
@javax.annotation.Nullable
public java.lang.String getProjectName() {
return this.jsiiGet("projectName", java.lang.String.class);
}
/**
* The physical, human-readable name of the CodeBuild Project.
*/
@Override
public void setProjectName(@javax.annotation.Nullable final java.lang.String value) {
this.jsiiSet("projectName", value);
}
/**
* Service Role to assume while running the build.
* If not specified, a role will be created.
*/
@Override
@javax.annotation.Nullable
public software.amazon.awscdk.services.iam.Role getRole() {
return this.jsiiGet("role", software.amazon.awscdk.services.iam.Role.class);
}
/**
* Service Role to assume while running the build.
* If not specified, a role will be created.
*/
@Override
public void setRole(@javax.annotation.Nullable final software.amazon.awscdk.services.iam.Role value) {
this.jsiiSet("role", value);
}
/**
* The number of minutes after which AWS CodeBuild stops the build if it's
* not complete. For valid values, see the timeoutInMinutes field in the AWS
* CodeBuild User Guide.
*/
@Override
@javax.annotation.Nullable
public java.lang.Number getTimeout() {
return this.jsiiGet("timeout", java.lang.Number.class);
}
/**
* The number of minutes after which AWS CodeBuild stops the build if it's
* not complete. For valid values, see the timeoutInMinutes field in the AWS
* CodeBuild User Guide.
*/
@Override
public void setTimeout(@javax.annotation.Nullable final java.lang.Number value) {
this.jsiiSet("timeout", value);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy