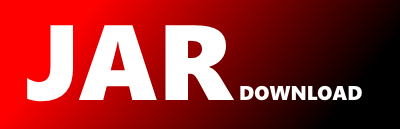
software.amazon.awscdk.services.codepipeline.actions.EcrSourceVariables Maven / Gradle / Ivy
Show all versions of codepipeline-actions Show documentation
package software.amazon.awscdk.services.codepipeline.actions;
/**
* The CodePipeline variables emitted by the ECR source Action.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.codepipeline.actions.*;
* EcrSourceVariables ecrSourceVariables = EcrSourceVariables.builder()
* .imageDigest("imageDigest")
* .imageTag("imageTag")
* .imageUri("imageUri")
* .registryId("registryId")
* .repositoryName("repositoryName")
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.74.0 (build 6d08790)", date = "2023-05-19T23:09:34.878Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.codepipeline.actions.$Module.class, fqn = "@aws-cdk/aws-codepipeline-actions.EcrSourceVariables")
@software.amazon.jsii.Jsii.Proxy(EcrSourceVariables.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface EcrSourceVariables extends software.amazon.jsii.JsiiSerializable {
/**
* The digest of the current image, in the form ':'.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getImageDigest();
/**
* The Docker tag of the current image.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getImageTag();
/**
* The full ECR Docker URI of the current image.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getImageUri();
/**
* The identifier of the registry.
*
* In ECR, this is usually the ID of the AWS account owning it.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getRegistryId();
/**
* The physical name of the repository that this action tracks.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull java.lang.String getRepositoryName();
/**
* @return a {@link Builder} of {@link EcrSourceVariables}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link EcrSourceVariables}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String imageDigest;
java.lang.String imageTag;
java.lang.String imageUri;
java.lang.String registryId;
java.lang.String repositoryName;
/**
* Sets the value of {@link EcrSourceVariables#getImageDigest}
* @param imageDigest The digest of the current image, in the form ':'. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder imageDigest(java.lang.String imageDigest) {
this.imageDigest = imageDigest;
return this;
}
/**
* Sets the value of {@link EcrSourceVariables#getImageTag}
* @param imageTag The Docker tag of the current image. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder imageTag(java.lang.String imageTag) {
this.imageTag = imageTag;
return this;
}
/**
* Sets the value of {@link EcrSourceVariables#getImageUri}
* @param imageUri The full ECR Docker URI of the current image. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder imageUri(java.lang.String imageUri) {
this.imageUri = imageUri;
return this;
}
/**
* Sets the value of {@link EcrSourceVariables#getRegistryId}
* @param registryId The identifier of the registry. This parameter is required.
* In ECR, this is usually the ID of the AWS account owning it.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder registryId(java.lang.String registryId) {
this.registryId = registryId;
return this;
}
/**
* Sets the value of {@link EcrSourceVariables#getRepositoryName}
* @param repositoryName The physical name of the repository that this action tracks. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder repositoryName(java.lang.String repositoryName) {
this.repositoryName = repositoryName;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link EcrSourceVariables}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public EcrSourceVariables build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link EcrSourceVariables}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements EcrSourceVariables {
private final java.lang.String imageDigest;
private final java.lang.String imageTag;
private final java.lang.String imageUri;
private final java.lang.String registryId;
private final java.lang.String repositoryName;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.imageDigest = software.amazon.jsii.Kernel.get(this, "imageDigest", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.imageTag = software.amazon.jsii.Kernel.get(this, "imageTag", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.imageUri = software.amazon.jsii.Kernel.get(this, "imageUri", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.registryId = software.amazon.jsii.Kernel.get(this, "registryId", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.repositoryName = software.amazon.jsii.Kernel.get(this, "repositoryName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.imageDigest = java.util.Objects.requireNonNull(builder.imageDigest, "imageDigest is required");
this.imageTag = java.util.Objects.requireNonNull(builder.imageTag, "imageTag is required");
this.imageUri = java.util.Objects.requireNonNull(builder.imageUri, "imageUri is required");
this.registryId = java.util.Objects.requireNonNull(builder.registryId, "registryId is required");
this.repositoryName = java.util.Objects.requireNonNull(builder.repositoryName, "repositoryName is required");
}
@Override
public final java.lang.String getImageDigest() {
return this.imageDigest;
}
@Override
public final java.lang.String getImageTag() {
return this.imageTag;
}
@Override
public final java.lang.String getImageUri() {
return this.imageUri;
}
@Override
public final java.lang.String getRegistryId() {
return this.registryId;
}
@Override
public final java.lang.String getRepositoryName() {
return this.repositoryName;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("imageDigest", om.valueToTree(this.getImageDigest()));
data.set("imageTag", om.valueToTree(this.getImageTag()));
data.set("imageUri", om.valueToTree(this.getImageUri()));
data.set("registryId", om.valueToTree(this.getRegistryId()));
data.set("repositoryName", om.valueToTree(this.getRepositoryName()));
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-codepipeline-actions.EcrSourceVariables"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
EcrSourceVariables.Jsii$Proxy that = (EcrSourceVariables.Jsii$Proxy) o;
if (!imageDigest.equals(that.imageDigest)) return false;
if (!imageTag.equals(that.imageTag)) return false;
if (!imageUri.equals(that.imageUri)) return false;
if (!registryId.equals(that.registryId)) return false;
return this.repositoryName.equals(that.repositoryName);
}
@Override
public final int hashCode() {
int result = this.imageDigest.hashCode();
result = 31 * result + (this.imageTag.hashCode());
result = 31 * result + (this.imageUri.hashCode());
result = 31 * result + (this.registryId.hashCode());
result = 31 * result + (this.repositoryName.hashCode());
return result;
}
}
}