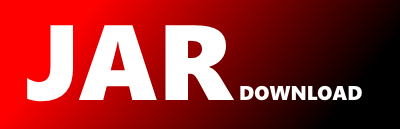
software.amazon.awscdk.services.codepipeline.actions.S3DeployActionProps Maven / Gradle / Ivy
Show all versions of codepipeline-actions Show documentation
package software.amazon.awscdk.services.codepipeline.actions;
/**
* Construction properties of the {@link S3DeployAction S3 deploy Action}.
*
* Example:
*
*
* Artifact sourceOutput = new Artifact();
* Bucket targetBucket = new Bucket(this, "MyBucket");
* Pipeline pipeline = new Pipeline(this, "MyPipeline");
* S3DeployAction deployAction = S3DeployAction.Builder.create()
* .actionName("S3Deploy")
* .bucket(targetBucket)
* .input(sourceOutput)
* .build();
* IStage deployStage = pipeline.addStage(StageOptions.builder()
* .stageName("Deploy")
* .actions(List.of(deployAction))
* .build());
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.74.0 (build 6d08790)", date = "2023-05-19T23:09:34.910Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.codepipeline.actions.$Module.class, fqn = "@aws-cdk/aws-codepipeline-actions.S3DeployActionProps")
@software.amazon.jsii.Jsii.Proxy(S3DeployActionProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public interface S3DeployActionProps extends software.amazon.jsii.JsiiSerializable, software.amazon.awscdk.services.codepipeline.CommonAwsActionProps {
/**
* The Amazon S3 bucket that is the deploy target.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull software.amazon.awscdk.services.s3.IBucket getBucket();
/**
* The input Artifact to deploy to Amazon S3.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@org.jetbrains.annotations.NotNull software.amazon.awscdk.services.codepipeline.Artifact getInput();
/**
* The specified canned ACL to objects deployed to Amazon S3.
*
* This overwrites any existing ACL that was applied to the object.
*
* Default: - the original object ACL
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.s3.BucketAccessControl getAccessControl() {
return null;
}
/**
* The caching behavior for requests/responses for objects in the bucket.
*
* The final cache control property will be the result of joining all of the provided array elements with a comma
* (plus a space after the comma).
*
* Default: - none, decided by the HTTP client
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.util.List getCacheControl() {
return null;
}
/**
* Should the deploy action extract the artifact before deploying to Amazon S3.
*
* Default: true
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.Boolean getExtract() {
return null;
}
/**
* The key of the target object.
*
* This is required if extract is false.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
default @org.jetbrains.annotations.Nullable java.lang.String getObjectKey() {
return null;
}
/**
* @return a {@link Builder} of {@link S3DeployActionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link S3DeployActionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
software.amazon.awscdk.services.s3.IBucket bucket;
software.amazon.awscdk.services.codepipeline.Artifact input;
software.amazon.awscdk.services.s3.BucketAccessControl accessControl;
java.util.List cacheControl;
java.lang.Boolean extract;
java.lang.String objectKey;
software.amazon.awscdk.services.iam.IRole role;
java.lang.String actionName;
java.lang.Number runOrder;
java.lang.String variablesNamespace;
/**
* Sets the value of {@link S3DeployActionProps#getBucket}
* @param bucket The Amazon S3 bucket that is the deploy target. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder bucket(software.amazon.awscdk.services.s3.IBucket bucket) {
this.bucket = bucket;
return this;
}
/**
* Sets the value of {@link S3DeployActionProps#getInput}
* @param input The input Artifact to deploy to Amazon S3. This parameter is required.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder input(software.amazon.awscdk.services.codepipeline.Artifact input) {
this.input = input;
return this;
}
/**
* Sets the value of {@link S3DeployActionProps#getAccessControl}
* @param accessControl The specified canned ACL to objects deployed to Amazon S3.
* This overwrites any existing ACL that was applied to the object.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder accessControl(software.amazon.awscdk.services.s3.BucketAccessControl accessControl) {
this.accessControl = accessControl;
return this;
}
/**
* Sets the value of {@link S3DeployActionProps#getCacheControl}
* @param cacheControl The caching behavior for requests/responses for objects in the bucket.
* The final cache control property will be the result of joining all of the provided array elements with a comma
* (plus a space after the comma).
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@SuppressWarnings("unchecked")
public Builder cacheControl(java.util.List extends software.amazon.awscdk.services.codepipeline.actions.CacheControl> cacheControl) {
this.cacheControl = (java.util.List)cacheControl;
return this;
}
/**
* Sets the value of {@link S3DeployActionProps#getExtract}
* @param extract Should the deploy action extract the artifact before deploying to Amazon S3.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder extract(java.lang.Boolean extract) {
this.extract = extract;
return this;
}
/**
* Sets the value of {@link S3DeployActionProps#getObjectKey}
* @param objectKey The key of the target object.
* This is required if extract is false.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder objectKey(java.lang.String objectKey) {
this.objectKey = objectKey;
return this;
}
/**
* Sets the value of {@link S3DeployActionProps#getRole}
* @param role The Role in which context's this Action will be executing in.
* The Pipeline's Role will assume this Role
* (the required permissions for that will be granted automatically)
* right before executing this Action.
* This Action will be passed into your {@link IAction.bind}
* method in the {@link ActionBindOptions.role} property.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder role(software.amazon.awscdk.services.iam.IRole role) {
this.role = role;
return this;
}
/**
* Sets the value of {@link S3DeployActionProps#getActionName}
* @param actionName The physical, human-readable name of the Action. This parameter is required.
* Note that Action names must be unique within a single Stage.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder actionName(java.lang.String actionName) {
this.actionName = actionName;
return this;
}
/**
* Sets the value of {@link S3DeployActionProps#getRunOrder}
* @param runOrder The runOrder property for this Action.
* RunOrder determines the relative order in which multiple Actions in the same Stage execute.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder runOrder(java.lang.Number runOrder) {
this.runOrder = runOrder;
return this;
}
/**
* Sets the value of {@link S3DeployActionProps#getVariablesNamespace}
* @param variablesNamespace The name of the namespace to use for variables emitted by this action.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder variablesNamespace(java.lang.String variablesNamespace) {
this.variablesNamespace = variablesNamespace;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link S3DeployActionProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public S3DeployActionProps build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link S3DeployActionProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements S3DeployActionProps {
private final software.amazon.awscdk.services.s3.IBucket bucket;
private final software.amazon.awscdk.services.codepipeline.Artifact input;
private final software.amazon.awscdk.services.s3.BucketAccessControl accessControl;
private final java.util.List cacheControl;
private final java.lang.Boolean extract;
private final java.lang.String objectKey;
private final software.amazon.awscdk.services.iam.IRole role;
private final java.lang.String actionName;
private final java.lang.Number runOrder;
private final java.lang.String variablesNamespace;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.bucket = software.amazon.jsii.Kernel.get(this, "bucket", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.s3.IBucket.class));
this.input = software.amazon.jsii.Kernel.get(this, "input", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.codepipeline.Artifact.class));
this.accessControl = software.amazon.jsii.Kernel.get(this, "accessControl", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.s3.BucketAccessControl.class));
this.cacheControl = software.amazon.jsii.Kernel.get(this, "cacheControl", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.codepipeline.actions.CacheControl.class)));
this.extract = software.amazon.jsii.Kernel.get(this, "extract", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.objectKey = software.amazon.jsii.Kernel.get(this, "objectKey", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.role = software.amazon.jsii.Kernel.get(this, "role", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.iam.IRole.class));
this.actionName = software.amazon.jsii.Kernel.get(this, "actionName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.runOrder = software.amazon.jsii.Kernel.get(this, "runOrder", software.amazon.jsii.NativeType.forClass(java.lang.Number.class));
this.variablesNamespace = software.amazon.jsii.Kernel.get(this, "variablesNamespace", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
@SuppressWarnings("unchecked")
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.bucket = java.util.Objects.requireNonNull(builder.bucket, "bucket is required");
this.input = java.util.Objects.requireNonNull(builder.input, "input is required");
this.accessControl = builder.accessControl;
this.cacheControl = (java.util.List)builder.cacheControl;
this.extract = builder.extract;
this.objectKey = builder.objectKey;
this.role = builder.role;
this.actionName = java.util.Objects.requireNonNull(builder.actionName, "actionName is required");
this.runOrder = builder.runOrder;
this.variablesNamespace = builder.variablesNamespace;
}
@Override
public final software.amazon.awscdk.services.s3.IBucket getBucket() {
return this.bucket;
}
@Override
public final software.amazon.awscdk.services.codepipeline.Artifact getInput() {
return this.input;
}
@Override
public final software.amazon.awscdk.services.s3.BucketAccessControl getAccessControl() {
return this.accessControl;
}
@Override
public final java.util.List getCacheControl() {
return this.cacheControl;
}
@Override
public final java.lang.Boolean getExtract() {
return this.extract;
}
@Override
public final java.lang.String getObjectKey() {
return this.objectKey;
}
@Override
public final software.amazon.awscdk.services.iam.IRole getRole() {
return this.role;
}
@Override
public final java.lang.String getActionName() {
return this.actionName;
}
@Override
public final java.lang.Number getRunOrder() {
return this.runOrder;
}
@Override
public final java.lang.String getVariablesNamespace() {
return this.variablesNamespace;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
data.set("bucket", om.valueToTree(this.getBucket()));
data.set("input", om.valueToTree(this.getInput()));
if (this.getAccessControl() != null) {
data.set("accessControl", om.valueToTree(this.getAccessControl()));
}
if (this.getCacheControl() != null) {
data.set("cacheControl", om.valueToTree(this.getCacheControl()));
}
if (this.getExtract() != null) {
data.set("extract", om.valueToTree(this.getExtract()));
}
if (this.getObjectKey() != null) {
data.set("objectKey", om.valueToTree(this.getObjectKey()));
}
if (this.getRole() != null) {
data.set("role", om.valueToTree(this.getRole()));
}
data.set("actionName", om.valueToTree(this.getActionName()));
if (this.getRunOrder() != null) {
data.set("runOrder", om.valueToTree(this.getRunOrder()));
}
if (this.getVariablesNamespace() != null) {
data.set("variablesNamespace", om.valueToTree(this.getVariablesNamespace()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-codepipeline-actions.S3DeployActionProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
S3DeployActionProps.Jsii$Proxy that = (S3DeployActionProps.Jsii$Proxy) o;
if (!bucket.equals(that.bucket)) return false;
if (!input.equals(that.input)) return false;
if (this.accessControl != null ? !this.accessControl.equals(that.accessControl) : that.accessControl != null) return false;
if (this.cacheControl != null ? !this.cacheControl.equals(that.cacheControl) : that.cacheControl != null) return false;
if (this.extract != null ? !this.extract.equals(that.extract) : that.extract != null) return false;
if (this.objectKey != null ? !this.objectKey.equals(that.objectKey) : that.objectKey != null) return false;
if (this.role != null ? !this.role.equals(that.role) : that.role != null) return false;
if (!actionName.equals(that.actionName)) return false;
if (this.runOrder != null ? !this.runOrder.equals(that.runOrder) : that.runOrder != null) return false;
return this.variablesNamespace != null ? this.variablesNamespace.equals(that.variablesNamespace) : that.variablesNamespace == null;
}
@Override
public final int hashCode() {
int result = this.bucket.hashCode();
result = 31 * result + (this.input.hashCode());
result = 31 * result + (this.accessControl != null ? this.accessControl.hashCode() : 0);
result = 31 * result + (this.cacheControl != null ? this.cacheControl.hashCode() : 0);
result = 31 * result + (this.extract != null ? this.extract.hashCode() : 0);
result = 31 * result + (this.objectKey != null ? this.objectKey.hashCode() : 0);
result = 31 * result + (this.role != null ? this.role.hashCode() : 0);
result = 31 * result + (this.actionName.hashCode());
result = 31 * result + (this.runOrder != null ? this.runOrder.hashCode() : 0);
result = 31 * result + (this.variablesNamespace != null ? this.variablesNamespace.hashCode() : 0);
return result;
}
}
}