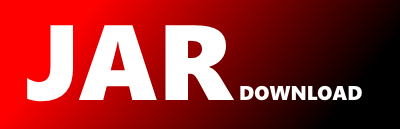
software.amazon.awscdk.services.codepipeline.actions.CodeBuildAction Maven / Gradle / Ivy
package software.amazon.awscdk.services.codepipeline.actions;
/**
* CodePipeline build action that uses AWS CodeBuild.
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.15.0 (build 585166b)", date = "2020-12-17T11:04:27.826Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.codepipeline.actions.$Module.class, fqn = "@aws-cdk/aws-codepipeline-actions.CodeBuildAction")
public class CodeBuildAction extends software.amazon.awscdk.services.codepipeline.actions.Action {
protected CodeBuildAction(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected CodeBuildAction(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
/**
* @param props This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public CodeBuildAction(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.codepipeline.actions.CodeBuildActionProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(props, "props is required") });
}
/**
* The method called when an Action is attached to a Pipeline.
*
* This method is guaranteed to be called only once for each Action instance.
*
* @param scope This parameter is required.
* @param _stage This parameter is required.
* @param options This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
protected @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.codepipeline.ActionConfig bound(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.core.Construct scope, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.codepipeline.IStage _stage, final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.codepipeline.ActionBindOptions options) {
return software.amazon.jsii.Kernel.call(this, "bound", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.codepipeline.ActionConfig.class), new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(_stage, "_stage is required"), java.util.Objects.requireNonNull(options, "options is required") });
}
/**
* Reference a CodePipeline variable defined by the CodeBuild project this action points to.
*
* Variables in CodeBuild actions are defined using the 'exported-variables' subsection of the 'env'
* section of the buildspec.
*
* @see https://docs.aws.amazon.com/codebuild/latest/userguide/build-spec-ref.html#build-spec-ref-syntax
* @param variableName the name of the variable to reference. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public @org.jetbrains.annotations.NotNull java.lang.String variable(final @org.jetbrains.annotations.NotNull java.lang.String variableName) {
return software.amazon.jsii.Kernel.call(this, "variable", software.amazon.jsii.NativeType.forClass(java.lang.String.class), new Object[] { java.util.Objects.requireNonNull(variableName, "variableName is required") });
}
/**
* A fluent builder for {@link software.amazon.awscdk.services.codepipeline.actions.CodeBuildAction}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static final class Builder implements software.amazon.jsii.Builder {
/**
* @return a new instance of {@link Builder}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public static Builder create() {
return new Builder();
}
private final software.amazon.awscdk.services.codepipeline.actions.CodeBuildActionProps.Builder props;
private Builder() {
this.props = new software.amazon.awscdk.services.codepipeline.actions.CodeBuildActionProps.Builder();
}
/**
* The physical, human-readable name of the Action.
*
* Note that Action names must be unique within a single Stage.
*
* @return {@code this}
* @param actionName The physical, human-readable name of the Action. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder actionName(final java.lang.String actionName) {
this.props.actionName(actionName);
return this;
}
/**
* The runOrder property for this Action.
*
* RunOrder determines the relative order in which multiple Actions in the same Stage execute.
*
* Default: 1
*
* @return {@code this}
* @see https://docs.aws.amazon.com/codepipeline/latest/userguide/reference-pipeline-structure.html
* @param runOrder The runOrder property for this Action. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder runOrder(final java.lang.Number runOrder) {
this.props.runOrder(runOrder);
return this;
}
/**
* The name of the namespace to use for variables emitted by this action.
*
* Default: - a name will be generated, based on the stage and action names,
* if any of the action's variables were referenced - otherwise,
* no namespace will be set
*
* @return {@code this}
* @param variablesNamespace The name of the namespace to use for variables emitted by this action. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder variablesNamespace(final java.lang.String variablesNamespace) {
this.props.variablesNamespace(variablesNamespace);
return this;
}
/**
* The Role in which context's this Action will be executing in.
*
* The Pipeline's Role will assume this Role
* (the required permissions for that will be granted automatically)
* right before executing this Action.
* This Action will be passed into your {@link IAction.bind}
* method in the {@link ActionBindOptions.role} property.
*
* Default: a new Role will be generated
*
* @return {@code this}
* @param role The Role in which context's this Action will be executing in. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder role(final software.amazon.awscdk.services.iam.IRole role) {
this.props.role(role);
return this;
}
/**
* The source to use as input for this action.
*
* @return {@code this}
* @param input The source to use as input for this action. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder input(final software.amazon.awscdk.services.codepipeline.Artifact input) {
this.props.input(input);
return this;
}
/**
* The action's Project.
*
* @return {@code this}
* @param project The action's Project. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder project(final software.amazon.awscdk.services.codebuild.IProject project) {
this.props.project(project);
return this;
}
/**
* The environment variables to pass to the CodeBuild project when this action executes.
*
* If a variable with the same name was set both on the project level, and here,
* this value will take precedence.
*
* Default: - No additional environment variables are specified.
*
* @return {@code this}
* @param environmentVariables The environment variables to pass to the CodeBuild project when this action executes. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder environmentVariables(final java.util.Map environmentVariables) {
this.props.environmentVariables(environmentVariables);
return this;
}
/**
* Trigger a batch build.
*
* Default: false
*
* @return {@code this}
* @param executeBatchBuild Trigger a batch build. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder executeBatchBuild(final java.lang.Boolean executeBatchBuild) {
this.props.executeBatchBuild(executeBatchBuild);
return this;
}
/**
* The list of additional input Artifacts for this action.
*
* The directories the additional inputs will be available at are available
* during the project's build in the CODEBUILD_SRC_DIR_ environment variables.
* The project's build always starts in the directory with the primary input artifact checked out,
* the one pointed to by the {@link input} property.
* For more information,
* see https://docs.aws.amazon.com/codebuild/latest/userguide/sample-multi-in-out.html .
*
* @return {@code this}
* @param extraInputs The list of additional input Artifacts for this action. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder extraInputs(final java.util.List extends software.amazon.awscdk.services.codepipeline.Artifact> extraInputs) {
this.props.extraInputs(extraInputs);
return this;
}
/**
* The list of output Artifacts for this action.
*
* Note: if you specify more than one output Artifact here,
* you cannot use the primary 'artifacts' section of the buildspec;
* you have to use the 'secondary-artifacts' section instead.
* See https://docs.aws.amazon.com/codebuild/latest/userguide/sample-multi-in-out.html
* for details.
*
* Default: the action will not have any outputs
*
* @return {@code this}
* @param outputs The list of output Artifacts for this action. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder outputs(final java.util.List extends software.amazon.awscdk.services.codepipeline.Artifact> outputs) {
this.props.outputs(outputs);
return this;
}
/**
* The type of the action that determines its CodePipeline Category - Build, or Test.
*
* Default: CodeBuildActionType.BUILD
*
* @return {@code this}
* @param type The type of the action that determines its CodePipeline Category - Build, or Test. This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
public Builder type(final software.amazon.awscdk.services.codepipeline.actions.CodeBuildActionType type) {
this.props.type(type);
return this;
}
/**
* @returns a newly built instance of {@link software.amazon.awscdk.services.codepipeline.actions.CodeBuildAction}.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Stable)
@Override
public software.amazon.awscdk.services.codepipeline.actions.CodeBuildAction build() {
return new software.amazon.awscdk.services.codepipeline.actions.CodeBuildAction(
this.props.build()
);
}
}
}