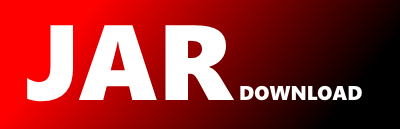
software.amazon.awscdk.services.codepipeline.actions.StackSetDeploymentModel Maven / Gradle / Ivy
Show all versions of codepipeline-actions Show documentation
package software.amazon.awscdk.services.codepipeline.actions;
/**
* Determines how IAM roles are created and managed.
*
* Example:
*
*
* Pipeline pipeline;
* Artifact sourceOutput;
* pipeline.addStage(StageOptions.builder()
* .stageName("DeployStackSets")
* .actions(List.of(
* // First, update the StackSet itself with the newest template
* CloudFormationDeployStackSetAction.Builder.create()
* .actionName("UpdateStackSet")
* .runOrder(1)
* .stackSetName("MyStackSet")
* .template(StackSetTemplate.fromArtifactPath(sourceOutput.atPath("template.yaml")))
* // Change this to 'StackSetDeploymentModel.organizations()' if you want to deploy to OUs
* .deploymentModel(StackSetDeploymentModel.selfManaged())
* // This deploys to a set of accounts
* .stackInstances(StackInstances.inAccounts(List.of("111111111111"), List.of("us-east-1", "eu-west-1")))
* .build(),
* // Afterwards, update/create additional instances in other accounts
* CloudFormationDeployStackInstancesAction.Builder.create()
* .actionName("AddMoreInstances")
* .runOrder(2)
* .stackSetName("MyStackSet")
* .stackInstances(StackInstances.inAccounts(List.of("222222222222", "333333333333"), List.of("us-east-1", "eu-west-1")))
* .build()))
* .build());
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.74.0 (build 6d08790)", date = "2023-05-19T23:09:34.947Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.codepipeline.actions.$Module.class, fqn = "@aws-cdk/aws-codepipeline-actions.StackSetDeploymentModel")
public abstract class StackSetDeploymentModel extends software.amazon.jsii.JsiiObject {
protected StackSetDeploymentModel(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected StackSetDeploymentModel(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
/**
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
protected StackSetDeploymentModel() {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this);
}
/**
* Deploy to AWS Organizations accounts.
*
* AWS CloudFormation StackSets automatically creates the IAM roles required
* to deploy to accounts managed by AWS Organizations. This requires an
* account to be a member of an Organization.
*
* Using this deployment model, you can specify either AWS Account Ids or
* Organization Unit Ids in the stackInstances
parameter.
*
* @param props
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.codepipeline.actions.StackSetDeploymentModel organizations(final @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.codepipeline.actions.OrganizationsDeploymentProps props) {
return software.amazon.jsii.JsiiObject.jsiiStaticCall(software.amazon.awscdk.services.codepipeline.actions.StackSetDeploymentModel.class, "organizations", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.codepipeline.actions.StackSetDeploymentModel.class), new Object[] { props });
}
/**
* Deploy to AWS Organizations accounts.
*
* AWS CloudFormation StackSets automatically creates the IAM roles required
* to deploy to accounts managed by AWS Organizations. This requires an
* account to be a member of an Organization.
*
* Using this deployment model, you can specify either AWS Account Ids or
* Organization Unit Ids in the stackInstances
parameter.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.codepipeline.actions.StackSetDeploymentModel organizations() {
return software.amazon.jsii.JsiiObject.jsiiStaticCall(software.amazon.awscdk.services.codepipeline.actions.StackSetDeploymentModel.class, "organizations", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.codepipeline.actions.StackSetDeploymentModel.class));
}
/**
* Deploy to AWS Accounts not managed by AWS Organizations.
*
* You are responsible for creating Execution Roles in every account you will
* be deploying to in advance to create the actual stack instances. Unless you
* specify overrides, StackSets expects the execution roles you create to have
* the default name AWSCloudFormationStackSetExecutionRole
. See the Grant
* self-managed
* permissions
* section of the CloudFormation documentation.
*
* The CDK will automatically create the central Administration Role in the
* Pipeline account which will be used to assume the Execution Role in each of
* the target accounts.
*
* If you wish to use a pre-created Administration Role, use Role.fromRoleName()
* or Role.fromRoleArn()
to import it, and pass it to this function:
*
*
* IRole existingAdminRole = Role.fromRoleName(this, "AdminRole", "AWSCloudFormationStackSetAdministrationRole");
* StackSetDeploymentModel deploymentModel = StackSetDeploymentModel.selfManaged(SelfManagedDeploymentProps.builder()
* // Use an existing Role. Leave this out to create a new Role.
* .administrationRole(existingAdminRole)
* .build());
*
*
* Using this deployment model, you can only specify AWS Account Ids in the
* stackInstances
parameter.
*
* @see https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/stacksets-prereqs-self-managed.html
* @param props
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.codepipeline.actions.StackSetDeploymentModel selfManaged(final @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.codepipeline.actions.SelfManagedDeploymentProps props) {
return software.amazon.jsii.JsiiObject.jsiiStaticCall(software.amazon.awscdk.services.codepipeline.actions.StackSetDeploymentModel.class, "selfManaged", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.codepipeline.actions.StackSetDeploymentModel.class), new Object[] { props });
}
/**
* Deploy to AWS Accounts not managed by AWS Organizations.
*
* You are responsible for creating Execution Roles in every account you will
* be deploying to in advance to create the actual stack instances. Unless you
* specify overrides, StackSets expects the execution roles you create to have
* the default name AWSCloudFormationStackSetExecutionRole
. See the Grant
* self-managed
* permissions
* section of the CloudFormation documentation.
*
* The CDK will automatically create the central Administration Role in the
* Pipeline account which will be used to assume the Execution Role in each of
* the target accounts.
*
* If you wish to use a pre-created Administration Role, use Role.fromRoleName()
* or Role.fromRoleArn()
to import it, and pass it to this function:
*
*
* IRole existingAdminRole = Role.fromRoleName(this, "AdminRole", "AWSCloudFormationStackSetAdministrationRole");
* StackSetDeploymentModel deploymentModel = StackSetDeploymentModel.selfManaged(SelfManagedDeploymentProps.builder()
* // Use an existing Role. Leave this out to create a new Role.
* .administrationRole(existingAdminRole)
* .build());
*
*
* Using this deployment model, you can only specify AWS Account Ids in the
* stackInstances
parameter.
*
* @see https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/stacksets-prereqs-self-managed.html
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.codepipeline.actions.StackSetDeploymentModel selfManaged() {
return software.amazon.jsii.JsiiObject.jsiiStaticCall(software.amazon.awscdk.services.codepipeline.actions.StackSetDeploymentModel.class, "selfManaged", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.codepipeline.actions.StackSetDeploymentModel.class));
}
/**
* A proxy class which represents a concrete javascript instance of this type.
*/
@software.amazon.jsii.Internal
private static final class Jsii$Proxy extends software.amazon.awscdk.services.codepipeline.actions.StackSetDeploymentModel {
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
}
}