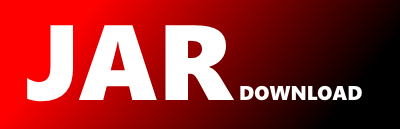
software.amazon.awscdk.services.codebuild.CfnProjectProps Maven / Gradle / Ivy
Show all versions of codebuild Show documentation
package software.amazon.awscdk.services.codebuild;
/**
* Properties for defining a `CfnProject`.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.codebuild.*;
* CfnProjectProps cfnProjectProps = CfnProjectProps.builder()
* .artifacts(ArtifactsProperty.builder()
* .type("type")
* // the properties below are optional
* .artifactIdentifier("artifactIdentifier")
* .encryptionDisabled(false)
* .location("location")
* .name("name")
* .namespaceType("namespaceType")
* .overrideArtifactName(false)
* .packaging("packaging")
* .path("path")
* .build())
* .environment(EnvironmentProperty.builder()
* .computeType("computeType")
* .image("image")
* .type("type")
* // the properties below are optional
* .certificate("certificate")
* .environmentVariables(List.of(EnvironmentVariableProperty.builder()
* .name("name")
* .value("value")
* // the properties below are optional
* .type("type")
* .build()))
* .imagePullCredentialsType("imagePullCredentialsType")
* .privilegedMode(false)
* .registryCredential(RegistryCredentialProperty.builder()
* .credential("credential")
* .credentialProvider("credentialProvider")
* .build())
* .build())
* .serviceRole("serviceRole")
* .source(SourceProperty.builder()
* .type("type")
* // the properties below are optional
* .auth(SourceAuthProperty.builder()
* .type("type")
* // the properties below are optional
* .resource("resource")
* .build())
* .buildSpec("buildSpec")
* .buildStatusConfig(BuildStatusConfigProperty.builder()
* .context("context")
* .targetUrl("targetUrl")
* .build())
* .gitCloneDepth(123)
* .gitSubmodulesConfig(GitSubmodulesConfigProperty.builder()
* .fetchSubmodules(false)
* .build())
* .insecureSsl(false)
* .location("location")
* .reportBuildStatus(false)
* .sourceIdentifier("sourceIdentifier")
* .build())
* // the properties below are optional
* .badgeEnabled(false)
* .buildBatchConfig(ProjectBuildBatchConfigProperty.builder()
* .batchReportMode("batchReportMode")
* .combineArtifacts(false)
* .restrictions(BatchRestrictionsProperty.builder()
* .computeTypesAllowed(List.of("computeTypesAllowed"))
* .maximumBuildsAllowed(123)
* .build())
* .serviceRole("serviceRole")
* .timeoutInMins(123)
* .build())
* .cache(ProjectCacheProperty.builder()
* .type("type")
* // the properties below are optional
* .location("location")
* .modes(List.of("modes"))
* .build())
* .concurrentBuildLimit(123)
* .description("description")
* .encryptionKey("encryptionKey")
* .fileSystemLocations(List.of(ProjectFileSystemLocationProperty.builder()
* .identifier("identifier")
* .location("location")
* .mountPoint("mountPoint")
* .type("type")
* // the properties below are optional
* .mountOptions("mountOptions")
* .build()))
* .logsConfig(LogsConfigProperty.builder()
* .cloudWatchLogs(CloudWatchLogsConfigProperty.builder()
* .status("status")
* // the properties below are optional
* .groupName("groupName")
* .streamName("streamName")
* .build())
* .s3Logs(S3LogsConfigProperty.builder()
* .status("status")
* // the properties below are optional
* .encryptionDisabled(false)
* .location("location")
* .build())
* .build())
* .name("name")
* .queuedTimeoutInMinutes(123)
* .resourceAccessRole("resourceAccessRole")
* .secondaryArtifacts(List.of(ArtifactsProperty.builder()
* .type("type")
* // the properties below are optional
* .artifactIdentifier("artifactIdentifier")
* .encryptionDisabled(false)
* .location("location")
* .name("name")
* .namespaceType("namespaceType")
* .overrideArtifactName(false)
* .packaging("packaging")
* .path("path")
* .build()))
* .secondarySources(List.of(SourceProperty.builder()
* .type("type")
* // the properties below are optional
* .auth(SourceAuthProperty.builder()
* .type("type")
* // the properties below are optional
* .resource("resource")
* .build())
* .buildSpec("buildSpec")
* .buildStatusConfig(BuildStatusConfigProperty.builder()
* .context("context")
* .targetUrl("targetUrl")
* .build())
* .gitCloneDepth(123)
* .gitSubmodulesConfig(GitSubmodulesConfigProperty.builder()
* .fetchSubmodules(false)
* .build())
* .insecureSsl(false)
* .location("location")
* .reportBuildStatus(false)
* .sourceIdentifier("sourceIdentifier")
* .build()))
* .secondarySourceVersions(List.of(ProjectSourceVersionProperty.builder()
* .sourceIdentifier("sourceIdentifier")
* // the properties below are optional
* .sourceVersion("sourceVersion")
* .build()))
* .sourceVersion("sourceVersion")
* .tags(List.of(CfnTag.builder()
* .key("key")
* .value("value")
* .build()))
* .timeoutInMinutes(123)
* .triggers(ProjectTriggersProperty.builder()
* .buildType("buildType")
* .filterGroups(List.of(List.of(WebhookFilterProperty.builder()
* .pattern("pattern")
* .type("type")
* // the properties below are optional
* .excludeMatchedPattern(false)
* .build())))
* .webhook(false)
* .build())
* .visibility("visibility")
* .vpcConfig(VpcConfigProperty.builder()
* .securityGroupIds(List.of("securityGroupIds"))
* .subnets(List.of("subnets"))
* .vpcId("vpcId")
* .build())
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.52.1 (build 5ccc8f6)", date = "2022-01-20T19:50:01.529Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.codebuild.$Module.class, fqn = "@aws-cdk/aws-codebuild.CfnProjectProps")
@software.amazon.jsii.Jsii.Proxy(CfnProjectProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface CfnProjectProps extends software.amazon.jsii.JsiiSerializable {
/**
* `Artifacts` is a property of the [AWS::CodeBuild::Project](https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-codebuild-project.html) resource that specifies output settings for artifacts generated by an AWS CodeBuild build.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.Object getArtifacts();
/**
* The build environment settings for the project, such as the environment type or the environment variables to use for the build environment.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.Object getEnvironment();
/**
* The ARN of the IAM role that enables AWS CodeBuild to interact with dependent AWS services on behalf of the AWS account.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getServiceRole();
/**
* The source code settings for the project, such as the source code's repository type and location.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.Object getSource();
/**
* Indicates whether AWS CodeBuild generates a publicly accessible URL for your project's build badge.
*
* For more information, see Build Badges Sample in the AWS CodeBuild User Guide .
*
*
*
* Including build badges with your project is currently not supported if the source type is CodePipeline. If you specify CODEPIPELINE
for the Source
property, do not specify the BadgeEnabled
property.
*
*
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getBadgeEnabled() {
return null;
}
/**
* A `ProjectBuildBatchConfig` object that defines the batch build options for the project.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getBuildBatchConfig() {
return null;
}
/**
* Settings that AWS CodeBuild uses to store and reuse build dependencies.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getCache() {
return null;
}
/**
* The maximum number of concurrent builds that are allowed for this project.
*
* New builds are only started if the current number of builds is less than or equal to this limit. If the current build count meets this limit, new builds are throttled and are not run.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Number getConcurrentBuildLimit() {
return null;
}
/**
* A description that makes the build project easy to identify.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getDescription() {
return null;
}
/**
* The AWS Key Management Service customer master key (CMK) to be used for encrypting the build output artifacts.
*
*
*
* You can use a cross-account KMS key to encrypt the build output artifacts if your service role has permission to that key.
*
*
*
* You can specify either the Amazon Resource Name (ARN) of the CMK or, if available, the CMK's alias (using the format alias/<alias-name>
). If you don't specify a value, CodeBuild uses the managed CMK for Amazon Simple Storage Service (Amazon S3).
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getEncryptionKey() {
return null;
}
/**
* An array of `ProjectFileSystemLocation` objects for a CodeBuild build project.
*
* A ProjectFileSystemLocation
object specifies the identifier
, location
, mountOptions
, mountPoint
, and type
of a file system created using Amazon Elastic File System.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getFileSystemLocations() {
return null;
}
/**
* Information about logs for the build project.
*
* A project can create logs in CloudWatch Logs, an S3 bucket, or both.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getLogsConfig() {
return null;
}
/**
* The name of the build project.
*
* The name must be unique across all of the projects in your AWS account .
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getName() {
return null;
}
/**
* The number of minutes a build is allowed to be queued before it times out.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Number getQueuedTimeoutInMinutes() {
return null;
}
/**
* The ARN of the IAM role that enables CodeBuild to access the CloudWatch Logs and Amazon S3 artifacts for the project's builds.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getResourceAccessRole() {
return null;
}
/**
* A list of `Artifacts` objects.
*
* Each artifacts object specifies output settings that the project generates during a build.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getSecondaryArtifacts() {
return null;
}
/**
* An array of `ProjectSource` objects.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getSecondarySources() {
return null;
}
/**
* An array of `ProjectSourceVersion` objects.
*
* If secondarySourceVersions
is specified at the build level, then they take over these secondarySourceVersions
(at the project level).
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getSecondarySourceVersions() {
return null;
}
/**
* A version of the build input to be built for this project.
*
* If not specified, the latest version is used. If specified, it must be one of:
*
*
* - For CodeCommit: the commit ID, branch, or Git tag to use.
* - For GitHub: the commit ID, pull request ID, branch name, or tag name that corresponds to the version of the source code you want to build. If a pull request ID is specified, it must use the format
pr/pull-request-ID
(for example pr/25
). If a branch name is specified, the branch's HEAD commit ID is used. If not specified, the default branch's HEAD commit ID is used.
* - For Bitbucket: the commit ID, branch name, or tag name that corresponds to the version of the source code you want to build. If a branch name is specified, the branch's HEAD commit ID is used. If not specified, the default branch's HEAD commit ID is used.
* - For Amazon S3: the version ID of the object that represents the build input ZIP file to use.
*
*
* If sourceVersion
is specified at the build level, then that version takes precedence over this sourceVersion
(at the project level).
*
* For more information, see Source Version Sample with CodeBuild in the AWS CodeBuild User Guide .
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getSourceVersion() {
return null;
}
/**
* An arbitrary set of tags (key-value pairs) for the AWS CodeBuild project.
*
* These tags are available for use by AWS services that support AWS CodeBuild build project tags.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getTags() {
return null;
}
/**
* How long, in minutes, from 5 to 480 (8 hours), for AWS CodeBuild to wait before timing out any related build that did not get marked as completed.
*
* The default is 60 minutes.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Number getTimeoutInMinutes() {
return null;
}
/**
* For an existing AWS CodeBuild build project that has its source code stored in a GitHub repository, enables AWS CodeBuild to begin automatically rebuilding the source code every time a code change is pushed to the repository.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getTriggers() {
return null;
}
/**
* Specifies the visibility of the project's builds. Possible values are:.
*
*
* - PUBLIC_READ - The project builds are visible to the public.
* - PRIVATE - The project builds are not visible to the public.
*
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getVisibility() {
return null;
}
/**
* `VpcConfig` specifies settings that enable AWS CodeBuild to access resources in an Amazon VPC.
*
* For more information, see Use AWS CodeBuild with Amazon Virtual Private Cloud in the AWS CodeBuild User Guide .
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Object getVpcConfig() {
return null;
}
/**
* @return a {@link Builder} of {@link CfnProjectProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link CfnProjectProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.Object artifacts;
java.lang.Object environment;
java.lang.String serviceRole;
java.lang.Object source;
java.lang.Object badgeEnabled;
java.lang.Object buildBatchConfig;
java.lang.Object cache;
java.lang.Number concurrentBuildLimit;
java.lang.String description;
java.lang.String encryptionKey;
java.lang.Object fileSystemLocations;
java.lang.Object logsConfig;
java.lang.String name;
java.lang.Number queuedTimeoutInMinutes;
java.lang.String resourceAccessRole;
java.lang.Object secondaryArtifacts;
java.lang.Object secondarySources;
java.lang.Object secondarySourceVersions;
java.lang.String sourceVersion;
java.util.List tags;
java.lang.Number timeoutInMinutes;
java.lang.Object triggers;
java.lang.String visibility;
java.lang.Object vpcConfig;
/**
* Sets the value of {@link CfnProjectProps#getArtifacts}
* @param artifacts `Artifacts` is a property of the [AWS::CodeBuild::Project](https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-codebuild-project.html) resource that specifies output settings for artifacts generated by an AWS CodeBuild build. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder artifacts(software.amazon.awscdk.services.codebuild.CfnProject.ArtifactsProperty artifacts) {
this.artifacts = artifacts;
return this;
}
/**
* Sets the value of {@link CfnProjectProps#getArtifacts}
* @param artifacts `Artifacts` is a property of the [AWS::CodeBuild::Project](https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-codebuild-project.html) resource that specifies output settings for artifacts generated by an AWS CodeBuild build. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder artifacts(software.amazon.awscdk.core.IResolvable artifacts) {
this.artifacts = artifacts;
return this;
}
/**
* Sets the value of {@link CfnProjectProps#getEnvironment}
* @param environment The build environment settings for the project, such as the environment type or the environment variables to use for the build environment. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder environment(software.amazon.awscdk.core.IResolvable environment) {
this.environment = environment;
return this;
}
/**
* Sets the value of {@link CfnProjectProps#getEnvironment}
* @param environment The build environment settings for the project, such as the environment type or the environment variables to use for the build environment. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder environment(software.amazon.awscdk.services.codebuild.CfnProject.EnvironmentProperty environment) {
this.environment = environment;
return this;
}
/**
* Sets the value of {@link CfnProjectProps#getServiceRole}
* @param serviceRole The ARN of the IAM role that enables AWS CodeBuild to interact with dependent AWS services on behalf of the AWS account. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder serviceRole(java.lang.String serviceRole) {
this.serviceRole = serviceRole;
return this;
}
/**
* Sets the value of {@link CfnProjectProps#getSource}
* @param source The source code settings for the project, such as the source code's repository type and location. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder source(software.amazon.awscdk.services.codebuild.CfnProject.SourceProperty source) {
this.source = source;
return this;
}
/**
* Sets the value of {@link CfnProjectProps#getSource}
* @param source The source code settings for the project, such as the source code's repository type and location. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder source(software.amazon.awscdk.core.IResolvable source) {
this.source = source;
return this;
}
/**
* Sets the value of {@link CfnProjectProps#getBadgeEnabled}
* @param badgeEnabled Indicates whether AWS CodeBuild generates a publicly accessible URL for your project's build badge.
* For more information, see Build Badges Sample in the AWS CodeBuild User Guide .
*
*
*
* Including build badges with your project is currently not supported if the source type is CodePipeline. If you specify CODEPIPELINE
for the Source
property, do not specify the BadgeEnabled
property.
*
*
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder badgeEnabled(java.lang.Boolean badgeEnabled) {
this.badgeEnabled = badgeEnabled;
return this;
}
/**
* Sets the value of {@link CfnProjectProps#getBadgeEnabled}
* @param badgeEnabled Indicates whether AWS CodeBuild generates a publicly accessible URL for your project's build badge.
* For more information, see Build Badges Sample in the AWS CodeBuild User Guide .
*
*
*
* Including build badges with your project is currently not supported if the source type is CodePipeline. If you specify CODEPIPELINE
for the Source
property, do not specify the BadgeEnabled
property.
*
*
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder badgeEnabled(software.amazon.awscdk.core.IResolvable badgeEnabled) {
this.badgeEnabled = badgeEnabled;
return this;
}
/**
* Sets the value of {@link CfnProjectProps#getBuildBatchConfig}
* @param buildBatchConfig A `ProjectBuildBatchConfig` object that defines the batch build options for the project.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder buildBatchConfig(software.amazon.awscdk.core.IResolvable buildBatchConfig) {
this.buildBatchConfig = buildBatchConfig;
return this;
}
/**
* Sets the value of {@link CfnProjectProps#getBuildBatchConfig}
* @param buildBatchConfig A `ProjectBuildBatchConfig` object that defines the batch build options for the project.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder buildBatchConfig(software.amazon.awscdk.services.codebuild.CfnProject.ProjectBuildBatchConfigProperty buildBatchConfig) {
this.buildBatchConfig = buildBatchConfig;
return this;
}
/**
* Sets the value of {@link CfnProjectProps#getCache}
* @param cache Settings that AWS CodeBuild uses to store and reuse build dependencies.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder cache(software.amazon.awscdk.core.IResolvable cache) {
this.cache = cache;
return this;
}
/**
* Sets the value of {@link CfnProjectProps#getCache}
* @param cache Settings that AWS CodeBuild uses to store and reuse build dependencies.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder cache(software.amazon.awscdk.services.codebuild.CfnProject.ProjectCacheProperty cache) {
this.cache = cache;
return this;
}
/**
* Sets the value of {@link CfnProjectProps#getConcurrentBuildLimit}
* @param concurrentBuildLimit The maximum number of concurrent builds that are allowed for this project.
* New builds are only started if the current number of builds is less than or equal to this limit. If the current build count meets this limit, new builds are throttled and are not run.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder concurrentBuildLimit(java.lang.Number concurrentBuildLimit) {
this.concurrentBuildLimit = concurrentBuildLimit;
return this;
}
/**
* Sets the value of {@link CfnProjectProps#getDescription}
* @param description A description that makes the build project easy to identify.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder description(java.lang.String description) {
this.description = description;
return this;
}
/**
* Sets the value of {@link CfnProjectProps#getEncryptionKey}
* @param encryptionKey The AWS Key Management Service customer master key (CMK) to be used for encrypting the build output artifacts.
*
*
* You can use a cross-account KMS key to encrypt the build output artifacts if your service role has permission to that key.
*
*
*
* You can specify either the Amazon Resource Name (ARN) of the CMK or, if available, the CMK's alias (using the format alias/<alias-name>
). If you don't specify a value, CodeBuild uses the managed CMK for Amazon Simple Storage Service (Amazon S3).
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder encryptionKey(java.lang.String encryptionKey) {
this.encryptionKey = encryptionKey;
return this;
}
/**
* Sets the value of {@link CfnProjectProps#getFileSystemLocations}
* @param fileSystemLocations An array of `ProjectFileSystemLocation` objects for a CodeBuild build project.
* A ProjectFileSystemLocation
object specifies the identifier
, location
, mountOptions
, mountPoint
, and type
of a file system created using Amazon Elastic File System.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder fileSystemLocations(software.amazon.awscdk.core.IResolvable fileSystemLocations) {
this.fileSystemLocations = fileSystemLocations;
return this;
}
/**
* Sets the value of {@link CfnProjectProps#getFileSystemLocations}
* @param fileSystemLocations An array of `ProjectFileSystemLocation` objects for a CodeBuild build project.
* A ProjectFileSystemLocation
object specifies the identifier
, location
, mountOptions
, mountPoint
, and type
of a file system created using Amazon Elastic File System.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder fileSystemLocations(java.util.List extends java.lang.Object> fileSystemLocations) {
this.fileSystemLocations = fileSystemLocations;
return this;
}
/**
* Sets the value of {@link CfnProjectProps#getLogsConfig}
* @param logsConfig Information about logs for the build project.
* A project can create logs in CloudWatch Logs, an S3 bucket, or both.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder logsConfig(software.amazon.awscdk.core.IResolvable logsConfig) {
this.logsConfig = logsConfig;
return this;
}
/**
* Sets the value of {@link CfnProjectProps#getLogsConfig}
* @param logsConfig Information about logs for the build project.
* A project can create logs in CloudWatch Logs, an S3 bucket, or both.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder logsConfig(software.amazon.awscdk.services.codebuild.CfnProject.LogsConfigProperty logsConfig) {
this.logsConfig = logsConfig;
return this;
}
/**
* Sets the value of {@link CfnProjectProps#getName}
* @param name The name of the build project.
* The name must be unique across all of the projects in your AWS account .
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder name(java.lang.String name) {
this.name = name;
return this;
}
/**
* Sets the value of {@link CfnProjectProps#getQueuedTimeoutInMinutes}
* @param queuedTimeoutInMinutes The number of minutes a build is allowed to be queued before it times out.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder queuedTimeoutInMinutes(java.lang.Number queuedTimeoutInMinutes) {
this.queuedTimeoutInMinutes = queuedTimeoutInMinutes;
return this;
}
/**
* Sets the value of {@link CfnProjectProps#getResourceAccessRole}
* @param resourceAccessRole The ARN of the IAM role that enables CodeBuild to access the CloudWatch Logs and Amazon S3 artifacts for the project's builds.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder resourceAccessRole(java.lang.String resourceAccessRole) {
this.resourceAccessRole = resourceAccessRole;
return this;
}
/**
* Sets the value of {@link CfnProjectProps#getSecondaryArtifacts}
* @param secondaryArtifacts A list of `Artifacts` objects.
* Each artifacts object specifies output settings that the project generates during a build.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder secondaryArtifacts(software.amazon.awscdk.core.IResolvable secondaryArtifacts) {
this.secondaryArtifacts = secondaryArtifacts;
return this;
}
/**
* Sets the value of {@link CfnProjectProps#getSecondaryArtifacts}
* @param secondaryArtifacts A list of `Artifacts` objects.
* Each artifacts object specifies output settings that the project generates during a build.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder secondaryArtifacts(java.util.List extends java.lang.Object> secondaryArtifacts) {
this.secondaryArtifacts = secondaryArtifacts;
return this;
}
/**
* Sets the value of {@link CfnProjectProps#getSecondarySources}
* @param secondarySources An array of `ProjectSource` objects.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder secondarySources(software.amazon.awscdk.core.IResolvable secondarySources) {
this.secondarySources = secondarySources;
return this;
}
/**
* Sets the value of {@link CfnProjectProps#getSecondarySources}
* @param secondarySources An array of `ProjectSource` objects.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder secondarySources(java.util.List extends java.lang.Object> secondarySources) {
this.secondarySources = secondarySources;
return this;
}
/**
* Sets the value of {@link CfnProjectProps#getSecondarySourceVersions}
* @param secondarySourceVersions An array of `ProjectSourceVersion` objects.
* If secondarySourceVersions
is specified at the build level, then they take over these secondarySourceVersions
(at the project level).
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder secondarySourceVersions(software.amazon.awscdk.core.IResolvable secondarySourceVersions) {
this.secondarySourceVersions = secondarySourceVersions;
return this;
}
/**
* Sets the value of {@link CfnProjectProps#getSecondarySourceVersions}
* @param secondarySourceVersions An array of `ProjectSourceVersion` objects.
* If secondarySourceVersions
is specified at the build level, then they take over these secondarySourceVersions
(at the project level).
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder secondarySourceVersions(java.util.List extends java.lang.Object> secondarySourceVersions) {
this.secondarySourceVersions = secondarySourceVersions;
return this;
}
/**
* Sets the value of {@link CfnProjectProps#getSourceVersion}
* @param sourceVersion A version of the build input to be built for this project.
* If not specified, the latest version is used. If specified, it must be one of:
*
*
* - For CodeCommit: the commit ID, branch, or Git tag to use.
* - For GitHub: the commit ID, pull request ID, branch name, or tag name that corresponds to the version of the source code you want to build. If a pull request ID is specified, it must use the format
pr/pull-request-ID
(for example pr/25
). If a branch name is specified, the branch's HEAD commit ID is used. If not specified, the default branch's HEAD commit ID is used.
* - For Bitbucket: the commit ID, branch name, or tag name that corresponds to the version of the source code you want to build. If a branch name is specified, the branch's HEAD commit ID is used. If not specified, the default branch's HEAD commit ID is used.
* - For Amazon S3: the version ID of the object that represents the build input ZIP file to use.
*
*
* If sourceVersion
is specified at the build level, then that version takes precedence over this sourceVersion
(at the project level).
*
* For more information, see Source Version Sample with CodeBuild in the AWS CodeBuild User Guide .
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder sourceVersion(java.lang.String sourceVersion) {
this.sourceVersion = sourceVersion;
return this;
}
/**
* Sets the value of {@link CfnProjectProps#getTags}
* @param tags An arbitrary set of tags (key-value pairs) for the AWS CodeBuild project.
* These tags are available for use by AWS services that support AWS CodeBuild build project tags.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@SuppressWarnings("unchecked")
public Builder tags(java.util.List extends software.amazon.awscdk.core.CfnTag> tags) {
this.tags = (java.util.List)tags;
return this;
}
/**
* Sets the value of {@link CfnProjectProps#getTimeoutInMinutes}
* @param timeoutInMinutes How long, in minutes, from 5 to 480 (8 hours), for AWS CodeBuild to wait before timing out any related build that did not get marked as completed.
* The default is 60 minutes.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder timeoutInMinutes(java.lang.Number timeoutInMinutes) {
this.timeoutInMinutes = timeoutInMinutes;
return this;
}
/**
* Sets the value of {@link CfnProjectProps#getTriggers}
* @param triggers For an existing AWS CodeBuild build project that has its source code stored in a GitHub repository, enables AWS CodeBuild to begin automatically rebuilding the source code every time a code change is pushed to the repository.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder triggers(software.amazon.awscdk.core.IResolvable triggers) {
this.triggers = triggers;
return this;
}
/**
* Sets the value of {@link CfnProjectProps#getTriggers}
* @param triggers For an existing AWS CodeBuild build project that has its source code stored in a GitHub repository, enables AWS CodeBuild to begin automatically rebuilding the source code every time a code change is pushed to the repository.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder triggers(software.amazon.awscdk.services.codebuild.CfnProject.ProjectTriggersProperty triggers) {
this.triggers = triggers;
return this;
}
/**
* Sets the value of {@link CfnProjectProps#getVisibility}
* @param visibility Specifies the visibility of the project's builds. Possible values are:.
*
* - PUBLIC_READ - The project builds are visible to the public.
* - PRIVATE - The project builds are not visible to the public.
*
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder visibility(java.lang.String visibility) {
this.visibility = visibility;
return this;
}
/**
* Sets the value of {@link CfnProjectProps#getVpcConfig}
* @param vpcConfig `VpcConfig` specifies settings that enable AWS CodeBuild to access resources in an Amazon VPC.
* For more information, see Use AWS CodeBuild with Amazon Virtual Private Cloud in the AWS CodeBuild User Guide .
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder vpcConfig(software.amazon.awscdk.core.IResolvable vpcConfig) {
this.vpcConfig = vpcConfig;
return this;
}
/**
* Sets the value of {@link CfnProjectProps#getVpcConfig}
* @param vpcConfig `VpcConfig` specifies settings that enable AWS CodeBuild to access resources in an Amazon VPC.
* For more information, see Use AWS CodeBuild with Amazon Virtual Private Cloud in the AWS CodeBuild User Guide .
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder vpcConfig(software.amazon.awscdk.services.codebuild.CfnProject.VpcConfigProperty vpcConfig) {
this.vpcConfig = vpcConfig;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link CfnProjectProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public CfnProjectProps build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link CfnProjectProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements CfnProjectProps {
private final java.lang.Object artifacts;
private final java.lang.Object environment;
private final java.lang.String serviceRole;
private final java.lang.Object source;
private final java.lang.Object badgeEnabled;
private final java.lang.Object buildBatchConfig;
private final java.lang.Object cache;
private final java.lang.Number concurrentBuildLimit;
private final java.lang.String description;
private final java.lang.String encryptionKey;
private final java.lang.Object fileSystemLocations;
private final java.lang.Object logsConfig;
private final java.lang.String name;
private final java.lang.Number queuedTimeoutInMinutes;
private final java.lang.String resourceAccessRole;
private final java.lang.Object secondaryArtifacts;
private final java.lang.Object secondarySources;
private final java.lang.Object secondarySourceVersions;
private final java.lang.String sourceVersion;
private final java.util.List tags;
private final java.lang.Number timeoutInMinutes;
private final java.lang.Object triggers;
private final java.lang.String visibility;
private final java.lang.Object vpcConfig;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.artifacts = software.amazon.jsii.Kernel.get(this, "artifacts", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.environment = software.amazon.jsii.Kernel.get(this, "environment", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.serviceRole = software.amazon.jsii.Kernel.get(this, "serviceRole", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.source = software.amazon.jsii.Kernel.get(this, "source", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.badgeEnabled = software.amazon.jsii.Kernel.get(this, "badgeEnabled", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.buildBatchConfig = software.amazon.jsii.Kernel.get(this, "buildBatchConfig", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.cache = software.amazon.jsii.Kernel.get(this, "cache", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.concurrentBuildLimit = software.amazon.jsii.Kernel.get(this, "concurrentBuildLimit", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.description = software.amazon.jsii.Kernel.get(this, "description", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.encryptionKey = software.amazon.jsii.Kernel.get(this, "encryptionKey", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.fileSystemLocations = software.amazon.jsii.Kernel.get(this, "fileSystemLocations", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.logsConfig = software.amazon.jsii.Kernel.get(this, "logsConfig", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.name = software.amazon.jsii.Kernel.get(this, "name", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.queuedTimeoutInMinutes = software.amazon.jsii.Kernel.get(this, "queuedTimeoutInMinutes", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.resourceAccessRole = software.amazon.jsii.Kernel.get(this, "resourceAccessRole", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.secondaryArtifacts = software.amazon.jsii.Kernel.get(this, "secondaryArtifacts", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.secondarySources = software.amazon.jsii.Kernel.get(this, "secondarySources", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.secondarySourceVersions = software.amazon.jsii.Kernel.get(this, "secondarySourceVersions", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.sourceVersion = software.amazon.jsii.Kernel.get(this, "sourceVersion", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.tags = software.amazon.jsii.Kernel.get(this, "tags", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.CfnTag.class)));
this.timeoutInMinutes = software.amazon.jsii.Kernel.get(this, "timeoutInMinutes", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.triggers = software.amazon.jsii.Kernel.get(this, "triggers", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
this.visibility = software.amazon.jsii.Kernel.get(this, "visibility", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.vpcConfig = software.amazon.jsii.Kernel.get(this, "vpcConfig", software.amazon.jsii.NativeType.forClass(java.lang.Object.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
@SuppressWarnings("unchecked")
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.artifacts = java.util.Objects.requireNonNull(builder.artifacts, "artifacts is required");
this.environment = java.util.Objects.requireNonNull(builder.environment, "environment is required");
this.serviceRole = java.util.Objects.requireNonNull(builder.serviceRole, "serviceRole is required");
this.source = java.util.Objects.requireNonNull(builder.source, "source is required");
this.badgeEnabled = builder.badgeEnabled;
this.buildBatchConfig = builder.buildBatchConfig;
this.cache = builder.cache;
this.concurrentBuildLimit = builder.concurrentBuildLimit;
this.description = builder.description;
this.encryptionKey = builder.encryptionKey;
this.fileSystemLocations = builder.fileSystemLocations;
this.logsConfig = builder.logsConfig;
this.name = builder.name;
this.queuedTimeoutInMinutes = builder.queuedTimeoutInMinutes;
this.resourceAccessRole = builder.resourceAccessRole;
this.secondaryArtifacts = builder.secondaryArtifacts;
this.secondarySources = builder.secondarySources;
this.secondarySourceVersions = builder.secondarySourceVersions;
this.sourceVersion = builder.sourceVersion;
this.tags = (java.util.List)builder.tags;
this.timeoutInMinutes = builder.timeoutInMinutes;
this.triggers = builder.triggers;
this.visibility = builder.visibility;
this.vpcConfig = builder.vpcConfig;
}
@Override
public final java.lang.Object getArtifacts() {
return this.artifacts;
}
@Override
public final java.lang.Object getEnvironment() {
return this.environment;
}
@Override
public final java.lang.String getServiceRole() {
return this.serviceRole;
}
@Override
public final java.lang.Object getSource() {
return this.source;
}
@Override
public final java.lang.Object getBadgeEnabled() {
return this.badgeEnabled;
}
@Override
public final java.lang.Object getBuildBatchConfig() {
return this.buildBatchConfig;
}
@Override
public final java.lang.Object getCache() {
return this.cache;
}
@Override
public final java.lang.Number getConcurrentBuildLimit() {
return this.concurrentBuildLimit;
}
@Override
public final java.lang.String getDescription() {
return this.description;
}
@Override
public final java.lang.String getEncryptionKey() {
return this.encryptionKey;
}
@Override
public final java.lang.Object getFileSystemLocations() {
return this.fileSystemLocations;
}
@Override
public final java.lang.Object getLogsConfig() {
return this.logsConfig;
}
@Override
public final java.lang.String getName() {
return this.name;
}
@Override
public final java.lang.Number getQueuedTimeoutInMinutes() {
return this.queuedTimeoutInMinutes;
}
@Override
public final java.lang.String getResourceAccessRole() {
return this.resourceAccessRole;
}
@Override
public final java.lang.Object getSecondaryArtifacts() {
return this.secondaryArtifacts;
}
@Override
public final java.lang.Object getSecondarySources() {
return this.secondarySources;
}
@Override
public final java.lang.Object getSecondarySourceVersions() {
return this.secondarySourceVersions;
}
@Override
public final java.lang.String getSourceVersion() {
return this.sourceVersion;
}
@Override
public final java.util.List getTags() {
return this.tags;
}
@Override
public final java.lang.Number getTimeoutInMinutes() {
return this.timeoutInMinutes;
}
@Override
public final java.lang.Object getTriggers() {
return this.triggers;
}
@Override
public final java.lang.String getVisibility() {
return this.visibility;
}
@Override
public final java.lang.Object getVpcConfig() {
return this.vpcConfig;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("artifacts", om.valueToTree(this.getArtifacts()));
data.set("environment", om.valueToTree(this.getEnvironment()));
data.set("serviceRole", om.valueToTree(this.getServiceRole()));
data.set("source", om.valueToTree(this.getSource()));
if (this.getBadgeEnabled() != null) {
data.set("badgeEnabled", om.valueToTree(this.getBadgeEnabled()));
}
if (this.getBuildBatchConfig() != null) {
data.set("buildBatchConfig", om.valueToTree(this.getBuildBatchConfig()));
}
if (this.getCache() != null) {
data.set("cache", om.valueToTree(this.getCache()));
}
if (this.getConcurrentBuildLimit() != null) {
data.set("concurrentBuildLimit", om.valueToTree(this.getConcurrentBuildLimit()));
}
if (this.getDescription() != null) {
data.set("description", om.valueToTree(this.getDescription()));
}
if (this.getEncryptionKey() != null) {
data.set("encryptionKey", om.valueToTree(this.getEncryptionKey()));
}
if (this.getFileSystemLocations() != null) {
data.set("fileSystemLocations", om.valueToTree(this.getFileSystemLocations()));
}
if (this.getLogsConfig() != null) {
data.set("logsConfig", om.valueToTree(this.getLogsConfig()));
}
if (this.getName() != null) {
data.set("name", om.valueToTree(this.getName()));
}
if (this.getQueuedTimeoutInMinutes() != null) {
data.set("queuedTimeoutInMinutes", om.valueToTree(this.getQueuedTimeoutInMinutes()));
}
if (this.getResourceAccessRole() != null) {
data.set("resourceAccessRole", om.valueToTree(this.getResourceAccessRole()));
}
if (this.getSecondaryArtifacts() != null) {
data.set("secondaryArtifacts", om.valueToTree(this.getSecondaryArtifacts()));
}
if (this.getSecondarySources() != null) {
data.set("secondarySources", om.valueToTree(this.getSecondarySources()));
}
if (this.getSecondarySourceVersions() != null) {
data.set("secondarySourceVersions", om.valueToTree(this.getSecondarySourceVersions()));
}
if (this.getSourceVersion() != null) {
data.set("sourceVersion", om.valueToTree(this.getSourceVersion()));
}
if (this.getTags() != null) {
data.set("tags", om.valueToTree(this.getTags()));
}
if (this.getTimeoutInMinutes() != null) {
data.set("timeoutInMinutes", om.valueToTree(this.getTimeoutInMinutes()));
}
if (this.getTriggers() != null) {
data.set("triggers", om.valueToTree(this.getTriggers()));
}
if (this.getVisibility() != null) {
data.set("visibility", om.valueToTree(this.getVisibility()));
}
if (this.getVpcConfig() != null) {
data.set("vpcConfig", om.valueToTree(this.getVpcConfig()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-codebuild.CfnProjectProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
CfnProjectProps.Jsii$Proxy that = (CfnProjectProps.Jsii$Proxy) o;
if (!artifacts.equals(that.artifacts)) return false;
if (!environment.equals(that.environment)) return false;
if (!serviceRole.equals(that.serviceRole)) return false;
if (!source.equals(that.source)) return false;
if (this.badgeEnabled != null ? !this.badgeEnabled.equals(that.badgeEnabled) : that.badgeEnabled != null) return false;
if (this.buildBatchConfig != null ? !this.buildBatchConfig.equals(that.buildBatchConfig) : that.buildBatchConfig != null) return false;
if (this.cache != null ? !this.cache.equals(that.cache) : that.cache != null) return false;
if (this.concurrentBuildLimit != null ? !this.concurrentBuildLimit.equals(that.concurrentBuildLimit) : that.concurrentBuildLimit != null) return false;
if (this.description != null ? !this.description.equals(that.description) : that.description != null) return false;
if (this.encryptionKey != null ? !this.encryptionKey.equals(that.encryptionKey) : that.encryptionKey != null) return false;
if (this.fileSystemLocations != null ? !this.fileSystemLocations.equals(that.fileSystemLocations) : that.fileSystemLocations != null) return false;
if (this.logsConfig != null ? !this.logsConfig.equals(that.logsConfig) : that.logsConfig != null) return false;
if (this.name != null ? !this.name.equals(that.name) : that.name != null) return false;
if (this.queuedTimeoutInMinutes != null ? !this.queuedTimeoutInMinutes.equals(that.queuedTimeoutInMinutes) : that.queuedTimeoutInMinutes != null) return false;
if (this.resourceAccessRole != null ? !this.resourceAccessRole.equals(that.resourceAccessRole) : that.resourceAccessRole != null) return false;
if (this.secondaryArtifacts != null ? !this.secondaryArtifacts.equals(that.secondaryArtifacts) : that.secondaryArtifacts != null) return false;
if (this.secondarySources != null ? !this.secondarySources.equals(that.secondarySources) : that.secondarySources != null) return false;
if (this.secondarySourceVersions != null ? !this.secondarySourceVersions.equals(that.secondarySourceVersions) : that.secondarySourceVersions != null) return false;
if (this.sourceVersion != null ? !this.sourceVersion.equals(that.sourceVersion) : that.sourceVersion != null) return false;
if (this.tags != null ? !this.tags.equals(that.tags) : that.tags != null) return false;
if (this.timeoutInMinutes != null ? !this.timeoutInMinutes.equals(that.timeoutInMinutes) : that.timeoutInMinutes != null) return false;
if (this.triggers != null ? !this.triggers.equals(that.triggers) : that.triggers != null) return false;
if (this.visibility != null ? !this.visibility.equals(that.visibility) : that.visibility != null) return false;
return this.vpcConfig != null ? this.vpcConfig.equals(that.vpcConfig) : that.vpcConfig == null;
}
@Override
public final int hashCode() {
int result = this.artifacts.hashCode();
result = 31 * result + (this.environment.hashCode());
result = 31 * result + (this.serviceRole.hashCode());
result = 31 * result + (this.source.hashCode());
result = 31 * result + (this.badgeEnabled != null ? this.badgeEnabled.hashCode() : 0);
result = 31 * result + (this.buildBatchConfig != null ? this.buildBatchConfig.hashCode() : 0);
result = 31 * result + (this.cache != null ? this.cache.hashCode() : 0);
result = 31 * result + (this.concurrentBuildLimit != null ? this.concurrentBuildLimit.hashCode() : 0);
result = 31 * result + (this.description != null ? this.description.hashCode() : 0);
result = 31 * result + (this.encryptionKey != null ? this.encryptionKey.hashCode() : 0);
result = 31 * result + (this.fileSystemLocations != null ? this.fileSystemLocations.hashCode() : 0);
result = 31 * result + (this.logsConfig != null ? this.logsConfig.hashCode() : 0);
result = 31 * result + (this.name != null ? this.name.hashCode() : 0);
result = 31 * result + (this.queuedTimeoutInMinutes != null ? this.queuedTimeoutInMinutes.hashCode() : 0);
result = 31 * result + (this.resourceAccessRole != null ? this.resourceAccessRole.hashCode() : 0);
result = 31 * result + (this.secondaryArtifacts != null ? this.secondaryArtifacts.hashCode() : 0);
result = 31 * result + (this.secondarySources != null ? this.secondarySources.hashCode() : 0);
result = 31 * result + (this.secondarySourceVersions != null ? this.secondarySourceVersions.hashCode() : 0);
result = 31 * result + (this.sourceVersion != null ? this.sourceVersion.hashCode() : 0);
result = 31 * result + (this.tags != null ? this.tags.hashCode() : 0);
result = 31 * result + (this.timeoutInMinutes != null ? this.timeoutInMinutes.hashCode() : 0);
result = 31 * result + (this.triggers != null ? this.triggers.hashCode() : 0);
result = 31 * result + (this.visibility != null ? this.visibility.hashCode() : 0);
result = 31 * result + (this.vpcConfig != null ? this.vpcConfig.hashCode() : 0);
return result;
}
}
}