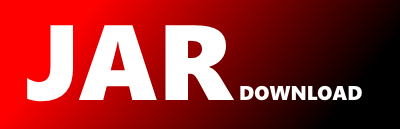
software.amazon.awscdk.services.codepipeline.actions.CodeCommitSourceVariables Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of codepipeline-actions Show documentation
Show all versions of codepipeline-actions Show documentation
Concrete Actions for AWS Code Pipeline
package software.amazon.awscdk.services.codepipeline.actions;
/**
* The CodePipeline variables emitted by the CodeCommit source Action.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.codepipeline.actions.*;
* CodeCommitSourceVariables codeCommitSourceVariables = CodeCommitSourceVariables.builder()
* .authorDate("authorDate")
* .branchName("branchName")
* .commitId("commitId")
* .commitMessage("commitMessage")
* .committerDate("committerDate")
* .repositoryName("repositoryName")
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.74.0 (build 6d08790)", date = "2023-05-19T23:09:34.852Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.codepipeline.actions.$Module.class, fqn = "@aws-cdk/aws-codepipeline-actions.CodeCommitSourceVariables")
@software.amazon.jsii.Jsii.Proxy(CodeCommitSourceVariables.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface CodeCommitSourceVariables extends software.amazon.jsii.JsiiSerializable {
/**
* The date the currently last commit on the tracked branch was authored, in ISO-8601 format.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getAuthorDate();
/**
* The name of the branch this action tracks.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getBranchName();
/**
* The SHA1 hash of the currently last commit on the tracked branch.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getCommitId();
/**
* The message of the currently last commit on the tracked branch.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getCommitMessage();
/**
* The date the currently last commit on the tracked branch was committed, in ISO-8601 format.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getCommitterDate();
/**
* The name of the repository this action points to.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getRepositoryName();
/**
* @return a {@link Builder} of {@link CodeCommitSourceVariables}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link CodeCommitSourceVariables}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String authorDate;
java.lang.String branchName;
java.lang.String commitId;
java.lang.String commitMessage;
java.lang.String committerDate;
java.lang.String repositoryName;
/**
* Sets the value of {@link CodeCommitSourceVariables#getAuthorDate}
* @param authorDate The date the currently last commit on the tracked branch was authored, in ISO-8601 format. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder authorDate(java.lang.String authorDate) {
this.authorDate = authorDate;
return this;
}
/**
* Sets the value of {@link CodeCommitSourceVariables#getBranchName}
* @param branchName The name of the branch this action tracks. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder branchName(java.lang.String branchName) {
this.branchName = branchName;
return this;
}
/**
* Sets the value of {@link CodeCommitSourceVariables#getCommitId}
* @param commitId The SHA1 hash of the currently last commit on the tracked branch. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder commitId(java.lang.String commitId) {
this.commitId = commitId;
return this;
}
/**
* Sets the value of {@link CodeCommitSourceVariables#getCommitMessage}
* @param commitMessage The message of the currently last commit on the tracked branch. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder commitMessage(java.lang.String commitMessage) {
this.commitMessage = commitMessage;
return this;
}
/**
* Sets the value of {@link CodeCommitSourceVariables#getCommitterDate}
* @param committerDate The date the currently last commit on the tracked branch was committed, in ISO-8601 format. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder committerDate(java.lang.String committerDate) {
this.committerDate = committerDate;
return this;
}
/**
* Sets the value of {@link CodeCommitSourceVariables#getRepositoryName}
* @param repositoryName The name of the repository this action points to. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder repositoryName(java.lang.String repositoryName) {
this.repositoryName = repositoryName;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link CodeCommitSourceVariables}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public CodeCommitSourceVariables build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link CodeCommitSourceVariables}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements CodeCommitSourceVariables {
private final java.lang.String authorDate;
private final java.lang.String branchName;
private final java.lang.String commitId;
private final java.lang.String commitMessage;
private final java.lang.String committerDate;
private final java.lang.String repositoryName;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.authorDate = software.amazon.jsii.Kernel.get(this, "authorDate", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.branchName = software.amazon.jsii.Kernel.get(this, "branchName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.commitId = software.amazon.jsii.Kernel.get(this, "commitId", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.commitMessage = software.amazon.jsii.Kernel.get(this, "commitMessage", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.committerDate = software.amazon.jsii.Kernel.get(this, "committerDate", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.repositoryName = software.amazon.jsii.Kernel.get(this, "repositoryName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.authorDate = java.util.Objects.requireNonNull(builder.authorDate, "authorDate is required");
this.branchName = java.util.Objects.requireNonNull(builder.branchName, "branchName is required");
this.commitId = java.util.Objects.requireNonNull(builder.commitId, "commitId is required");
this.commitMessage = java.util.Objects.requireNonNull(builder.commitMessage, "commitMessage is required");
this.committerDate = java.util.Objects.requireNonNull(builder.committerDate, "committerDate is required");
this.repositoryName = java.util.Objects.requireNonNull(builder.repositoryName, "repositoryName is required");
}
@Override
public final java.lang.String getAuthorDate() {
return this.authorDate;
}
@Override
public final java.lang.String getBranchName() {
return this.branchName;
}
@Override
public final java.lang.String getCommitId() {
return this.commitId;
}
@Override
public final java.lang.String getCommitMessage() {
return this.commitMessage;
}
@Override
public final java.lang.String getCommitterDate() {
return this.committerDate;
}
@Override
public final java.lang.String getRepositoryName() {
return this.repositoryName;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("authorDate", om.valueToTree(this.getAuthorDate()));
data.set("branchName", om.valueToTree(this.getBranchName()));
data.set("commitId", om.valueToTree(this.getCommitId()));
data.set("commitMessage", om.valueToTree(this.getCommitMessage()));
data.set("committerDate", om.valueToTree(this.getCommitterDate()));
data.set("repositoryName", om.valueToTree(this.getRepositoryName()));
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-codepipeline-actions.CodeCommitSourceVariables"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
CodeCommitSourceVariables.Jsii$Proxy that = (CodeCommitSourceVariables.Jsii$Proxy) o;
if (!authorDate.equals(that.authorDate)) return false;
if (!branchName.equals(that.branchName)) return false;
if (!commitId.equals(that.commitId)) return false;
if (!commitMessage.equals(that.commitMessage)) return false;
if (!committerDate.equals(that.committerDate)) return false;
return this.repositoryName.equals(that.repositoryName);
}
@Override
public final int hashCode() {
int result = this.authorDate.hashCode();
result = 31 * result + (this.branchName.hashCode());
result = 31 * result + (this.commitId.hashCode());
result = 31 * result + (this.commitMessage.hashCode());
result = 31 * result + (this.committerDate.hashCode());
result = 31 * result + (this.repositoryName.hashCode());
return result;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy