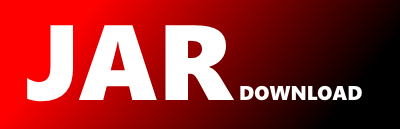
software.amazon.awscdk.services.cognito.identitypool.IdentityPoolProviders Maven / Gradle / Ivy
Show all versions of cognito-identitypool Show documentation
package software.amazon.awscdk.services.cognito.identitypool;
/**
* (experimental) External Identity Providers To Connect to User Pools and Identity Pools.
*
* Example:
*
*
* // The code below shows an example of how to instantiate this type.
* // The values are placeholders you should change.
* import software.amazon.awscdk.services.cognito.identitypool.*;
* IdentityPoolProviders identityPoolProviders = IdentityPoolProviders.builder()
* .amazon(IdentityPoolAmazonLoginProvider.builder()
* .appId("appId")
* .build())
* .apple(IdentityPoolAppleLoginProvider.builder()
* .servicesId("servicesId")
* .build())
* .digits(IdentityPoolDigitsLoginProvider.builder()
* .consumerKey("consumerKey")
* .consumerSecret("consumerSecret")
* .build())
* .facebook(IdentityPoolFacebookLoginProvider.builder()
* .appId("appId")
* .build())
* .google(IdentityPoolGoogleLoginProvider.builder()
* .clientId("clientId")
* .build())
* .twitter(IdentityPoolTwitterLoginProvider.builder()
* .consumerKey("consumerKey")
* .consumerSecret("consumerSecret")
* .build())
* .build();
*
*/
@javax.annotation.Generated(value = "jsii-pacmak/1.52.1 (build 5ccc8f6)", date = "2022-01-27T11:49:00.789Z")
@software.amazon.jsii.Jsii(module = software.amazon.awscdk.services.cognito.identitypool.$Module.class, fqn = "@aws-cdk/aws-cognito-identitypool.IdentityPoolProviders")
@software.amazon.jsii.Jsii.Proxy(IdentityPoolProviders.Jsii$Proxy.class)
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public interface IdentityPoolProviders extends software.amazon.jsii.JsiiSerializable {
/**
* (experimental) App Id for Amazon Identity Federation.
*
* Default: - No Amazon Authentication Provider used without OpenIdConnect or a User Pool
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.cognito.identitypool.IdentityPoolAmazonLoginProvider getAmazon() {
return null;
}
/**
* (experimental) Services Id for Apple Identity Federation.
*
* Default: - No Apple Authentication Provider used without OpenIdConnect or a User Pool
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.cognito.identitypool.IdentityPoolAppleLoginProvider getApple() {
return null;
}
/**
* (experimental) Consumer Key and Secret for Digits Identity Federation.
*
* Default: - No Digits Authentication Provider used without OpenIdConnect or a User Pool
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.cognito.identitypool.IdentityPoolDigitsLoginProvider getDigits() {
return null;
}
/**
* (experimental) App Id for Facebook Identity Federation.
*
* Default: - No Facebook Authentication Provider used without OpenIdConnect or a User Pool
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.cognito.identitypool.IdentityPoolFacebookLoginProvider getFacebook() {
return null;
}
/**
* (experimental) Client Id for Google Identity Federation.
*
* Default: - No Google Authentication Provider used without OpenIdConnect or a User Pool
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.cognito.identitypool.IdentityPoolGoogleLoginProvider getGoogle() {
return null;
}
/**
* (experimental) Consumer Key and Secret for Twitter Identity Federation.
*
* Default: - No Twitter Authentication Provider used without OpenIdConnect or a User Pool
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
default @org.jetbrains.annotations.Nullable software.amazon.awscdk.services.cognito.identitypool.IdentityPoolTwitterLoginProvider getTwitter() {
return null;
}
/**
* @return a {@link Builder} of {@link IdentityPoolProviders}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
static Builder builder() {
return new Builder();
}
/**
* A builder for {@link IdentityPoolProviders}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public static final class Builder implements software.amazon.jsii.Builder {
software.amazon.awscdk.services.cognito.identitypool.IdentityPoolAmazonLoginProvider amazon;
software.amazon.awscdk.services.cognito.identitypool.IdentityPoolAppleLoginProvider apple;
software.amazon.awscdk.services.cognito.identitypool.IdentityPoolDigitsLoginProvider digits;
software.amazon.awscdk.services.cognito.identitypool.IdentityPoolFacebookLoginProvider facebook;
software.amazon.awscdk.services.cognito.identitypool.IdentityPoolGoogleLoginProvider google;
software.amazon.awscdk.services.cognito.identitypool.IdentityPoolTwitterLoginProvider twitter;
/**
* Sets the value of {@link IdentityPoolProviders#getAmazon}
* @param amazon App Id for Amazon Identity Federation.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder amazon(software.amazon.awscdk.services.cognito.identitypool.IdentityPoolAmazonLoginProvider amazon) {
this.amazon = amazon;
return this;
}
/**
* Sets the value of {@link IdentityPoolProviders#getApple}
* @param apple Services Id for Apple Identity Federation.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder apple(software.amazon.awscdk.services.cognito.identitypool.IdentityPoolAppleLoginProvider apple) {
this.apple = apple;
return this;
}
/**
* Sets the value of {@link IdentityPoolProviders#getDigits}
* @param digits Consumer Key and Secret for Digits Identity Federation.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder digits(software.amazon.awscdk.services.cognito.identitypool.IdentityPoolDigitsLoginProvider digits) {
this.digits = digits;
return this;
}
/**
* Sets the value of {@link IdentityPoolProviders#getFacebook}
* @param facebook App Id for Facebook Identity Federation.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder facebook(software.amazon.awscdk.services.cognito.identitypool.IdentityPoolFacebookLoginProvider facebook) {
this.facebook = facebook;
return this;
}
/**
* Sets the value of {@link IdentityPoolProviders#getGoogle}
* @param google Client Id for Google Identity Federation.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder google(software.amazon.awscdk.services.cognito.identitypool.IdentityPoolGoogleLoginProvider google) {
this.google = google;
return this;
}
/**
* Sets the value of {@link IdentityPoolProviders#getTwitter}
* @param twitter Consumer Key and Secret for Twitter Identity Federation.
* @return {@code this}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
public Builder twitter(software.amazon.awscdk.services.cognito.identitypool.IdentityPoolTwitterLoginProvider twitter) {
this.twitter = twitter;
return this;
}
/**
* Builds the configured instance.
* @return a new instance of {@link IdentityPoolProviders}
* @throws NullPointerException if any required attribute was not provided
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@Override
public IdentityPoolProviders build() {
return new Jsii$Proxy(this);
}
}
/**
* An implementation for {@link IdentityPoolProviders}
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
@software.amazon.jsii.Internal
final class Jsii$Proxy extends software.amazon.jsii.JsiiObject implements IdentityPoolProviders {
private final software.amazon.awscdk.services.cognito.identitypool.IdentityPoolAmazonLoginProvider amazon;
private final software.amazon.awscdk.services.cognito.identitypool.IdentityPoolAppleLoginProvider apple;
private final software.amazon.awscdk.services.cognito.identitypool.IdentityPoolDigitsLoginProvider digits;
private final software.amazon.awscdk.services.cognito.identitypool.IdentityPoolFacebookLoginProvider facebook;
private final software.amazon.awscdk.services.cognito.identitypool.IdentityPoolGoogleLoginProvider google;
private final software.amazon.awscdk.services.cognito.identitypool.IdentityPoolTwitterLoginProvider twitter;
/**
* Constructor that initializes the object based on values retrieved from the JsiiObject.
* @param objRef Reference to the JSII managed object.
*/
protected Jsii$Proxy(final software.amazon.jsii.JsiiObjectRef objRef) {
super(objRef);
this.amazon = software.amazon.jsii.Kernel.get(this, "amazon", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.cognito.identitypool.IdentityPoolAmazonLoginProvider.class));
this.apple = software.amazon.jsii.Kernel.get(this, "apple", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.cognito.identitypool.IdentityPoolAppleLoginProvider.class));
this.digits = software.amazon.jsii.Kernel.get(this, "digits", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.cognito.identitypool.IdentityPoolDigitsLoginProvider.class));
this.facebook = software.amazon.jsii.Kernel.get(this, "facebook", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.cognito.identitypool.IdentityPoolFacebookLoginProvider.class));
this.google = software.amazon.jsii.Kernel.get(this, "google", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.cognito.identitypool.IdentityPoolGoogleLoginProvider.class));
this.twitter = software.amazon.jsii.Kernel.get(this, "twitter", software.amazon.jsii.NativeType.forClass(software.amazon.awscdk.services.cognito.identitypool.IdentityPoolTwitterLoginProvider.class));
}
/**
* Constructor that initializes the object based on literal property values passed by the {@link Builder}.
*/
protected Jsii$Proxy(final Builder builder) {
super(software.amazon.jsii.JsiiObject.InitializationMode.JSII);
this.amazon = builder.amazon;
this.apple = builder.apple;
this.digits = builder.digits;
this.facebook = builder.facebook;
this.google = builder.google;
this.twitter = builder.twitter;
}
@Override
public final software.amazon.awscdk.services.cognito.identitypool.IdentityPoolAmazonLoginProvider getAmazon() {
return this.amazon;
}
@Override
public final software.amazon.awscdk.services.cognito.identitypool.IdentityPoolAppleLoginProvider getApple() {
return this.apple;
}
@Override
public final software.amazon.awscdk.services.cognito.identitypool.IdentityPoolDigitsLoginProvider getDigits() {
return this.digits;
}
@Override
public final software.amazon.awscdk.services.cognito.identitypool.IdentityPoolFacebookLoginProvider getFacebook() {
return this.facebook;
}
@Override
public final software.amazon.awscdk.services.cognito.identitypool.IdentityPoolGoogleLoginProvider getGoogle() {
return this.google;
}
@Override
public final software.amazon.awscdk.services.cognito.identitypool.IdentityPoolTwitterLoginProvider getTwitter() {
return this.twitter;
}
@Override
@software.amazon.jsii.Internal
public com.fasterxml.jackson.databind.JsonNode $jsii$toJson() {
final com.fasterxml.jackson.databind.ObjectMapper om = software.amazon.jsii.JsiiObjectMapper.INSTANCE;
final com.fasterxml.jackson.databind.node.ObjectNode data = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
if (this.getAmazon() != null) {
data.set("amazon", om.valueToTree(this.getAmazon()));
}
if (this.getApple() != null) {
data.set("apple", om.valueToTree(this.getApple()));
}
if (this.getDigits() != null) {
data.set("digits", om.valueToTree(this.getDigits()));
}
if (this.getFacebook() != null) {
data.set("facebook", om.valueToTree(this.getFacebook()));
}
if (this.getGoogle() != null) {
data.set("google", om.valueToTree(this.getGoogle()));
}
if (this.getTwitter() != null) {
data.set("twitter", om.valueToTree(this.getTwitter()));
}
final com.fasterxml.jackson.databind.node.ObjectNode struct = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
struct.set("fqn", om.valueToTree("@aws-cdk/aws-cognito-identitypool.IdentityPoolProviders"));
struct.set("data", data);
final com.fasterxml.jackson.databind.node.ObjectNode obj = com.fasterxml.jackson.databind.node.JsonNodeFactory.instance.objectNode();
obj.set("$jsii.struct", struct);
return obj;
}
@Override
public final boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
IdentityPoolProviders.Jsii$Proxy that = (IdentityPoolProviders.Jsii$Proxy) o;
if (this.amazon != null ? !this.amazon.equals(that.amazon) : that.amazon != null) return false;
if (this.apple != null ? !this.apple.equals(that.apple) : that.apple != null) return false;
if (this.digits != null ? !this.digits.equals(that.digits) : that.digits != null) return false;
if (this.facebook != null ? !this.facebook.equals(that.facebook) : that.facebook != null) return false;
if (this.google != null ? !this.google.equals(that.google) : that.google != null) return false;
return this.twitter != null ? this.twitter.equals(that.twitter) : that.twitter == null;
}
@Override
public final int hashCode() {
int result = this.amazon != null ? this.amazon.hashCode() : 0;
result = 31 * result + (this.apple != null ? this.apple.hashCode() : 0);
result = 31 * result + (this.digits != null ? this.digits.hashCode() : 0);
result = 31 * result + (this.facebook != null ? this.facebook.hashCode() : 0);
result = 31 * result + (this.google != null ? this.google.hashCode() : 0);
result = 31 * result + (this.twitter != null ? this.twitter.hashCode() : 0);
return result;
}
}
}