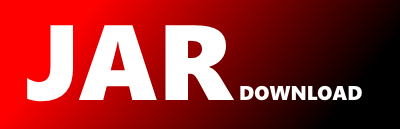
software.amazon.awscdk.services.codepipeline.actions.ManualApprovalActionProps Maven / Gradle / Ivy
Show all versions of codepipeline-actions Show documentation
package software.amazon.awscdk.services.codepipeline.actions;
/**
* Construction properties of the {@link ManualApprovalAction}.
*
* Example:
*
*
* Pipeline pipeline = new Pipeline(this, "MyPipeline");
* IStage approveStage = pipeline.addStage(StageOptions.builder().stageName("Approve").build());
* ManualApprovalAction manualApprovalAction = ManualApprovalAction.Builder.create()
* .actionName("Approve")
* .build();
* approveStage.addAction(manualApprovalAction);
* IRole role = Role.fromRoleArn(this, "Admin", Arn.format(ArnComponents.builder().service("iam").resource("role").resourceName("Admin").build(), this));
* manualApprovalAction.grantManualApproval(role);
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.74.0 (build 6d08790)", date = "2023-05-19T23:09:34.906Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.codepipeline.actions.$Module.class, fqn = "@aws-cdk/aws-codepipeline-actions.ManualApprovalActionProps")
@software.amazon.jsii.Jsii.Proxy(ManualApprovalActionProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface ManualApprovalActionProps extends software.amazon.jsii.JsiiSerializable, software.amazon.awscdk.services.codepipeline.CommonAwsActionProps {
/**
* Any additional information that you want to include in the notification email message.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getAdditionalInformation() {
return null;
}
/**
* URL you want to provide to the reviewer as part of the approval request.
*
* Default: - the approval request will not have an external link
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getExternalEntityLink() {
return null;
}
/**
* Optional SNS topic to send notifications to when an approval is pending.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.sns.ITopic getNotificationTopic() {
return null;
}
/**
* A list of email addresses to subscribe to notifications when this Action is pending approval.
*
* If this has been provided, but not notificationTopic
,
* a new Topic will be created.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getNotifyEmails() {
return null;
}
/**
* @return a {@link Builder} of {@link ManualApprovalActionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link ManualApprovalActionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.String additionalInformation;
java.lang.String externalEntityLink;
software.amazon.awscdk.services.sns.ITopic notificationTopic;
java.util.List notifyEmails;
software.amazon.awscdk.services.iam.IRole role;
java.lang.String actionName;
java.lang.Number runOrder;
java.lang.String variablesNamespace;
/**
* Sets the value of {@link ManualApprovalActionProps#getAdditionalInformation}
* @param additionalInformation Any additional information that you want to include in the notification email message.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder additionalInformation(java.lang.String additionalInformation) {
this.additionalInformation = additionalInformation;
return this;
}
/**
* Sets the value of {@link ManualApprovalActionProps#getExternalEntityLink}
* @param externalEntityLink URL you want to provide to the reviewer as part of the approval request.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder externalEntityLink(java.lang.String externalEntityLink) {
this.externalEntityLink = externalEntityLink;
return this;
}
/**
* Sets the value of {@link ManualApprovalActionProps#getNotificationTopic}
* @param notificationTopic Optional SNS topic to send notifications to when an approval is pending.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder notificationTopic(software.amazon.awscdk.services.sns.ITopic notificationTopic) {
this.notificationTopic = notificationTopic;
return this;
}
/**
* Sets the value of {@link ManualApprovalActionProps#getNotifyEmails}
* @param notifyEmails A list of email addresses to subscribe to notifications when this Action is pending approval.
* If this has been provided, but not notificationTopic
,
* a new Topic will be created.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder notifyEmails(java.util.List notifyEmails) {
this.notifyEmails = notifyEmails;
return this;
}
/**
* Sets the value of {@link ManualApprovalActionProps#getRole}
* @param role The Role in which context's this Action will be executing in.
* The Pipeline's Role will assume this Role
* (the required permissions for that will be granted automatically)
* right before executing this Action.
* This Action will be passed into your {@link IAction.bind}
* method in the {@link ActionBindOptions.role} property.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder role(software.amazon.awscdk.services.iam.IRole role) {
this.role = role;
return this;
}
/**
* Sets the value of {@link ManualApprovalActionProps#getActionName}
* @param actionName The physical, human-readable name of the Action. This parameter is required.
* Note that Action names must be unique within a single Stage.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder actionName(java.lang.String actionName) {
this.actionName = actionName;
return this;
}
/**
* Sets the value of {@link ManualApprovalActionProps#getRunOrder}
* @param runOrder The runOrder property for this Action.
* RunOrder determines the relative order in which multiple Actions in the same Stage execute.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder runOrder(java.lang.Number runOrder) {
this.runOrder = runOrder;
return this;
}
/**
* Sets the value of {@link ManualApprovalActionProps#getVariablesNamespace}
* @param variablesNamespace The name of the namespace to use for variables emitted by this action.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder variablesNamespace(java.lang.String variablesNamespace) {
this.variablesNamespace = variablesNamespace;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link ManualApprovalActionProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public ManualApprovalActionProps build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link ManualApprovalActionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements ManualApprovalActionProps {
private final java.lang.String additionalInformation;
private final java.lang.String externalEntityLink;
private final software.amazon.awscdk.services.sns.ITopic notificationTopic;
private final java.util.List notifyEmails;
private final software.amazon.awscdk.services.iam.IRole role;
private final java.lang.String actionName;
private final java.lang.Number runOrder;
private final java.lang.String variablesNamespace;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.additionalInformation = software.amazon.jsii.Kernel.get(this, "additionalInformation", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.externalEntityLink = software.amazon.jsii.Kernel.get(this, "externalEntityLink", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.notificationTopic = software.amazon.jsii.Kernel.get(this, "notificationTopic", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.sns.ITopic.class));
this.notifyEmails = software.amazon.jsii.Kernel.get(this, "notifyEmails", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(java.lang.String.class)));
this.role = software.amazon.jsii.Kernel.get(this, "role", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.iam.IRole.class));
this.actionName = software.amazon.jsii.Kernel.get(this, "actionName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.runOrder = software.amazon.jsii.Kernel.get(this, "runOrder", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.variablesNamespace = software.amazon.jsii.Kernel.get(this, "variablesNamespace", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.additionalInformation = builder.additionalInformation;
this.externalEntityLink = builder.externalEntityLink;
this.notificationTopic = builder.notificationTopic;
this.notifyEmails = builder.notifyEmails;
this.role = builder.role;
this.actionName = java.util.Objects.requireNonNull(builder.actionName, "actionName is required");
this.runOrder = builder.runOrder;
this.variablesNamespace = builder.variablesNamespace;
}
@Override
public final java.lang.String getAdditionalInformation() {
return this.additionalInformation;
}
@Override
public final java.lang.String getExternalEntityLink() {
return this.externalEntityLink;
}
@Override
public final software.amazon.awscdk.services.sns.ITopic getNotificationTopic() {
return this.notificationTopic;
}
@Override
public final java.util.List getNotifyEmails() {
return this.notifyEmails;
}
@Override
public final software.amazon.awscdk.services.iam.IRole getRole() {
return this.role;
}
@Override
public final java.lang.String getActionName() {
return this.actionName;
}
@Override
public final java.lang.Number getRunOrder() {
return this.runOrder;
}
@Override
public final java.lang.String getVariablesNamespace() {
return this.variablesNamespace;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getAdditionalInformation() != null) {
data.set("additionalInformation", om.valueToTree(this.getAdditionalInformation()));
}
if (this.getExternalEntityLink() != null) {
data.set("externalEntityLink", om.valueToTree(this.getExternalEntityLink()));
}
if (this.getNotificationTopic() != null) {
data.set("notificationTopic", om.valueToTree(this.getNotificationTopic()));
}
if (this.getNotifyEmails() != null) {
data.set("notifyEmails", om.valueToTree(this.getNotifyEmails()));
}
if (this.getRole() != null) {
data.set("role", om.valueToTree(this.getRole()));
}
data.set("actionName", om.valueToTree(this.getActionName()));
if (this.getRunOrder() != null) {
data.set("runOrder", om.valueToTree(this.getRunOrder()));
}
if (this.getVariablesNamespace() != null) {
data.set("variablesNamespace", om.valueToTree(this.getVariablesNamespace()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-codepipeline-actions.ManualApprovalActionProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
ManualApprovalActionProps.Jsii$Proxy that = (ManualApprovalActionProps.Jsii$Proxy) o;
if (this.additionalInformation != null ? !this.additionalInformation.equals(that.additionalInformation) : that.additionalInformation != null) return false;
if (this.externalEntityLink != null ? !this.externalEntityLink.equals(that.externalEntityLink) : that.externalEntityLink != null) return false;
if (this.notificationTopic != null ? !this.notificationTopic.equals(that.notificationTopic) : that.notificationTopic != null) return false;
if (this.notifyEmails != null ? !this.notifyEmails.equals(that.notifyEmails) : that.notifyEmails != null) return false;
if (this.role != null ? !this.role.equals(that.role) : that.role != null) return false;
if (!actionName.equals(that.actionName)) return false;
if (this.runOrder != null ? !this.runOrder.equals(that.runOrder) : that.runOrder != null) return false;
return this.variablesNamespace != null ? this.variablesNamespace.equals(that.variablesNamespace) : that.variablesNamespace == null;
}
@Override
public final int hashCode() {
int result = this.additionalInformation != null ? this.additionalInformation.hashCode() : 0;
result = 31 * result + (this.externalEntityLink != null ? this.externalEntityLink.hashCode() : 0);
result = 31 * result + (this.notificationTopic != null ? this.notificationTopic.hashCode() : 0);
result = 31 * result + (this.notifyEmails != null ? this.notifyEmails.hashCode() : 0);
result = 31 * result + (this.role != null ? this.role.hashCode() : 0);
result = 31 * result + (this.actionName.hashCode());
result = 31 * result + (this.runOrder != null ? this.runOrder.hashCode() : 0);
result = 31 * result + (this.variablesNamespace != null ? this.variablesNamespace.hashCode() : 0);
return result;
}
}
}