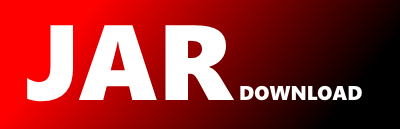
software.amazon.awscdk.services.cognito.identitypool.IdentityPoolProps Maven / Gradle / Ivy
Show all versions of cognito-identitypool Show documentation
package software.amazon.awscdk.services.cognito.identitypool;
/**
* (experimental) Props for the IdentityPool construct.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.cognito.identitypool.*;
* import software.amazon.awscdk.services.iam.*;
* IdentityPoolProviderUrl identityPoolProviderUrl;
* OpenIdConnectProvider openIdConnectProvider;
* Role role;
* SamlProvider samlProvider;
* UserPoolAuthenticationProvider userPoolAuthenticationProvider;
* IdentityPoolProps identityPoolProps = IdentityPoolProps.builder()
* .allowClassicFlow(false)
* .allowUnauthenticatedIdentities(false)
* .authenticatedRole(role)
* .authenticationProviders(IdentityPoolAuthenticationProviders.builder()
* .amazon(IdentityPoolAmazonLoginProvider.builder()
* .appId("appId")
* .build())
* .apple(IdentityPoolAppleLoginProvider.builder()
* .servicesId("servicesId")
* .build())
* .customProvider("customProvider")
* .digits(IdentityPoolDigitsLoginProvider.builder()
* .consumerKey("consumerKey")
* .consumerSecret("consumerSecret")
* .build())
* .facebook(IdentityPoolFacebookLoginProvider.builder()
* .appId("appId")
* .build())
* .google(IdentityPoolGoogleLoginProvider.builder()
* .clientId("clientId")
* .build())
* .openIdConnectProviders(List.of(openIdConnectProvider))
* .samlProviders(List.of(samlProvider))
* .twitter(IdentityPoolTwitterLoginProvider.builder()
* .consumerKey("consumerKey")
* .consumerSecret("consumerSecret")
* .build())
* .userPools(List.of(userPoolAuthenticationProvider))
* .build())
* .identityPoolName("identityPoolName")
* .roleMappings(List.of(IdentityPoolRoleMapping.builder()
* .providerUrl(identityPoolProviderUrl)
* // the properties below are optional
* .resolveAmbiguousRoles(false)
* .rules(List.of(RoleMappingRule.builder()
* .claim("claim")
* .claimValue("claimValue")
* .mappedRole(role)
* // the properties below are optional
* .matchType(RoleMappingMatchType.EQUALS)
* .build()))
* .useToken(false)
* .build()))
* .unauthenticatedRole(role)
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.52.1 (build 5ccc8f6)", date = "2022-01-27T11:49:00.787Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.cognito.identitypool.$Module.class, fqn = "@aws-cdk/aws-cognito-identitypool.IdentityPoolProps")
@software.amazon.jsii.Jsii.Proxy(IdentityPoolProps.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public interface IdentityPoolProps extends software.amazon.jsii.JsiiSerializable {
/**
* (experimental) Enables the Basic (Classic) authentication flow.
*
* Default: - Classic Flow not allowed
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.Boolean getAllowClassicFlow() {
return null;
}
/**
* (experimental) Wwhether the identity pool supports unauthenticated logins.
*
* Default: - false
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.Boolean getAllowUnauthenticatedIdentities() {
return null;
}
/**
* (experimental) The Default Role to be assumed by Authenticated Users.
*
* Default: - A Default Authenticated Role will be added
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.iam.IRole getAuthenticatedRole() {
return null;
}
/**
* (experimental) Authentication providers for using in identity pool.
*
* Default: - No Authentication Providers passed directly to Identity Pool
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.cognito.identitypool.IdentityPoolAuthenticationProviders getAuthenticationProviders() {
return null;
}
/**
* (experimental) The name of the Identity Pool.
*
* Default: - automatically generated name by CloudFormation at deploy time
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.lang.String getIdentityPoolName() {
return null;
}
/**
* (experimental) Rules for mapping roles to users.
*
* Default: - no Role Mappings
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable java.util.List getRoleMappings() {
return null;
}
/**
* (experimental) The Default Role to be assumed by Unauthenticated Users.
*
* Default: - A Default Unauthenticated Role will be added
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.iam.IRole getUnauthenticatedRole() {
return null;
}
/**
* @return a {@link Builder} of {@link IdentityPoolProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link IdentityPoolProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static final class Builder implements software.amazon.jsii.Builder {
java.lang.Boolean allowClassicFlow;
java.lang.Boolean allowUnauthenticatedIdentities;
software.amazon.awscdk.services.iam.IRole authenticatedRole;
software.amazon.awscdk.services.cognito.identitypool.IdentityPoolAuthenticationProviders authenticationProviders;
java.lang.String identityPoolName;
java.util.List roleMappings;
software.amazon.awscdk.services.iam.IRole unauthenticatedRole;
/**
* Sets the value of {@link IdentityPoolProps#getAllowClassicFlow}
* @param allowClassicFlow Enables the Basic (Classic) authentication flow.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder allowClassicFlow(java.lang.Boolean allowClassicFlow) {
this.allowClassicFlow = allowClassicFlow;
return this;
}
/**
* Sets the value of {@link IdentityPoolProps#getAllowUnauthenticatedIdentities}
* @param allowUnauthenticatedIdentities Wwhether the identity pool supports unauthenticated logins.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder allowUnauthenticatedIdentities(java.lang.Boolean allowUnauthenticatedIdentities) {
this.allowUnauthenticatedIdentities = allowUnauthenticatedIdentities;
return this;
}
/**
* Sets the value of {@link IdentityPoolProps#getAuthenticatedRole}
* @param authenticatedRole The Default Role to be assumed by Authenticated Users.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder authenticatedRole(software.amazon.awscdk.services.iam.IRole authenticatedRole) {
this.authenticatedRole = authenticatedRole;
return this;
}
/**
* Sets the value of {@link IdentityPoolProps#getAuthenticationProviders}
* @param authenticationProviders Authentication providers for using in identity pool.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder authenticationProviders(software.amazon.awscdk.services.cognito.identitypool.IdentityPoolAuthenticationProviders authenticationProviders) {
this.authenticationProviders = authenticationProviders;
return this;
}
/**
* Sets the value of {@link IdentityPoolProps#getIdentityPoolName}
* @param identityPoolName The name of the Identity Pool.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder identityPoolName(java.lang.String identityPoolName) {
this.identityPoolName = identityPoolName;
return this;
}
/**
* Sets the value of {@link IdentityPoolProps#getRoleMappings}
* @param roleMappings Rules for mapping roles to users.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@SuppressWarnings("unchecked")
public Builder roleMappings(java.util.List extends software.amazon.awscdk.services.cognito.identitypool.IdentityPoolRoleMapping> roleMappings) {
this.roleMappings = (java.util.List)roleMappings;
return this;
}
/**
* Sets the value of {@link IdentityPoolProps#getUnauthenticatedRole}
* @param unauthenticatedRole The Default Role to be assumed by Unauthenticated Users.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder unauthenticatedRole(software.amazon.awscdk.services.iam.IRole unauthenticatedRole) {
this.unauthenticatedRole = unauthenticatedRole;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link IdentityPoolProps}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@Override
public IdentityPoolProps build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link IdentityPoolProps}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements IdentityPoolProps {
private final java.lang.Boolean allowClassicFlow;
private final java.lang.Boolean allowUnauthenticatedIdentities;
private final software.amazon.awscdk.services.iam.IRole authenticatedRole;
private final software.amazon.awscdk.services.cognito.identitypool.IdentityPoolAuthenticationProviders authenticationProviders;
private final java.lang.String identityPoolName;
private final java.util.List roleMappings;
private final software.amazon.awscdk.services.iam.IRole unauthenticatedRole;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.allowClassicFlow = software.amazon.jsii.Kernel.get(this, "allowClassicFlow", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.allowUnauthenticatedIdentities = software.amazon.jsii.Kernel.get(this, "allowUnauthenticatedIdentities", software.amazon.jsii.NativeType.forClass(java.lang.Boolean.class));
this.authenticatedRole = software.amazon.jsii.Kernel.get(this, "authenticatedRole", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.iam.IRole.class));
this.authenticationProviders = software.amazon.jsii.Kernel.get(this, "authenticationProviders", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.cognito.identitypool.IdentityPoolAuthenticationProviders.class));
this.identityPoolName = software.amazon.jsii.Kernel.get(this, "identityPoolName", software.amazon.jsii.NativeType.forClass(java.lang.String.class));
this.roleMappings = software.amazon.jsii.Kernel.get(this, "roleMappings", software.amazon.jsii.NativeType.listOf(software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.cognito.identitypool.IdentityPoolRoleMapping.class)));
this.unauthenticatedRole = software.amazon.jsii.Kernel.get(this, "unauthenticatedRole", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.iam.IRole.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
@SuppressWarnings("unchecked")
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.allowClassicFlow = builder.allowClassicFlow;
this.allowUnauthenticatedIdentities = builder.allowUnauthenticatedIdentities;
this.authenticatedRole = builder.authenticatedRole;
this.authenticationProviders = builder.authenticationProviders;
this.identityPoolName = builder.identityPoolName;
this.roleMappings = (java.util.List)builder.roleMappings;
this.unauthenticatedRole = builder.unauthenticatedRole;
}
@Override
public final java.lang.Boolean getAllowClassicFlow() {
return this.allowClassicFlow;
}
@Override
public final java.lang.Boolean getAllowUnauthenticatedIdentities() {
return this.allowUnauthenticatedIdentities;
}
@Override
public final software.amazon.awscdk.services.iam.IRole getAuthenticatedRole() {
return this.authenticatedRole;
}
@Override
public final software.amazon.awscdk.services.cognito.identitypool.IdentityPoolAuthenticationProviders getAuthenticationProviders() {
return this.authenticationProviders;
}
@Override
public final java.lang.String getIdentityPoolName() {
return this.identityPoolName;
}
@Override
public final java.util.List getRoleMappings() {
return this.roleMappings;
}
@Override
public final software.amazon.awscdk.services.iam.IRole getUnauthenticatedRole() {
return this.unauthenticatedRole;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getAllowClassicFlow() != null) {
data.set("allowClassicFlow", om.valueToTree(this.getAllowClassicFlow()));
}
if (this.getAllowUnauthenticatedIdentities() != null) {
data.set("allowUnauthenticatedIdentities", om.valueToTree(this.getAllowUnauthenticatedIdentities()));
}
if (this.getAuthenticatedRole() != null) {
data.set("authenticatedRole", om.valueToTree(this.getAuthenticatedRole()));
}
if (this.getAuthenticationProviders() != null) {
data.set("authenticationProviders", om.valueToTree(this.getAuthenticationProviders()));
}
if (this.getIdentityPoolName() != null) {
data.set("identityPoolName", om.valueToTree(this.getIdentityPoolName()));
}
if (this.getRoleMappings() != null) {
data.set("roleMappings", om.valueToTree(this.getRoleMappings()));
}
if (this.getUnauthenticatedRole() != null) {
data.set("unauthenticatedRole", om.valueToTree(this.getUnauthenticatedRole()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-cognito-identitypool.IdentityPoolProps"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
IdentityPoolProps.Jsii$Proxy that = (IdentityPoolProps.Jsii$Proxy) o;
if (this.allowClassicFlow != null ? !this.allowClassicFlow.equals(that.allowClassicFlow) : that.allowClassicFlow != null) return false;
if (this.allowUnauthenticatedIdentities != null ? !this.allowUnauthenticatedIdentities.equals(that.allowUnauthenticatedIdentities) : that.allowUnauthenticatedIdentities != null) return false;
if (this.authenticatedRole != null ? !this.authenticatedRole.equals(that.authenticatedRole) : that.authenticatedRole != null) return false;
if (this.authenticationProviders != null ? !this.authenticationProviders.equals(that.authenticationProviders) : that.authenticationProviders != null) return false;
if (this.identityPoolName != null ? !this.identityPoolName.equals(that.identityPoolName) : that.identityPoolName != null) return false;
if (this.roleMappings != null ? !this.roleMappings.equals(that.roleMappings) : that.roleMappings != null) return false;
return this.unauthenticatedRole != null ? this.unauthenticatedRole.equals(that.unauthenticatedRole) : that.unauthenticatedRole == null;
}
@Override
public final int hashCode() {
int result = this.allowClassicFlow != null ? this.allowClassicFlow.hashCode() : 0;
result = 31 * result + (this.allowUnauthenticatedIdentities != null ? this.allowUnauthenticatedIdentities.hashCode() : 0);
result = 31 * result + (this.authenticatedRole != null ? this.authenticatedRole.hashCode() : 0);
result = 31 * result + (this.authenticationProviders != null ? this.authenticationProviders.hashCode() : 0);
result = 31 * result + (this.identityPoolName != null ? this.identityPoolName.hashCode() : 0);
result = 31 * result + (this.roleMappings != null ? this.roleMappings.hashCode() : 0);
result = 31 * result + (this.unauthenticatedRole != null ? this.unauthenticatedRole.hashCode() : 0);
return result;
}
}
}