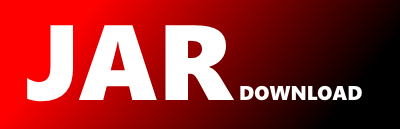
software.amazon.awscdk.services.cognito.identitypool.IdentityPool Maven / Gradle / Ivy
Show all versions of cognito-identitypool Show documentation
package software.amazon.awscdk.services.cognito.identitypool;
/**
* (experimental) Define a Cognito Identity Pool.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.cognito.identitypool.*;
* import software.amazon.awscdk.services.iam.*;
* IdentityPoolProviderUrl identityPoolProviderUrl;
* OpenIdConnectProvider openIdConnectProvider;
* Role role;
* SamlProvider samlProvider;
* UserPoolAuthenticationProvider userPoolAuthenticationProvider;
* IdentityPool identityPool = IdentityPool.Builder.create(this, "MyIdentityPool")
* .allowClassicFlow(false)
* .allowUnauthenticatedIdentities(false)
* .authenticatedRole(role)
* .authenticationProviders(IdentityPoolAuthenticationProviders.builder()
* .amazon(IdentityPoolAmazonLoginProvider.builder()
* .appId("appId")
* .build())
* .apple(IdentityPoolAppleLoginProvider.builder()
* .servicesId("servicesId")
* .build())
* .customProvider("customProvider")
* .digits(IdentityPoolDigitsLoginProvider.builder()
* .consumerKey("consumerKey")
* .consumerSecret("consumerSecret")
* .build())
* .facebook(IdentityPoolFacebookLoginProvider.builder()
* .appId("appId")
* .build())
* .google(IdentityPoolGoogleLoginProvider.builder()
* .clientId("clientId")
* .build())
* .openIdConnectProviders(List.of(openIdConnectProvider))
* .samlProviders(List.of(samlProvider))
* .twitter(IdentityPoolTwitterLoginProvider.builder()
* .consumerKey("consumerKey")
* .consumerSecret("consumerSecret")
* .build())
* .userPools(List.of(userPoolAuthenticationProvider))
* .build())
* .identityPoolName("identityPoolName")
* .roleMappings(List.of(IdentityPoolRoleMapping.builder()
* .providerUrl(identityPoolProviderUrl)
* // the properties below are optional
* .resolveAmbiguousRoles(false)
* .rules(List.of(RoleMappingRule.builder()
* .claim("claim")
* .claimValue("claimValue")
* .mappedRole(role)
* // the properties below are optional
* .matchType(RoleMappingMatchType.EQUALS)
* .build()))
* .useToken(false)
* .build()))
* .unauthenticatedRole(role)
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.52.1 (build 5ccc8f6)", date = "2022-01-27T11:49:00.782Z")
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.cognito.identitypool.$Module.class, fqn = "@aws-cdk/aws-cognito-identitypool.IdentityPool")
public class IdentityPool extends software.amazon.awscdk.core.Resource implements software.amazon.awscdk.services.cognito.identitypool.IIdentityPool {
protected IdentityPool(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
}
protected IdentityPool(final software.amazon.jsii.JsiiObject.InitializationMode initializationMode) {
super(initializationMode);
}
/**
* @param scope This parameter is required.
* @param id This parameter is required.
* @param props
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public IdentityPool(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.cognito.identitypool.IdentityPoolProps props) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), props });
}
/**
* @param scope This parameter is required.
* @param id This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public IdentityPool(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
software.amazon.jsii.JsiiEngine.getInstance().createNewObject(this, new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required") });
}
/**
* (experimental) Import an existing Identity Pool from its Arn.
*
* @param scope This parameter is required.
* @param id This parameter is required.
* @param identityPoolArn This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.cognito.identitypool.IIdentityPool fromIdentityPoolArn(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull java.lang.String identityPoolArn) {
return software.amazon.jsii.JsiiObject.jsiiStaticCall(software.amazon.awscdk.services.cognito.identitypool.IdentityPool.class, "fromIdentityPoolArn", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.cognito.identitypool.IIdentityPool.class), new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(identityPoolArn, "identityPoolArn is required") });
}
/**
* (experimental) Import an existing Identity Pool from its id.
*
* @param scope This parameter is required.
* @param id This parameter is required.
* @param identityPoolId This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.cognito.identitypool.IIdentityPool fromIdentityPoolId(final @org.jetbrains.annotations.NotNull software.constructs.Construct scope, final @org.jetbrains.annotations.NotNull java.lang.String id, final @org.jetbrains.annotations.NotNull java.lang.String identityPoolId) {
return software.amazon.jsii.JsiiObject.jsiiStaticCall(software.amazon.awscdk.services.cognito.identitypool.IdentityPool.class, "fromIdentityPoolId", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.cognito.identitypool.IIdentityPool.class), new Object[] { java.util.Objects.requireNonNull(scope, "scope is required"), java.util.Objects.requireNonNull(id, "id is required"), java.util.Objects.requireNonNull(identityPoolId, "identityPoolId is required") });
}
/**
* (experimental) Adds Role Mappings to Identity Pool.
*
* @param roleMappings This parameter is required.
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public void addRoleMappings(final @org.jetbrains.annotations.NotNull software.amazon.awscdk.services.cognito.identitypool.IdentityPoolRoleMapping... roleMappings) {
software.amazon.jsii.Kernel.call(this, "addRoleMappings", software.amazon.jsii.NativeType.VOID, java.util.Arrays.