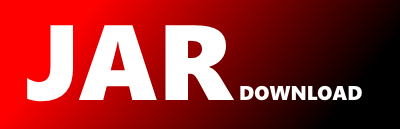
software.amazon.awscdk.services.cognito.identitypool.package-info Maven / Gradle / Ivy
Show all versions of cognito-identitypool Show documentation
/**
* Amazon Cognito Identity Pool Construct Library
*
*
*
* Identity Pools are in a separate module while the API is being stabilized. Once we stabilize the module, they will
* be included into the stable aws-cognito library. Please provide feedback on this experience by
* creating an issue here
*
*
---
*
*
*
*
*
* The APIs of higher level constructs in this module are experimental and under active development.
* They are subject to non-backward compatible changes or removal in any future version. These are
* not subject to the Semantic Versioning model and breaking changes will be
* announced in the release notes. This means that while you may use them, you may need to update
* your source code when upgrading to a newer version of this package.
*
*
*
*
*
*
*
* Amazon Cognito Identity Pools enable you to grant your users access to other AWS services.
*
* Identity Pools are one of the two main components of Amazon Cognito, which provides authentication, authorization, and
* user management for your web and mobile apps. Your users can sign in directly with a user name and password, or through
* a third party such as Facebook, Amazon, Google or Apple.
*
* The other main component in Amazon Cognito is user pools. User Pools are user directories that provide sign-up and
* sign-in options for your app users.
*
* This module is part of the AWS Cloud Development Kit project.
*
*
Table of Contents
*
*
* - Identity Pools
*
*
*
*
*
Identity Pools
*
* Identity pools provide temporary AWS credentials for users who are guests (unauthenticated) and for users who have been
* authenticated and received a token. An identity pool is a store of user identity data specific to an account.
*
* Identity pools can be used in conjunction with Cognito User Pools or by accessing external federated identity providers
* directly. Learn more at Amazon Cognito Identity Pools.
*
*
Authenticated and Unauthenticated Identities
*
* Identity pools define two types of identities: authenticated(user
) and unauthenticated (guest
). Every identity in
* an identity pool is either authenticated or unauthenticated. Each identity pool has a default role for authenticated
* identities, and a default role for unauthenticated identities. Absent other overriding rules (see below), these are the
* roles that will be assumed by the corresponding users in the authentication process.
*
* A basic Identity Pool with minimal configuration has no required props, with default authenticated (user) and
* unauthenticated (guest) roles applied to the identity pool:
*
*
* // Example automatically generated from non-compiling source. May contain errors.
* new IdentityPool(this, "myIdentityPool");
*
*
* By default, both the authenticated and unauthenticated roles will have no permissions attached. Grant permissions
* to roles using the public authenticatedRole
and unauthenticatedRole
properties:
*
*
* // Example automatically generated from non-compiling source. May contain errors.
* Object identityPool = new IdentityPool(this, "myIdentityPool");
* Object table = new Table(this, "MyTable");
*
* // Grant permissions to authenticated users
* table.grantReadWriteData(identityPool.getAuthenticatedRole());
* // Grant permissions to unauthenticated guest users
* table.grantRead(identityPool.getUnauthenticatedRole());
*
* //Or add policy statements straight to the role
* identityPool.authenticatedRole.addToPrincipalPolicy(PolicyStatement.Builder.create()
* .effect(Effect.getALLOW())
* .actions(List.of("dynamodb:*"))
* .resources(List.of("*"))
* .build());
*
*
* The default roles can also be supplied in IdentityPoolProps
:
*
*
* // Example automatically generated from non-compiling source. May contain errors.
* Object stack = new Stack();
* Object authenticatedRole = Role.Builder.create(stack, "authRole")
* .assumedBy(new ServicePrincipal("service.amazonaws.com"))
* .build();
* Object unauthenticatedRole = Role.Builder.create(stack, "unauthRole")
* .assumedBy(new ServicePrincipal("service.amazonaws.com"))
* .build();
* Object identityPool = IdentityPool.Builder.create(stack, "TestIdentityPoolActions")
* .authenticatedRole(authenticatedRole).unauthenticatedRole(unauthenticatedRole)
* .build();
*
*
*
Authentication Providers
*
* Authenticated identities belong to users who are authenticated by a public login provider (Amazon Cognito user pools,
* Login with Amazon, Sign in with Apple, Facebook, Google, SAML, or any OpenID Connect Providers) or a developer provider
* (your own backend authentication process).
*
* Authentication providers can be associated with an Identity Pool by first associating them with a Cognito User Pool or by
* associating the provider directly with the identity pool.
*
*
User Pool Authentication Provider
*
* In order to attach a user pool to an identity pool as an authentication provider, the identity pool needs properties
* from both the user pool and the user pool client. For this reason identity pools use a UserPoolAuthenticationProvider
* to gather the necessary properties from the user pool constructs.
*
*
* // Example automatically generated from non-compiling source. May contain errors.
* Object userPool = new UserPool(this, "Pool");
*
* IdentityPool.Builder.create(this, "myidentitypool")
* .identityPoolName("myidentitypool")
* .authenticationProviders(Map.of(
* "userPools", List.of(UserPoolAuthenticationProvider.Builder.create().userPool(userPool).build())))
* .build();
*
*
* User pools can also be associated with an identity pool after instantiation. The Identity Pool's addUserPoolAuthentication
method
* returns the User Pool Client that has been created:
*
*
* // Example automatically generated from non-compiling source. May contain errors.
* Object userPool = new UserPool(this, "Pool");
* Object userPoolClient = identityPool.addUserPoolAuthentication(Map.of(
* "userPools", List.of(UserPoolAuthenticationProvider.Builder.create().userPool(userPool).build())));
*
*
*
Server Side Token Check
*
* With the IdentityPool
CDK Construct, by default the pool is configured to check with the integrated user pools to
* make sure that the user has not been globally signed out or deleted before the identity pool provides an OIDC token or
* AWS credentials for the user.
*
* If the user is signed out or deleted, the identity pool will return a 400 Not Authorized error. This setting can be
* disabled, however, in several ways.
*
* Setting disableServerSideTokenCheck
to true will change the default behavior to no server side token check. Learn
* more here:
*
*
* // Example automatically generated from non-compiling source. May contain errors.
* Object userPool = new UserPool(this, "Pool");
* identityPool.addUserPoolAuthentication(Map.of(
* "userPool", UserPoolAuthenticationProvider.Builder.create()
* .userPool(userPool)
* .disableServerSideTokenCheck(true)
* .build()));
*
*
*
Associating an External Provider Directly
*
* One or more external identity providers can be associated with an identity pool directly using
* authenticationProviders
:
*
*
* // Example automatically generated from non-compiling source. May contain errors.
* IdentityPool.Builder.create(this, "myidentitypool")
* .identityPoolName("myidentitypool")
* .authenticationProviders(Map.of(
* "amazon", Map.of(
* "appId", "amzn1.application.12312k3j234j13rjiwuenf"),
* "facebook", Map.of(
* "appId", "1234567890123"),
* "google", Map.of(
* "clientId", "12345678012.apps.googleusercontent.com"),
* "apple", Map.of(
* "servicesId", "com.myappleapp.auth"),
* "twitter", Map.of(
* "consumerKey", "my-twitter-id",
* "consumerSecret", "my-twitter-secret")))
* .build();
*
*
* To associate more than one provider of the same type with the identity pool, use User
* Pools, OpenIdConnect, or SAML. Only one provider per external service can be attached directly to the identity pool.
*
*
OpenId Connect and Saml
*
* OpenID Connect is an open standard for
* authentication that is supported by a number of login providers. Amazon Cognito supports linking of identities with
* OpenID Connect providers that are configured through AWS Identity and Access Management.
*
* An identity provider that supports Security Assertion Markup Language 2.0 (SAML 2.0) can be used to provide a simple
* onboarding flow for users. The SAML-supporting identity provider specifies the IAM roles that can be assumed by users
* so that different users can be granted different sets of permissions. Associating an OpenId Connect or Saml provider
* with an identity pool:
*
*
* // Example automatically generated from non-compiling source. May contain errors.
* Object openIdConnectProvider = new OpenIdConnectProvider(this, "my-openid-connect-provider", ...);
* Object samlProvider = new SamlProvider(this, "my-saml-provider", ...);
*
* IdentityPool.Builder.create(this, "myidentitypool")
* .identityPoolName("myidentitypool")
* .authenticationProviders(Map.of(
* "openIdConnectProvider", openIdConnectProvider,
* "samlProvider", samlProvider))
* .build();
*
*
*
Custom Providers
*
* The identity pool's behavior can be customized further using custom developer authenticated identities.
* With developer authenticated identities, users can be registered and authenticated via an existing authentication
* process while still using Amazon Cognito to synchronize user data and access AWS resources.
*
* Like the supported external providers, though, only one custom provider can be directly associated with the identity
* pool.
*
*
* // Example automatically generated from non-compiling source. May contain errors.
* IdentityPool.Builder.create(this, "myidentitypool")
* .identityPoolName("myidentitypool")
* .authenticationProviders(Map.of(
* "google", "12345678012.apps.googleusercontent.com",
* "openIdConnectProvider", openIdConnectProvider,
* "customProvider", "my-custom-provider.example.com"))
* .build();
*
*
*
Role Mapping
*
* In addition to setting default roles for authenticated and unauthenticated users, identity pools can also be used to
* define rules to choose the role for each user based on claims in the user's ID token by using Role Mapping. When using
* role mapping, it's important to be aware of some of the permissions the role will need. An in depth
* review of roles and role mapping can be found here.
*
* Using a token-based approach to role mapping will allow mapped roles to be passed through the cognito:roles
or
* cognito:preferred_role
claims from the identity provider:
*
*
* // Example automatically generated from non-compiling source. May contain errors.
* IdentityPool.Builder.create(this, "myidentitypool")
* .identityPoolName("myidentitypool")
* .roleMappings(List.of(Map.of(
* "providerUrl", IdentityPoolProviderUrl.getAMAZON(),
* "useToken", true)))
* .build();
*
*
* Using a rule-based approach to role mapping allows roles to be assigned based on custom claims passed from the identity provider:
*
*
* // Example automatically generated from non-compiling source. May contain errors.
* IdentityPool.Builder.create(this, "myidentitypool")
* .identityPoolName("myidentitypool")
* // Assign specific roles to users based on whether or not the custom admin claim is passed from the identity provider
* .roleMappings(List.of(Map.of(
* "providerUrl", IdentityPoolProviderUrl.getAMAZON(),
* "rules", List.of(Map.of(
* "claim", "custom:admin",
* "claimValue", "admin",
* "mappedRole", adminRole), Map.of(
* "claim", "custom:admin",
* "claimValue", "admin",
* "matchType", RoleMappingMatchType.getNOTEQUAL(),
* "mappedRole", nonAdminRole)))))
* .build();
*
*
* Role mappings can also be added after instantiation with the Identity Pool's addRoleMappings
method:
*
*
* // Example automatically generated from non-compiling source. May contain errors.
* identityPool.addRoleMappings(myAddedRoleMapping1, myAddedRoleMapping2, myAddedRoleMapping3);
*
*
*
Provider Urls
*
* Role mappings must be associated with the url of an Identity Provider which can be supplied
* IdentityPoolProviderUrl
. Supported Providers have static Urls that can be used:
*
*
* // Example automatically generated from non-compiling source. May contain errors.
* IdentityPool.Builder.create(this, "myidentitypool")
* .identityPoolName("myidentitypool")
* .roleMappings(List.of(Map.of(
* "providerUrl", IdentityPoolProviderUrl.getFACEBOOK(),
* "useToken", true)))
* .build();
*
*
* For identity providers that don't have static Urls, a custom Url or User Pool Client Url can be supplied:
*
*
* // Example automatically generated from non-compiling source. May contain errors.
* IdentityPool.Builder.create(this, "myidentitypool")
* .identityPoolName("myidentitypool")
* .roleMappings(List.of(Map.of(
* "providerUrl", IdentityPoolProviderUrl.userPool("cognito-idp.my-idp-region.amazonaws.com/my-idp-region_abcdefghi:app_client_id"),
* "useToken", true), Map.of(
* "providerUrl", IdentityPoolProviderUrl.custom("my-custom-provider.com"),
* "useToken", true)))
* .build();
*
*
* See here for more information.
*
*
Authentication Flow
*
* Identity Pool Authentication Flow defaults to the enhanced, simplified flow. The Classic (basic) Authentication Flow
* can also be implemented using allowClassicFlow
:
*
*
* // Example automatically generated from non-compiling source. May contain errors.
* IdentityPool.Builder.create(this, "myidentitypool")
* .identityPoolName("myidentitypool")
* .allowClassicFlow(true)
* .build();
*
*
*
Cognito Sync
*
* It's now recommended to integrate AWS AppSync for synchronizing app data across devices, so
* Cognito Sync features like PushSync
, CognitoEvents
, and CognitoStreams
are not a part of IdentityPool
. More
* information can be found here.
*
*
Importing Identity Pools
*
* You can import existing identity pools into your stack using Identity Pool static methods with the Identity Pool Id or
* Arn:
*
*
* // Example automatically generated from non-compiling source. May contain errors.
* IdentityPool.fromIdentityPoolId(this, "my-imported-identity-pool", "us-east-1:dj2823ryiwuhef937");
* IdentityPool.fromIdentityPoolArn(this, "my-imported-identity-pool", "arn:aws:cognito-identity:us-east-1:123456789012:identitypool/us-east-1:dj2823ryiwuhef937");
*
*/
@software.amazon.jsii.Stability(software.amazon.jsii.Stability.Level.Experimental)
package software.amazon.awscdk.services.cognito.identitypool;