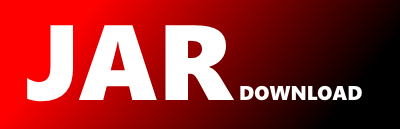
software.amazon.awscdk.services.codepipeline.actions.CloudFormationDeployStackInstancesAction Maven / Gradle / Ivy
Show all versions of codepipeline-actions Show documentation
package software.amazon.awscdk.services.codepipeline.actions;
/**
* CodePipeline action to create/update Stack Instances of a StackSet.
*
* After the initial creation of a stack set, you can add new stack instances by
* using CloudFormationStackInstances. Template parameter values can be
* overridden at the stack instance level during create or update stack set
* instance operations.
*
* Each stack set has one template and set of template parameters. When you
* update the template or template parameters, you update them for the entire
* set. Then all instance statuses are set to OUTDATED until the changes are
* deployed to that instance.
*
* Example:
*
*
* Pipeline pipeline;
* Artifact sourceOutput;
* pipeline.addStage(StageOptions.builder()
* .stageName("DeployStackSets")
* .actions(List.of(
* // First, update the StackSet itself with the newest template
* CloudFormationDeployStackSetAction.Builder.create()
* .actionName("UpdateStackSet")
* .runOrder(1)
* .stackSetName("MyStackSet")
* .template(StackSetTemplate.fromArtifactPath(sourceOutput.atPath("template.yaml")))
* // Change this to 'StackSetDeploymentModel.organizations()' if you want to deploy to OUs
* .deploymentModel(StackSetDeploymentModel.selfManaged())
* // This deploys to a set of accounts
* .stackInstances(StackInstances.inAccounts(List.of("111111111111"), List.of("us-east-1", "eu-west-1")))
* .build(),
* // Afterwards, update/create additional instances in other accounts
* CloudFormationDeployStackInstancesAction.Builder.create()
* .actionName("AddMoreInstances")
* .runOrder(2)
* .stackSetName("MyStackSet")
* .stackInstances(StackInstances.inAccounts(List.of("222222222222", "333333333333"), List.of("us-east-1", "eu-west-1")))
* .build()))
* .build());
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.74.0 (build 6d08790)", date = "2023-05-19T23:09:34.813Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.codepipeline.actions.$Module.class, fqn = "@aws-cdk/aws-codepipeline-actions.CloudFormationDeployStackInstancesAction")
public class CloudFormationDeployStackInstancesAction extends software.amazon.awscdk.services.codepipeline.actions.Action {
protected CloudFormationDeployStackInstancesAction(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected CloudFormationDeployStackInstancesAction(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
/**
* @param props This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public CloudFormationDeployStackInstancesAction(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.codepipeline.actions.CloudFormationDeployStackInstancesActionProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* This is a renamed version of the {@link IAction.bind} method.
*
* @param scope This parameter is required.
* @param _stage This parameter is required.
* @param options This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
protected @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.codepipeline.ActionConfig bound(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.Construct scope, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.codepipeline.IStage _stage, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.codepipeline.ActionBindOptions options) {
return software.amazon.jsii.Kernel.call(this, "bound", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.codepipeline.ActionConfig.class), new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(_stage, "_stage is required"), java.util.Objects.requireNonNull(options, "options is required") });
}
/**
* A fluent builder for {@link software.amazon.awscdk.services.codepipeline.actions.CloudFormationDeployStackInstancesAction}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static Builder create() {
return new Builder();
}
private final software.amazon.awscdk.services.codepipeline.actions.CloudFormationDeployStackInstancesActionProps.Builder props;
private Builder() {
this.props = new software.amazon.awscdk.services.codepipeline.actions.CloudFormationDeployStackInstancesActionProps.Builder();
}
/**
* The physical, human-readable name of the Action.
*
* Note that Action names must be unique within a single Stage.
*
* @return {@code this}
* @param actionName The physical, human-readable name of the Action. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder actionName(final java.lang.String actionName) {
this.props.actionName(actionName);
return this;
}
/**
* The runOrder property for this Action.
*
* RunOrder determines the relative order in which multiple Actions in the same Stage execute.
*
* Default: 1
*
* @return {@code this}
* @see https://docs.aws.amazon.com/codepipeline/latest/userguide/reference-pipeline-structure.html
* @param runOrder The runOrder property for this Action. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder runOrder(final java.lang.Number runOrder) {
this.props.runOrder(runOrder);
return this;
}
/**
* The name of the namespace to use for variables emitted by this action.
*
* Default: - a name will be generated, based on the stage and action names,
* if any of the action's variables were referenced - otherwise,
* no namespace will be set
*
* @return {@code this}
* @param variablesNamespace The name of the namespace to use for variables emitted by this action. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder variablesNamespace(final java.lang.String variablesNamespace) {
this.props.variablesNamespace(variablesNamespace);
return this;
}
/**
* The Role in which context's this Action will be executing in.
*
* The Pipeline's Role will assume this Role
* (the required permissions for that will be granted automatically)
* right before executing this Action.
* This Action will be passed into your {@link IAction.bind}
* method in the {@link ActionBindOptions.role} property.
*
* Default: a new Role will be generated
*
* @return {@code this}
* @param role The Role in which context's this Action will be executing in. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder role(final software.amazon.awscdk.services.iam.IRole role) {
this.props.role(role);
return this;
}
/**
* The percentage of accounts per Region for which this stack operation can fail before AWS CloudFormation stops the operation in that Region.
*
* If
* the operation is stopped in a Region, AWS CloudFormation doesn't attempt the operation in subsequent Regions. When calculating the number
* of accounts based on the specified percentage, AWS CloudFormation rounds down to the next whole number.
*
* Default: 0%
*
* @return {@code this}
* @param failureTolerancePercentage The percentage of accounts per Region for which this stack operation can fail before AWS CloudFormation stops the operation in that Region. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder failureTolerancePercentage(final java.lang.Number failureTolerancePercentage) {
this.props.failureTolerancePercentage(failureTolerancePercentage);
return this;
}
/**
* The maximum percentage of accounts in which to perform this operation at one time.
*
* When calculating the number of accounts based on the specified
* percentage, AWS CloudFormation rounds down to the next whole number. If rounding down would result in zero, AWS CloudFormation sets the number as
* one instead. Although you use this setting to specify the maximum, for large deployments the actual number of accounts acted upon concurrently
* may be lower due to service throttling.
*
* Default: 1%
*
* @return {@code this}
* @param maxAccountConcurrencyPercentage The maximum percentage of accounts in which to perform this operation at one time. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder maxAccountConcurrencyPercentage(final java.lang.Number maxAccountConcurrencyPercentage) {
this.props.maxAccountConcurrencyPercentage(maxAccountConcurrencyPercentage);
return this;
}
/**
* The AWS Region the StackSet is in.
*
* Note that a cross-region Pipeline requires replication buckets to function correctly.
* You can provide their names with the PipelineProps.crossRegionReplicationBuckets
property.
* If you don't, the CodePipeline Construct will create new Stacks in your CDK app containing those buckets,
* that you will need to cdk deploy
before deploying the main, Pipeline-containing Stack.
*
* Default: - same region as the Pipeline
*
* @return {@code this}
* @param stackSetRegion The AWS Region the StackSet is in. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder stackSetRegion(final java.lang.String stackSetRegion) {
this.props.stackSetRegion(stackSetRegion);
return this;
}
/**
* Specify where to create or update Stack Instances.
*
* You can specify either AWS Accounts Ids or AWS Organizations Organizational Units.
*
* @return {@code this}
* @param stackInstances Specify where to create or update Stack Instances. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder stackInstances(final software.amazon.awscdk.services.codepipeline.actions.StackInstances stackInstances) {
this.props.stackInstances(stackInstances);
return this;
}
/**
* The name of the StackSet we are adding instances to.
*
* @return {@code this}
* @param stackSetName The name of the StackSet we are adding instances to. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder stackSetName(final java.lang.String stackSetName) {
this.props.stackSetName(stackSetName);
return this;
}
/**
* Parameter values that only apply to the current Stack Instances.
*
* These parameters are shared between all instances added by this action.
*
* Default: - no parameters will be overridden
*
* @return {@code this}
* @param parameterOverrides Parameter values that only apply to the current Stack Instances. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder parameterOverrides(final software.amazon.awscdk.services.codepipeline.actions.StackSetParameters parameterOverrides) {
this.props.parameterOverrides(parameterOverrides);
return this;
}
/**
* @returns a newly built instance of {@link software.amazon.awscdk.services.codepipeline.actions.CloudFormationDeployStackInstancesAction}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public software.amazon.awscdk.services.codepipeline.actions.CloudFormationDeployStackInstancesAction build() {
return new software.amazon.awscdk.services.codepipeline.actions.CloudFormationDeployStackInstancesAction(
this.props.build()
);
}
}
}