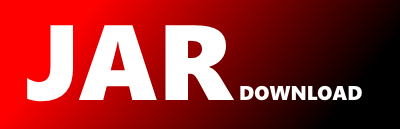
software.amazon.awscdk.services.codepipeline.actions.CloudFormationExecuteChangeSetActionProps Maven / Gradle / Ivy
Show all versions of codepipeline-actions Show documentation
package software.amazon.awscdk.services.codepipeline.actions;
/**
* Properties for the CloudFormationExecuteChangeSetAction.
*
* Example:
*
*
* // Source stage: read from repository
* Repository repo = Repository.Builder.create(stack, "TemplateRepo")
* .repositoryName("template-repo")
* .build();
* Artifact sourceOutput = new Artifact("SourceArtifact");
* CodeCommitSourceAction source = CodeCommitSourceAction.Builder.create()
* .actionName("Source")
* .repository(repo)
* .output(sourceOutput)
* .trigger(CodeCommitTrigger.POLL)
* .build();
* Map<String, Object> sourceStage = Map.of(
* "stageName", "Source",
* "actions", List.of(source));
* // Deployment stage: create and deploy changeset with manual approval
* String stackName = "OurStack";
* String changeSetName = "StagedChangeSet";
* Map<String, Object> prodStage = Map.of(
* "stageName", "Deploy",
* "actions", List.of(
* CloudFormationCreateReplaceChangeSetAction.Builder.create()
* .actionName("PrepareChanges")
* .stackName(stackName)
* .changeSetName(changeSetName)
* .adminPermissions(true)
* .templatePath(sourceOutput.atPath("template.yaml"))
* .runOrder(1)
* .build(),
* ManualApprovalAction.Builder.create()
* .actionName("ApproveChanges")
* .runOrder(2)
* .build(),
* CloudFormationExecuteChangeSetAction.Builder.create()
* .actionName("ExecuteChanges")
* .stackName(stackName)
* .changeSetName(changeSetName)
* .runOrder(3)
* .build()));
* Pipeline.Builder.create(stack, "Pipeline")
* .stages(List.of(sourceStage, prodStage))
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.74.0 (build 6d08790)", date = "2023-05-19T23:09:34.825Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.codepipeline.actions.$Module.class, fqn = "@aws-cdk/aws-codepipeline-actions.CloudFormationExecuteChangeSetActionProps")
@software.amazon.jsii.Jsii.Proxy(CloudFormationExecuteChangeSetActionProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface CloudFormationExecuteChangeSetActionProps extends software.amazon.jsii.JsiiSerializable, software.amazon.awscdk.services.codepipeline.CommonAwsActionProps {
/**
* Name of the change set to execute.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getChangeSetName();
/**
* The name of the stack to apply this action to.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getStackName();
/**
* The AWS account this Action is supposed to operate in.
*
* Note: if you specify the role
property,
* this is ignored - the action will operate in the same region the passed role does.
*
* Default: - action resides in the same account as the pipeline
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getAccount() {
return null;
}
/**
* The name of the output artifact to generate.
*
* Only applied if outputFileName
is set as well.
*
* Default: Automatically generated artifact name.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.codepipeline.Artifact getOutput() {
return null;
}
/**
* A name for the filename in the output artifact to store the AWS CloudFormation call's result.
*
* The file will contain the result of the call to AWS CloudFormation (for example
* the call to UpdateStack or CreateChangeSet).
*
* AWS CodePipeline adds the file to the output artifact after performing
* the specified action.
*
* Default: No output artifact generated
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getOutputFileName() {
return null;
}
/**
* The AWS region the given Action resides in.
*
* Note that a cross-region Pipeline requires replication buckets to function correctly.
* You can provide their names with the {@link PipelineProps#crossRegionReplicationBuckets} property.
* If you don't, the CodePipeline Construct will create new Stacks in your CDK app containing those buckets,
* that you will need to cdk deploy
before deploying the main, Pipeline-containing Stack.
*
* Default: the Action resides in the same region as the Pipeline
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getRegion() {
return null;
}
/**
* @return a {@link Builder} of {@link CloudFormationExecuteChangeSetActionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link CloudFormationExecuteChangeSetActionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String changeSetName;
java.lang.String stackName;
java.lang.String account;
software.amazon.awscdk.services.codepipeline.Artifact output;
java.lang.String outputFileName;
java.lang.String region;
software.amazon.awscdk.services.iam.IRole role;
java.lang.String actionName;
java.lang.Number runOrder;
java.lang.String variablesNamespace;
/**
* Sets the value of {@link CloudFormationExecuteChangeSetActionProps#getChangeSetName}
* @param changeSetName Name of the change set to execute. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder changeSetName(java.lang.String changeSetName) {
this.changeSetName = changeSetName;
return this;
}
/**
* Sets the value of {@link CloudFormationExecuteChangeSetActionProps#getStackName}
* @param stackName The name of the stack to apply this action to. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder stackName(java.lang.String stackName) {
this.stackName = stackName;
return this;
}
/**
* Sets the value of {@link CloudFormationExecuteChangeSetActionProps#getAccount}
* @param account The AWS account this Action is supposed to operate in.
* Note: if you specify the role
property,
* this is ignored - the action will operate in the same region the passed role does.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder account(java.lang.String account) {
this.account = account;
return this;
}
/**
* Sets the value of {@link CloudFormationExecuteChangeSetActionProps#getOutput}
* @param output The name of the output artifact to generate.
* Only applied if outputFileName
is set as well.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder output(software.amazon.awscdk.services.codepipeline.Artifact output) {
this.output = output;
return this;
}
/**
* Sets the value of {@link CloudFormationExecuteChangeSetActionProps#getOutputFileName}
* @param outputFileName A name for the filename in the output artifact to store the AWS CloudFormation call's result.
* The file will contain the result of the call to AWS CloudFormation (for example
* the call to UpdateStack or CreateChangeSet).
*
* AWS CodePipeline adds the file to the output artifact after performing
* the specified action.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder outputFileName(java.lang.String outputFileName) {
this.outputFileName = outputFileName;
return this;
}
/**
* Sets the value of {@link CloudFormationExecuteChangeSetActionProps#getRegion}
* @param region The AWS region the given Action resides in.
* Note that a cross-region Pipeline requires replication buckets to function correctly.
* You can provide their names with the {@link PipelineProps#crossRegionReplicationBuckets} property.
* If you don't, the CodePipeline Construct will create new Stacks in your CDK app containing those buckets,
* that you will need to cdk deploy
before deploying the main, Pipeline-containing Stack.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder region(java.lang.String region) {
this.region = region;
return this;
}
/**
* Sets the value of {@link CloudFormationExecuteChangeSetActionProps#getRole}
* @param role The Role in which context's this Action will be executing in.
* The Pipeline's Role will assume this Role
* (the required permissions for that will be granted automatically)
* right before executing this Action.
* This Action will be passed into your {@link IAction.bind}
* method in the {@link ActionBindOptions.role} property.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder role(software.amazon.awscdk.services.iam.IRole role) {
this.role = role;
return this;
}
/**
* Sets the value of {@link CloudFormationExecuteChangeSetActionProps#getActionName}
* @param actionName The physical, human-readable name of the Action. This parameter is required.
* Note that Action names must be unique within a single Stage.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder actionName(java.lang.String actionName) {
this.actionName = actionName;
return this;
}
/**
* Sets the value of {@link CloudFormationExecuteChangeSetActionProps#getRunOrder}
* @param runOrder The runOrder property for this Action.
* RunOrder determines the relative order in which multiple Actions in the same Stage execute.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder runOrder(java.lang.Number runOrder) {
this.runOrder = runOrder;
return this;
}
/**
* Sets the value of {@link CloudFormationExecuteChangeSetActionProps#getVariablesNamespace}
* @param variablesNamespace The name of the namespace to use for variables emitted by this action.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder variablesNamespace(java.lang.String variablesNamespace) {
this.variablesNamespace = variablesNamespace;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link CloudFormationExecuteChangeSetActionProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public CloudFormationExecuteChangeSetActionProps build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link CloudFormationExecuteChangeSetActionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements CloudFormationExecuteChangeSetActionProps {
private final java.lang.String changeSetName;
private final java.lang.String stackName;
private final java.lang.String account;
private final software.amazon.awscdk.services.codepipeline.Artifact output;
private final java.lang.String outputFileName;
private final java.lang.String region;
private final software.amazon.awscdk.services.iam.IRole role;
private final java.lang.String actionName;
private final java.lang.Number runOrder;
private final java.lang.String variablesNamespace;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.changeSetName = software.amazon.jsii.Kernel.get(this, "changeSetName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.stackName = software.amazon.jsii.Kernel.get(this, "stackName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.account = software.amazon.jsii.Kernel.get(this, "account", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.output = software.amazon.jsii.Kernel.get(this, "output", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.codepipeline.Artifact.class));
this.outputFileName = software.amazon.jsii.Kernel.get(this, "outputFileName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.region = software.amazon.jsii.Kernel.get(this, "region", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.role = software.amazon.jsii.Kernel.get(this, "role", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.iam.IRole.class));
this.actionName = software.amazon.jsii.Kernel.get(this, "actionName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.runOrder = software.amazon.jsii.Kernel.get(this, "runOrder", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.variablesNamespace = software.amazon.jsii.Kernel.get(this, "variablesNamespace", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.changeSetName = java.util.Objects.requireNonNull(builder.changeSetName, "changeSetName is required");
this.stackName = java.util.Objects.requireNonNull(builder.stackName, "stackName is required");
this.account = builder.account;
this.output = builder.output;
this.outputFileName = builder.outputFileName;
this.region = builder.region;
this.role = builder.role;
this.actionName = java.util.Objects.requireNonNull(builder.actionName, "actionName is required");
this.runOrder = builder.runOrder;
this.variablesNamespace = builder.variablesNamespace;
}
@Override
public final java.lang.String getChangeSetName() {
return this.changeSetName;
}
@Override
public final java.lang.String getStackName() {
return this.stackName;
}
@Override
public final java.lang.String getAccount() {
return this.account;
}
@Override
public final software.amazon.awscdk.services.codepipeline.Artifact getOutput() {
return this.output;
}
@Override
public final java.lang.String getOutputFileName() {
return this.outputFileName;
}
@Override
public final java.lang.String getRegion() {
return this.region;
}
@Override
public final software.amazon.awscdk.services.iam.IRole getRole() {
return this.role;
}
@Override
public final java.lang.String getActionName() {
return this.actionName;
}
@Override
public final java.lang.Number getRunOrder() {
return this.runOrder;
}
@Override
public final java.lang.String getVariablesNamespace() {
return this.variablesNamespace;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("changeSetName", om.valueToTree(this.getChangeSetName()));
data.set("stackName", om.valueToTree(this.getStackName()));
if (this.getAccount() != null) {
data.set("account", om.valueToTree(this.getAccount()));
}
if (this.getOutput() != null) {
data.set("output", om.valueToTree(this.getOutput()));
}
if (this.getOutputFileName() != null) {
data.set("outputFileName", om.valueToTree(this.getOutputFileName()));
}
if (this.getRegion() != null) {
data.set("region", om.valueToTree(this.getRegion()));
}
if (this.getRole() != null) {
data.set("role", om.valueToTree(this.getRole()));
}
data.set("actionName", om.valueToTree(this.getActionName()));
if (this.getRunOrder() != null) {
data.set("runOrder", om.valueToTree(this.getRunOrder()));
}
if (this.getVariablesNamespace() != null) {
data.set("variablesNamespace", om.valueToTree(this.getVariablesNamespace()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-codepipeline-actions.CloudFormationExecuteChangeSetActionProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
CloudFormationExecuteChangeSetActionProps.Jsii$Proxy that = (CloudFormationExecuteChangeSetActionProps.Jsii$Proxy) o;
if (!changeSetName.equals(that.changeSetName)) return false;
if (!stackName.equals(that.stackName)) return false;
if (this.account != null ? !this.account.equals(that.account) : that.account != null) return false;
if (this.output != null ? !this.output.equals(that.output) : that.output != null) return false;
if (this.outputFileName != null ? !this.outputFileName.equals(that.outputFileName) : that.outputFileName != null) return false;
if (this.region != null ? !this.region.equals(that.region) : that.region != null) return false;
if (this.role != null ? !this.role.equals(that.role) : that.role != null) return false;
if (!actionName.equals(that.actionName)) return false;
if (this.runOrder != null ? !this.runOrder.equals(that.runOrder) : that.runOrder != null) return false;
return this.variablesNamespace != null ? this.variablesNamespace.equals(that.variablesNamespace) : that.variablesNamespace == null;
}
@Override
public final int hashCode() {
int result = this.changeSetName.hashCode();
result = 31 * result + (this.stackName.hashCode());
result = 31 * result + (this.account != null ? this.account.hashCode() : 0);
result = 31 * result + (this.output != null ? this.output.hashCode() : 0);
result = 31 * result + (this.outputFileName != null ? this.outputFileName.hashCode() : 0);
result = 31 * result + (this.region != null ? this.region.hashCode() : 0);
result = 31 * result + (this.role != null ? this.role.hashCode() : 0);
result = 31 * result + (this.actionName.hashCode());
result = 31 * result + (this.runOrder != null ? this.runOrder.hashCode() : 0);
result = 31 * result + (this.variablesNamespace != null ? this.variablesNamespace.hashCode() : 0);
return result;
}
}
}