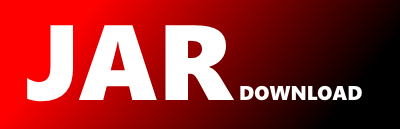
software.amazon.awscdk.services.codebuild.CommonProjectProps Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of codebuild Show documentation
Show all versions of codebuild Show documentation
CDK Constructs for AWS CodeBuild
package software.amazon.awscdk.services.codebuild;
@javax.annotation.Generated(value = "jsii-pacmak/0.10.5 (build 46bc9b0)", date = "2019-05-06T20:55:05.110Z")
public interface CommonProjectProps extends software.amazon.jsii.JsiiSerializable {
/**
* Whether to allow the CodeBuild to send all network traffic.
*
* If set to false, you must individually add traffic rules to allow the
* CodeBuild project to connect to network targets.
*
* Only used if 'vpc' is supplied.
*
* Default: true
*/
java.lang.Boolean getAllowAllOutbound();
/**
* Indicates whether AWS CodeBuild generates a publicly accessible URL for your project's build badge.
*
* For more information, see Build Badges Sample
* in the AWS CodeBuild User Guide.
*/
java.lang.Boolean getBadge();
/**
* Run a script from an asset as build script.
*
* If supplied together with buildSpec, the asset script will be run
* _after_ the existing commands in buildspec.
*
* This feature can also be used without a source, to simply run an
* arbitrary script in a serverless way.
*
* Default: No asset build script
*/
software.amazon.awscdk.assets.Asset getBuildScriptAsset();
/**
* The script in the asset to run.
*
* Default: build.sh
*/
java.lang.String getBuildScriptAssetEntrypoint();
/**
* Filename or contents of buildspec in JSON format.
*
* @see https://docs.aws.amazon.com/codebuild/latest/userguide/build-spec-ref.html#build-spec-ref-example
*/
java.lang.Object getBuildSpec();
/**
* Bucket to store cached source artifacts If not specified, source artifacts will not be cached.
*/
software.amazon.awscdk.services.s3.IBucket getCacheBucket();
/**
* Subdirectory to store cached artifacts.
*/
java.lang.String getCacheDir();
/**
* A description of the project.
*
* Use the description to identify the purpose
* of the project.
*/
java.lang.String getDescription();
/**
* Encryption key to use to read and write artifacts If not specified, a role will be created.
*/
software.amazon.awscdk.services.kms.IEncryptionKey getEncryptionKey();
/**
* Build environment to use for the build.
*/
software.amazon.awscdk.services.codebuild.BuildEnvironment getEnvironment();
/**
* Additional environment variables to add to the build environment.
*/
java.util.Map getEnvironmentVariables();
/**
* The physical, human-readable name of the CodeBuild Project.
*/
java.lang.String getProjectName();
/**
* Service Role to assume while running the build. If not specified, a role will be created.
*/
software.amazon.awscdk.services.iam.IRole getRole();
/**
* What security group to associate with the codebuild project's network interfaces. If no security group is identified, one will be created automatically.
*
* Only used if 'vpc' is supplied.
*/
java.util.List getSecurityGroups();
/**
* Where to place the network interfaces within the VPC.
*
* Only used if 'vpc' is supplied.
*
* Default: All private subnets
*/
software.amazon.awscdk.services.ec2.SubnetSelection getSubnetSelection();
/**
* The number of minutes after which AWS CodeBuild stops the build if it's not complete.
*
* For valid values, see the timeoutInMinutes field in the AWS
* CodeBuild User Guide.
*/
java.lang.Number getTimeout();
/**
* VPC network to place codebuild network interfaces.
*
* Specify this if the codebuild project needs to access resources in a VPC.
*/
software.amazon.awscdk.services.ec2.IVpcNetwork getVpc();
/**
* @return a {@link Builder} of {@link CommonProjectProps}
*/
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link CommonProjectProps}
*/
final class Builder {
@javax.annotation.Nullable
private java.lang.Boolean _allowAllOutbound;
@javax.annotation.Nullable
private java.lang.Boolean _badge;
@javax.annotation.Nullable
private software.amazon.awscdk.assets.Asset _buildScriptAsset;
@javax.annotation.Nullable
private java.lang.String _buildScriptAssetEntrypoint;
@javax.annotation.Nullable
private java.lang.Object _buildSpec;
@javax.annotation.Nullable
private software.amazon.awscdk.services.s3.IBucket _cacheBucket;
@javax.annotation.Nullable
private java.lang.String _cacheDir;
@javax.annotation.Nullable
private java.lang.String _description;
@javax.annotation.Nullable
private software.amazon.awscdk.services.kms.IEncryptionKey _encryptionKey;
@javax.annotation.Nullable
private software.amazon.awscdk.services.codebuild.BuildEnvironment _environment;
@javax.annotation.Nullable
private java.util.Map _environmentVariables;
@javax.annotation.Nullable
private java.lang.String _projectName;
@javax.annotation.Nullable
private software.amazon.awscdk.services.iam.IRole _role;
@javax.annotation.Nullable
private java.util.List _securityGroups;
@javax.annotation.Nullable
private software.amazon.awscdk.services.ec2.SubnetSelection _subnetSelection;
@javax.annotation.Nullable
private java.lang.Number _timeout;
@javax.annotation.Nullable
private software.amazon.awscdk.services.ec2.IVpcNetwork _vpc;
/**
* Sets the value of AllowAllOutbound
* @param value Whether to allow the CodeBuild to send all network traffic.
* @return {@code this}
*/
public Builder withAllowAllOutbound(@javax.annotation.Nullable final java.lang.Boolean value) {
this._allowAllOutbound = value;
return this;
}
/**
* Sets the value of Badge
* @param value Indicates whether AWS CodeBuild generates a publicly accessible URL for your project's build badge.
* @return {@code this}
*/
public Builder withBadge(@javax.annotation.Nullable final java.lang.Boolean value) {
this._badge = value;
return this;
}
/**
* Sets the value of BuildScriptAsset
* @param value Run a script from an asset as build script.
* @return {@code this}
*/
public Builder withBuildScriptAsset(@javax.annotation.Nullable final software.amazon.awscdk.assets.Asset value) {
this._buildScriptAsset = value;
return this;
}
/**
* Sets the value of BuildScriptAssetEntrypoint
* @param value The script in the asset to run.
* @return {@code this}
*/
public Builder withBuildScriptAssetEntrypoint(@javax.annotation.Nullable final java.lang.String value) {
this._buildScriptAssetEntrypoint = value;
return this;
}
/**
* Sets the value of BuildSpec
* @param value Filename or contents of buildspec in JSON format.
* @return {@code this}
*/
public Builder withBuildSpec(@javax.annotation.Nullable final java.lang.Object value) {
this._buildSpec = value;
return this;
}
/**
* Sets the value of CacheBucket
* @param value Bucket to store cached source artifacts If not specified, source artifacts will not be cached.
* @return {@code this}
*/
public Builder withCacheBucket(@javax.annotation.Nullable final software.amazon.awscdk.services.s3.IBucket value) {
this._cacheBucket = value;
return this;
}
/**
* Sets the value of CacheDir
* @param value Subdirectory to store cached artifacts.
* @return {@code this}
*/
public Builder withCacheDir(@javax.annotation.Nullable final java.lang.String value) {
this._cacheDir = value;
return this;
}
/**
* Sets the value of Description
* @param value A description of the project.
* @return {@code this}
*/
public Builder withDescription(@javax.annotation.Nullable final java.lang.String value) {
this._description = value;
return this;
}
/**
* Sets the value of EncryptionKey
* @param value Encryption key to use to read and write artifacts If not specified, a role will be created.
* @return {@code this}
*/
public Builder withEncryptionKey(@javax.annotation.Nullable final software.amazon.awscdk.services.kms.IEncryptionKey value) {
this._encryptionKey = value;
return this;
}
/**
* Sets the value of Environment
* @param value Build environment to use for the build.
* @return {@code this}
*/
public Builder withEnvironment(@javax.annotation.Nullable final software.amazon.awscdk.services.codebuild.BuildEnvironment value) {
this._environment = value;
return this;
}
/**
* Sets the value of EnvironmentVariables
* @param value Additional environment variables to add to the build environment.
* @return {@code this}
*/
public Builder withEnvironmentVariables(@javax.annotation.Nullable final java.util.Map value) {
this._environmentVariables = value;
return this;
}
/**
* Sets the value of ProjectName
* @param value The physical, human-readable name of the CodeBuild Project.
* @return {@code this}
*/
public Builder withProjectName(@javax.annotation.Nullable final java.lang.String value) {
this._projectName = value;
return this;
}
/**
* Sets the value of Role
* @param value Service Role to assume while running the build. If not specified, a role will be created.
* @return {@code this}
*/
public Builder withRole(@javax.annotation.Nullable final software.amazon.awscdk.services.iam.IRole value) {
this._role = value;
return this;
}
/**
* Sets the value of SecurityGroups
* @param value What security group to associate with the codebuild project's network interfaces. If no security group is identified, one will be created automatically.
* @return {@code this}
*/
public Builder withSecurityGroups(@javax.annotation.Nullable final java.util.List value) {
this._securityGroups = value;
return this;
}
/**
* Sets the value of SubnetSelection
* @param value Where to place the network interfaces within the VPC.
* @return {@code this}
*/
public Builder withSubnetSelection(@javax.annotation.Nullable final software.amazon.awscdk.services.ec2.SubnetSelection value) {
this._subnetSelection = value;
return this;
}
/**
* Sets the value of Timeout
* @param value The number of minutes after which AWS CodeBuild stops the build if it's not complete.
* @return {@code this}
*/
public Builder withTimeout(@javax.annotation.Nullable final java.lang.Number value) {
this._timeout = value;
return this;
}
/**
* Sets the value of Vpc
* @param value VPC network to place codebuild network interfaces.
* @return {@code this}
*/
public Builder withVpc(@javax.annotation.Nullable final software.amazon.awscdk.services.ec2.IVpcNetwork value) {
this._vpc = value;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link CommonProjectProps}
* @throws NullPointerException if any required attribute was not provided
*/
public CommonProjectProps build() {
return new CommonProjectProps() {
@javax.annotation.Nullable
private final java.lang.Boolean $allowAllOutbound = _allowAllOutbound;
@javax.annotation.Nullable
private final java.lang.Boolean $badge = _badge;
@javax.annotation.Nullable
private final software.amazon.awscdk.assets.Asset $buildScriptAsset = _buildScriptAsset;
@javax.annotation.Nullable
private final java.lang.String $buildScriptAssetEntrypoint = _buildScriptAssetEntrypoint;
@javax.annotation.Nullable
private final java.lang.Object $buildSpec = _buildSpec;
@javax.annotation.Nullable
private final software.amazon.awscdk.services.s3.IBucket $cacheBucket = _cacheBucket;
@javax.annotation.Nullable
private final java.lang.String $cacheDir = _cacheDir;
@javax.annotation.Nullable
private final java.lang.String $description = _description;
@javax.annotation.Nullable
private final software.amazon.awscdk.services.kms.IEncryptionKey $encryptionKey = _encryptionKey;
@javax.annotation.Nullable
private final software.amazon.awscdk.services.codebuild.BuildEnvironment $environment = _environment;
@javax.annotation.Nullable
private final java.util.Map $environmentVariables = _environmentVariables;
@javax.annotation.Nullable
private final java.lang.String $projectName = _projectName;
@javax.annotation.Nullable
private final software.amazon.awscdk.services.iam.IRole $role = _role;
@javax.annotation.Nullable
private final java.util.List $securityGroups = _securityGroups;
@javax.annotation.Nullable
private final software.amazon.awscdk.services.ec2.SubnetSelection $subnetSelection = _subnetSelection;
@javax.annotation.Nullable
private final java.lang.Number $timeout = _timeout;
@javax.annotation.Nullable
private final software.amazon.awscdk.services.ec2.IVpcNetwork $vpc = _vpc;
@Override
public java.lang.Boolean getAllowAllOutbound() {
return this.$allowAllOutbound;
}
@Override
public java.lang.Boolean getBadge() {
return this.$badge;
}
@Override
public software.amazon.awscdk.assets.Asset getBuildScriptAsset() {
return this.$buildScriptAsset;
}
@Override
public java.lang.String getBuildScriptAssetEntrypoint() {
return this.$buildScriptAssetEntrypoint;
}
@Override
public java.lang.Object getBuildSpec() {
return this.$buildSpec;
}
@Override
public software.amazon.awscdk.services.s3.IBucket getCacheBucket() {
return this.$cacheBucket;
}
@Override
public java.lang.String getCacheDir() {
return this.$cacheDir;
}
@Override
public java.lang.String getDescription() {
return this.$description;
}
@Override
public software.amazon.awscdk.services.kms.IEncryptionKey getEncryptionKey() {
return this.$encryptionKey;
}
@Override
public software.amazon.awscdk.services.codebuild.BuildEnvironment getEnvironment() {
return this.$environment;
}
@Override
public java.util.Map getEnvironmentVariables() {
return this.$environmentVariables;
}
@Override
public java.lang.String getProjectName() {
return this.$projectName;
}
@Override
public software.amazon.awscdk.services.iam.IRole getRole() {
return this.$role;
}
@Override
public java.util.List getSecurityGroups() {
return this.$securityGroups;
}
@Override
public software.amazon.awscdk.services.ec2.SubnetSelection getSubnetSelection() {
return this.$subnetSelection;
}
@Override
public java.lang.Number getTimeout() {
return this.$timeout;
}
@Override
public software.amazon.awscdk.services.ec2.IVpcNetwork getVpc() {
return this.$vpc;
}
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("allowAllOutbound", om.valueToTree(this.getAllowAllOutbound()));
obj.set("badge", om.valueToTree(this.getBadge()));
obj.set("buildScriptAsset", om.valueToTree(this.getBuildScriptAsset()));
obj.set("buildScriptAssetEntrypoint", om.valueToTree(this.getBuildScriptAssetEntrypoint()));
obj.set("buildSpec", om.valueToTree(this.getBuildSpec()));
obj.set("cacheBucket", om.valueToTree(this.getCacheBucket()));
obj.set("cacheDir", om.valueToTree(this.getCacheDir()));
obj.set("description", om.valueToTree(this.getDescription()));
obj.set("encryptionKey", om.valueToTree(this.getEncryptionKey()));
obj.set("environment", om.valueToTree(this.getEnvironment()));
obj.set("environmentVariables", om.valueToTree(this.getEnvironmentVariables()));
obj.set("projectName", om.valueToTree(this.getProjectName()));
obj.set("role", om.valueToTree(this.getRole()));
obj.set("securityGroups", om.valueToTree(this.getSecurityGroups()));
obj.set("subnetSelection", om.valueToTree(this.getSubnetSelection()));
obj.set("timeout", om.valueToTree(this.getTimeout()));
obj.set("vpc", om.valueToTree(this.getVpc()));
return obj;
}
};
}
}
/**
* A proxy class which represents a concrete javascript instance of this type.
*/
final static class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements software.amazon.awscdk.services.codebuild.CommonProjectProps {
protected Jsii$Proxy(final software.amazon.jsii.JsiiObject.InitializationMode mode) {
super(mode);
}
/**
* Whether to allow the CodeBuild to send all network traffic.
*
* If set to false, you must individually add traffic rules to allow the
* CodeBuild project to connect to network targets.
*
* Only used if 'vpc' is supplied.
*
* Default: true
*/
@Override
@javax.annotation.Nullable
public java.lang.Boolean getAllowAllOutbound() {
return this.jsiiGet("allowAllOutbound", java.lang.Boolean.class);
}
/**
* Indicates whether AWS CodeBuild generates a publicly accessible URL for your project's build badge.
*
* For more information, see Build Badges Sample
* in the AWS CodeBuild User Guide.
*/
@Override
@javax.annotation.Nullable
public java.lang.Boolean getBadge() {
return this.jsiiGet("badge", java.lang.Boolean.class);
}
/**
* Run a script from an asset as build script.
*
* If supplied together with buildSpec, the asset script will be run
* _after_ the existing commands in buildspec.
*
* This feature can also be used without a source, to simply run an
* arbitrary script in a serverless way.
*
* Default: No asset build script
*/
@Override
@javax.annotation.Nullable
public software.amazon.awscdk.assets.Asset getBuildScriptAsset() {
return this.jsiiGet("buildScriptAsset", software.amazon.awscdk.assets.Asset.class);
}
/**
* The script in the asset to run.
*
* Default: build.sh
*/
@Override
@javax.annotation.Nullable
public java.lang.String getBuildScriptAssetEntrypoint() {
return this.jsiiGet("buildScriptAssetEntrypoint", java.lang.String.class);
}
/**
* Filename or contents of buildspec in JSON format.
*
* @see https://docs.aws.amazon.com/codebuild/latest/userguide/build-spec-ref.html#build-spec-ref-example
*/
@Override
@javax.annotation.Nullable
public java.lang.Object getBuildSpec() {
return this.jsiiGet("buildSpec", java.lang.Object.class);
}
/**
* Bucket to store cached source artifacts If not specified, source artifacts will not be cached.
*/
@Override
@javax.annotation.Nullable
public software.amazon.awscdk.services.s3.IBucket getCacheBucket() {
return this.jsiiGet("cacheBucket", software.amazon.awscdk.services.s3.IBucket.class);
}
/**
* Subdirectory to store cached artifacts.
*/
@Override
@javax.annotation.Nullable
public java.lang.String getCacheDir() {
return this.jsiiGet("cacheDir", java.lang.String.class);
}
/**
* A description of the project.
*
* Use the description to identify the purpose
* of the project.
*/
@Override
@javax.annotation.Nullable
public java.lang.String getDescription() {
return this.jsiiGet("description", java.lang.String.class);
}
/**
* Encryption key to use to read and write artifacts If not specified, a role will be created.
*/
@Override
@javax.annotation.Nullable
public software.amazon.awscdk.services.kms.IEncryptionKey getEncryptionKey() {
return this.jsiiGet("encryptionKey", software.amazon.awscdk.services.kms.IEncryptionKey.class);
}
/**
* Build environment to use for the build.
*/
@Override
@javax.annotation.Nullable
public software.amazon.awscdk.services.codebuild.BuildEnvironment getEnvironment() {
return this.jsiiGet("environment", software.amazon.awscdk.services.codebuild.BuildEnvironment.class);
}
/**
* Additional environment variables to add to the build environment.
*/
@Override
@javax.annotation.Nullable
public java.util.Map getEnvironmentVariables() {
return this.jsiiGet("environmentVariables", java.util.Map.class);
}
/**
* The physical, human-readable name of the CodeBuild Project.
*/
@Override
@javax.annotation.Nullable
public java.lang.String getProjectName() {
return this.jsiiGet("projectName", java.lang.String.class);
}
/**
* Service Role to assume while running the build. If not specified, a role will be created.
*/
@Override
@javax.annotation.Nullable
public software.amazon.awscdk.services.iam.IRole getRole() {
return this.jsiiGet("role", software.amazon.awscdk.services.iam.IRole.class);
}
/**
* What security group to associate with the codebuild project's network interfaces. If no security group is identified, one will be created automatically.
*
* Only used if 'vpc' is supplied.
*/
@Override
@javax.annotation.Nullable
public java.util.List getSecurityGroups() {
return this.jsiiGet("securityGroups", java.util.List.class);
}
/**
* Where to place the network interfaces within the VPC.
*
* Only used if 'vpc' is supplied.
*
* Default: All private subnets
*/
@Override
@javax.annotation.Nullable
public software.amazon.awscdk.services.ec2.SubnetSelection getSubnetSelection() {
return this.jsiiGet("subnetSelection", software.amazon.awscdk.services.ec2.SubnetSelection.class);
}
/**
* The number of minutes after which AWS CodeBuild stops the build if it's not complete.
*
* For valid values, see the timeoutInMinutes field in the AWS
* CodeBuild User Guide.
*/
@Override
@javax.annotation.Nullable
public java.lang.Number getTimeout() {
return this.jsiiGet("timeout", java.lang.Number.class);
}
/**
* VPC network to place codebuild network interfaces.
*
* Specify this if the codebuild project needs to access resources in a VPC.
*/
@Override
@javax.annotation.Nullable
public software.amazon.awscdk.services.ec2.IVpcNetwork getVpc() {
return this.jsiiGet("vpc", software.amazon.awscdk.services.ec2.IVpcNetwork.class);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy