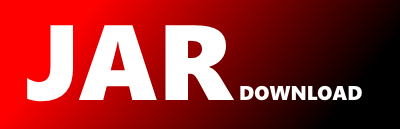
software.amazon.awscdk.services.codepipeline.actions.CloudFormationCreateUpdateStackActionProps Maven / Gradle / Ivy
Show all versions of codepipeline-actions Show documentation
package software.amazon.awscdk.services.codepipeline.actions;
/**
* Properties for the CloudFormationCreateUpdateStackAction.
*
* Example:
*
*
* import software.amazon.awscdk.core.PhysicalName;
* // in stack for account 123456789012...
* Stack otherAccountStack;
* Role actionRole = Role.Builder.create(otherAccountStack, "ActionRole")
* .assumedBy(new AccountPrincipal("123456789012"))
* // the role has to have a physical name set
* .roleName(PhysicalName.GENERATE_IF_NEEDED)
* .build();
* // in the pipeline stack...
* Artifact sourceOutput = new Artifact();
* CloudFormationCreateUpdateStackAction.Builder.create()
* .actionName("CloudFormationCreateUpdate")
* .stackName("MyStackName")
* .adminPermissions(true)
* .templatePath(sourceOutput.atPath("template.yaml"))
* .role(actionRole)
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.74.0 (build 6d08790)", date = "2023-05-19T23:09:34.804Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.codepipeline.actions.$Module.class, fqn = "@aws-cdk/aws-codepipeline-actions.CloudFormationCreateUpdateStackActionProps")
@software.amazon.jsii.Jsii.Proxy(CloudFormationCreateUpdateStackActionProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface CloudFormationCreateUpdateStackActionProps extends software.amazon.jsii.JsiiSerializable, software.amazon.awscdk.services.codepipeline.CommonAwsActionProps {
/**
* Whether to grant full permissions to CloudFormation while deploying this template.
*
* Setting this to true
affects the defaults for role
and capabilities
, if you
* don't specify any alternatives.
*
* The default role that will be created for you will have full (i.e., *
)
* permissions on all resources, and the deployment will have named IAM
* capabilities (i.e., able to create all IAM resources).
*
* This is a shorthand that you can use if you fully trust the templates that
* are deployed in this pipeline. If you want more fine-grained permissions,
* use addToRolePolicy
and capabilities
to control what the CloudFormation
* deployment is allowed to do.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.Boolean getAdminPermissions();
/**
* The name of the stack to apply this action to.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getStackName();
/**
* Input artifact with the CloudFormation template to deploy.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull software.amazon.awscdk.services.codepipeline.ArtifactPath getTemplatePath();
/**
* The AWS account this Action is supposed to operate in.
*
* Note: if you specify the role
property,
* this is ignored - the action will operate in the same region the passed role does.
*
* Default: - action resides in the same account as the pipeline
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getAccount() {
return null;
}
/**
* (deprecated) Acknowledge certain changes made as part of deployment.
*
* For stacks that contain certain resources, explicit acknowledgement that AWS CloudFormation
* might create or update those resources. For example, you must specify AnonymousIAM
or NamedIAM
* if your stack template contains AWS Identity and Access Management (IAM) resources. For more
* information see the link below.
*
* Default: None, unless `adminPermissions` is true
*
* @see https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/using-iam-template.html#using-iam-capabilities
* @deprecated use {@link cfnCapabilities} instead
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Deprecated)
@Deprecated
default @org.jetbrains.annotations.Nullable java.util.List getCapabilities() {
return null;
}
/**
* Acknowledge certain changes made as part of deployment.
*
* For stacks that contain certain resources,
* explicit acknowledgement is required that AWS CloudFormation might create or update those resources.
* For example, you must specify ANONYMOUS_IAM
or NAMED_IAM
if your stack template contains AWS
* Identity and Access Management (IAM) resources.
* For more information, see the link below.
*
* Default: None, unless `adminPermissions` is true
*
* @see https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/using-iam-template.html#using-iam-capabilities
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getCfnCapabilities() {
return null;
}
/**
* IAM role to assume when deploying changes.
*
* If not specified, a fresh role is created. The role is created with zero
* permissions unless adminPermissions
is true, in which case the role will have
* full permissions.
*
* Default: A fresh role with full or no permissions (depending on the value of `adminPermissions`).
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.iam.IRole getDeploymentRole() {
return null;
}
/**
* The list of additional input Artifacts for this Action.
*
* This is especially useful when used in conjunction with the parameterOverrides
property.
* For example, if you have:
*
* parameterOverrides: {
* 'Param1': action1.outputArtifact.bucketName,
* 'Param2': action2.outputArtifact.objectKey,
* }
*
* , if the output Artifacts of action1
and action2
were not used to
* set either the templateConfiguration
or the templatePath
properties,
* you need to make sure to include them in the extraInputs
-
* otherwise, you'll get an "unrecognized Artifact" error during your Pipeline's execution.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getExtraInputs() {
return null;
}
/**
* The name of the output artifact to generate.
*
* Only applied if outputFileName
is set as well.
*
* Default: Automatically generated artifact name.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.codepipeline.Artifact getOutput() {
return null;
}
/**
* A name for the filename in the output artifact to store the AWS CloudFormation call's result.
*
* The file will contain the result of the call to AWS CloudFormation (for example
* the call to UpdateStack or CreateChangeSet).
*
* AWS CodePipeline adds the file to the output artifact after performing
* the specified action.
*
* Default: No output artifact generated
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getOutputFileName() {
return null;
}
/**
* Additional template parameters.
*
* Template parameters specified here take precedence over template parameters
* found in the artifact specified by the templateConfiguration
property.
*
* We recommend that you use the template configuration file to specify
* most of your parameter values. Use parameter overrides to specify only
* dynamic parameter values (values that are unknown until you run the
* pipeline).
*
* All parameter names must be present in the stack template.
*
* Note: the entire object cannot be more than 1kB.
*
* Default: No overrides
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.Map getParameterOverrides() {
return null;
}
/**
* The AWS region the given Action resides in.
*
* Note that a cross-region Pipeline requires replication buckets to function correctly.
* You can provide their names with the {@link PipelineProps#crossRegionReplicationBuckets} property.
* If you don't, the CodePipeline Construct will create new Stacks in your CDK app containing those buckets,
* that you will need to cdk deploy
before deploying the main, Pipeline-containing Stack.
*
* Default: the Action resides in the same region as the Pipeline
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getRegion() {
return null;
}
/**
* Replace the stack if it's in a failed state.
*
* If this is set to true and the stack is in a failed state (one of
* ROLLBACK_COMPLETE, ROLLBACK_FAILED, CREATE_FAILED, DELETE_FAILED, or
* UPDATE_ROLLBACK_FAILED), AWS CloudFormation deletes the stack and then
* creates a new stack.
*
* If this is not set to true and the stack is in a failed state,
* the deployment fails.
*
* Default: false
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Boolean getReplaceOnFailure() {
return null;
}
/**
* Input artifact to use for template parameters values and stack policy.
*
* The template configuration file should contain a JSON object that should look like this:
* { "Parameters": {...}, "Tags": {...}, "StackPolicy": {... }}
. For more information,
* see AWS CloudFormation Artifacts.
*
* Note that if you include sensitive information, such as passwords, restrict access to this
* file.
*
* Default: No template configuration based on input artifacts
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.codepipeline.ArtifactPath getTemplateConfiguration() {
return null;
}
/**
* @return a {@link Builder} of {@link CloudFormationCreateUpdateStackActionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link CloudFormationCreateUpdateStackActionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.Boolean adminPermissions;
java.lang.String stackName;
software.amazon.awscdk.services.codepipeline.ArtifactPath templatePath;
java.lang.String account;
java.util.List capabilities;
java.util.List cfnCapabilities;
software.amazon.awscdk.services.iam.IRole deploymentRole;
java.util.List extraInputs;
software.amazon.awscdk.services.codepipeline.Artifact output;
java.lang.String outputFileName;
java.util.Map parameterOverrides;
java.lang.String region;
java.lang.Boolean replaceOnFailure;
software.amazon.awscdk.services.codepipeline.ArtifactPath templateConfiguration;
software.amazon.awscdk.services.iam.IRole role;
java.lang.String actionName;
java.lang.Number runOrder;
java.lang.String variablesNamespace;
/**
* Sets the value of {@link CloudFormationCreateUpdateStackActionProps#getAdminPermissions}
* @param adminPermissions Whether to grant full permissions to CloudFormation while deploying this template. This parameter is required.
* Setting this to true
affects the defaults for role
and capabilities
, if you
* don't specify any alternatives.
*
* The default role that will be created for you will have full (i.e., *
)
* permissions on all resources, and the deployment will have named IAM
* capabilities (i.e., able to create all IAM resources).
*
* This is a shorthand that you can use if you fully trust the templates that
* are deployed in this pipeline. If you want more fine-grained permissions,
* use addToRolePolicy
and capabilities
to control what the CloudFormation
* deployment is allowed to do.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder adminPermissions(java.lang.Boolean adminPermissions) {
this.adminPermissions = adminPermissions;
return this;
}
/**
* Sets the value of {@link CloudFormationCreateUpdateStackActionProps#getStackName}
* @param stackName The name of the stack to apply this action to. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder stackName(java.lang.String stackName) {
this.stackName = stackName;
return this;
}
/**
* Sets the value of {@link CloudFormationCreateUpdateStackActionProps#getTemplatePath}
* @param templatePath Input artifact with the CloudFormation template to deploy. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder templatePath(software.amazon.awscdk.services.codepipeline.ArtifactPath templatePath) {
this.templatePath = templatePath;
return this;
}
/**
* Sets the value of {@link CloudFormationCreateUpdateStackActionProps#getAccount}
* @param account The AWS account this Action is supposed to operate in.
* Note: if you specify the role
property,
* this is ignored - the action will operate in the same region the passed role does.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder account(java.lang.String account) {
this.account = account;
return this;
}
/**
* Sets the value of {@link CloudFormationCreateUpdateStackActionProps#getCapabilities}
* @param capabilities Acknowledge certain changes made as part of deployment.
* For stacks that contain certain resources, explicit acknowledgement that AWS CloudFormation
* might create or update those resources. For example, you must specify AnonymousIAM
or NamedIAM
* if your stack template contains AWS Identity and Access Management (IAM) resources. For more
* information see the link below.
* @return {@code this}
* @deprecated use {@link cfnCapabilities} instead
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Deprecated)
@Deprecated
@SuppressWarnings("unchecked")
public Builder capabilities(java.util.List extends software.amazon.awscdk.services.cloudformation.CloudFormationCapabilities> capabilities) {
this.capabilities = (java.util.List)capabilities;
return this;
}
/**
* Sets the value of {@link CloudFormationCreateUpdateStackActionProps#getCfnCapabilities}
* @param cfnCapabilities Acknowledge certain changes made as part of deployment.
* For stacks that contain certain resources,
* explicit acknowledgement is required that AWS CloudFormation might create or update those resources.
* For example, you must specify ANONYMOUS_IAM
or NAMED_IAM
if your stack template contains AWS
* Identity and Access Management (IAM) resources.
* For more information, see the link below.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@SuppressWarnings("unchecked")
public Builder cfnCapabilities(java.util.List extends software.amazon.awscdk.core.CfnCapabilities> cfnCapabilities) {
this.cfnCapabilities = (java.util.List)cfnCapabilities;
return this;
}
/**
* Sets the value of {@link CloudFormationCreateUpdateStackActionProps#getDeploymentRole}
* @param deploymentRole IAM role to assume when deploying changes.
* If not specified, a fresh role is created. The role is created with zero
* permissions unless adminPermissions
is true, in which case the role will have
* full permissions.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder deploymentRole(software.amazon.awscdk.services.iam.IRole deploymentRole) {
this.deploymentRole = deploymentRole;
return this;
}
/**
* Sets the value of {@link CloudFormationCreateUpdateStackActionProps#getExtraInputs}
* @param extraInputs The list of additional input Artifacts for this Action.
* This is especially useful when used in conjunction with the parameterOverrides
property.
* For example, if you have:
*
* parameterOverrides: {
* 'Param1': action1.outputArtifact.bucketName,
* 'Param2': action2.outputArtifact.objectKey,
* }
*
* , if the output Artifacts of action1
and action2
were not used to
* set either the templateConfiguration
or the templatePath
properties,
* you need to make sure to include them in the extraInputs
-
* otherwise, you'll get an "unrecognized Artifact" error during your Pipeline's execution.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@SuppressWarnings("unchecked")
public Builder extraInputs(java.util.List extends software.amazon.awscdk.services.codepipeline.Artifact> extraInputs) {
this.extraInputs = (java.util.List)extraInputs;
return this;
}
/**
* Sets the value of {@link CloudFormationCreateUpdateStackActionProps#getOutput}
* @param output The name of the output artifact to generate.
* Only applied if outputFileName
is set as well.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder output(software.amazon.awscdk.services.codepipeline.Artifact output) {
this.output = output;
return this;
}
/**
* Sets the value of {@link CloudFormationCreateUpdateStackActionProps#getOutputFileName}
* @param outputFileName A name for the filename in the output artifact to store the AWS CloudFormation call's result.
* The file will contain the result of the call to AWS CloudFormation (for example
* the call to UpdateStack or CreateChangeSet).
*
* AWS CodePipeline adds the file to the output artifact after performing
* the specified action.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder outputFileName(java.lang.String outputFileName) {
this.outputFileName = outputFileName;
return this;
}
/**
* Sets the value of {@link CloudFormationCreateUpdateStackActionProps#getParameterOverrides}
* @param parameterOverrides Additional template parameters.
* Template parameters specified here take precedence over template parameters
* found in the artifact specified by the templateConfiguration
property.
*
* We recommend that you use the template configuration file to specify
* most of your parameter values. Use parameter overrides to specify only
* dynamic parameter values (values that are unknown until you run the
* pipeline).
*
* All parameter names must be present in the stack template.
*
* Note: the entire object cannot be more than 1kB.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@SuppressWarnings("unchecked")
public Builder parameterOverrides(java.util.Map parameterOverrides) {
this.parameterOverrides = (java.util.Map)parameterOverrides;
return this;
}
/**
* Sets the value of {@link CloudFormationCreateUpdateStackActionProps#getRegion}
* @param region The AWS region the given Action resides in.
* Note that a cross-region Pipeline requires replication buckets to function correctly.
* You can provide their names with the {@link PipelineProps#crossRegionReplicationBuckets} property.
* If you don't, the CodePipeline Construct will create new Stacks in your CDK app containing those buckets,
* that you will need to cdk deploy
before deploying the main, Pipeline-containing Stack.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder region(java.lang.String region) {
this.region = region;
return this;
}
/**
* Sets the value of {@link CloudFormationCreateUpdateStackActionProps#getReplaceOnFailure}
* @param replaceOnFailure Replace the stack if it's in a failed state.
* If this is set to true and the stack is in a failed state (one of
* ROLLBACK_COMPLETE, ROLLBACK_FAILED, CREATE_FAILED, DELETE_FAILED, or
* UPDATE_ROLLBACK_FAILED), AWS CloudFormation deletes the stack and then
* creates a new stack.
*
* If this is not set to true and the stack is in a failed state,
* the deployment fails.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder replaceOnFailure(java.lang.Boolean replaceOnFailure) {
this.replaceOnFailure = replaceOnFailure;
return this;
}
/**
* Sets the value of {@link CloudFormationCreateUpdateStackActionProps#getTemplateConfiguration}
* @param templateConfiguration Input artifact to use for template parameters values and stack policy.
* The template configuration file should contain a JSON object that should look like this:
* { "Parameters": {...}, "Tags": {...}, "StackPolicy": {... }}
. For more information,
* see AWS CloudFormation Artifacts.
*
* Note that if you include sensitive information, such as passwords, restrict access to this
* file.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder templateConfiguration(software.amazon.awscdk.services.codepipeline.ArtifactPath templateConfiguration) {
this.templateConfiguration = templateConfiguration;
return this;
}
/**
* Sets the value of {@link CloudFormationCreateUpdateStackActionProps#getRole}
* @param role The Role in which context's this Action will be executing in.
* The Pipeline's Role will assume this Role
* (the required permissions for that will be granted automatically)
* right before executing this Action.
* This Action will be passed into your {@link IAction.bind}
* method in the {@link ActionBindOptions.role} property.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder role(software.amazon.awscdk.services.iam.IRole role) {
this.role = role;
return this;
}
/**
* Sets the value of {@link CloudFormationCreateUpdateStackActionProps#getActionName}
* @param actionName The physical, human-readable name of the Action. This parameter is required.
* Note that Action names must be unique within a single Stage.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder actionName(java.lang.String actionName) {
this.actionName = actionName;
return this;
}
/**
* Sets the value of {@link CloudFormationCreateUpdateStackActionProps#getRunOrder}
* @param runOrder The runOrder property for this Action.
* RunOrder determines the relative order in which multiple Actions in the same Stage execute.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder runOrder(java.lang.Number runOrder) {
this.runOrder = runOrder;
return this;
}
/**
* Sets the value of {@link CloudFormationCreateUpdateStackActionProps#getVariablesNamespace}
* @param variablesNamespace The name of the namespace to use for variables emitted by this action.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder variablesNamespace(java.lang.String variablesNamespace) {
this.variablesNamespace = variablesNamespace;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link CloudFormationCreateUpdateStackActionProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public CloudFormationCreateUpdateStackActionProps build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link CloudFormationCreateUpdateStackActionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements CloudFormationCreateUpdateStackActionProps {
private final java.lang.Boolean adminPermissions;
private final java.lang.String stackName;
private final software.amazon.awscdk.services.codepipeline.ArtifactPath templatePath;
private final java.lang.String account;
private final java.util.List capabilities;
private final java.util.List cfnCapabilities;
private final software.amazon.awscdk.services.iam.IRole deploymentRole;
private final java.util.List extraInputs;
private final software.amazon.awscdk.services.codepipeline.Artifact output;
private final java.lang.String outputFileName;
private final java.util.Map parameterOverrides;
private final java.lang.String region;
private final java.lang.Boolean replaceOnFailure;
private final software.amazon.awscdk.services.codepipeline.ArtifactPath templateConfiguration;
private final software.amazon.awscdk.services.iam.IRole role;
private final java.lang.String actionName;
private final java.lang.Number runOrder;
private final java.lang.String variablesNamespace;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.adminPermissions = software.amazon.jsii.Kernel.get(this, "adminPermissions", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.stackName = software.amazon.jsii.Kernel.get(this, "stackName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.templatePath = software.amazon.jsii.Kernel.get(this, "templatePath", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.codepipeline.ArtifactPath.class));
this.account = software.amazon.jsii.Kernel.get(this, "account", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.capabilities = software.amazon.jsii.Kernel.get(this, "capabilities", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.cloudformation.CloudFormationCapabilities.class)));
this.cfnCapabilities = software.amazon.jsii.Kernel.get(this, "cfnCapabilities", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.core.CfnCapabilities.class)));
this.deploymentRole = software.amazon.jsii.Kernel.get(this, "deploymentRole", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.iam.IRole.class));
this.extraInputs = software.amazon.jsii.Kernel.get(this, "extraInputs", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.codepipeline.Artifact.class)));
this.output = software.amazon.jsii.Kernel.get(this, "output", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.codepipeline.Artifact.class));
this.outputFileName = software.amazon.jsii.Kernel.get(this, "outputFileName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.parameterOverrides = software.amazon.jsii.Kernel.get(this, "parameterOverrides", software.amazon.jsii.NativeType.mapOf(software.amazon.jsii.NativeType.forClass(java.lang.Object.class)));
this.region = software.amazon.jsii.Kernel.get(this, "region", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.replaceOnFailure = software.amazon.jsii.Kernel.get(this, "replaceOnFailure", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.templateConfiguration = software.amazon.jsii.Kernel.get(this, "templateConfiguration", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.codepipeline.ArtifactPath.class));
this.role = software.amazon.jsii.Kernel.get(this, "role", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.iam.IRole.class));
this.actionName = software.amazon.jsii.Kernel.get(this, "actionName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.runOrder = software.amazon.jsii.Kernel.get(this, "runOrder", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.variablesNamespace = software.amazon.jsii.Kernel.get(this, "variablesNamespace", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
@SuppressWarnings("unchecked")
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.adminPermissions = java.util.Objects.requireNonNull(builder.adminPermissions, "adminPermissions is required");
this.stackName = java.util.Objects.requireNonNull(builder.stackName, "stackName is required");
this.templatePath = java.util.Objects.requireNonNull(builder.templatePath, "templatePath is required");
this.account = builder.account;
this.capabilities = (java.util.List)builder.capabilities;
this.cfnCapabilities = (java.util.List)builder.cfnCapabilities;
this.deploymentRole = builder.deploymentRole;
this.extraInputs = (java.util.List)builder.extraInputs;
this.output = builder.output;
this.outputFileName = builder.outputFileName;
this.parameterOverrides = (java.util.Map)builder.parameterOverrides;
this.region = builder.region;
this.replaceOnFailure = builder.replaceOnFailure;
this.templateConfiguration = builder.templateConfiguration;
this.role = builder.role;
this.actionName = java.util.Objects.requireNonNull(builder.actionName, "actionName is required");
this.runOrder = builder.runOrder;
this.variablesNamespace = builder.variablesNamespace;
}
@Override
public final java.lang.Boolean getAdminPermissions() {
return this.adminPermissions;
}
@Override
public final java.lang.String getStackName() {
return this.stackName;
}
@Override
public final software.amazon.awscdk.services.codepipeline.ArtifactPath getTemplatePath() {
return this.templatePath;
}
@Override
public final java.lang.String getAccount() {
return this.account;
}
@Override
public final java.util.List getCapabilities() {
return this.capabilities;
}
@Override
public final java.util.List getCfnCapabilities() {
return this.cfnCapabilities;
}
@Override
public final software.amazon.awscdk.services.iam.IRole getDeploymentRole() {
return this.deploymentRole;
}
@Override
public final java.util.List getExtraInputs() {
return this.extraInputs;
}
@Override
public final software.amazon.awscdk.services.codepipeline.Artifact getOutput() {
return this.output;
}
@Override
public final java.lang.String getOutputFileName() {
return this.outputFileName;
}
@Override
public final java.util.Map getParameterOverrides() {
return this.parameterOverrides;
}
@Override
public final java.lang.String getRegion() {
return this.region;
}
@Override
public final java.lang.Boolean getReplaceOnFailure() {
return this.replaceOnFailure;
}
@Override
public final software.amazon.awscdk.services.codepipeline.ArtifactPath getTemplateConfiguration() {
return this.templateConfiguration;
}
@Override
public final software.amazon.awscdk.services.iam.IRole getRole() {
return this.role;
}
@Override
public final java.lang.String getActionName() {
return this.actionName;
}
@Override
public final java.lang.Number getRunOrder() {
return this.runOrder;
}
@Override
public final java.lang.String getVariablesNamespace() {
return this.variablesNamespace;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("adminPermissions", om.valueToTree(this.getAdminPermissions()));
data.set("stackName", om.valueToTree(this.getStackName()));
data.set("templatePath", om.valueToTree(this.getTemplatePath()));
if (this.getAccount() != null) {
data.set("account", om.valueToTree(this.getAccount()));
}
if (this.getCapabilities() != null) {
data.set("capabilities", om.valueToTree(this.getCapabilities()));
}
if (this.getCfnCapabilities() != null) {
data.set("cfnCapabilities", om.valueToTree(this.getCfnCapabilities()));
}
if (this.getDeploymentRole() != null) {
data.set("deploymentRole", om.valueToTree(this.getDeploymentRole()));
}
if (this.getExtraInputs() != null) {
data.set("extraInputs", om.valueToTree(this.getExtraInputs()));
}
if (this.getOutput() != null) {
data.set("output", om.valueToTree(this.getOutput()));
}
if (this.getOutputFileName() != null) {
data.set("outputFileName", om.valueToTree(this.getOutputFileName()));
}
if (this.getParameterOverrides() != null) {
data.set("parameterOverrides", om.valueToTree(this.getParameterOverrides()));
}
if (this.getRegion() != null) {
data.set("region", om.valueToTree(this.getRegion()));
}
if (this.getReplaceOnFailure() != null) {
data.set("replaceOnFailure", om.valueToTree(this.getReplaceOnFailure()));
}
if (this.getTemplateConfiguration() != null) {
data.set("templateConfiguration", om.valueToTree(this.getTemplateConfiguration()));
}
if (this.getRole() != null) {
data.set("role", om.valueToTree(this.getRole()));
}
data.set("actionName", om.valueToTree(this.getActionName()));
if (this.getRunOrder() != null) {
data.set("runOrder", om.valueToTree(this.getRunOrder()));
}
if (this.getVariablesNamespace() != null) {
data.set("variablesNamespace", om.valueToTree(this.getVariablesNamespace()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-codepipeline-actions.CloudFormationCreateUpdateStackActionProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
CloudFormationCreateUpdateStackActionProps.Jsii$Proxy that = (CloudFormationCreateUpdateStackActionProps.Jsii$Proxy) o;
if (!adminPermissions.equals(that.adminPermissions)) return false;
if (!stackName.equals(that.stackName)) return false;
if (!templatePath.equals(that.templatePath)) return false;
if (this.account != null ? !this.account.equals(that.account) : that.account != null) return false;
if (this.capabilities != null ? !this.capabilities.equals(that.capabilities) : that.capabilities != null) return false;
if (this.cfnCapabilities != null ? !this.cfnCapabilities.equals(that.cfnCapabilities) : that.cfnCapabilities != null) return false;
if (this.deploymentRole != null ? !this.deploymentRole.equals(that.deploymentRole) : that.deploymentRole != null) return false;
if (this.extraInputs != null ? !this.extraInputs.equals(that.extraInputs) : that.extraInputs != null) return false;
if (this.output != null ? !this.output.equals(that.output) : that.output != null) return false;
if (this.outputFileName != null ? !this.outputFileName.equals(that.outputFileName) : that.outputFileName != null) return false;
if (this.parameterOverrides != null ? !this.parameterOverrides.equals(that.parameterOverrides) : that.parameterOverrides != null) return false;
if (this.region != null ? !this.region.equals(that.region) : that.region != null) return false;
if (this.replaceOnFailure != null ? !this.replaceOnFailure.equals(that.replaceOnFailure) : that.replaceOnFailure != null) return false;
if (this.templateConfiguration != null ? !this.templateConfiguration.equals(that.templateConfiguration) : that.templateConfiguration != null) return false;
if (this.role != null ? !this.role.equals(that.role) : that.role != null) return false;
if (!actionName.equals(that.actionName)) return false;
if (this.runOrder != null ? !this.runOrder.equals(that.runOrder) : that.runOrder != null) return false;
return this.variablesNamespace != null ? this.variablesNamespace.equals(that.variablesNamespace) : that.variablesNamespace == null;
}
@Override
public final int hashCode() {
int result = this.adminPermissions.hashCode();
result = 31 * result + (this.stackName.hashCode());
result = 31 * result + (this.templatePath.hashCode());
result = 31 * result + (this.account != null ? this.account.hashCode() : 0);
result = 31 * result + (this.capabilities != null ? this.capabilities.hashCode() : 0);
result = 31 * result + (this.cfnCapabilities != null ? this.cfnCapabilities.hashCode() : 0);
result = 31 * result + (this.deploymentRole != null ? this.deploymentRole.hashCode() : 0);
result = 31 * result + (this.extraInputs != null ? this.extraInputs.hashCode() : 0);
result = 31 * result + (this.output != null ? this.output.hashCode() : 0);
result = 31 * result + (this.outputFileName != null ? this.outputFileName.hashCode() : 0);
result = 31 * result + (this.parameterOverrides != null ? this.parameterOverrides.hashCode() : 0);
result = 31 * result + (this.region != null ? this.region.hashCode() : 0);
result = 31 * result + (this.replaceOnFailure != null ? this.replaceOnFailure.hashCode() : 0);
result = 31 * result + (this.templateConfiguration != null ? this.templateConfiguration.hashCode() : 0);
result = 31 * result + (this.role != null ? this.role.hashCode() : 0);
result = 31 * result + (this.actionName.hashCode());
result = 31 * result + (this.runOrder != null ? this.runOrder.hashCode() : 0);
result = 31 * result + (this.variablesNamespace != null ? this.variablesNamespace.hashCode() : 0);
return result;
}
}
}