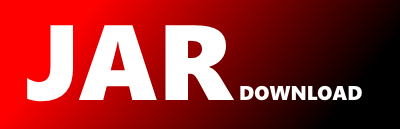
software.amazon.awscdk.services.codepipeline.actions.CloudFormationExecuteChangeSetAction Maven / Gradle / Ivy
Show all versions of codepipeline-actions Show documentation
package software.amazon.awscdk.services.codepipeline.actions;
/**
* CodePipeline action to execute a prepared change set.
*
* Example:
*
*
* // Source stage: read from repository
* Repository repo = Repository.Builder.create(stack, "TemplateRepo")
* .repositoryName("template-repo")
* .build();
* Artifact sourceOutput = new Artifact("SourceArtifact");
* CodeCommitSourceAction source = CodeCommitSourceAction.Builder.create()
* .actionName("Source")
* .repository(repo)
* .output(sourceOutput)
* .trigger(CodeCommitTrigger.POLL)
* .build();
* Map<String, Object> sourceStage = Map.of(
* "stageName", "Source",
* "actions", List.of(source));
* // Deployment stage: create and deploy changeset with manual approval
* String stackName = "OurStack";
* String changeSetName = "StagedChangeSet";
* Map<String, Object> prodStage = Map.of(
* "stageName", "Deploy",
* "actions", List.of(
* CloudFormationCreateReplaceChangeSetAction.Builder.create()
* .actionName("PrepareChanges")
* .stackName(stackName)
* .changeSetName(changeSetName)
* .adminPermissions(true)
* .templatePath(sourceOutput.atPath("template.yaml"))
* .runOrder(1)
* .build(),
* ManualApprovalAction.Builder.create()
* .actionName("ApproveChanges")
* .runOrder(2)
* .build(),
* CloudFormationExecuteChangeSetAction.Builder.create()
* .actionName("ExecuteChanges")
* .stackName(stackName)
* .changeSetName(changeSetName)
* .runOrder(3)
* .build()));
* Pipeline.Builder.create(stack, "Pipeline")
* .stages(List.of(sourceStage, prodStage))
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.74.0 (build 6d08790)", date = "2023-05-19T23:09:34.823Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.codepipeline.actions.$Module.class, fqn = "@aws-cdk/aws-codepipeline-actions.CloudFormationExecuteChangeSetAction")
public class CloudFormationExecuteChangeSetAction extends software.amazon.awscdk.services.codepipeline.actions.Action {
protected CloudFormationExecuteChangeSetAction(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected CloudFormationExecuteChangeSetAction(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
/**
* @param props This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public CloudFormationExecuteChangeSetAction(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.codepipeline.actions.CloudFormationExecuteChangeSetActionProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* This is a renamed version of the {@link IAction.bind} method.
*
* @param scope This parameter is required.
* @param stage This parameter is required.
* @param options This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
protected @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.codepipeline.ActionConfig bound(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.Construct scope, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.codepipeline.IStage stage, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.codepipeline.ActionBindOptions options) {
return software.amazon.jsii.Kernel.call(this, "bound", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.codepipeline.ActionConfig.class), new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(stage, "stage is required"), java.util.Objects.requireNonNull(options, "options is required") });
}
/**
* A fluent builder for {@link software.amazon.awscdk.services.codepipeline.actions.CloudFormationExecuteChangeSetAction}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static Builder create() {
return new Builder();
}
private final software.amazon.awscdk.services.codepipeline.actions.CloudFormationExecuteChangeSetActionProps.Builder props;
private Builder() {
this.props = new software.amazon.awscdk.services.codepipeline.actions.CloudFormationExecuteChangeSetActionProps.Builder();
}
/**
* The physical, human-readable name of the Action.
*
* Note that Action names must be unique within a single Stage.
*
* @return {@code this}
* @param actionName The physical, human-readable name of the Action. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder actionName(final java.lang.String actionName) {
this.props.actionName(actionName);
return this;
}
/**
* The runOrder property for this Action.
*
* RunOrder determines the relative order in which multiple Actions in the same Stage execute.
*
* Default: 1
*
* @return {@code this}
* @see https://docs.aws.amazon.com/codepipeline/latest/userguide/reference-pipeline-structure.html
* @param runOrder The runOrder property for this Action. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder runOrder(final java.lang.Number runOrder) {
this.props.runOrder(runOrder);
return this;
}
/**
* The name of the namespace to use for variables emitted by this action.
*
* Default: - a name will be generated, based on the stage and action names,
* if any of the action's variables were referenced - otherwise,
* no namespace will be set
*
* @return {@code this}
* @param variablesNamespace The name of the namespace to use for variables emitted by this action. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder variablesNamespace(final java.lang.String variablesNamespace) {
this.props.variablesNamespace(variablesNamespace);
return this;
}
/**
* The Role in which context's this Action will be executing in.
*
* The Pipeline's Role will assume this Role
* (the required permissions for that will be granted automatically)
* right before executing this Action.
* This Action will be passed into your {@link IAction.bind}
* method in the {@link ActionBindOptions.role} property.
*
* Default: a new Role will be generated
*
* @return {@code this}
* @param role The Role in which context's this Action will be executing in. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder role(final software.amazon.awscdk.services.iam.IRole role) {
this.props.role(role);
return this;
}
/**
* Name of the change set to execute.
*
* @return {@code this}
* @param changeSetName Name of the change set to execute. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder changeSetName(final java.lang.String changeSetName) {
this.props.changeSetName(changeSetName);
return this;
}
/**
* The name of the stack to apply this action to.
*
* @return {@code this}
* @param stackName The name of the stack to apply this action to. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder stackName(final java.lang.String stackName) {
this.props.stackName(stackName);
return this;
}
/**
* The AWS account this Action is supposed to operate in.
*
* Note: if you specify the role
property,
* this is ignored - the action will operate in the same region the passed role does.
*
* Default: - action resides in the same account as the pipeline
*
* @return {@code this}
* @param account The AWS account this Action is supposed to operate in. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder account(final java.lang.String account) {
this.props.account(account);
return this;
}
/**
* The name of the output artifact to generate.
*
* Only applied if outputFileName
is set as well.
*
* Default: Automatically generated artifact name.
*
* @return {@code this}
* @param output The name of the output artifact to generate. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder output(final software.amazon.awscdk.services.codepipeline.Artifact output) {
this.props.output(output);
return this;
}
/**
* A name for the filename in the output artifact to store the AWS CloudFormation call's result.
*
* The file will contain the result of the call to AWS CloudFormation (for example
* the call to UpdateStack or CreateChangeSet).
*
* AWS CodePipeline adds the file to the output artifact after performing
* the specified action.
*
* Default: No output artifact generated
*
* @return {@code this}
* @param outputFileName A name for the filename in the output artifact to store the AWS CloudFormation call's result. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder outputFileName(final java.lang.String outputFileName) {
this.props.outputFileName(outputFileName);
return this;
}
/**
* The AWS region the given Action resides in.
*
* Note that a cross-region Pipeline requires replication buckets to function correctly.
* You can provide their names with the {@link PipelineProps#crossRegionReplicationBuckets} property.
* If you don't, the CodePipeline Construct will create new Stacks in your CDK app containing those buckets,
* that you will need to cdk deploy
before deploying the main, Pipeline-containing Stack.
*
* Default: the Action resides in the same region as the Pipeline
*
* @return {@code this}
* @param region The AWS region the given Action resides in. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder region(final java.lang.String region) {
this.props.region(region);
return this;
}
/**
* @returns a newly built instance of {@link software.amazon.awscdk.services.codepipeline.actions.CloudFormationExecuteChangeSetAction}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public software.amazon.awscdk.services.codepipeline.actions.CloudFormationExecuteChangeSetAction build() {
return new software.amazon.awscdk.services.codepipeline.actions.CloudFormationExecuteChangeSetAction(
this.props.build()
);
}
}
}