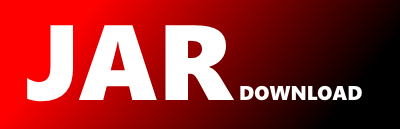
software.amazon.awscdk.services.codepipeline.actions.CodeDeployEcsDeployActionProps Maven / Gradle / Ivy
Show all versions of codepipeline-actions Show documentation
package software.amazon.awscdk.services.codepipeline.actions;
/**
* Construction properties of the {@link CodeDeployEcsDeployAction CodeDeploy ECS deploy CodePipeline Action}.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.codedeploy.*;
* import software.amazon.awscdk.services.codepipeline.*;
* import software.amazon.awscdk.services.codepipeline.actions.*;
* import software.amazon.awscdk.services.iam.*;
* Artifact artifact;
* ArtifactPath artifactPath;
* IEcsDeploymentGroup ecsDeploymentGroup;
* Role role;
* CodeDeployEcsDeployActionProps codeDeployEcsDeployActionProps = CodeDeployEcsDeployActionProps.builder()
* .actionName("actionName")
* .deploymentGroup(ecsDeploymentGroup)
* // the properties below are optional
* .appSpecTemplateFile(artifactPath)
* .appSpecTemplateInput(artifact)
* .containerImageInputs(List.of(CodeDeployEcsContainerImageInput.builder()
* .input(artifact)
* // the properties below are optional
* .taskDefinitionPlaceholder("taskDefinitionPlaceholder")
* .build()))
* .role(role)
* .runOrder(123)
* .taskDefinitionTemplateFile(artifactPath)
* .taskDefinitionTemplateInput(artifact)
* .variablesNamespace("variablesNamespace")
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.74.0 (build 6d08790)", date = "2023-05-19T23:09:34.860Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.codepipeline.actions.$Module.class, fqn = "@aws-cdk/aws-codepipeline-actions.CodeDeployEcsDeployActionProps")
@software.amazon.jsii.Jsii.Proxy(CodeDeployEcsDeployActionProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface CodeDeployEcsDeployActionProps extends software.amazon.jsii.JsiiSerializable, software.amazon.awscdk.services.codepipeline.CommonAwsActionProps {
/**
* The CodeDeploy ECS Deployment Group to deploy to.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull software.amazon.awscdk.services.codedeploy.IEcsDeploymentGroup getDeploymentGroup();
/**
* The name of the CodeDeploy AppSpec file.
*
* During deployment, a new task definition will be registered
* with ECS, and the new task definition ID will be inserted into
* the CodeDeploy AppSpec file. The AppSpec file contents will be
* provided to CodeDeploy for the deployment.
*
* Use this property if you want to use a different name for this file than the default 'appspec.yaml'.
* If you use this property, you don't need to specify the appSpecTemplateInput
property.
*
* Default: - one of this property, or `appSpecTemplateInput`, is required
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.codepipeline.ArtifactPath getAppSpecTemplateFile() {
return null;
}
/**
* The artifact containing the CodeDeploy AppSpec file.
*
* During deployment, a new task definition will be registered
* with ECS, and the new task definition ID will be inserted into
* the CodeDeploy AppSpec file. The AppSpec file contents will be
* provided to CodeDeploy for the deployment.
*
* If you use this property, it's assumed the file is called 'appspec.yaml'.
* If your AppSpec file uses a different filename, leave this property empty,
* and use the appSpecTemplateFile
property instead.
*
* Default: - one of this property, or `appSpecTemplateFile`, is required
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.codepipeline.Artifact getAppSpecTemplateInput() {
return null;
}
/**
* Configuration for dynamically updated images in the task definition.
*
* Provide pairs of an image details input artifact and a placeholder string
* that will be used to dynamically update the ECS task definition template
* file prior to deployment. A maximum of 4 images can be given.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getContainerImageInputs() {
return null;
}
/**
* The name of the ECS task definition template file.
*
* During deployment, the task definition template file contents
* will be registered with ECS.
*
* Use this property if you want to use a different name for this file than the default 'taskdef.json'.
* If you use this property, you don't need to specify the taskDefinitionTemplateInput
property.
*
* Default: - one of this property, or `taskDefinitionTemplateInput`, is required
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.codepipeline.ArtifactPath getTaskDefinitionTemplateFile() {
return null;
}
/**
* The artifact containing the ECS task definition template file.
*
* During deployment, the task definition template file contents
* will be registered with ECS.
*
* If you use this property, it's assumed the file is called 'taskdef.json'.
* If your task definition template uses a different filename, leave this property empty,
* and use the taskDefinitionTemplateFile
property instead.
*
* Default: - one of this property, or `taskDefinitionTemplateFile`, is required
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.codepipeline.Artifact getTaskDefinitionTemplateInput() {
return null;
}
/**
* @return a {@link Builder} of {@link CodeDeployEcsDeployActionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link CodeDeployEcsDeployActionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
software.amazon.awscdk.services.codedeploy.IEcsDeploymentGroup deploymentGroup;
software.amazon.awscdk.services.codepipeline.ArtifactPath appSpecTemplateFile;
software.amazon.awscdk.services.codepipeline.Artifact appSpecTemplateInput;
java.util.List containerImageInputs;
software.amazon.awscdk.services.codepipeline.ArtifactPath taskDefinitionTemplateFile;
software.amazon.awscdk.services.codepipeline.Artifact taskDefinitionTemplateInput;
software.amazon.awscdk.services.iam.IRole role;
java.lang.String actionName;
java.lang.Number runOrder;
java.lang.String variablesNamespace;
/**
* Sets the value of {@link CodeDeployEcsDeployActionProps#getDeploymentGroup}
* @param deploymentGroup The CodeDeploy ECS Deployment Group to deploy to. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder deploymentGroup(software.amazon.awscdk.services.codedeploy.IEcsDeploymentGroup deploymentGroup) {
this.deploymentGroup = deploymentGroup;
return this;
}
/**
* Sets the value of {@link CodeDeployEcsDeployActionProps#getAppSpecTemplateFile}
* @param appSpecTemplateFile The name of the CodeDeploy AppSpec file.
* During deployment, a new task definition will be registered
* with ECS, and the new task definition ID will be inserted into
* the CodeDeploy AppSpec file. The AppSpec file contents will be
* provided to CodeDeploy for the deployment.
*
* Use this property if you want to use a different name for this file than the default 'appspec.yaml'.
* If you use this property, you don't need to specify the appSpecTemplateInput
property.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder appSpecTemplateFile(software.amazon.awscdk.services.codepipeline.ArtifactPath appSpecTemplateFile) {
this.appSpecTemplateFile = appSpecTemplateFile;
return this;
}
/**
* Sets the value of {@link CodeDeployEcsDeployActionProps#getAppSpecTemplateInput}
* @param appSpecTemplateInput The artifact containing the CodeDeploy AppSpec file.
* During deployment, a new task definition will be registered
* with ECS, and the new task definition ID will be inserted into
* the CodeDeploy AppSpec file. The AppSpec file contents will be
* provided to CodeDeploy for the deployment.
*
* If you use this property, it's assumed the file is called 'appspec.yaml'.
* If your AppSpec file uses a different filename, leave this property empty,
* and use the appSpecTemplateFile
property instead.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder appSpecTemplateInput(software.amazon.awscdk.services.codepipeline.Artifact appSpecTemplateInput) {
this.appSpecTemplateInput = appSpecTemplateInput;
return this;
}
/**
* Sets the value of {@link CodeDeployEcsDeployActionProps#getContainerImageInputs}
* @param containerImageInputs Configuration for dynamically updated images in the task definition.
* Provide pairs of an image details input artifact and a placeholder string
* that will be used to dynamically update the ECS task definition template
* file prior to deployment. A maximum of 4 images can be given.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@SuppressWarnings("unchecked")
public Builder containerImageInputs(java.util.List extends software.amazon.awscdk.services.codepipeline.actions.CodeDeployEcsContainerImageInput> containerImageInputs) {
this.containerImageInputs = (java.util.List)containerImageInputs;
return this;
}
/**
* Sets the value of {@link CodeDeployEcsDeployActionProps#getTaskDefinitionTemplateFile}
* @param taskDefinitionTemplateFile The name of the ECS task definition template file.
* During deployment, the task definition template file contents
* will be registered with ECS.
*
* Use this property if you want to use a different name for this file than the default 'taskdef.json'.
* If you use this property, you don't need to specify the taskDefinitionTemplateInput
property.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder taskDefinitionTemplateFile(software.amazon.awscdk.services.codepipeline.ArtifactPath taskDefinitionTemplateFile) {
this.taskDefinitionTemplateFile = taskDefinitionTemplateFile;
return this;
}
/**
* Sets the value of {@link CodeDeployEcsDeployActionProps#getTaskDefinitionTemplateInput}
* @param taskDefinitionTemplateInput The artifact containing the ECS task definition template file.
* During deployment, the task definition template file contents
* will be registered with ECS.
*
* If you use this property, it's assumed the file is called 'taskdef.json'.
* If your task definition template uses a different filename, leave this property empty,
* and use the taskDefinitionTemplateFile
property instead.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder taskDefinitionTemplateInput(software.amazon.awscdk.services.codepipeline.Artifact taskDefinitionTemplateInput) {
this.taskDefinitionTemplateInput = taskDefinitionTemplateInput;
return this;
}
/**
* Sets the value of {@link CodeDeployEcsDeployActionProps#getRole}
* @param role The Role in which context's this Action will be executing in.
* The Pipeline's Role will assume this Role
* (the required permissions for that will be granted automatically)
* right before executing this Action.
* This Action will be passed into your {@link IAction.bind}
* method in the {@link ActionBindOptions.role} property.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder role(software.amazon.awscdk.services.iam.IRole role) {
this.role = role;
return this;
}
/**
* Sets the value of {@link CodeDeployEcsDeployActionProps#getActionName}
* @param actionName The physical, human-readable name of the Action. This parameter is required.
* Note that Action names must be unique within a single Stage.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder actionName(java.lang.String actionName) {
this.actionName = actionName;
return this;
}
/**
* Sets the value of {@link CodeDeployEcsDeployActionProps#getRunOrder}
* @param runOrder The runOrder property for this Action.
* RunOrder determines the relative order in which multiple Actions in the same Stage execute.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder runOrder(java.lang.Number runOrder) {
this.runOrder = runOrder;
return this;
}
/**
* Sets the value of {@link CodeDeployEcsDeployActionProps#getVariablesNamespace}
* @param variablesNamespace The name of the namespace to use for variables emitted by this action.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder variablesNamespace(java.lang.String variablesNamespace) {
this.variablesNamespace = variablesNamespace;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link CodeDeployEcsDeployActionProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public CodeDeployEcsDeployActionProps build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link CodeDeployEcsDeployActionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements CodeDeployEcsDeployActionProps {
private final software.amazon.awscdk.services.codedeploy.IEcsDeploymentGroup deploymentGroup;
private final software.amazon.awscdk.services.codepipeline.ArtifactPath appSpecTemplateFile;
private final software.amazon.awscdk.services.codepipeline.Artifact appSpecTemplateInput;
private final java.util.List containerImageInputs;
private final software.amazon.awscdk.services.codepipeline.ArtifactPath taskDefinitionTemplateFile;
private final software.amazon.awscdk.services.codepipeline.Artifact taskDefinitionTemplateInput;
private final software.amazon.awscdk.services.iam.IRole role;
private final java.lang.String actionName;
private final java.lang.Number runOrder;
private final java.lang.String variablesNamespace;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.deploymentGroup = software.amazon.jsii.Kernel.get(this, "deploymentGroup", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.codedeploy.IEcsDeploymentGroup.class));
this.appSpecTemplateFile = software.amazon.jsii.Kernel.get(this, "appSpecTemplateFile", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.codepipeline.ArtifactPath.class));
this.appSpecTemplateInput = software.amazon.jsii.Kernel.get(this, "appSpecTemplateInput", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.codepipeline.Artifact.class));
this.containerImageInputs = software.amazon.jsii.Kernel.get(this, "containerImageInputs", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.codepipeline.actions.CodeDeployEcsContainerImageInput.class)));
this.taskDefinitionTemplateFile = software.amazon.jsii.Kernel.get(this, "taskDefinitionTemplateFile", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.codepipeline.ArtifactPath.class));
this.taskDefinitionTemplateInput = software.amazon.jsii.Kernel.get(this, "taskDefinitionTemplateInput", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.codepipeline.Artifact.class));
this.role = software.amazon.jsii.Kernel.get(this, "role", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.iam.IRole.class));
this.actionName = software.amazon.jsii.Kernel.get(this, "actionName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.runOrder = software.amazon.jsii.Kernel.get(this, "runOrder", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.variablesNamespace = software.amazon.jsii.Kernel.get(this, "variablesNamespace", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
@SuppressWarnings("unchecked")
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.deploymentGroup = java.util.Objects.requireNonNull(builder.deploymentGroup, "deploymentGroup is required");
this.appSpecTemplateFile = builder.appSpecTemplateFile;
this.appSpecTemplateInput = builder.appSpecTemplateInput;
this.containerImageInputs = (java.util.List)builder.containerImageInputs;
this.taskDefinitionTemplateFile = builder.taskDefinitionTemplateFile;
this.taskDefinitionTemplateInput = builder.taskDefinitionTemplateInput;
this.role = builder.role;
this.actionName = java.util.Objects.requireNonNull(builder.actionName, "actionName is required");
this.runOrder = builder.runOrder;
this.variablesNamespace = builder.variablesNamespace;
}
@Override
public final software.amazon.awscdk.services.codedeploy.IEcsDeploymentGroup getDeploymentGroup() {
return this.deploymentGroup;
}
@Override
public final software.amazon.awscdk.services.codepipeline.ArtifactPath getAppSpecTemplateFile() {
return this.appSpecTemplateFile;
}
@Override
public final software.amazon.awscdk.services.codepipeline.Artifact getAppSpecTemplateInput() {
return this.appSpecTemplateInput;
}
@Override
public final java.util.List getContainerImageInputs() {
return this.containerImageInputs;
}
@Override
public final software.amazon.awscdk.services.codepipeline.ArtifactPath getTaskDefinitionTemplateFile() {
return this.taskDefinitionTemplateFile;
}
@Override
public final software.amazon.awscdk.services.codepipeline.Artifact getTaskDefinitionTemplateInput() {
return this.taskDefinitionTemplateInput;
}
@Override
public final software.amazon.awscdk.services.iam.IRole getRole() {
return this.role;
}
@Override
public final java.lang.String getActionName() {
return this.actionName;
}
@Override
public final java.lang.Number getRunOrder() {
return this.runOrder;
}
@Override
public final java.lang.String getVariablesNamespace() {
return this.variablesNamespace;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("deploymentGroup", om.valueToTree(this.getDeploymentGroup()));
if (this.getAppSpecTemplateFile() != null) {
data.set("appSpecTemplateFile", om.valueToTree(this.getAppSpecTemplateFile()));
}
if (this.getAppSpecTemplateInput() != null) {
data.set("appSpecTemplateInput", om.valueToTree(this.getAppSpecTemplateInput()));
}
if (this.getContainerImageInputs() != null) {
data.set("containerImageInputs", om.valueToTree(this.getContainerImageInputs()));
}
if (this.getTaskDefinitionTemplateFile() != null) {
data.set("taskDefinitionTemplateFile", om.valueToTree(this.getTaskDefinitionTemplateFile()));
}
if (this.getTaskDefinitionTemplateInput() != null) {
data.set("taskDefinitionTemplateInput", om.valueToTree(this.getTaskDefinitionTemplateInput()));
}
if (this.getRole() != null) {
data.set("role", om.valueToTree(this.getRole()));
}
data.set("actionName", om.valueToTree(this.getActionName()));
if (this.getRunOrder() != null) {
data.set("runOrder", om.valueToTree(this.getRunOrder()));
}
if (this.getVariablesNamespace() != null) {
data.set("variablesNamespace", om.valueToTree(this.getVariablesNamespace()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-codepipeline-actions.CodeDeployEcsDeployActionProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
CodeDeployEcsDeployActionProps.Jsii$Proxy that = (CodeDeployEcsDeployActionProps.Jsii$Proxy) o;
if (!deploymentGroup.equals(that.deploymentGroup)) return false;
if (this.appSpecTemplateFile != null ? !this.appSpecTemplateFile.equals(that.appSpecTemplateFile) : that.appSpecTemplateFile != null) return false;
if (this.appSpecTemplateInput != null ? !this.appSpecTemplateInput.equals(that.appSpecTemplateInput) : that.appSpecTemplateInput != null) return false;
if (this.containerImageInputs != null ? !this.containerImageInputs.equals(that.containerImageInputs) : that.containerImageInputs != null) return false;
if (this.taskDefinitionTemplateFile != null ? !this.taskDefinitionTemplateFile.equals(that.taskDefinitionTemplateFile) : that.taskDefinitionTemplateFile != null) return false;
if (this.taskDefinitionTemplateInput != null ? !this.taskDefinitionTemplateInput.equals(that.taskDefinitionTemplateInput) : that.taskDefinitionTemplateInput != null) return false;
if (this.role != null ? !this.role.equals(that.role) : that.role != null) return false;
if (!actionName.equals(that.actionName)) return false;
if (this.runOrder != null ? !this.runOrder.equals(that.runOrder) : that.runOrder != null) return false;
return this.variablesNamespace != null ? this.variablesNamespace.equals(that.variablesNamespace) : that.variablesNamespace == null;
}
@Override
public final int hashCode() {
int result = this.deploymentGroup.hashCode();
result = 31 * result + (this.appSpecTemplateFile != null ? this.appSpecTemplateFile.hashCode() : 0);
result = 31 * result + (this.appSpecTemplateInput != null ? this.appSpecTemplateInput.hashCode() : 0);
result = 31 * result + (this.containerImageInputs != null ? this.containerImageInputs.hashCode() : 0);
result = 31 * result + (this.taskDefinitionTemplateFile != null ? this.taskDefinitionTemplateFile.hashCode() : 0);
result = 31 * result + (this.taskDefinitionTemplateInput != null ? this.taskDefinitionTemplateInput.hashCode() : 0);
result = 31 * result + (this.role != null ? this.role.hashCode() : 0);
result = 31 * result + (this.actionName.hashCode());
result = 31 * result + (this.runOrder != null ? this.runOrder.hashCode() : 0);
result = 31 * result + (this.variablesNamespace != null ? this.variablesNamespace.hashCode() : 0);
return result;
}
}
}