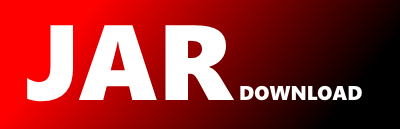
software.amazon.awscdk.services.codepipeline.actions.CodeDeployServerDeployAction Maven / Gradle / Ivy
Show all versions of codepipeline-actions Show documentation
package software.amazon.awscdk.services.codepipeline.actions;
/**
* Example:
*
*
* ServerDeploymentGroup deploymentGroup;
* Pipeline pipeline = Pipeline.Builder.create(this, "MyPipeline")
* .pipelineName("MyPipeline")
* .build();
* // add the source and build Stages to the Pipeline...
* Artifact buildOutput = new Artifact();
* CodeDeployServerDeployAction deployAction = CodeDeployServerDeployAction.Builder.create()
* .actionName("CodeDeploy")
* .input(buildOutput)
* .deploymentGroup(deploymentGroup)
* .build();
* pipeline.addStage(StageOptions.builder()
* .stageName("Deploy")
* .actions(List.of(deployAction))
* .build());
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.74.0 (build 6d08790)", date = "2023-05-19T23:09:34.862Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.codepipeline.actions.$Module.class, fqn = "@aws-cdk/aws-codepipeline-actions.CodeDeployServerDeployAction")
public class CodeDeployServerDeployAction extends software.amazon.awscdk.services.codepipeline.actions.Action {
protected CodeDeployServerDeployAction(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected CodeDeployServerDeployAction(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
/**
* @param props This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public CodeDeployServerDeployAction(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.codepipeline.actions.CodeDeployServerDeployActionProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* This is a renamed version of the {@link IAction.bind} method.
*
* @param _scope This parameter is required.
* @param _stage This parameter is required.
* @param options This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
protected @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.codepipeline.ActionConfig bound(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.Construct _scope, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.codepipeline.IStage _stage, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.codepipeline.ActionBindOptions options) {
return software.amazon.jsii.Kernel.call(this, "bound", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.codepipeline.ActionConfig.class), new Object[] { java.util.Objects.requireNonNull(_scope, "_scope is required"), java.util.Objects.requireNonNull(_stage, "_stage is required"), java.util.Objects.requireNonNull(options, "options is required") });
}
/**
* A fluent builder for {@link software.amazon.awscdk.services.codepipeline.actions.CodeDeployServerDeployAction}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static Builder create() {
return new Builder();
}
private final software.amazon.awscdk.services.codepipeline.actions.CodeDeployServerDeployActionProps.Builder props;
private Builder() {
this.props = new software.amazon.awscdk.services.codepipeline.actions.CodeDeployServerDeployActionProps.Builder();
}
/**
* The physical, human-readable name of the Action.
*
* Note that Action names must be unique within a single Stage.
*
* @return {@code this}
* @param actionName The physical, human-readable name of the Action. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder actionName(final java.lang.String actionName) {
this.props.actionName(actionName);
return this;
}
/**
* The runOrder property for this Action.
*
* RunOrder determines the relative order in which multiple Actions in the same Stage execute.
*
* Default: 1
*
* @return {@code this}
* @see https://docs.aws.amazon.com/codepipeline/latest/userguide/reference-pipeline-structure.html
* @param runOrder The runOrder property for this Action. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder runOrder(final java.lang.Number runOrder) {
this.props.runOrder(runOrder);
return this;
}
/**
* The name of the namespace to use for variables emitted by this action.
*
* Default: - a name will be generated, based on the stage and action names,
* if any of the action's variables were referenced - otherwise,
* no namespace will be set
*
* @return {@code this}
* @param variablesNamespace The name of the namespace to use for variables emitted by this action. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder variablesNamespace(final java.lang.String variablesNamespace) {
this.props.variablesNamespace(variablesNamespace);
return this;
}
/**
* The Role in which context's this Action will be executing in.
*
* The Pipeline's Role will assume this Role
* (the required permissions for that will be granted automatically)
* right before executing this Action.
* This Action will be passed into your {@link IAction.bind}
* method in the {@link ActionBindOptions.role} property.
*
* Default: a new Role will be generated
*
* @return {@code this}
* @param role The Role in which context's this Action will be executing in. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder role(final software.amazon.awscdk.services.iam.IRole role) {
this.props.role(role);
return this;
}
/**
* The CodeDeploy server Deployment Group to deploy to.
*
* @return {@code this}
* @param deploymentGroup The CodeDeploy server Deployment Group to deploy to. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder deploymentGroup(final software.amazon.awscdk.services.codedeploy.IServerDeploymentGroup deploymentGroup) {
this.props.deploymentGroup(deploymentGroup);
return this;
}
/**
* The source to use as input for deployment.
*
* @return {@code this}
* @param input The source to use as input for deployment. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder input(final software.amazon.awscdk.services.codepipeline.Artifact input) {
this.props.input(input);
return this;
}
/**
* @returns a newly built instance of {@link software.amazon.awscdk.services.codepipeline.actions.CodeDeployServerDeployAction}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public software.amazon.awscdk.services.codepipeline.actions.CodeDeployServerDeployAction build() {
return new software.amazon.awscdk.services.codepipeline.actions.CodeDeployServerDeployAction(
this.props.build()
);
}
}
}