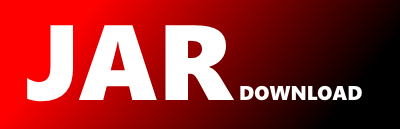
software.amazon.awscdk.services.codepipeline.actions.CloudFormationDeleteStackAction Maven / Gradle / Ivy
Show all versions of codepipeline-actions Show documentation
package software.amazon.awscdk.services.codepipeline.actions;
/**
* CodePipeline action to delete a stack.
*
* Deletes a stack. If you specify a stack that doesn't exist, the action completes successfully
* without deleting a stack.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.cloudformation.*;
* import software.amazon.awscdk.services.codepipeline.*;
* import software.amazon.awscdk.services.codepipeline.actions.*;
* import software.amazon.awscdk.services.iam.*;
* import software.amazon.awscdk.core.*;
* Artifact artifact;
* ArtifactPath artifactPath;
* Object parameterOverrides;
* Role role;
* CloudFormationDeleteStackAction cloudFormationDeleteStackAction = CloudFormationDeleteStackAction.Builder.create()
* .actionName("actionName")
* .adminPermissions(false)
* .stackName("stackName")
* // the properties below are optional
* .account("account")
* .capabilities(List.of(CloudFormationCapabilities.NONE))
* .cfnCapabilities(List.of(CfnCapabilities.NONE))
* .deploymentRole(role)
* .extraInputs(List.of(artifact))
* .output(artifact)
* .outputFileName("outputFileName")
* .parameterOverrides(Map.of(
* "parameterOverridesKey", parameterOverrides))
* .region("region")
* .role(role)
* .runOrder(123)
* .templateConfiguration(artifactPath)
* .variablesNamespace("variablesNamespace")
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.74.0 (build 6d08790)", date = "2023-05-19T23:09:34.807Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.codepipeline.actions.$Module.class, fqn = "@aws-cdk/aws-codepipeline-actions.CloudFormationDeleteStackAction")
public class CloudFormationDeleteStackAction extends software.amazon.awscdk.services.codepipeline.actions.Action {
protected CloudFormationDeleteStackAction(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected CloudFormationDeleteStackAction(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
/**
* @param props This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public CloudFormationDeleteStackAction(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.codepipeline.actions.CloudFormationDeleteStackActionProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* Add statement to the service role assumed by CloudFormation while executing this action.
*
* @param statement This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.Boolean addToDeploymentRolePolicy(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.iam.PolicyStatement statement) {
return software.amazon.jsii.Kernel.call(this, "addToDeploymentRolePolicy", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class), new Object[] { java.util.Objects.requireNonNull(statement, "statement is required") });
}
/**
* This is a renamed version of the {@link IAction.bind} method.
*
* @param scope This parameter is required.
* @param stage This parameter is required.
* @param options This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
protected @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.codepipeline.ActionConfig bound(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.Construct scope, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.codepipeline.IStage stage, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.codepipeline.ActionBindOptions options) {
return software.amazon.jsii.Kernel.call(this, "bound", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.codepipeline.ActionConfig.class), new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(stage, "stage is required"), java.util.Objects.requireNonNull(options, "options is required") });
}
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.iam.IRole getDeploymentRole() {
return software.amazon.jsii.Kernel.get(this, "deploymentRole", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.iam.IRole.class));
}
/**
* A fluent builder for {@link software.amazon.awscdk.services.codepipeline.actions.CloudFormationDeleteStackAction}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static Builder create() {
return new Builder();
}
private final software.amazon.awscdk.services.codepipeline.actions.CloudFormationDeleteStackActionProps.Builder props;
private Builder() {
this.props = new software.amazon.awscdk.services.codepipeline.actions.CloudFormationDeleteStackActionProps.Builder();
}
/**
* The physical, human-readable name of the Action.
*
* Note that Action names must be unique within a single Stage.
*
* @return {@code this}
* @param actionName The physical, human-readable name of the Action. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder actionName(final java.lang.String actionName) {
this.props.actionName(actionName);
return this;
}
/**
* The runOrder property for this Action.
*
* RunOrder determines the relative order in which multiple Actions in the same Stage execute.
*
* Default: 1
*
* @return {@code this}
* @see https://docs.aws.amazon.com/codepipeline/latest/userguide/reference-pipeline-structure.html
* @param runOrder The runOrder property for this Action. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder runOrder(final java.lang.Number runOrder) {
this.props.runOrder(runOrder);
return this;
}
/**
* The name of the namespace to use for variables emitted by this action.
*
* Default: - a name will be generated, based on the stage and action names,
* if any of the action's variables were referenced - otherwise,
* no namespace will be set
*
* @return {@code this}
* @param variablesNamespace The name of the namespace to use for variables emitted by this action. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder variablesNamespace(final java.lang.String variablesNamespace) {
this.props.variablesNamespace(variablesNamespace);
return this;
}
/**
* The Role in which context's this Action will be executing in.
*
* The Pipeline's Role will assume this Role
* (the required permissions for that will be granted automatically)
* right before executing this Action.
* This Action will be passed into your {@link IAction.bind}
* method in the {@link ActionBindOptions.role} property.
*
* Default: a new Role will be generated
*
* @return {@code this}
* @param role The Role in which context's this Action will be executing in. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder role(final software.amazon.awscdk.services.iam.IRole role) {
this.props.role(role);
return this;
}
/**
* Whether to grant full permissions to CloudFormation while deploying this template.
*
* Setting this to true
affects the defaults for role
and capabilities
, if you
* don't specify any alternatives.
*
* The default role that will be created for you will have full (i.e., *
)
* permissions on all resources, and the deployment will have named IAM
* capabilities (i.e., able to create all IAM resources).
*
* This is a shorthand that you can use if you fully trust the templates that
* are deployed in this pipeline. If you want more fine-grained permissions,
* use addToRolePolicy
and capabilities
to control what the CloudFormation
* deployment is allowed to do.
*
* @return {@code this}
* @param adminPermissions Whether to grant full permissions to CloudFormation while deploying this template. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder adminPermissions(final java.lang.Boolean adminPermissions) {
this.props.adminPermissions(adminPermissions);
return this;
}
/**
* The name of the stack to apply this action to.
*
* @return {@code this}
* @param stackName The name of the stack to apply this action to. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder stackName(final java.lang.String stackName) {
this.props.stackName(stackName);
return this;
}
/**
* The AWS account this Action is supposed to operate in.
*
* Note: if you specify the role
property,
* this is ignored - the action will operate in the same region the passed role does.
*
* Default: - action resides in the same account as the pipeline
*
* @return {@code this}
* @param account The AWS account this Action is supposed to operate in. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder account(final java.lang.String account) {
this.props.account(account);
return this;
}
/**
* (deprecated) Acknowledge certain changes made as part of deployment.
*
* For stacks that contain certain resources, explicit acknowledgement that AWS CloudFormation
* might create or update those resources. For example, you must specify AnonymousIAM
or NamedIAM
* if your stack template contains AWS Identity and Access Management (IAM) resources. For more
* information see the link below.
*
* Default: None, unless `adminPermissions` is true
*
* @return {@code this}
* @see https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/using-iam-template.html#using-iam-capabilities
* @deprecated use {@link cfnCapabilities} instead
* @param capabilities Acknowledge certain changes made as part of deployment. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Deprecated)
@Deprecated
public Builder capabilities(final java.util.List extends software.amazon.awscdk.services.cloudformation.CloudFormationCapabilities> capabilities) {
this.props.capabilities(capabilities);
return this;
}
/**
* Acknowledge certain changes made as part of deployment.
*
* For stacks that contain certain resources,
* explicit acknowledgement is required that AWS CloudFormation might create or update those resources.
* For example, you must specify ANONYMOUS_IAM
or NAMED_IAM
if your stack template contains AWS
* Identity and Access Management (IAM) resources.
* For more information, see the link below.
*
* Default: None, unless `adminPermissions` is true
*
* @return {@code this}
* @see https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/using-iam-template.html#using-iam-capabilities
* @param cfnCapabilities Acknowledge certain changes made as part of deployment. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder cfnCapabilities(final java.util.List extends software.amazon.awscdk.core.CfnCapabilities> cfnCapabilities) {
this.props.cfnCapabilities(cfnCapabilities);
return this;
}
/**
* IAM role to assume when deploying changes.
*
* If not specified, a fresh role is created. The role is created with zero
* permissions unless adminPermissions
is true, in which case the role will have
* full permissions.
*
* Default: A fresh role with full or no permissions (depending on the value of `adminPermissions`).
*
* @return {@code this}
* @param deploymentRole IAM role to assume when deploying changes. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder deploymentRole(final software.amazon.awscdk.services.iam.IRole deploymentRole) {
this.props.deploymentRole(deploymentRole);
return this;
}
/**
* The list of additional input Artifacts for this Action.
*
* This is especially useful when used in conjunction with the parameterOverrides
property.
* For example, if you have:
*
* parameterOverrides: {
* 'Param1': action1.outputArtifact.bucketName,
* 'Param2': action2.outputArtifact.objectKey,
* }
*
* , if the output Artifacts of action1
and action2
were not used to
* set either the templateConfiguration
or the templatePath
properties,
* you need to make sure to include them in the extraInputs
-
* otherwise, you'll get an "unrecognized Artifact" error during your Pipeline's execution.
*
* @return {@code this}
* @param extraInputs The list of additional input Artifacts for this Action. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder extraInputs(final java.util.List extends software.amazon.awscdk.services.codepipeline.Artifact> extraInputs) {
this.props.extraInputs(extraInputs);
return this;
}
/**
* The name of the output artifact to generate.
*
* Only applied if outputFileName
is set as well.
*
* Default: Automatically generated artifact name.
*
* @return {@code this}
* @param output The name of the output artifact to generate. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder output(final software.amazon.awscdk.services.codepipeline.Artifact output) {
this.props.output(output);
return this;
}
/**
* A name for the filename in the output artifact to store the AWS CloudFormation call's result.
*
* The file will contain the result of the call to AWS CloudFormation (for example
* the call to UpdateStack or CreateChangeSet).
*
* AWS CodePipeline adds the file to the output artifact after performing
* the specified action.
*
* Default: No output artifact generated
*
* @return {@code this}
* @param outputFileName A name for the filename in the output artifact to store the AWS CloudFormation call's result. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder outputFileName(final java.lang.String outputFileName) {
this.props.outputFileName(outputFileName);
return this;
}
/**
* Additional template parameters.
*
* Template parameters specified here take precedence over template parameters
* found in the artifact specified by the templateConfiguration
property.
*
* We recommend that you use the template configuration file to specify
* most of your parameter values. Use parameter overrides to specify only
* dynamic parameter values (values that are unknown until you run the
* pipeline).
*
* All parameter names must be present in the stack template.
*
* Note: the entire object cannot be more than 1kB.
*
* Default: No overrides
*
* @return {@code this}
* @param parameterOverrides Additional template parameters. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder parameterOverrides(final java.util.Map parameterOverrides) {
this.props.parameterOverrides(parameterOverrides);
return this;
}
/**
* The AWS region the given Action resides in.
*
* Note that a cross-region Pipeline requires replication buckets to function correctly.
* You can provide their names with the {@link PipelineProps#crossRegionReplicationBuckets} property.
* If you don't, the CodePipeline Construct will create new Stacks in your CDK app containing those buckets,
* that you will need to cdk deploy
before deploying the main, Pipeline-containing Stack.
*
* Default: the Action resides in the same region as the Pipeline
*
* @return {@code this}
* @param region The AWS region the given Action resides in. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder region(final java.lang.String region) {
this.props.region(region);
return this;
}
/**
* Input artifact to use for template parameters values and stack policy.
*
* The template configuration file should contain a JSON object that should look like this:
* { "Parameters": {...}, "Tags": {...}, "StackPolicy": {... }}
. For more information,
* see AWS CloudFormation Artifacts.
*
* Note that if you include sensitive information, such as passwords, restrict access to this
* file.
*
* Default: No template configuration based on input artifacts
*
* @return {@code this}
* @param templateConfiguration Input artifact to use for template parameters values and stack policy. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder templateConfiguration(final software.amazon.awscdk.services.codepipeline.ArtifactPath templateConfiguration) {
this.props.templateConfiguration(templateConfiguration);
return this;
}
/**
* @returns a newly built instance of {@link software.amazon.awscdk.services.codepipeline.actions.CloudFormationDeleteStackAction}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public software.amazon.awscdk.services.codepipeline.actions.CloudFormationDeleteStackAction build() {
return new software.amazon.awscdk.services.codepipeline.actions.CloudFormationDeleteStackAction(
this.props.build()
);
}
}
}