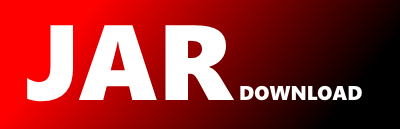
software.amazon.awscdk.services.codepipeline.actions.CodeBuildActionProps Maven / Gradle / Ivy
Show all versions of codepipeline-actions Show documentation
package software.amazon.awscdk.services.codepipeline.actions;
/**
* Construction properties of the {@link CodeBuildAction CodeBuild build CodePipeline action}.
*
* Example:
*
*
* // Create a Cloudfront Web Distribution
* import software.amazon.awscdk.services.cloudfront.*;
* Distribution distribution;
* // Create the build project that will invalidate the cache
* PipelineProject invalidateBuildProject = PipelineProject.Builder.create(this, "InvalidateProject")
* .buildSpec(BuildSpec.fromObject(Map.of(
* "version", "0.2",
* "phases", Map.of(
* "build", Map.of(
* "commands", List.of("aws cloudfront create-invalidation --distribution-id ${CLOUDFRONT_ID} --paths \"/*\""))))))
* .environmentVariables(Map.of(
* "CLOUDFRONT_ID", BuildEnvironmentVariable.builder().value(distribution.getDistributionId()).build()))
* .build();
* // Add Cloudfront invalidation permissions to the project
* String distributionArn = String.format("arn:aws:cloudfront::%s:distribution/%s", this.account, distribution.getDistributionId());
* invalidateBuildProject.addToRolePolicy(PolicyStatement.Builder.create()
* .resources(List.of(distributionArn))
* .actions(List.of("cloudfront:CreateInvalidation"))
* .build());
* // Create the pipeline (here only the S3 deploy and Invalidate cache build)
* Bucket deployBucket = new Bucket(this, "DeployBucket");
* Artifact deployInput = new Artifact();
* Pipeline.Builder.create(this, "Pipeline")
* .stages(List.of(StageProps.builder()
* .stageName("Deploy")
* .actions(List.of(
* S3DeployAction.Builder.create()
* .actionName("S3Deploy")
* .bucket(deployBucket)
* .input(deployInput)
* .runOrder(1)
* .build(),
* CodeBuildAction.Builder.create()
* .actionName("InvalidateCache")
* .project(invalidateBuildProject)
* .input(deployInput)
* .runOrder(2)
* .build()))
* .build()))
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.74.0 (build 6d08790)", date = "2023-05-19T23:09:34.845Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.codepipeline.actions.$Module.class, fqn = "@aws-cdk/aws-codepipeline-actions.CodeBuildActionProps")
@software.amazon.jsii.Jsii.Proxy(CodeBuildActionProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface CodeBuildActionProps extends software.amazon.jsii.JsiiSerializable, software.amazon.awscdk.services.codepipeline.CommonAwsActionProps {
/**
* The source to use as input for this action.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull software.amazon.awscdk.services.codepipeline.Artifact getInput();
/**
* The action's Project.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull software.amazon.awscdk.services.codebuild.IProject getProject();
/**
* Whether to check for the presence of any secrets in the environment variables of the default type, BuildEnvironmentVariableType.PLAINTEXT. Since using a secret for the value of that kind of variable would result in it being displayed in plain text in the AWS Console, the construct will throw an exception if it detects a secret was passed there. Pass this property as false if you want to skip this validation, and keep using a secret in a plain text environment variable.
*
* Default: true
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Boolean getCheckSecretsInPlainTextEnvVariables() {
return null;
}
/**
* Combine the build artifacts for a batch builds.
*
* Enabling this will combine the build artifacts into the same location for batch builds.
* If executeBatchBuild
is not set to true
, this property is ignored.
*
* Default: false
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Boolean getCombineBatchBuildArtifacts() {
return null;
}
/**
* The environment variables to pass to the CodeBuild project when this action executes.
*
* If a variable with the same name was set both on the project level, and here,
* this value will take precedence.
*
* Default: - No additional environment variables are specified.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.Map getEnvironmentVariables() {
return null;
}
/**
* Trigger a batch build.
*
* Enabling this will enable batch builds on the CodeBuild project.
*
* Default: false
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Boolean getExecuteBatchBuild() {
return null;
}
/**
* The list of additional input Artifacts for this action.
*
* The directories the additional inputs will be available at are available
* during the project's build in the CODEBUILD_SRC_DIR_ environment variables.
* The project's build always starts in the directory with the primary input artifact checked out,
* the one pointed to by the {@link input} property.
* For more information,
* see https://docs.aws.amazon.com/codebuild/latest/userguide/sample-multi-in-out.html .
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getExtraInputs() {
return null;
}
/**
* The list of output Artifacts for this action.
*
* Note: if you specify more than one output Artifact here,
* you cannot use the primary 'artifacts' section of the buildspec;
* you have to use the 'secondary-artifacts' section instead.
* See https://docs.aws.amazon.com/codebuild/latest/userguide/sample-multi-in-out.html
* for details.
*
* Default: the action will not have any outputs
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getOutputs() {
return null;
}
/**
* The type of the action that determines its CodePipeline Category - Build, or Test.
*
* Default: CodeBuildActionType.BUILD
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.codepipeline.actions.CodeBuildActionType getType() {
return null;
}
/**
* @return a {@link Builder} of {@link CodeBuildActionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link CodeBuildActionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
software.amazon.awscdk.services.codepipeline.Artifact input;
software.amazon.awscdk.services.codebuild.IProject project;
java.lang.Boolean checkSecretsInPlainTextEnvVariables;
java.lang.Boolean combineBatchBuildArtifacts;
java.util.Map environmentVariables;
java.lang.Boolean executeBatchBuild;
java.util.List extraInputs;
java.util.List outputs;
software.amazon.awscdk.services.codepipeline.actions.CodeBuildActionType type;
software.amazon.awscdk.services.iam.IRole role;
java.lang.String actionName;
java.lang.Number runOrder;
java.lang.String variablesNamespace;
/**
* Sets the value of {@link CodeBuildActionProps#getInput}
* @param input The source to use as input for this action. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder input(software.amazon.awscdk.services.codepipeline.Artifact input) {
this.input = input;
return this;
}
/**
* Sets the value of {@link CodeBuildActionProps#getProject}
* @param project The action's Project. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder project(software.amazon.awscdk.services.codebuild.IProject project) {
this.project = project;
return this;
}
/**
* Sets the value of {@link CodeBuildActionProps#getCheckSecretsInPlainTextEnvVariables}
* @param checkSecretsInPlainTextEnvVariables Whether to check for the presence of any secrets in the environment variables of the default type, BuildEnvironmentVariableType.PLAINTEXT. Since using a secret for the value of that kind of variable would result in it being displayed in plain text in the AWS Console, the construct will throw an exception if it detects a secret was passed there. Pass this property as false if you want to skip this validation, and keep using a secret in a plain text environment variable.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder checkSecretsInPlainTextEnvVariables(java.lang.Boolean checkSecretsInPlainTextEnvVariables) {
this.checkSecretsInPlainTextEnvVariables = checkSecretsInPlainTextEnvVariables;
return this;
}
/**
* Sets the value of {@link CodeBuildActionProps#getCombineBatchBuildArtifacts}
* @param combineBatchBuildArtifacts Combine the build artifacts for a batch builds.
* Enabling this will combine the build artifacts into the same location for batch builds.
* If executeBatchBuild
is not set to true
, this property is ignored.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder combineBatchBuildArtifacts(java.lang.Boolean combineBatchBuildArtifacts) {
this.combineBatchBuildArtifacts = combineBatchBuildArtifacts;
return this;
}
/**
* Sets the value of {@link CodeBuildActionProps#getEnvironmentVariables}
* @param environmentVariables The environment variables to pass to the CodeBuild project when this action executes.
* If a variable with the same name was set both on the project level, and here,
* this value will take precedence.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@SuppressWarnings("unchecked")
public Builder environmentVariables(java.util.Map environmentVariables) {
this.environmentVariables = (java.util.Map)environmentVariables;
return this;
}
/**
* Sets the value of {@link CodeBuildActionProps#getExecuteBatchBuild}
* @param executeBatchBuild Trigger a batch build.
* Enabling this will enable batch builds on the CodeBuild project.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder executeBatchBuild(java.lang.Boolean executeBatchBuild) {
this.executeBatchBuild = executeBatchBuild;
return this;
}
/**
* Sets the value of {@link CodeBuildActionProps#getExtraInputs}
* @param extraInputs The list of additional input Artifacts for this action.
* The directories the additional inputs will be available at are available
* during the project's build in the CODEBUILD_SRC_DIR_ environment variables.
* The project's build always starts in the directory with the primary input artifact checked out,
* the one pointed to by the {@link input} property.
* For more information,
* see https://docs.aws.amazon.com/codebuild/latest/userguide/sample-multi-in-out.html .
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@SuppressWarnings("unchecked")
public Builder extraInputs(java.util.List extends software.amazon.awscdk.services.codepipeline.Artifact> extraInputs) {
this.extraInputs = (java.util.List)extraInputs;
return this;
}
/**
* Sets the value of {@link CodeBuildActionProps#getOutputs}
* @param outputs The list of output Artifacts for this action.
* Note: if you specify more than one output Artifact here,
* you cannot use the primary 'artifacts' section of the buildspec;
* you have to use the 'secondary-artifacts' section instead.
* See https://docs.aws.amazon.com/codebuild/latest/userguide/sample-multi-in-out.html
* for details.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@SuppressWarnings("unchecked")
public Builder outputs(java.util.List extends software.amazon.awscdk.services.codepipeline.Artifact> outputs) {
this.outputs = (java.util.List)outputs;
return this;
}
/**
* Sets the value of {@link CodeBuildActionProps#getType}
* @param type The type of the action that determines its CodePipeline Category - Build, or Test.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder type(software.amazon.awscdk.services.codepipeline.actions.CodeBuildActionType type) {
this.type = type;
return this;
}
/**
* Sets the value of {@link CodeBuildActionProps#getRole}
* @param role The Role in which context's this Action will be executing in.
* The Pipeline's Role will assume this Role
* (the required permissions for that will be granted automatically)
* right before executing this Action.
* This Action will be passed into your {@link IAction.bind}
* method in the {@link ActionBindOptions.role} property.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder role(software.amazon.awscdk.services.iam.IRole role) {
this.role = role;
return this;
}
/**
* Sets the value of {@link CodeBuildActionProps#getActionName}
* @param actionName The physical, human-readable name of the Action. This parameter is required.
* Note that Action names must be unique within a single Stage.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder actionName(java.lang.String actionName) {
this.actionName = actionName;
return this;
}
/**
* Sets the value of {@link CodeBuildActionProps#getRunOrder}
* @param runOrder The runOrder property for this Action.
* RunOrder determines the relative order in which multiple Actions in the same Stage execute.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder runOrder(java.lang.Number runOrder) {
this.runOrder = runOrder;
return this;
}
/**
* Sets the value of {@link CodeBuildActionProps#getVariablesNamespace}
* @param variablesNamespace The name of the namespace to use for variables emitted by this action.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder variablesNamespace(java.lang.String variablesNamespace) {
this.variablesNamespace = variablesNamespace;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link CodeBuildActionProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public CodeBuildActionProps build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link CodeBuildActionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements CodeBuildActionProps {
private final software.amazon.awscdk.services.codepipeline.Artifact input;
private final software.amazon.awscdk.services.codebuild.IProject project;
private final java.lang.Boolean checkSecretsInPlainTextEnvVariables;
private final java.lang.Boolean combineBatchBuildArtifacts;
private final java.util.Map environmentVariables;
private final java.lang.Boolean executeBatchBuild;
private final java.util.List extraInputs;
private final java.util.List outputs;
private final software.amazon.awscdk.services.codepipeline.actions.CodeBuildActionType type;
private final software.amazon.awscdk.services.iam.IRole role;
private final java.lang.String actionName;
private final java.lang.Number runOrder;
private final java.lang.String variablesNamespace;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.input = software.amazon.jsii.Kernel.get(this, "input", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.codepipeline.Artifact.class));
this.project = software.amazon.jsii.Kernel.get(this, "project", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.codebuild.IProject.class));
this.checkSecretsInPlainTextEnvVariables = software.amazon.jsii.Kernel.get(this, "checkSecretsInPlainTextEnvVariables", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.combineBatchBuildArtifacts = software.amazon.jsii.Kernel.get(this, "combineBatchBuildArtifacts", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.environmentVariables = software.amazon.jsii.Kernel.get(this, "environmentVariables", software.amazon.jsii.NativeType.mapOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.codebuild.BuildEnvironmentVariable.class)));
this.executeBatchBuild = software.amazon.jsii.Kernel.get(this, "executeBatchBuild", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.extraInputs = software.amazon.jsii.Kernel.get(this, "extraInputs", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.codepipeline.Artifact.class)));
this.outputs = software.amazon.jsii.Kernel.get(this, "outputs", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.codepipeline.Artifact.class)));
this.type = software.amazon.jsii.Kernel.get(this, "type", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.codepipeline.actions.CodeBuildActionType.class));
this.role = software.amazon.jsii.Kernel.get(this, "role", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.iam.IRole.class));
this.actionName = software.amazon.jsii.Kernel.get(this, "actionName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.runOrder = software.amazon.jsii.Kernel.get(this, "runOrder", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.variablesNamespace = software.amazon.jsii.Kernel.get(this, "variablesNamespace", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
@SuppressWarnings("unchecked")
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.input = java.util.Objects.requireNonNull(builder.input, "input is required");
this.project = java.util.Objects.requireNonNull(builder.project, "project is required");
this.checkSecretsInPlainTextEnvVariables = builder.checkSecretsInPlainTextEnvVariables;
this.combineBatchBuildArtifacts = builder.combineBatchBuildArtifacts;
this.environmentVariables = (java.util.Map)builder.environmentVariables;
this.executeBatchBuild = builder.executeBatchBuild;
this.extraInputs = (java.util.List)builder.extraInputs;
this.outputs = (java.util.List)builder.outputs;
this.type = builder.type;
this.role = builder.role;
this.actionName = java.util.Objects.requireNonNull(builder.actionName, "actionName is required");
this.runOrder = builder.runOrder;
this.variablesNamespace = builder.variablesNamespace;
}
@Override
public final software.amazon.awscdk.services.codepipeline.Artifact getInput() {
return this.input;
}
@Override
public final software.amazon.awscdk.services.codebuild.IProject getProject() {
return this.project;
}
@Override
public final java.lang.Boolean getCheckSecretsInPlainTextEnvVariables() {
return this.checkSecretsInPlainTextEnvVariables;
}
@Override
public final java.lang.Boolean getCombineBatchBuildArtifacts() {
return this.combineBatchBuildArtifacts;
}
@Override
public final java.util.Map getEnvironmentVariables() {
return this.environmentVariables;
}
@Override
public final java.lang.Boolean getExecuteBatchBuild() {
return this.executeBatchBuild;
}
@Override
public final java.util.List getExtraInputs() {
return this.extraInputs;
}
@Override
public final java.util.List getOutputs() {
return this.outputs;
}
@Override
public final software.amazon.awscdk.services.codepipeline.actions.CodeBuildActionType getType() {
return this.type;
}
@Override
public final software.amazon.awscdk.services.iam.IRole getRole() {
return this.role;
}
@Override
public final java.lang.String getActionName() {
return this.actionName;
}
@Override
public final java.lang.Number getRunOrder() {
return this.runOrder;
}
@Override
public final java.lang.String getVariablesNamespace() {
return this.variablesNamespace;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("input", om.valueToTree(this.getInput()));
data.set("project", om.valueToTree(this.getProject()));
if (this.getCheckSecretsInPlainTextEnvVariables() != null) {
data.set("checkSecretsInPlainTextEnvVariables", om.valueToTree(this.getCheckSecretsInPlainTextEnvVariables()));
}
if (this.getCombineBatchBuildArtifacts() != null) {
data.set("combineBatchBuildArtifacts", om.valueToTree(this.getCombineBatchBuildArtifacts()));
}
if (this.getEnvironmentVariables() != null) {
data.set("environmentVariables", om.valueToTree(this.getEnvironmentVariables()));
}
if (this.getExecuteBatchBuild() != null) {
data.set("executeBatchBuild", om.valueToTree(this.getExecuteBatchBuild()));
}
if (this.getExtraInputs() != null) {
data.set("extraInputs", om.valueToTree(this.getExtraInputs()));
}
if (this.getOutputs() != null) {
data.set("outputs", om.valueToTree(this.getOutputs()));
}
if (this.getType() != null) {
data.set("type", om.valueToTree(this.getType()));
}
if (this.getRole() != null) {
data.set("role", om.valueToTree(this.getRole()));
}
data.set("actionName", om.valueToTree(this.getActionName()));
if (this.getRunOrder() != null) {
data.set("runOrder", om.valueToTree(this.getRunOrder()));
}
if (this.getVariablesNamespace() != null) {
data.set("variablesNamespace", om.valueToTree(this.getVariablesNamespace()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-codepipeline-actions.CodeBuildActionProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
CodeBuildActionProps.Jsii$Proxy that = (CodeBuildActionProps.Jsii$Proxy) o;
if (!input.equals(that.input)) return false;
if (!project.equals(that.project)) return false;
if (this.checkSecretsInPlainTextEnvVariables != null ? !this.checkSecretsInPlainTextEnvVariables.equals(that.checkSecretsInPlainTextEnvVariables) : that.checkSecretsInPlainTextEnvVariables != null) return false;
if (this.combineBatchBuildArtifacts != null ? !this.combineBatchBuildArtifacts.equals(that.combineBatchBuildArtifacts) : that.combineBatchBuildArtifacts != null) return false;
if (this.environmentVariables != null ? !this.environmentVariables.equals(that.environmentVariables) : that.environmentVariables != null) return false;
if (this.executeBatchBuild != null ? !this.executeBatchBuild.equals(that.executeBatchBuild) : that.executeBatchBuild != null) return false;
if (this.extraInputs != null ? !this.extraInputs.equals(that.extraInputs) : that.extraInputs != null) return false;
if (this.outputs != null ? !this.outputs.equals(that.outputs) : that.outputs != null) return false;
if (this.type != null ? !this.type.equals(that.type) : that.type != null) return false;
if (this.role != null ? !this.role.equals(that.role) : that.role != null) return false;
if (!actionName.equals(that.actionName)) return false;
if (this.runOrder != null ? !this.runOrder.equals(that.runOrder) : that.runOrder != null) return false;
return this.variablesNamespace != null ? this.variablesNamespace.equals(that.variablesNamespace) : that.variablesNamespace == null;
}
@Override
public final int hashCode() {
int result = this.input.hashCode();
result = 31 * result + (this.project.hashCode());
result = 31 * result + (this.checkSecretsInPlainTextEnvVariables != null ? this.checkSecretsInPlainTextEnvVariables.hashCode() : 0);
result = 31 * result + (this.combineBatchBuildArtifacts != null ? this.combineBatchBuildArtifacts.hashCode() : 0);
result = 31 * result + (this.environmentVariables != null ? this.environmentVariables.hashCode() : 0);
result = 31 * result + (this.executeBatchBuild != null ? this.executeBatchBuild.hashCode() : 0);
result = 31 * result + (this.extraInputs != null ? this.extraInputs.hashCode() : 0);
result = 31 * result + (this.outputs != null ? this.outputs.hashCode() : 0);
result = 31 * result + (this.type != null ? this.type.hashCode() : 0);
result = 31 * result + (this.role != null ? this.role.hashCode() : 0);
result = 31 * result + (this.actionName.hashCode());
result = 31 * result + (this.runOrder != null ? this.runOrder.hashCode() : 0);
result = 31 * result + (this.variablesNamespace != null ? this.variablesNamespace.hashCode() : 0);
return result;
}
}
}