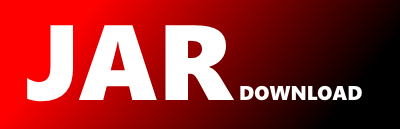
software.amazon.awscdk.services.codepipeline.actions.StackInstances Maven / Gradle / Ivy
Show all versions of codepipeline-actions Show documentation
package software.amazon.awscdk.services.codepipeline.actions;
/**
* Where Stack Instances will be created from the StackSet.
*
* Example:
*
*
* Pipeline pipeline;
* Artifact sourceOutput;
* pipeline.addStage(StageOptions.builder()
* .stageName("DeployStackSets")
* .actions(List.of(
* // First, update the StackSet itself with the newest template
* CloudFormationDeployStackSetAction.Builder.create()
* .actionName("UpdateStackSet")
* .runOrder(1)
* .stackSetName("MyStackSet")
* .template(StackSetTemplate.fromArtifactPath(sourceOutput.atPath("template.yaml")))
* // Change this to 'StackSetDeploymentModel.organizations()' if you want to deploy to OUs
* .deploymentModel(StackSetDeploymentModel.selfManaged())
* // This deploys to a set of accounts
* .stackInstances(StackInstances.inAccounts(List.of("111111111111"), List.of("us-east-1", "eu-west-1")))
* .build(),
* // Afterwards, update/create additional instances in other accounts
* CloudFormationDeployStackInstancesAction.Builder.create()
* .actionName("AddMoreInstances")
* .runOrder(2)
* .stackSetName("MyStackSet")
* .stackInstances(StackInstances.inAccounts(List.of("222222222222", "333333333333"), List.of("us-east-1", "eu-west-1")))
* .build()))
* .build());
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.74.0 (build 6d08790)", date = "2023-05-19T23:09:34.945Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.codepipeline.actions.$Module.class, fqn = "@aws-cdk/aws-codepipeline-actions.StackInstances")
public abstract class StackInstances extends software.amazon.jsii.JsiiObject {
protected StackInstances(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected StackInstances(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
protected StackInstances() {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this);
}
/**
* Create stack instances in a set of accounts or organizational units taken from the pipeline artifacts, and a set of regions The file must be a JSON file containing a list of strings.
*
* For example:
*
*
* [
* "111111111111",
* "222222222222",
* "333333333333"
* ]
*
*
* Stack Instances will be created in every combination of region and account, or region and
* Organizational Units (OUs).
*
* If this is set of Organizational Units, you must have selected StackSetDeploymentModel.organizations()
* as deployment model.
*
* @param artifactPath This parameter is required.
* @param regions This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.codepipeline.actions.StackInstances fromArtifactPath(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.codepipeline.ArtifactPath artifactPath, final @org.jetbrains.annotations.NotNull java.util.List regions) {
return software.amazon.jsii.JsiiObject.jsiiStaticCall(software.amazon.awscdk.services.codepipeline.actions.StackInstances.class, "fromArtifactPath", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.codepipeline.actions.StackInstances.class), new Object[] { java.util.Objects.requireNonNull(artifactPath, "artifactPath is required"), java.util.Objects.requireNonNull(regions, "regions is required") });
}
/**
* Create stack instances in a set of accounts and regions passed as literal lists.
*
* Stack Instances will be created in every combination of region and account.
*
*
*
* NOTE: StackInstances.inAccounts()
and StackInstances.inOrganizationalUnits()
* have exactly the same behavior, and you can use them interchangeably if you want.
* The only difference between them is that your code clearly indicates what entity
* it's working with.
*
*
*
* @param accounts This parameter is required.
* @param regions This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.codepipeline.actions.StackInstances inAccounts(final @org.jetbrains.annotations.NotNull java.util.List accounts, final @org.jetbrains.annotations.NotNull java.util.List regions) {
return software.amazon.jsii.JsiiObject.jsiiStaticCall(software.amazon.awscdk.services.codepipeline.actions.StackInstances.class, "inAccounts", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.codepipeline.actions.StackInstances.class), new Object[] { java.util.Objects.requireNonNull(accounts, "accounts is required"), java.util.Objects.requireNonNull(regions, "regions is required") });
}
/**
* Create stack instances in all accounts in a set of Organizational Units (OUs) and regions passed as literal lists.
*
* If you want to deploy to Organization Units, you must choose have created the StackSet
* with deploymentModel: DeploymentModel.organizations()
.
*
* Stack Instances will be created in every combination of region and account.
*
*
*
* NOTE: StackInstances.inAccounts()
and StackInstances.inOrganizationalUnits()
* have exactly the same behavior, and you can use them interchangeably if you want.
* The only difference between them is that your code clearly indicates what entity
* it's working with.
*
*
*
* @param ous This parameter is required.
* @param regions This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.codepipeline.actions.StackInstances inOrganizationalUnits(final @org.jetbrains.annotations.NotNull java.util.List ous, final @org.jetbrains.annotations.NotNull java.util.List regions) {
return software.amazon.jsii.JsiiObject.jsiiStaticCall(software.amazon.awscdk.services.codepipeline.actions.StackInstances.class, "inOrganizationalUnits", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.codepipeline.actions.StackInstances.class), new Object[] { java.util.Objects.requireNonNull(ous, "ous is required"), java.util.Objects.requireNonNull(regions, "regions is required") });
}
/**
* A proxy class which represents a concrete javascript instance of this type.
*/
@software.amazon.jsii.Internal
private static final class Jsii$Proxy extends software.amazon.awscdk.services.codepipeline.actions.StackInstances {
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
}
}